python tutorial - Python question and answer - learn python - python programming
python interview questions :201
How about dictionary comprehension?
>>> D1 = dict((k,k**3) for k in L)
>>> D1
{0: 0, 1: 1, 2: 8, 3: 27, 4: 64, 5: 125}
click below button to copy the code. By Python tutorial team
- Or for Python 3:
>>> D2 = {k:k**3 for k in L}
>>> D2
{1: 1, 2: 8, 3: 27}
click below button to copy the code. By Python tutorial team
python interview questions :202
Sum of all elements in a list ([1,2,3,...,100] with one line of code ?
>>> Ans = sum(range(101))
>>> Ans
click below button to copy the code. By Python tutorial team
5050
python interview questions :203
How about summing only odd elements?
>>> sum(range(1,101,2))
click below button to copy the code. By Python tutorial team
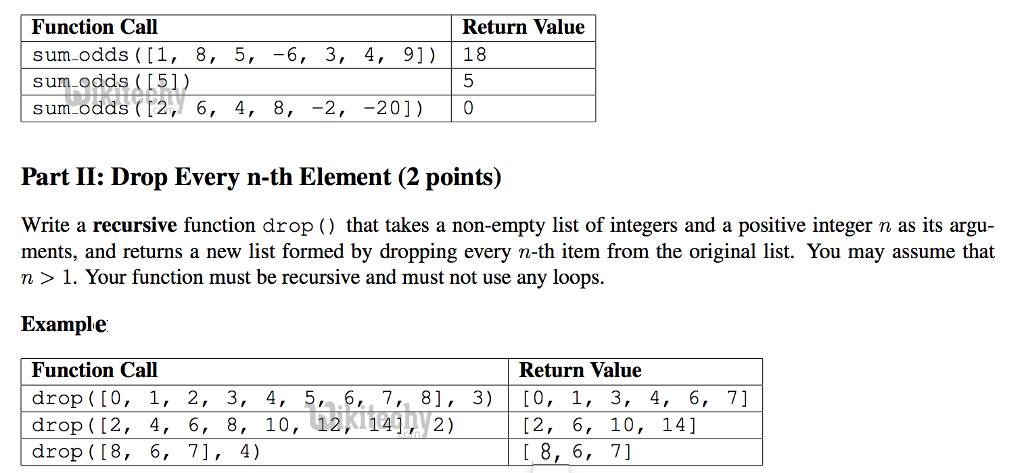
Learn python - python tutorial - odd - python examples - python programs
python interview questions :204
How to truncate division operation?
- The truncating division operator (also known as floor division) truncates the result to an integer and works with both integers and floating-point numbers.
- As of this writing, the true division operator (/) also truncates the result to an integer if the operands are integers. Therefore, 7/4 is 1, not 1.75.
- However, this behavior is scheduled to change in a future version of Python, so you will need to be careful.
- The modulo operator returns the remainder of the division x // y. For example, 7 % 4 is 3.
- For floating-point numbers, the modulo operator returns the floating-point remainder of x // y, which is x (x // y) * y. For complex numbers, the modulo (%) and truncating division operators (//) are invalid.
- Division of two number like this:
>>> 5.5/2
click below button to copy the code. By Python tutorial team
Output:
2.75
>>> 5.5//2
click below button to copy the code. By Python tutorial team
Output:
2.0
python interview questions :205
How to Print a list of file in a directory?
- If you want to print a list of files in a directory including the sub-directories. You may want to do it recursively.
import os
def file_list(dir):
basedir = dir
subdir_list = []
for item in os.listdir(dir):
fullpath = os.path.join(basedir,item)
if os.path.isdir(fullpath):
subdir_list.append(fullpath)
else:
print fullpath
for d in subdir_list:
file_list(d)
file_list('/dir')
click below button to copy the code. By Python tutorial team
Output:
./d3/f1
./d3/d4/d7/f2
./d3/d4/d7/f3
./d3/d5/d9/d10/f4
./d3/d5/d9/d10/f5
[2, 3, 5, 7, 11, 13, 17, 19, 23, 29, 31, 37, 41, 43, 47, 53, 59, 61, 67, 71, 73, 79, 83, 89, 97]
python interview questions :206
How to Make a prime number list from (1,100)?
import math
def isPrime(n):
if n <= 1:
return False
if n == 2:
return True
if n % 2 == 0:
return False
for t in range(3, int(math.sqrt(n)+1),2):
if n % t == 0:
return False
return True
print [n for n in range(100) if isPrime(n)]
click below button to copy the code. By Python tutorial team
Output:
[2, 3, 5, 7, 11, 13, 17, 19, 23, 29, 31, 37, 41, 43, 47, 53, 59, 61, 67, 71, 73, 79, 83]
python interview questions :207
How to reverse a string using recursive function?
- In the following recursive code, at every call, the first string will go to the end and stored in stack. So, when there is only one character left as an input string, the code starts rewind and retrieve the character one by one.
- The net effect is it gets the character in reverse. It becomes obvious when we see the printed output below:
def reverse(input):
print input
if len(input) <= 1:
return input
return reverse(input[1:]) + input[0]
s = 'reverse'
print(reverse(s))
click below button to copy the code. By Python tutorial team
Output:
reverse
everse
verse
erse
rse
se
e
esrever
python interview questions :208
How to Merge the overlapped range?
- You want to merge all overlapping ranges and return a list of distinct ranges. For example:
[(1, 5), (2, 4), (3, 6)] ---> [(1, 6)]
[(1, 3), (2, 4), (5, 6)] ---> [(1, 4), (5, 6)]
click below button to copy the code. By Python tutorial team
- Here is the code:
L = [(4,9), (20, 22), (1, 3), (24, 32), (23, 31), (40,50), (12, 15), (8,13)]
# L = [(1, 5), (2, 4), (3, 6)]
# L = [(1, 3), (2, 4), (5, 6)]
L = sorted(L)
print L
Lnew = []
st = L[0][0]
end = L[0][1]
for item in L[1:]:
if end >= item[0]:
if end < item[1]:
end = item[1]
else:
Lnew.append((st,end))
st = item[0]
end = item[1]
Lnew.append((st,end))
print Lnew
click below button to copy the code. By Python tutorial team
Output:
[(1, 3), (4, 9), (8, 13), (12, 15), (20, 22), (23, 31), (24, 32), (40, 50)]
[(1, 3), (4, 15), (20, 22), (23, 32), (40, 50)]
python interview questions :209
How to Initialize dictionary with list - I ?
- Sometimes If you want to construct dictionary whose values are lists.
- In the following example, we make a dictionary like {'Country': [cities,...], }:
cities = {'San Francisco': 'US', 'London':'UK',
'Manchester':'UK', 'Paris':'France',
'Los Angeles':'US', 'Seoul':'Korea'}
# => {'US':['San Francisco', 'Los Angeles'], 'UK':[,], ...}
from collections import defaultdict
# using collections.defaultdict()
d1 = defaultdict(list) # initialize dict with list
for k,v in cities.items():
d1[v].append(k)
print d1
# using dict.setdefault(key, default=None)
d2 = {}
for k,v in cities.items():
d2.setdefault(v,[]).append(k)
print d2
click below button to copy the code. By Python tutorial team
Output:
defaultdict(<type 'list'>, {'Korea': ['Seoul'], 'US': ['Los Angeles', 'San Francisco'], 'UK': ['Manchester', 'London'], 'France': ['Paris']}) {'Korea': ['Seoul'], 'US': ['Los Angeles', 'San Francisco'], 'UK': ['Manchester', 'London'], 'France': ['Paris']}
python interview questions :210
How to Initialize dictionary with list - II?
- A little bit simpler problem. If youhave a list of numbers:
L = [1,2,4,8,16,32,64,128,256,512,1024,32768,65536,4294967296]
click below button to copy the code. By Python tutorial team
L = [1,2,4,8,16,32,64,128,256,512,1024,32768,65536,4294967296]
from collections import defaultdict
d = defaultdict(list)
for i in L:
d[len(str(i))].append(i)
print d
print {k:v for k,v in d.items()}
click below button to copy the code. By Python tutorial team
Output:
defaultdict(<type 'list'>, {1: [1, 2, 4, 8], 2: [16, 32, 64], 3: [128, 256, 512], 4: [1024], 5: [32768, 65536], 10: [4294967296]})
{1: [1, 2, 4, 8], 2: [16, 32, 64], 3: [128, 256, 512], 4: [1024], 5: [32768, 65536], 10: [4294967296]}