AngularJS DOM
- The AngularJS provides directives for binding application data to the HTML DOM element’s attribute.
- These are the HTML DOM attributes used in AngularJS.
- Show as ng-show directive.
- Hide as ng-hide directive.
- Disabled as ng-disabled directive.
Syntax for HTML DOM in AngularJS:
<element ng-show="Boolean value"></element>
<element ng-hide="Boolean value"></element>
<element ng-disabled="Boolean value"></element>
Sample code for HTML DOM in AngularJS:
Tryit<!DOCTYPE html>
<html>
<head>
<title>Wikitechy AngularJS Tutorials</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.5.6/
angular.min.js"> </script>
</head>
<body>
<div ng-app="myApp" ng-controller="DOMCtrl">
<h3>HTML DOM in AngularJS</h3>
Enter Name :
<input type="text" ng-model="name" ng-hide="myhide" ng-show="myshow"
ng-disabled="mydisabled" />
<p>myhide : {{myhide}}</p>
<p>myshow : {{myshow}}</p>
<p>mydisabled : {{mydisabled}}</p>
<input type="button" value="Show" ng-click="showFun()" />
<input type="button" value="Hide" ng-click="hideFun()" />
<input type="button" value="Disable" ng-click="disFun()" />
</div>
<script>
var app = angular.module( 'myApp', [] );
app.controller("DOMCtrl", function($scope) {
$scope.showFun = function () {
$scope.myhide = false;
$scope.myshow = true;
$scope.mydisabled = false;
};
$scope.hideFun = function () {
$scope.myhide = true;
$scope.myshow = false;
$scope.mydisabled = false;
};
$scope.disFun = function () {
$scope.myhide = false;
$scope.myshow = true;
$scope.mydisabled = true;
};
});
</script>
</body>
</html>
Data:
- Set of data has been used in our AngularJS Application.
myhide = false;
myshow = true;
mydisabled = true;
HTML:
- Viewable HTML contents in AngularJS Application.
<div ng-app="myApp" ng-controller="DOMCtrl">
<h3>HTML DOM in AngularJS</h3>
Enter Name :
<input type="text" ng-model="name" ng-hide="myhide" ng-show="myshow"
ng-disabled="mydisabled" />
<p>myhide : {{myhide}}</p>
<p>myshow : {{myshow}}</p>
<p>mydisabled : {{mydisabled}}</p>
<input type="button" value="Show" ng-click="showFun()" />
<input type="button" value="Hide" ng-click="hideFun()" />
<input type="button" value="Disable" ng-click="disFun()" />
</div>
Logic:
- Controller logic for the AngularJS application.
app.controller("DOMCtrl", function($scope) {
$scope.showFun = function () {
$scope.myhide = false;
$scope.myshow = true;
$scope.mydisabled = false;
};
$scope.hideFun = function () {
$scope.myhide = true;
$scope.myshow = false;
$scope.mydisabled = false;
};
$scope.disFun = function () {
$scope.myhide = false;
$scope.myshow = true;
$scope.mydisabled = true;
};
});
Code Explanation for HTML DOM in AngularJS:
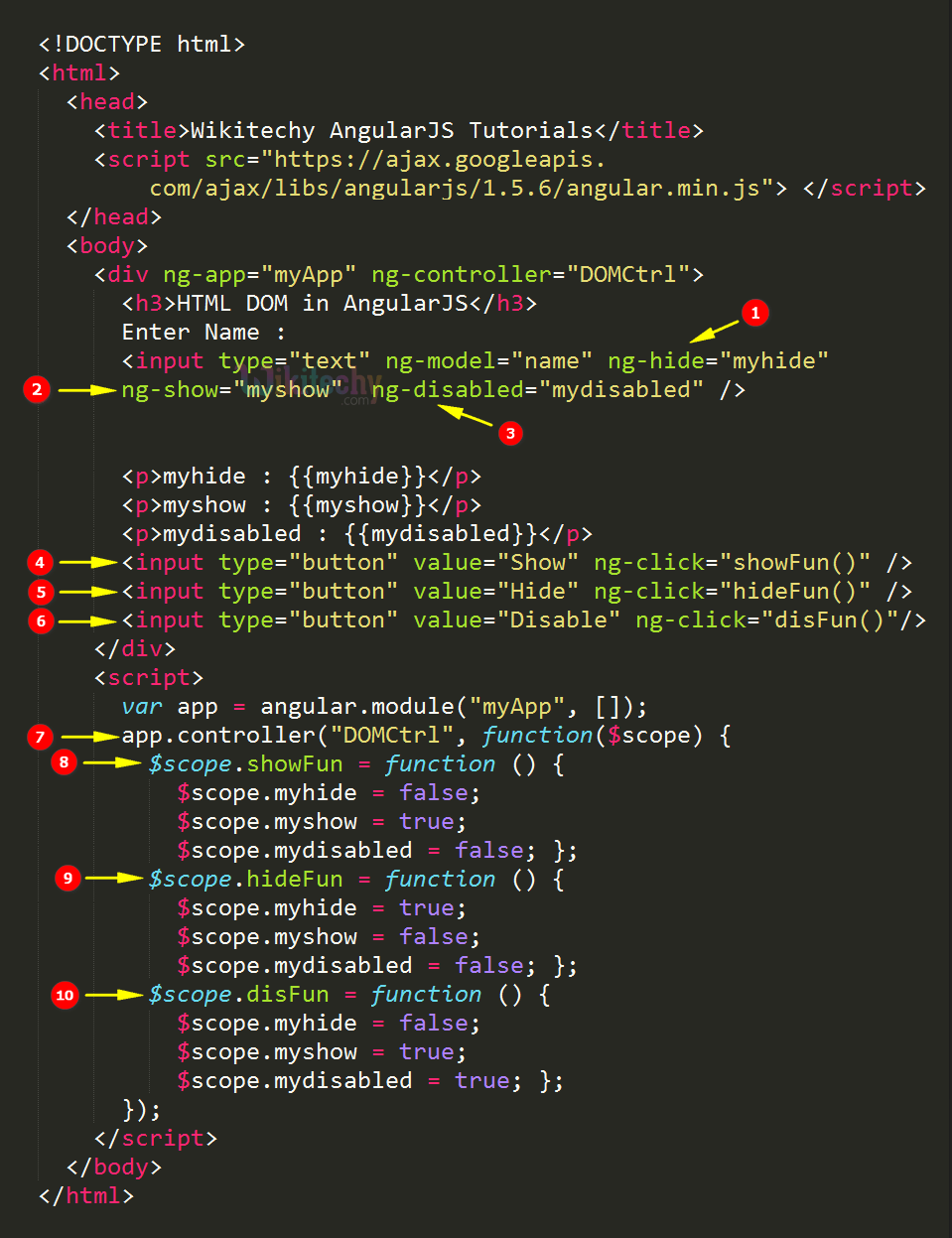
- The ng-hide directive is used to hide or show the textbox by changing the Boolean value myhide.
- The ng-show directive is used to hide or show the textbox by changing the Boolean value myshow.
- The ng-disabled directive is used to disable or enable the textbox by changing the Boolean value mydisabled.
- The “Show” button used to call the “showFun()” function.
- The “Hide” button used to call the “hideFun()” function.
- The “Disable” button used to call the “disFun()” function.
- The “DOMCtrl” used to create the controller for the Application with $scope object argument.
- The showFun is a function used to set myshow as true, myhide and mydisabled as false variable.
- The hideFun is a function used to set myhide as true, myshow and mydisabled as false variable.
- The disFun is a function used to set mydisabled and myshow as true and myhide as false variable.
Sample Output for $interval Service in AngularJS:
- The Values are empty on the page load.
- When user click the Show button then the textbox will be visible.
- When user Click the Hide button then the textbox will be disappear.
- When user click the Disable button then the textbox will be disabled so that user cannot edit the text.
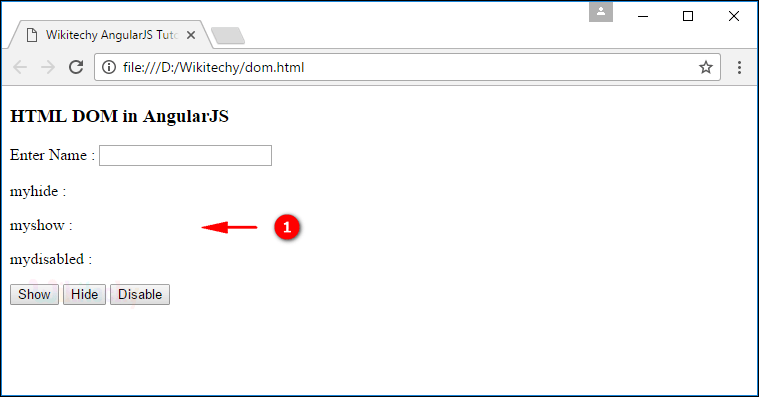
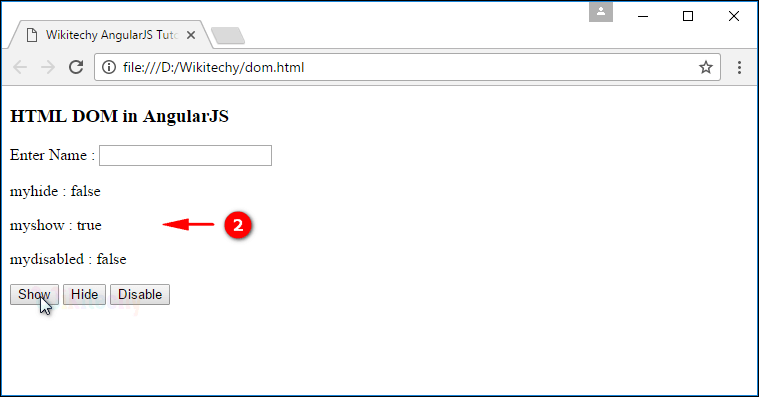
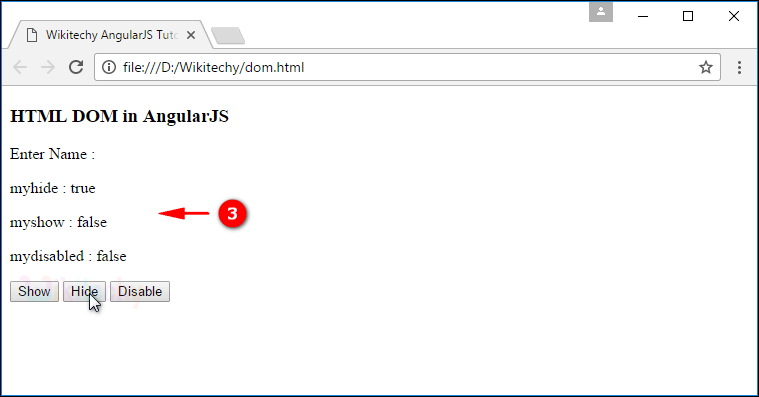
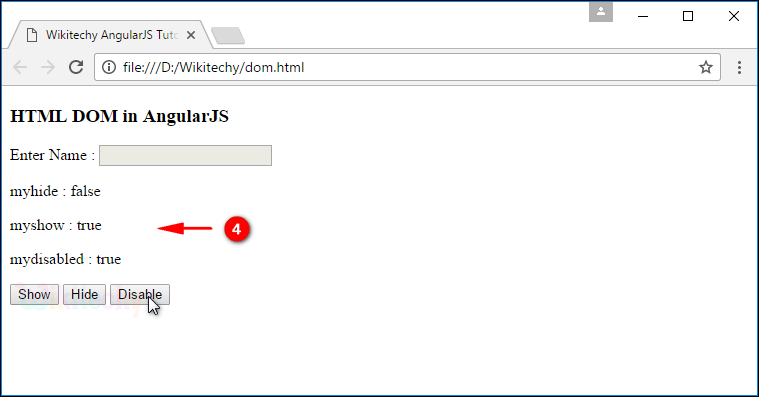