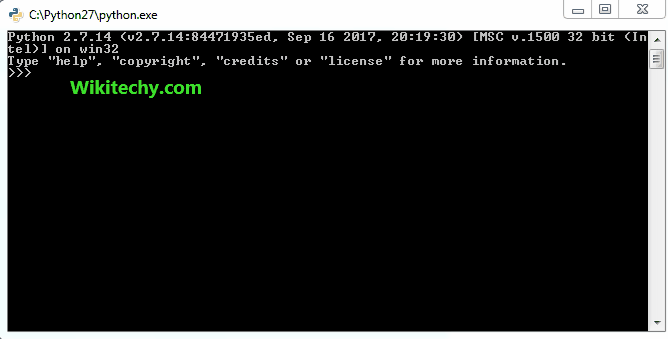
Learn Python - Python tutorial - python exception - Python examples - Python programs
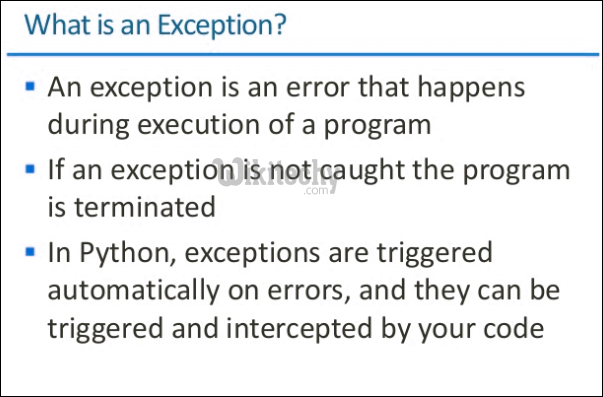
Like other languages, python also provides the runtime errors via exception handling method with the help of try-except. Some of the standard exceptions which are most frequent include IndexError, ImportError, IOError, ZeroDivisionError, TypeError.
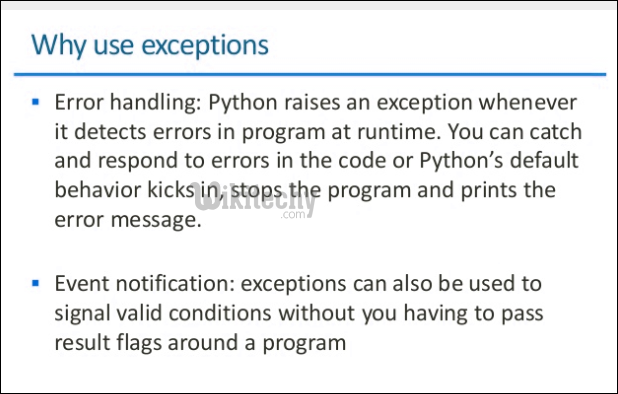
Exception is the base class for all the exceptions in python. You can check the exception hierarchy
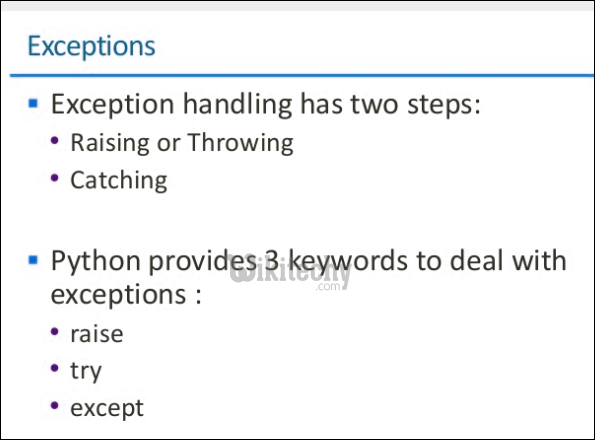
Let us try to access the array element whose index is out of bound and handle the corresponding exception.
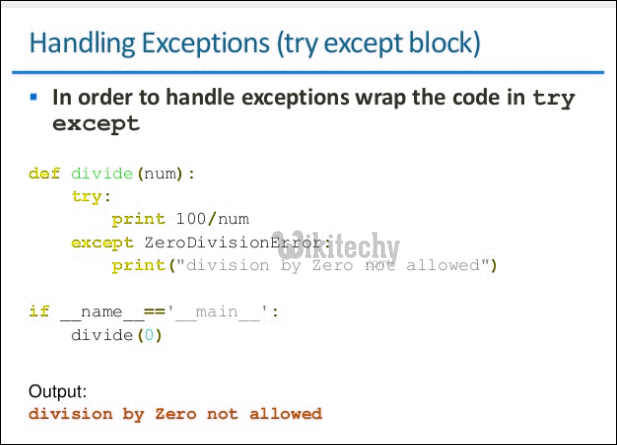
python - Sample - python code :
# Python program to handle simple runtime error
a = [1, 2, 3]
try:
print "Second element = %d" %(a[1])
# Throws error since there are only 3 elements in array
print "Fourth element = %d" %(a[3])
except IndexError:
print "An error occurred"
click below button to copy the code. By Python tutorial team
Output:
Second element = 2 An error occurred
A try statement can have more than one except clause, to specify handlers for different exceptions. Please note that at most one handler will be executed.
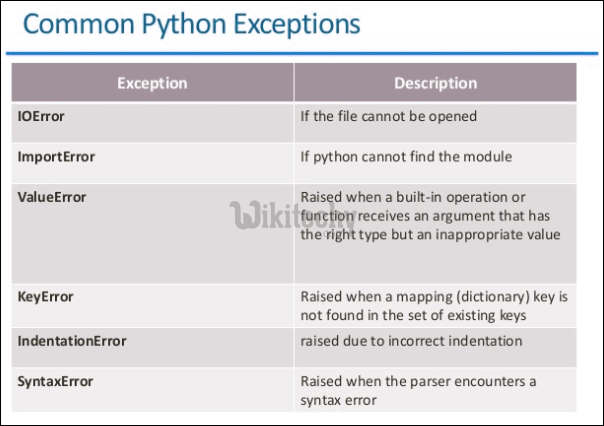
python - Sample - python code :
# Program to handle multiple errors with one except statement
try :
a = 3
if a < 4 :
# throws ZeroDivisionError for a = 3
b = a/(a-3)
# throws NameError if a >= 4
print "Value of b = ", b
# note that braces () are necessary here for multiple exceptions
except(ZeroDivisionError, NameError):
print "\nError Occurred and Handled"
click below button to copy the code. By Python tutorial team
python tutorial - Output :
Error Occurred and Handled
If you change the value of ‘a’ to greater than or equal to 4, the the output will be
Value of b = Error Occurred and Handled
The output above is so because as soon as python tries to access the value of b, NameError occurs.
Else Clause:
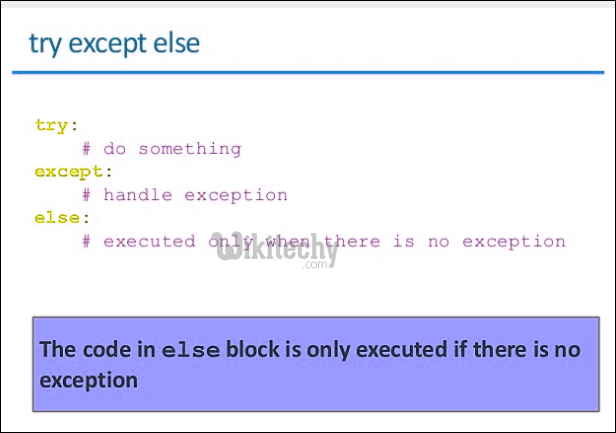
In python, you can also use else clause on try-except block which must be present after all the except clauses. The code enters the else block only if the try clause does not raise an exception.
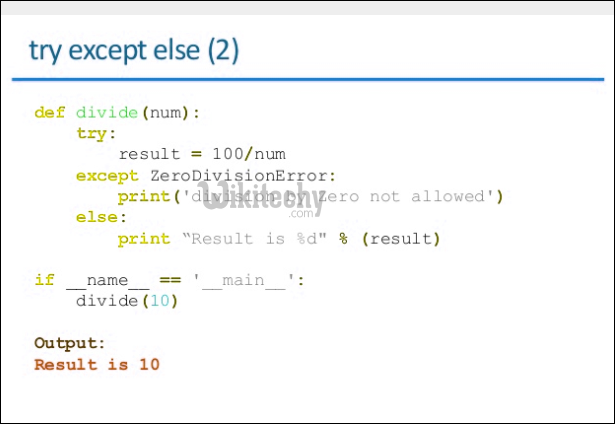
python - Sample - python code :
# Program to depict else clause with try-except
# Function which returns a/b
def AbyB(a , b):
try:
c = ((a+b) / (a-b))
except ZeroDivisionError:
print "a/b result in 0"
else:
print c
# Driver program to test above function
AbyB(2.0, 3.0)
AbyB(3.0, 3.0)
click below button to copy the code. By Python tutorial team
The output for above program will be :
-5.0 a/b result in 0
Raising Exception:
The raise statement allows the programmer to force a specific exception to occur. The sole argument in raise indicates the exception to be raised. This must be either an exception instance or an exception class (a class that derives from Exception).
python - Sample - python code :
# Program to depict Raising Exception
try:
raise NameError("Hi there") # Raise Error
except NameError:
print "An exception"
raise # To determine whether the exception was raised or not
click below button to copy the code. By Python tutorial team
The output of the above code will simply line printed as “An exception” but a Runtime error will also occur in the last due to raise statement in the last line. So, the output on your command line will look like
Traceback (most recent call last): File "003dff3d748c75816b7f849be98b91b8.py", line 4, in raise NameError("Hi there") # Raise Error NameError: Hi there