AngularJS Forms
- The AngularJS provides many additional supports such as validation, data-binding to the HTML form.
- These are the input elements used in HTML form.
- <button> element.
- <input> element.
- <select> element.
- <textarea> element.
Syntax for text in AngularJS:
Enter Name :
<input type="text" ng-model="name" />
Syntax for radio button in AngularJS:
Select Gender :
<input type="radio" ng-model="gender" value="Male" />Male
<input type="radio" ng-model="gender" value="Female" />Female
Syntax for select button in AngularJS:
Select Profession :
<select ng-model="profession">
<option value="Student">Student
<option value="Employee">Employee
</select>
Syntax for check box button in AngularJS:
<input type="checkbox" ng-model="agree"/>I Accept Terms and Conditions.
Sample code for Form in AngularJS:
Tryit<!DOCTYPE html>
<html>
<head>
<title>Wikitechy AngularJS Tutorials</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.5.6/
angular.min.js"> </script>
</head>
<body>
<div ng-app="">
<form>
<h3>HTML Form in AngularJS</h3>
Enter Name :
<input type="text" ng-model="name" />
Select Gender :
<input type="radio" ng-model="gender" value="Male" />Male
<input type="radio" ng-model="gender" value="Female" />Female
Select Profession :
<select ng-model="profession">
<option value="Student">Student
<option value="Employee">Employee
</select><br><br>
<input type="checkbox" ng-model="agree"/>
I Accept Terms and Conditions.
</form>
<h3 ng-show="name">Hello {{name}}</h3>
<h3 ng-show="gender">You are Select : {{gender}}</h3>
<h3 ng-show="profession">You are Select : {{profession}}</h3>
<h3 ng-show="agree">
Thank You for Accept our Terms And Conditions.
</h3>
</div>
</body>
</html>
Code Explanation for Form in AngularJS:
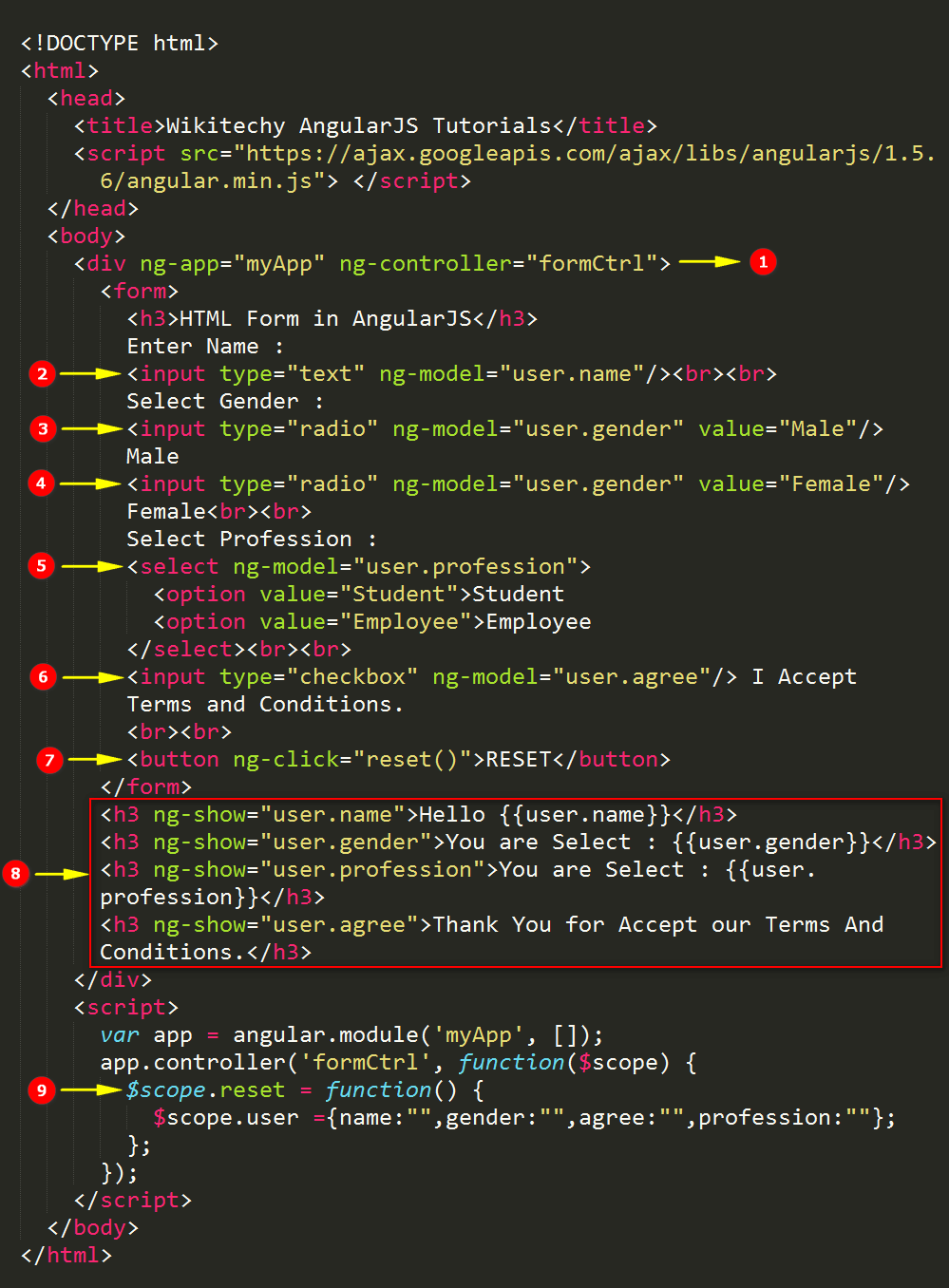
- The ng-app specifies the root element (<div>) to define AngularJS application with controller “formCtrl”.
- The textbox is defined for getting name from user.
- The radio buttons are defined for getting Male gender from user.
- The radio buttons are defined for getting Female gender from user.
- The select box is defined for getting profession from user.
- The check box is defined for user accept the terms and conditions.
- The Reset is defined for Reset the form details.
- The information from the form is bind to the <h3> tag with ng-show attribute.
- The reset function is used to reset all values in the form.
Sample Output for Form in AngularJS:
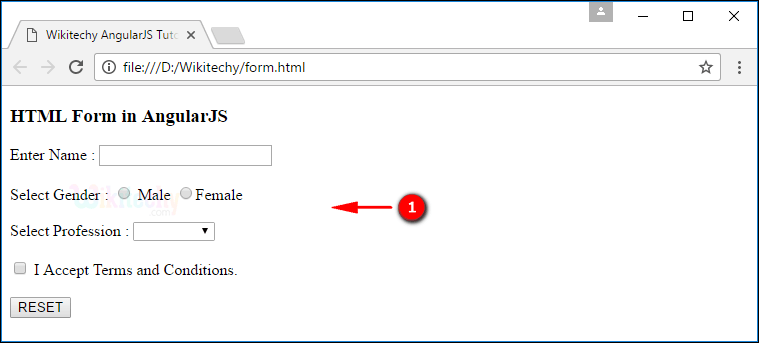
- The form is displayed on page load.
- When user enter input to the form then that will be bind to the bottom of the form.
- Then User Click the reset button.
- All details are Resetted.
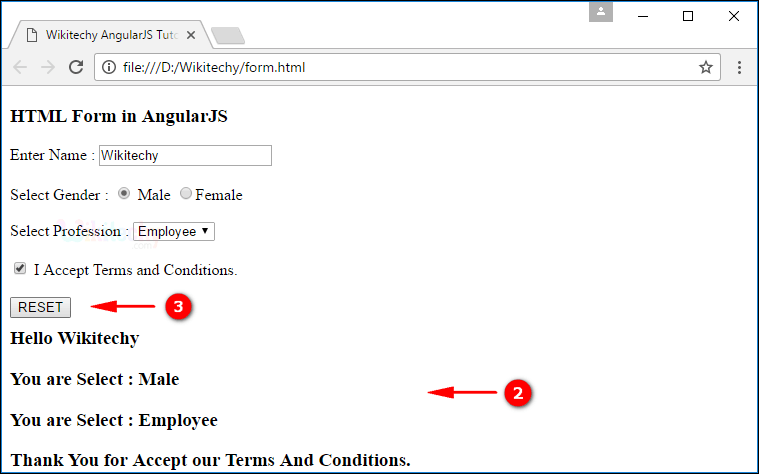
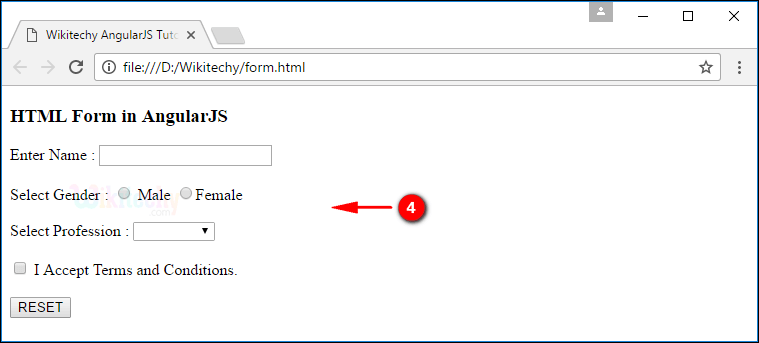