python tutorial - Python coding interview questions and answers - learn python - python programming
python interview questions :51
Find the first tuple in anything that is or might contain a tuple?
- If I actually needed something like this, I would do something like:
def first_tuple(t):
return t if isinstance(t,tuple) else next(x for x in t if isinstance(x,tuple))
click below button to copy the code. By Python tutorial team
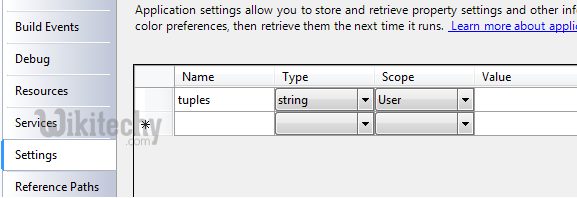
Learn python - python tutorial - tupule-settings - python examples - python programs
demo:
>>> first_tuple((3,5))
(3, 5)
>>> first_tuple([(3, 5), [200, 100, 100]])
(3, 5)
>>> first_tuple([[100, 100, 100], (3, 5)])
(3, 5)
>>> first_tuple([(3, 5), (4, 7)])
(3, 5)
>>> first_tuple([[],[]])
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
File "<stdin>", line 2, in first_tuple
StopIteration
click below button to copy the code. By Python tutorial team
- Generally speaking, you shouldn't need something like this. IMHO, this seems like it is probably a poor design and the data-structure here should be reconsidered.
python interview questions :52
python (init) with base classes?
It's been noted that in Python 3.0+ you can use
super().__init__()
click below button to copy the code. By Python tutorial team
- To make your call, which is concise and does not require you to reference the parent OR class names explicitly, which can be handy. I just want to add that for Python 2.7 or under, you can achieve the same name-insensitive approach by writing self.__class__ instead of the class name, i.e.
super(self.__class__, self).__init__()
click below button to copy the code. By Python tutorial team
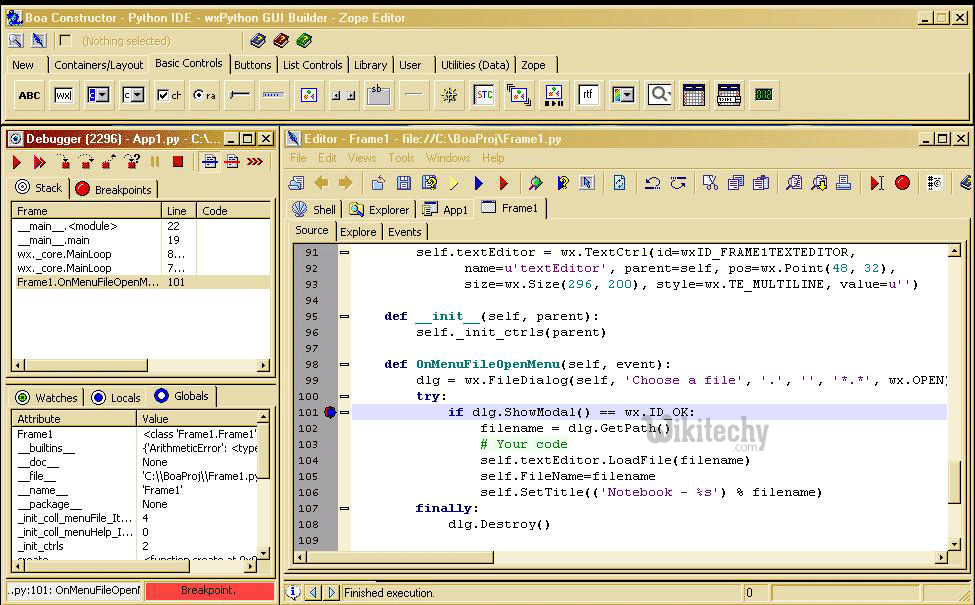
Learn python - python tutorial - python-ide - python examples - python programs
- However, this breaks if you use multiple levels of inheritance, where self.__class__ could return a child class --- meaning that neither you nor anyone else can inherit from a class that uses this pattern. For example:
class Polygon(object):
def __init__(self, id):
self.id = id
class Rectangle(Polygon):
def __init__(self, id, width, height):
super(self.__class__, self).__init__(id)
self.shape = (width, height)
class Square(Rectangle):
pass
click below button to copy the code. By Python tutorial team
- Here I have a class Square, which is a sub-class of Rectangle. Say I don't want to write a separate constructor for Square because the constructor for Rectangle is good enough, but for whatever reason I want to implement a Square so I can reimplement some other method.
- When I create a Square using
mSquare = Square('a', 10,10),
Python calls the constructor for Rectangle because I haven't given Square its own constructor. However, in the constructor for Rectangle, the callsuper(self.__class__,self)
is going to return the superclass of mSquare, so it calls the constructor for Rectangle again. This is how the infinite loop happens, as was mentioned by @S_C. In this case, when I runsuper(...).__init__()
I am calling the constructor for Rectangle but since I give it no arguments, I will get an error.
python interview questions :53
Python ctypes and char**?
- I use this in my code:
POINTER(POINTER(c_char))
click below button to copy the code. By Python tutorial team
- But I think both are equivalent.
Edit: Actually they are not http://docs.python.org/2/library/ctypes.html#ctypes.c_char_p
ctypes.c_char_p Represents the C char * datatype when it points to a zero-terminated string. For a general character pointer that may also point to binary data, POINTER(c_char) must be used. The constructor accepts an integer address, or a string.
- So
POINTER(POINTER(c_char))
is for binary data, andPOINTER(c_char_p)
is a pointer to a C null-terminated string.
python interview questions :54
Confusing […] List in Python: What is it?
- It can also appear if you have a circular structure with a list pointing to itself. Like this:
>>> a = [1,2]
>>> a.append(a)
>>> a
[1, 2, [...]]
>>>
click below button to copy the code. By Python tutorial team
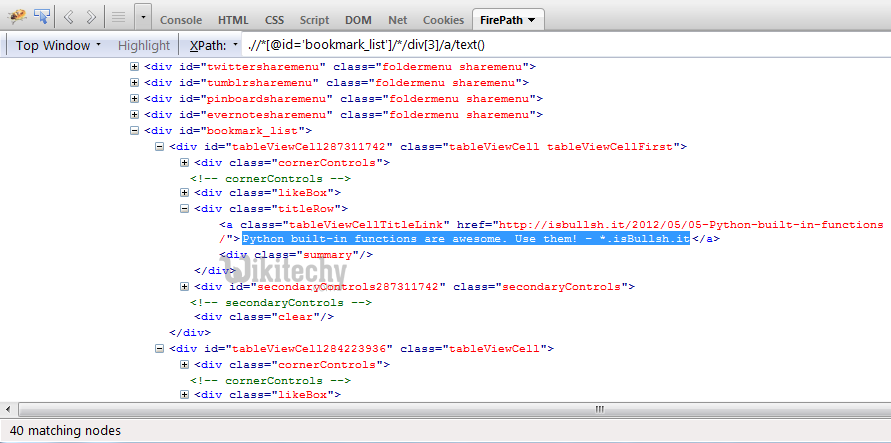
Learn python - python tutorial - python-filepath - python examples - python programs
- Since python can't print out the structure (it would be an infinite loop) it uses the ellipsis to show that there is recursion in the structure.
- I'm not quite sure if the question was what what going on or how to fix it, but I'll try to correct the functions above.
- In both of them, you first make two recursive calls, which add data to the list y, and then AGAIN append the returned data to y. This means the same data will be present several times in the result.
- Either just collect all the data without adding to any y, with something like
return [x[2]]+keys(x[0])+keys(x[1])
click below button to copy the code. By Python tutorial team
- or just do the appending in the calls, with something like
y += [x[2]]
keys(x[0], y) #Add left children to y...
keys(x[1], y) #Add right children to y...
return y
click below button to copy the code. By Python tutorial team
- (Of course, both these snippets need handling for empty lists etc)
- @Abgan also noted that you really don't want y=[] in the initializer.
If you would have used a PrettyPrinter, the output would had been self explanatory
>>> l = [1,2,3,4]
>>> l[0]=l
>>> l
[[...], 2, 3, 4]
>>> pp = pprint.PrettyPrinter(indent = 4)
>>> pp.pprint(l)
[<Recursion on list with id=70327632>, 2, 3, 4]
>>> id(l)
70327632
click below button to copy the code. By Python tutorial team
python interview questions :55
Regex for the dot character?
- Well, a dot is a special character in a regex pattern. So if you want to sepcify a dot you need to escape the special charactr dot like that:
import re
regx = re.compile('\.ab..')
ss = ',ab123 .ab4578 !ab1298 .abUVMO'
print regx.findall(ss)
# result: ['.ab45', '.abUV']
click below button to copy the code. By Python tutorial team
- Python datetime weird behavior
- datetime.replace returns a new datetime instance.
- In your first example you are ignoring the return value of datetime.replace and are then doing datetime.strftime on your old datetime instance.
- This causes the inequality you are experiencing.
To make both examples equal you would have to edit the verbose one to look like:
>>> a = datetime.fromtimestamp(1373576406)
>>> a = a.replace(tzinfo=tzutc())
>>> a.strftime('%s')
'1373576406
click below button to copy the code. By Python tutorial team
python interview questions :56
python what is this line doing?
As an alternative to all that string slicing, which is quite inefficient, consider using a bytearray
>>> import struct
>>> p=bytearray('foobar')
>>> p
bytearray(b'foobar')
>>> p[4]=struct.pack(">b", 64)
>>> p
bytearray(b'foob@r')
click below button to copy the code. By Python tutorial team
bytearray has lots of string methods available
>>> dir(p)
['__add__', '__alloc__', '__class__', '__contains__', '__delattr__',
'__delitem__', '__doc__', '__eq__', '__format__', '__ge__',
'__getattribute__', '__getitem__', '__gt__', '__hash__', '__iadd__',
'__imul__', '__init__', '__iter__', '__le__', '__len__', '__lt__',
'__mul__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__',
'__rmul__', '__setattr__', '__setitem__', '__sizeof__', '__str__',
'__subclasshook__', 'append', 'capitalize', 'center', 'count', 'decode',
'endswith', 'expandtabs', 'extend', 'find', 'fromhex', 'index', 'insert',
'isalnum', 'isalpha', 'isdigit', 'islower', 'isspace', 'istitle', 'isupper',
'join', 'ljust', 'lower', 'lstrip', 'partition', 'pop', 'remove', 'replace',
'reverse', 'rfind', 'rindex', 'rjust', 'rpartition', 'rsplit', 'rstrip',
'split', 'splitlines', 'startswith', 'strip', 'swapcase', 'title',
'translate', 'upper', 'zfill']
click below button to copy the code. By Python tutorial team
and is easy to convert back to string when you are done mutating it
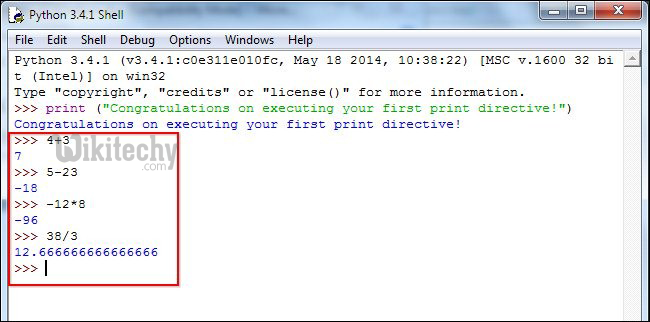
Learn python - python tutorial - python-print-directive - python examples - python programs
>>> str(p)
'foob@r'
click below button to copy the code. By Python tutorial team
python interview questions :57
Why Python http server cause android VolleyError?
- It is not related to Android (hint: you may check your server from simple web browser). According to protocol specification HTTP server must start its response with line
HTTP/<version> <code> <optional message>
click below button to copy the code. By Python tutorial team
- Then server sends other headers, blank line and optional binary body (in some cases). It turns out that status message behaves like a header although it is not explicitly exposed in documentation. Since that you have to call end_headers() after send_response().
- Just add it to your code.
def do_GET(self):
print("DO GET")
self.send_response(200, "OK")
self.end_headers()
# Send optional body in HTML, XML, JSON, binary data, etc.
self.wfile.write(bytes('<html><body>Hello!</body></html>', 'utf-8'))
click below button to copy the code. By Python tutorial team
- If you add the body you may enter in browser http://localhost:7000 and see hello message. After that re-check server from your Andriod application.
- Also change http.server.SimpleHTTPRequestHandler to http.server.BaseHTTPRequestHandler, latter is enough in your case.
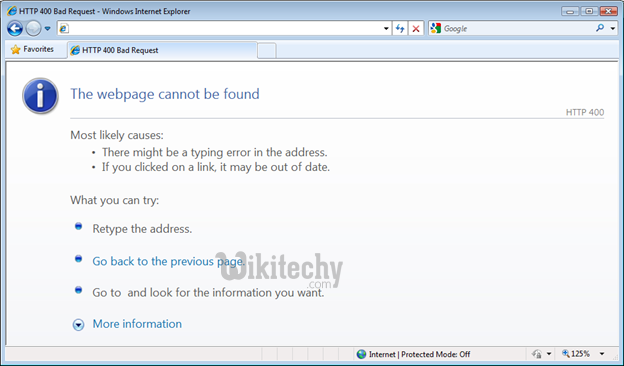
Learn python - python tutorial - windows-internet-explorer - python examples - python programs
python interview questions :58
Why Python http server cause android VolleyError?
- It is not related to Android (hint: you may check your server from simple web browser). According to protocol specification HTTP server must start its response with line
HTTP/<version> <code> <optional message>
click below button to copy the code. By Python tutorial team
- Then server sends other headers, blank line and optional binary body (in some cases). It turns out that status message behaves like a header although it is not explicitly exposed in documentation. Since that you have to call
end_headers()
aftersend_response().
- Just add it to your code.
def do_GET(self):
print("DO GET")
self.send_response(200, "OK")
self.end_headers()
# Send optional body in HTML, XML, JSON, binary data, etc.
self.wfile.write(bytes('<html><body>Hello!</body></html>', 'utf-8'))
click below button to copy the code. By Python tutorial team
- If you add the body you may enter in browser http://localhost:7000 and see hello message. After that re-check server from your Andriod application.
- Also change http.server.SimpleHTTPRequestHandler to http.server.BaseHTTPRequestHandler, latter is enough in your case.
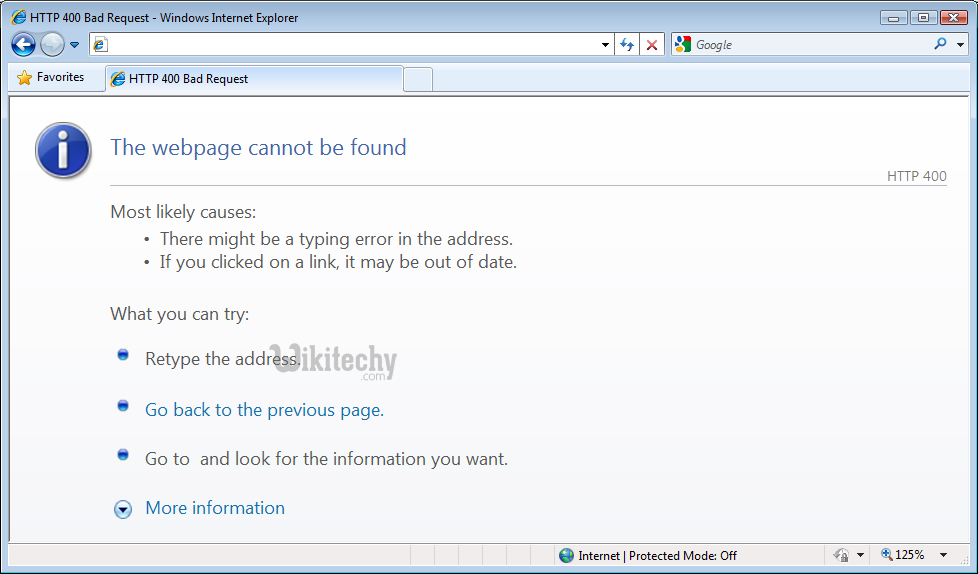
Learn python - python tutorial - webpage-cannot-be-found - python examples - python programs
python interview questions :59
What style does PyCharm / IntelliJ use for Python docstrings ?
- Those are the Sphinx style doc strings.
- Python has some strong opinions about doc strings, see PEP257.
python interview questions :60
Which cassandra python pacakge to be used?
- Pycassa is an older python driver that is thrift based while python-driver is a newer CQL3 driver based on cassandra's binary protocol. Thrift isn't going away but it has become a legacy API in cassandra so my advice going forward is to use the newer python driver.
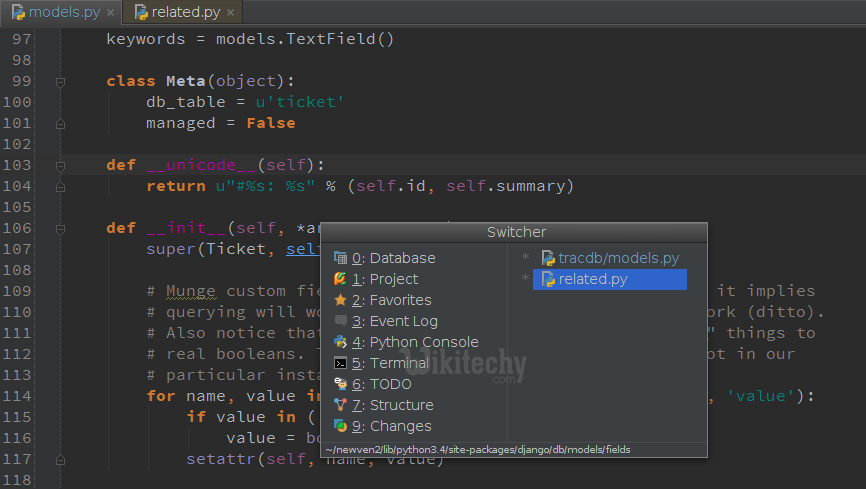
Learn python - python tutorial - related-python - python examples - python programs
- I wrote a blog that you might find helpful, which uses the Twissandra example application with the DataStax python-driver to provide an overview of CRUD and using prepared statements etc.
- As for cql I haven't had any experience with this one, but the homepage of the project says it all:
This driver has been deprecated. Please use python-driver https://github.com/datastax/python-driver instead