AngularJS LimitTo
- The limitTo filter is used to filter an array or string or number, which returns only the specified number of elements.
- Mostly the json filter is useful for debugging an application. If you used positive number (like 2) then the elements are taken from the beginning of the array or number or string.
- If you used negative number (like -2) then the elements are taken from the end of the array or number or string.
Syntax for limitTo filter in AngularJS :
{{ object | limitTo : limit : begin }}
Parameter value for limitTo filter in AngularJS :
Value | Type | Description |
---|---|---|
limit | string number |
The length of the return array or string. If the type of the value is a number. It is used to define how many elements to return. |
string number |
number |
The begin value is used to define where to be begin the limitation. This is an optional value. The default value of begin is 0. |
Sample coding for limitTo filter in AngularJS :
Tryit<!DOCTYPE html>
<html>
<head>
<title>Wikitechy AngularJS Tutorials</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.5.6/
angular.min.js"> </script>
</head>
<body>
<div ng-app="myApp" ng-controller="limitCtrl">
<h2>Wikitechy limitTo filter in AngularJS</h2>
<ul>
<h3>Display the first two items from a {{flowers}} array.</h3>
<li ng-repeat="x in flowers| limitTo : 2 ">{{ x }}</li>
<h3>Display a last two items from a {{flowers}} array.</h3>
<li ng-repeat="x in flowers| limitTo : -2 ">{{ x }}</li>
<h3>Display the first two items from a "{{word}}" string.</h3>
<li ng-repeat="x in word| limitTo : 2 ">{{ x }}</li>
<h3>Display two items from a " {{ number }} " number, starting
at position 1.</h3>
<li ng-repeat="x in number| limitTo : 2 : 1 ">{{ x }}
</li>
</ul>
</div>
<script>
var app = angular.module( 'myApp', [] );
app.controller('limitCtrl', function($scope) {
$scope.flowers = ["Lily", "Rose", "Jasmine","Poppy"];
$scope.word = "welcome";
$scope.number = 231291171229;
});
</script>
</body>
</html>
limitTo filter in AngularJS:
<li ng-repeat="x in flowers| limitTo : 2 "> {{ x }} </li>
<li ng-repeat="x in flowers| limitTo : -2 "> {{ x }} </li>
<li ng-repeat="x in word| limitTo : 2 "> {{ x }} </li>
<li ng-repeat="x in number| limitTo : 2 : 1 ">{{ x }} </li>
- The limitTo filter is used in AngularJS application with limit and begin value.
Data:
- Collection of data has been defined in AngularJS application.
flowers = ["Lily", "Rose", "Jasmine","Poppy"];
word = "welcome";
number = 231291171229;
Logic:
- Controller logic for the AngularJS Application.
app.controller('limitCtrl', function($scope) {
$scope.flowers = ["Lily", "Rose", "Jasmine","Poppy"];
$scope.word = "welcome";
$scope.number = 231291171229;
});
HTML:
- Viewable HTML contents in AngularJS Application.
<div ng-app="myApp" ng-controller="limitCtrl">
<h2>Wikitechy limitTo filter in AngularJS</h2>
<ul>
<h3>Display the first two items from a {{flowers}} array.</h3>
<li ng-repeat="x in flowers| limitTo : 2 ">{{ x }}</li>
<h3>Display a last two items from a {{flowers}} array.</h3>
<li ng-repeat="x in flowers| limitTo : -2 ">{{ x }}</li>
<h3>Display the first two items from a "{{word}}" string.</h3>
<li ng-repeat="x in word| limitTo : 2 ">{{ x }}</li>
<h3>Display two items from a " {{ number }} " number, starting
at position 1.</h3>
<li ng-repeat="x in number| limitTo : 2 : 1 ">{{ x }}</li>
</ul>
</div>
Code Explanation for limitTo filter in AngularJS:
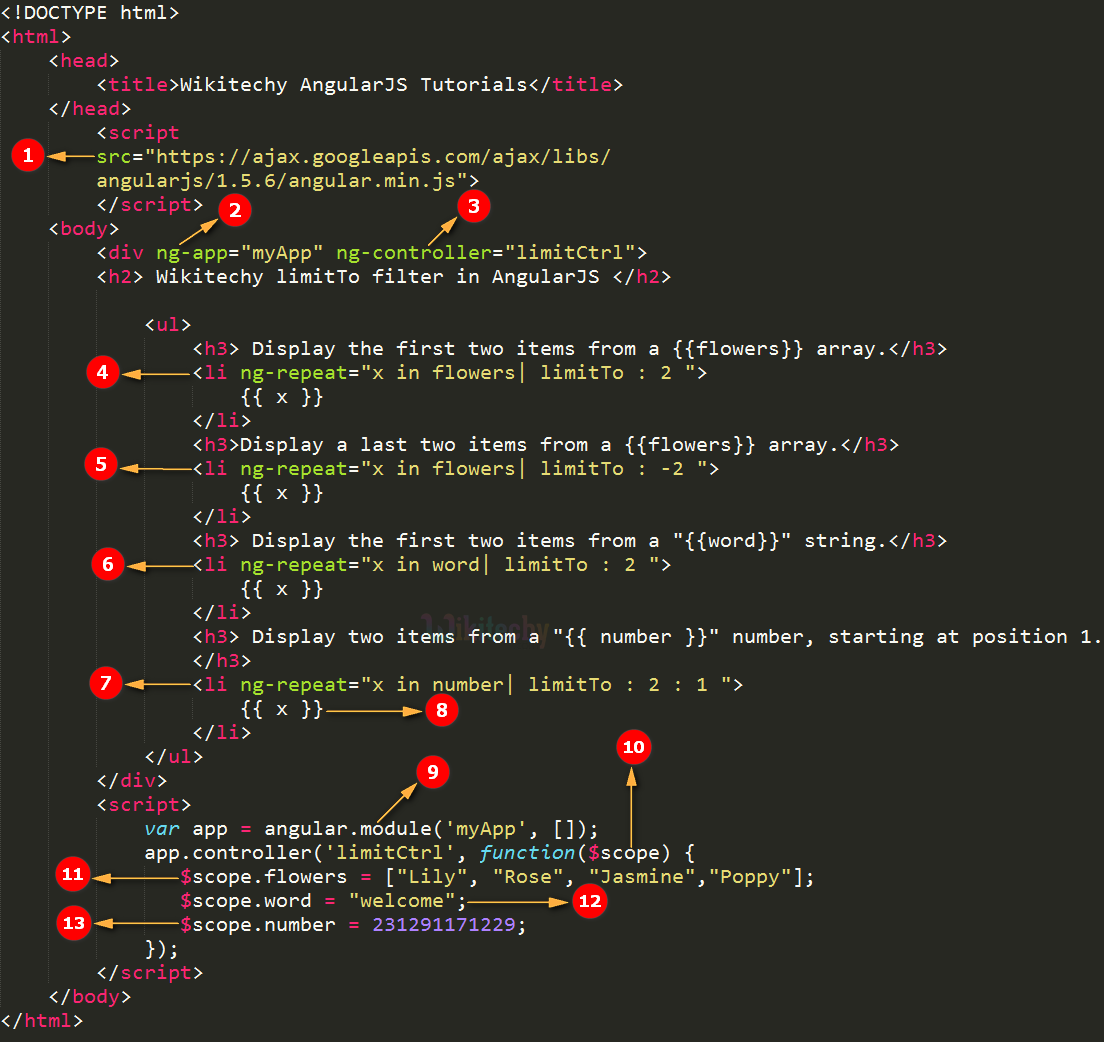
- AngularJS is distributed as a JavaScript file, and can be added to a HTML page with a <script> tag.
- The AngularJS application is defined by ng-app="myApp". The application runs inside the <div> tag. It’s also used to define a <div> tag as a root element.
- The ng-controller=”limitCtrl” is an AngularJS directive. It is used to define a controller name as “limitCtrl”.
- <li ng-repeat"x in flowers| limitTo : 2 "> Here, the limitTo is used to display a first two items from flowers array because the limit value is 2.
- <li ng-repeat="x in flowers| limitTo : -2 "> here, the limitTo is used to display a last two items from flowers array because the limit value is -2.
- <li ng-repeat="x in word| limitTo : 2 "> Here, the limitTo is used to displays a first two items from the string word because the limit value is 2.
- <li ng-repeat="x in number| limitTo: 2: 1"> Here, the limitTo is used to display a two items from the number because the limit value is 2 and here, the begin value 1 is used to specifies that the limitation will be start at position 1.
- The {{ x }} is used to bind a data in AngularJS application.
- Here we create a module by using angular.module function.
- Here we have declared a controller limitCtrl module using apps.controller() function. The value of the controller modules is stored in scope object. In AngularJS, $scope is passed as first argument to apps.controller during its constructor definition.
- Here we have set the value of $scope.flowers array as [“Lily”, “Rose”, “Jasmine”, “Poppy”].
- Here we have set the value of $scope.word as “welcome”.
- Here we have set the value of $scope.number as 231291171229;
Sample Output for limitTo filter in AngularJS :
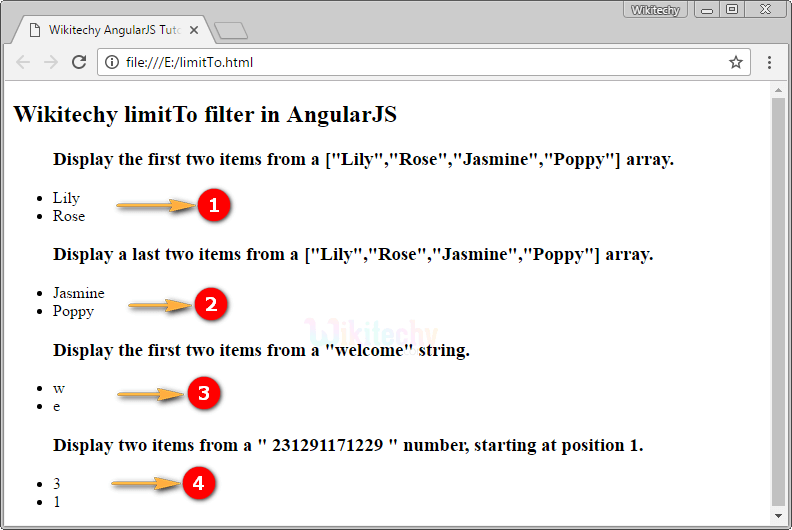
- Display a first two items (Lily, Rose) from a flowers array because the limitTo filter is used 2 as a limit value.
- Display a last two items (Jasmine, Poppy) from a flowers array because the limitTo filter is used -2 as a limit value.
- Display a first two items (w, e) from a welcome string because the limitTo filter is used 2 as a limit value.
- Display a two items (3, 1) from a number “231291171229” (Example array position is [01234567……]) because the limitTo filter is used 2 as a limit value. And here, the begin value 1 is used to specifies that the limitation will be start at position 1.