AngularJS Orderby
- The orderBy filter is used to sort an array.
- By default, the strings are sorted in alphabetically.
- By default, the numbers are sorted in numerically.
- The orderBy filter also supports a comparator function based on the values computed using the expression to build.
- The minus symbol (-) is used to sort an array in descending order.
Syntax for orderBy filter in AngularJS:
{{ orderBy_expression | orderBy : expression : reverse : comparator}}
Parameter value for orderBy filter in AngularJS:
Value | Type | Description |
---|---|---|
expression | array<(function() | string)> string function() |
The expression is used to define the order of the element. The expression can be one of array, string and function. Array: an array item can be both function and string. If you use an array when you need more than one object property to define the sorting order. String: If the array is an array of object, you can sort the array by the value of one of the object properties. The optional prefix + or – is used to control the sorting direction as ascending or descending in expression. The default order is ascending order. If no property is provided, the collection items itself is used in comparison. Function: It is allowed the user to create a custom function for sorting. |
reverse | boolean | This field value is optional. This is also a boolean value. If true, reverse the sorting order. |
comparator | function() | This field value is optional. It is allowed the user to create a custom comparator function for sorting. When you used a custom comparator function, you must pass a reverse argument value for sorting. If you set the reverse value as false, then it returns the default order i.e. the ascending order. |
Sample coding for orderBy filter in AngularJS:
Tryit<!DOCTYPE html>
<html>
<head>
<title>Wikitechy AngularJS Tutorials</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.5.6/
angular.min.js"> </script>
</head>
<body>
<div ng-app="myApp" ng-controller="orderCtrl">
<h1>Wikitechy orderBy filter in AngularJS</h1>
<ul>
<h3>Technology list in ascending order</h3>
<li ng-repeat="x in technology | orderBy : 'tut' ">{{x.tut}}</li>
<h3>Technology list in descending order</h3>
<li ng-repeat="x in technology | orderBy : '-tut'">{{x.tut}}</li>
</ul>
</div>
<script>
var app = angular.module( 'myApp', [] );
app.controller('orderCtrl', function($scope) {
$scope.technology =[
{"tut" :"HTML"},
{"tut" :"AngularJS"},
{"tut" :"CSS"},
{"tut" :"C"},
{"tut" :"JAVA"},
{"tut" :"PHP"},
];
});
</script>
</body>
</html>
orderBy Filter in AngularJS:
- The orderBy filter is used to displays a technology array item in ascending and descending order.
<li ng-repeat="x in technology | orderBy : 'tut' ">{{x.tut}}</li>
<li ng-repeat="x in technology | orderBy : '-tut' ">{{x.tut}}</li>
Data:
- Collection of data has been defined using array for our AngularJS Application.
technology =[
{"tut" :"HTML"},
{"tut" :"AngularJS"},
{"tut" :"CSS"},
{"tut" :"C"},
{"tut" :"JAVA"},
{"tut" :"PHP"},
];
Logic:
- Controller logic for the AngularJS Application.
app.controller('orderCtrl', function($scope) {
$scope.technology =[
{"tut" :"HTML"},
{"tut" :"AngularJS"},
{"tut" :"CSS"},
{"tut" :"C"},
{"tut" :"JAVA"},
{"tut" :"PHP"},
];
});
HTML:
- Viewable HTML contents in AngularJS Application.
<div ng-app="myApp" ng-controller="orderCtrl">
<h1>Wikitechy orderBy filter in AngularJS</h1>
<ul>
<h3>Technology list in ascending order</h3>
<li ng-repeat="x in technology | orderBy : 'tut' ">{{x.tut}}</li>
<h3>Technology list in descending order</h3>
<li ng-repeat="x in technology | orderBy : '-tut'">{{x.tut}}</li>
</ul>
</div>
Code Explanation for orderBy filter in AngularJS:
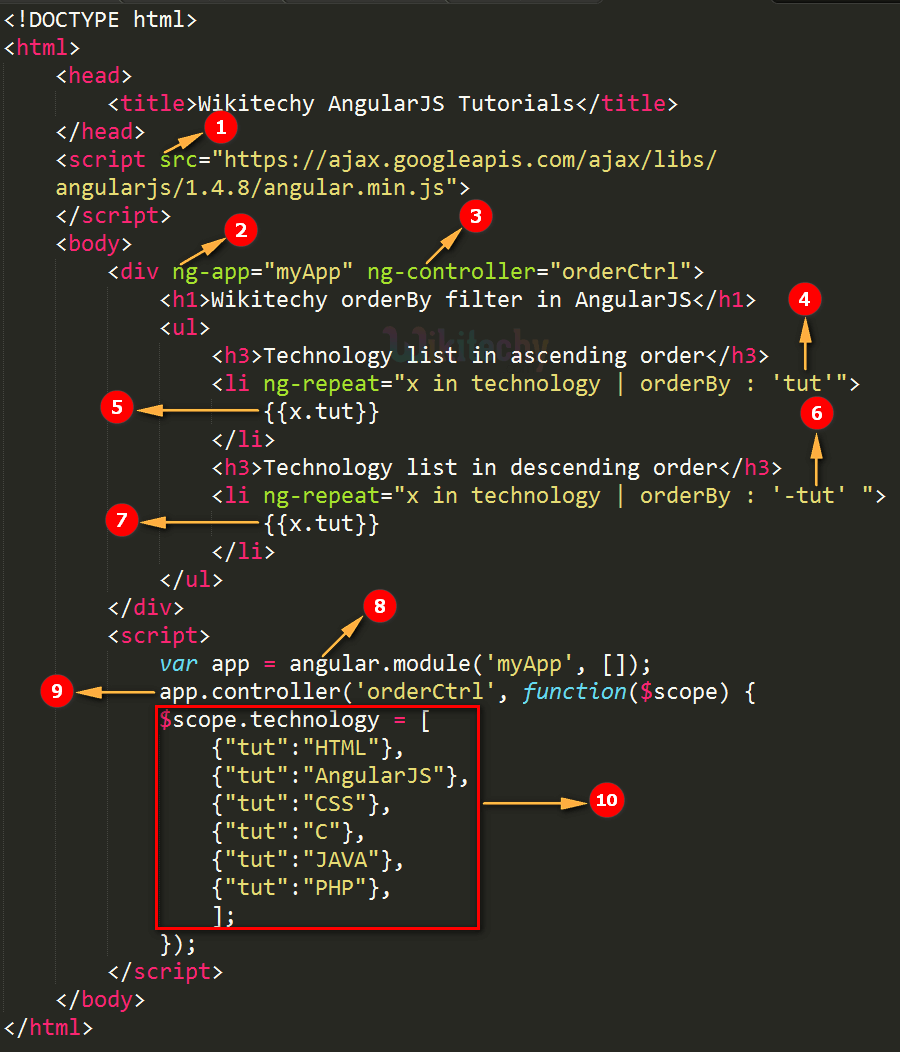
- AngularJS is distributed as a JavaScript file, and can be added to a HTML page with a <script> tag.
- The AngularJS application is defined by ng-app="myApp". The application runs inside the <div> tag. It’s also used to define a <div> tag as a root element.
- The ng-controller=”orderCtrl” is an AngularJS directive. It is used to define a controller name as “orderCtrl”.
- <li ng-repeat="x in technology| orderBy: ‘tut’ "> is used to display technology array element list in ascending order.
- {{ x.tut}} is used to bind a technology array list in ascending order.
- <li ng-repeat="x in technology| orderBy: ‘- tut’ "> is used to order a technology array element list in descending order.
- {{ x.tut}} is used to bind a technology array list in descending order.
- Here we create a module by using angular.module function.
- Here we have declared a controller orderCtrl module using apps.controller() function. The value of the controller modules is stored in scope object. In AngularJS, $scope is passed as first argument to apps.controller during its constructor definition.
- Here we have set the value of $scope.technology array as [{"tut":"HTML"}, {"tut" :"AngularJS"},{"tut" :"CSS"}, {"tut" :"C"}, {"tut" :"JAVA"}, {"tut" :"PHP"}, ];
Sample Output for number filter in AngularJS:
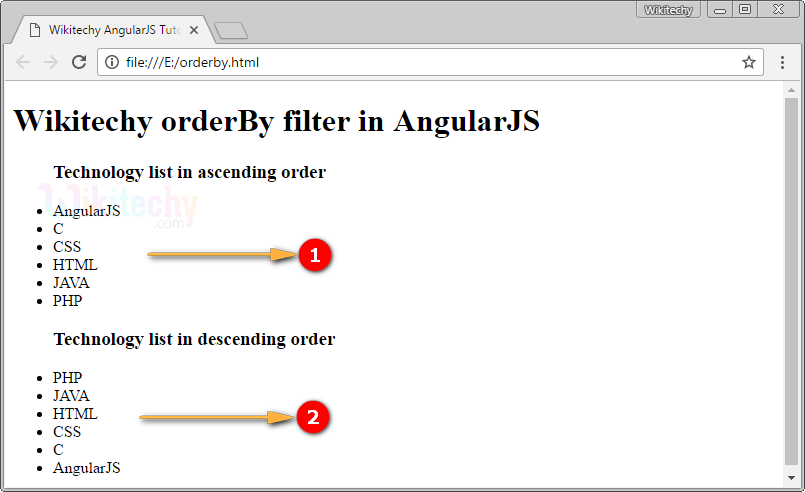
- Display an array technology element in ascending order.
- Display an array technology element in descending order.