AngularJS Filters
- Filters are providing additional support to the AngularJS application by format the value of an expression or directives which are displayed to the users.
- AngularJS provides collection of built-in filters.
- AngularJS transform the data by specified filter.
- Filters can be used in view templates, controllers or services.
- Following are some important built-in filters in AngularJS.
Syntax:
Regular filter
{ { expression | filter } }
Ex. {{50 | currency}}
The expression 50 executed as a currency format using currency filter. The result will be $50.
Chaining filter
{ { expression | filter1 | filter2 | …… } }
The result of filter (filter1) can be applied to another filter (filter2). It is Called “chaining “.
Filters with arguments
{ { expression | filter : argument1 : argument2: ……… } }
Ex: {{12345 | number: 2}}
The expression 10000 executed as a decimal points using the number filter. The result will be 12345.00
Sample coding for Filters in Expression and Directives:
Tryit<!DOCTYPE html>
<html>
<head>
<title>Wikitechy AngularJS Tutorials</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.5.6/
angular.min.js"> </script>
</head>
<body>
<div ng-app="myApp" ng-controller="namesCtrl">
<h3>Using expression and directive in AngularJS Tutorial</h3>
<p>Looping with objects:</p>
<ul>
<li ng-repeat="x in names | orderBy :'country'">
{{ x.name + ', ' + x.country | uppercase }}
</li>
<ul>
</div>
<script>
angular.module('myApp', []).controller('namesCtrl', function($scope) {
$scope.names = [
{name:'Jani',country:'Denmark'},
{name:'Carl',country:'America'},
{name:'Margareth',country:'England'},
];
});
</script>
</body>
</html>
Data:
$scope.names = [
{name:'Jani',country:'Denmark'},
{name:'Carl',country:'America'},
{name:'Margareth',country:'England'},
];
- Scopes is an object that refers to the application model in an AnguarJS application.
HTML:
<div ng-app="myApp" ng-controller="namesCtrl">
<h3>Using expression and directive in AngularJS Tutorial</h3>
<p>Looping with objects:</p>
<ul>
<li ng-repeat="x in names | orderBy :'country'">
{{ x.name + ', ' + x.country | uppercase }}
</li>
</ul>
</div>
- Viewable HTML contents in AngularJS application
Logic:
angular.module('myApp', []).controller('namesCtrl', function($scope) {
$scope.names = [
{name:'Jani',country:'Denmark'},
{name:'Carl',country:'America'},
{name:'Margareth',country:'England'},
]
});
- Controller logic for the AngularJS application.
Code explanation for Filters in Expression and Directives:
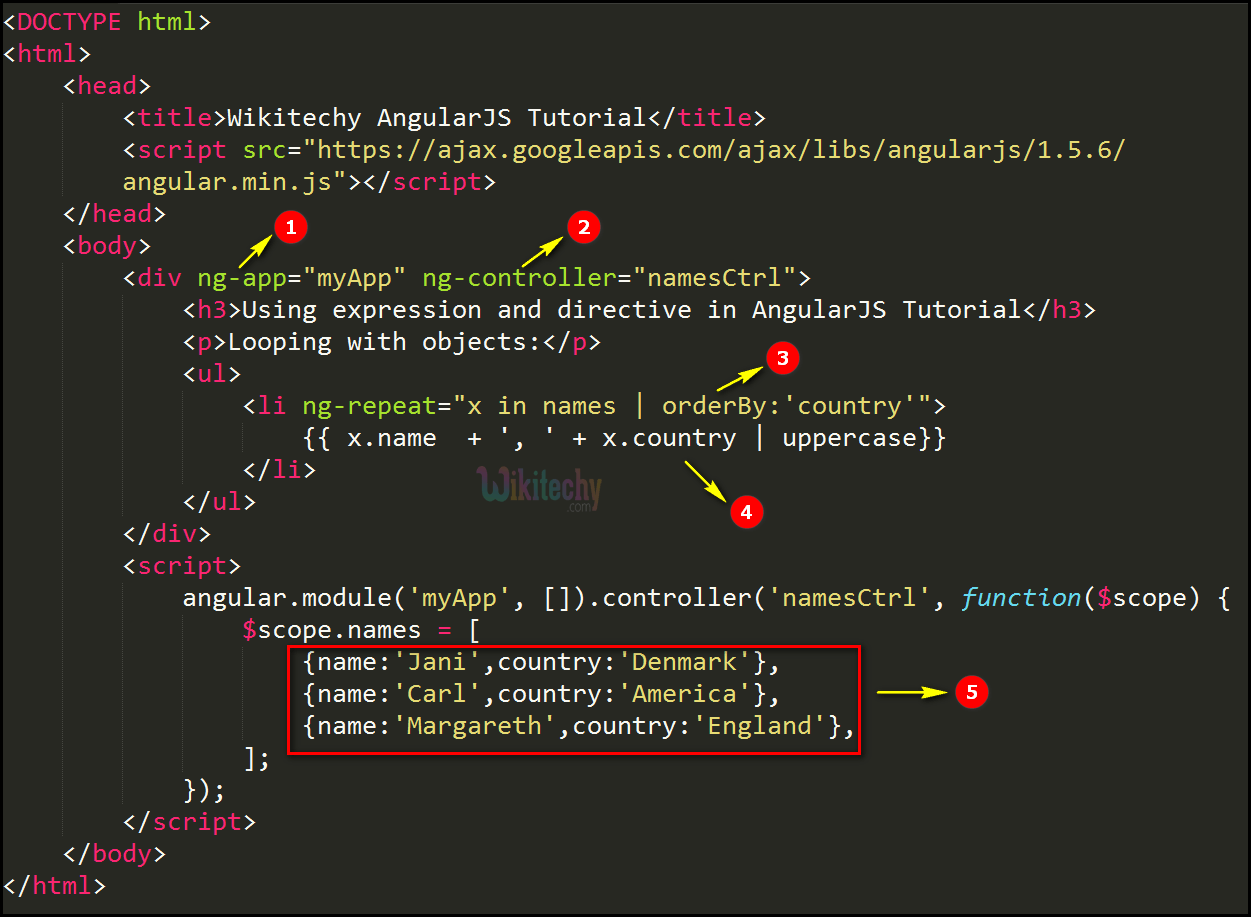
- The ng-app specifies the root element (myApp) to define AngularJS application.
- The ng-controller specifies the application controller in AngularJS the controller value is given as “namesCtrl”.
- Here the orderBy filter using by directive (like ng-repeat) and the output will be displayed in the <li> tag.
- The uppercase filter is using by expression ( { { } } ) and the output will be displayed in the <li> tag.
- $scope.name is declare the objects as name and country.
Sample output for Filters in Expression and Directives:
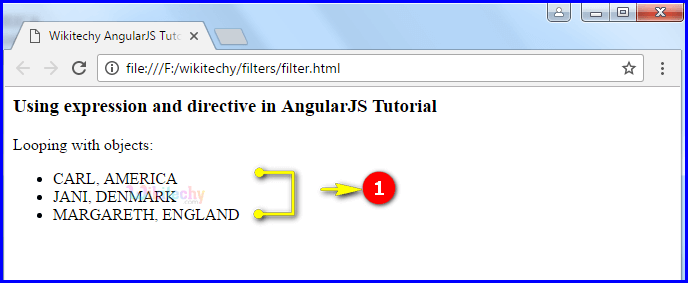
- The output displays the country in orderBy using directive as well as the output displays the name and country in uppercase using expression.
AngularJS Filters list:
Filter | Description |
---|---|
currency | It is used to formats a number as a currency(i.e $589) Current Locale is the default symbol. |
date | It is used to design a date for specified format. |
filter | It is used to select a subset of items from an array and it returns the new array. |
json | It is used to convert a JavaScript object into JSON string. |
limitTo | It is used to returns an array or a string containing only a specified number of elements |
lowercase | It is used to converts string to a lowercase. |
number | It is used to formats a number to a string or text. |
orderBy | It is used to specify orders an array by an expression |
uppercase | It is used to converts string to a uppercase. |