AngularJS Isolated Scopes
- A new “isolate” scopes is created for the directive’s element.
- An “isolate” scope does not prototypically inherit from its parent scope.
- The scope option is used to create an isolate scope.
- An “isolate” scope is valuable when creating reusable components, which should not incidentally read or alter data in the parent scope.
- As the name recommends, the isolate scope of the directive isolate everything except models that you have explicitly added to the scope: {} hash object.
Sample coding for Isolate Scope in AngularJS:
Tryit<!DOCTYPE html>
<html>
<head>
<title>Wikitechy AngularJS Tutorials</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.5.6/
angular.min.js"> </script>
</head>
<body>
<h2>Wikitechy Isolate Scope in AngularJS</h2>
<div ng-app="myApp" ng-controller="Controller">
<myfriend information="adam"></myfriend></br>
<myfriend information="angel"></myfriend>
</div>
<script>
var app= angular.module('myApp', [ ]);
app.controller('Controller',[ ‘$scope’, function($scope) {
$scope.adam = { name: 'Adam', age:32, city: 'London' };
$scope.angel = { name: 'Angel', age:21, city: 'New York' };
}])
.directive('myfriend', function() {
return {
scope: {
friendInfo: '=information'
},
template:'Name: {{friendInfo.name}} Age: {{friendInfo.age}}
City: {{friendInfo.city}}'
};
});
</script>
</body>
</html>
Code Explanation for Isolate Scope in AngularJS:
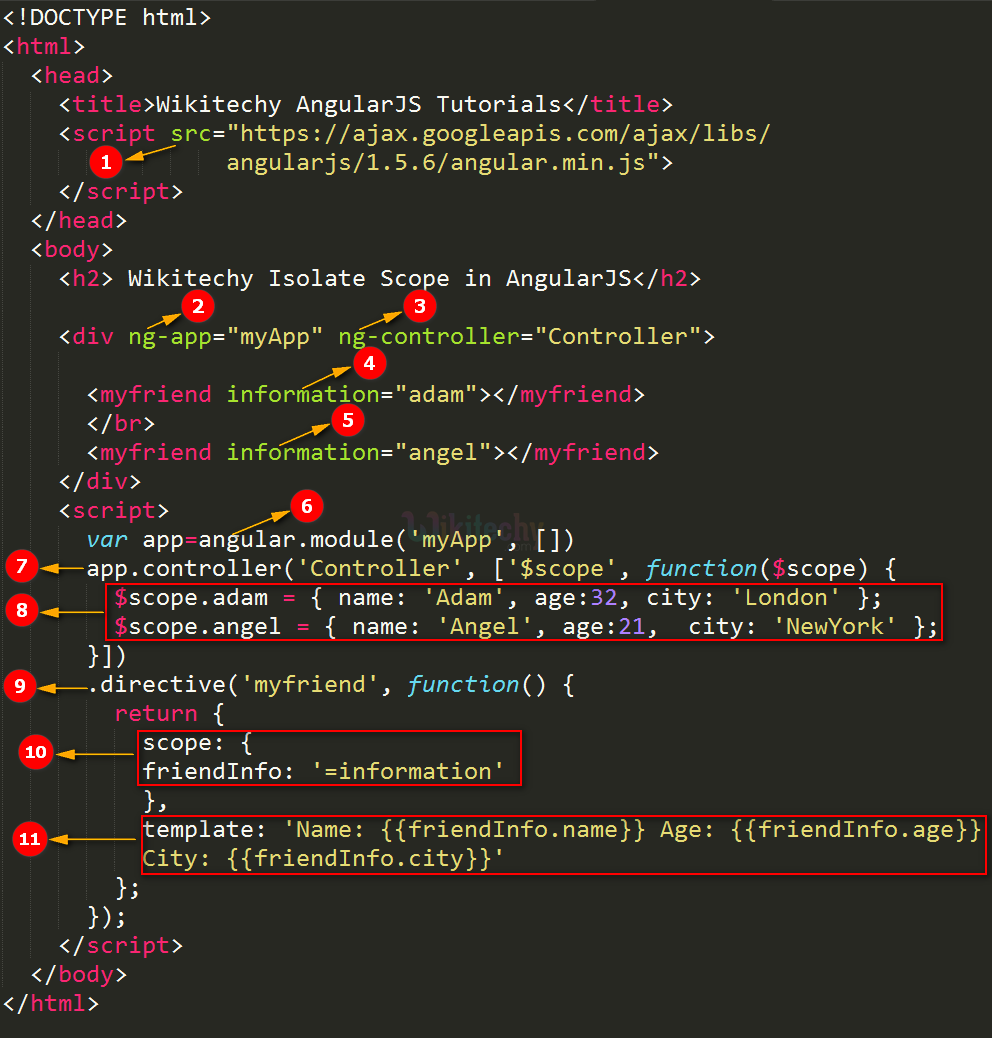
- AngularJS is distributed as a JavaScript file, and can be added to a HTML page with a <script> tag.
- The AngularJS application is defined by