python tutorial - Interview questions on python scripting - learn python - python programming
python interview questions :21
How efficient/fast is Python's 'in'? (Time Complexity wise)
- In Python, what is the efficiency of the in keyword, such as in:
a = [1, 2, 3]
if 4 in a:
...
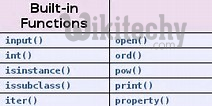
Learn python - python tutorial - complexity - python examples - python programs
python interview questions :22
How to deal with containing character codes?
- \201 is a character code recognised in Python. What is the best way to ignore this in strings.
s = '\2016'
s = s.replace('\\', '/')
print s #6
click below button to copy the code. By Python tutorial team
python interview questions :23
Which command do you use to exit help window or help command prompt?
- When you type quit at the help’s command prompt, python shell prompt will appear by closing the help window automatically.
python interview questions :24
Does the functions help() and dir() list the names of all the built_in functions and variables? If no, how would you list them?
- No. Built-in functions such as max(), min(), filter(), map(), etc is not apparent immediately as they are available as part of standard module.
- To view them, we can pass the module ” builtins ” as an argument to “dir()”. It will display thebuilt-in functions, exceptions, and other objects as a list.>>>dir(__builtins )
[‘ArithmeticError’, ‘AssertionError’, ‘AttributeError’, ……… ]
python interview questions :25
Explain how Python does Compile-time and Run-time code checking?
- Python performs some amount of compile-time checking, but most of them check type, name, etc are postponed until code execution. Consequently, if the Python code references a user -defined function that does not exist, the code will compile successfully.
- In fact, the code will fail with an exception only when the code execution path references the function which does not exists.
python interview questions :26
Whenever Python exists Why does all the memory is not de-allocated / freed when Python exits?
- Whenever Python exits, especially those python modules which are having circular references to other objects or the objects that are referenced from the global namespaces are not always de - allocated/freed/uncollectable.
- It is impossible to deallocate those portions of memory that are reserved by the C library.
- On exit, because of having its own efficient clean up mechanism, Python would try to deallocate/
- destroy every object.
python interview questions :27
Explain Python's zip() function.?
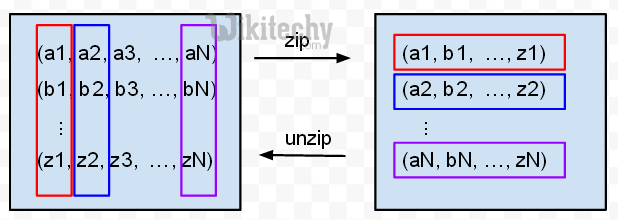
Learn python - python tutorial - efficient - python examples - python programs
- zip() function- it will take multiple lists say list1, list2, etc and transform them into a single list of tuples by taking the corresponding elements of the lists that are passed as parameters. Eg:
list1 = ['A',
'B','C'] and list2 = [10,20,30].
zip(list1, list2) # results in a list of tuples say [('A',10),('B',20),('C',30)]
click below button to copy the code. By Python tutorial team
- whenever the given lists are of different lengths, zip stops generating tuples when the first list ends.
python interview questions :28
Explain Python's pass by references Vs pass by value ?
- In Python, by default, all the parameters (arguments) are passed “by reference” to the functions. Thus, if you change the value of the parameter within a function, the change is reflected in the calling function.
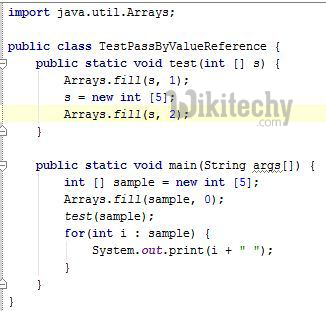
Learn python - python tutorial - strings - python examples - python programs
- We can even observe the pass “by value” kind of a behaviour whenever we pass the arguments to functions that are of type say numbers, strings, tuples. This is because of the immutable nature of them.
python interview questions :29
Explain the characteristics of Python's Objects ?
- As Python’s Objects are instances of classes, they are created at the time of instantiation. Eg: object-name = class-name(arguments)
- one or more variables can reference the same object in Python
- Every object holds unique id and it can be obtained by using id() method. Eg: id(obj-name) will return unique id of the given object.
- every object can be either mutable or immutable based on the type of data they hold.
- Whenever an object is not being used in the code, it gets destroyed automatically garbage collected or destroyed contents of objects can be converted into string representation using a method
python interview questions :30
Explain how to overload constructors or methods in Python ?
- Python’s constructor - _init__ () is a first method of a class. Whenever we try to instantiate a object __init__() is automatically invoked by python to initialize members of an object.