AngularJS Number
- The number filter is used to format a number as a string or text.
- The input value must be a number, otherwise it will just be return.
- The fractionSize value is used to define the number of decimal.
Syntax for number filter in AngularJS:
{{ number_expression | number : fractionSize }}
Parameter value for number filter in AngularJS:
Value | Type | Description |
---|---|---|
fractionSize | number string |
The fractionSize is an optional value. if The value must be a number. It is used to specifying the number of decimal. The default value of fractionSize is 3. |
Sample coding for number filter in AngularJS:
Tryit<!DOCTYPE html>
<html>
<head>
<title>Wikitechy AngularJS Tutorials</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.5.6/
angular.min.js"> </script>
</head>
<body>
<div ng-app="myApp" ng-controller="numberCtrl">
<h1>Wikitechy number filter in AngularJS</h1>
<h3>The original number : {{value}}</h3>
<h3>Default formatting: {{ value | number}}</h3>
<h3>No fractions : {{ value | number: 0 }}</h3>
<h3>Negative number: {{ -value | number: 4 }} </h3>
</div>
<script>
var app = angular.module( 'myApp', [] );
app.controller('numberCtrl', function($scope) {
$scope. value = 2312.9117;
});
</script>
</body>
</html>
number Filter in AngularJS:
- The number filter used to displays a different number format in our AngularJS application.
<h3>The original number : {{value}}</h3>
<h3>Default formatting: {{ value | number}}</h3>
<h3>No fractions : {{ value | number: 0 }}</h3>
<h3>Negative number: {{ -value | number: 4 }} </h3>
Data:
- The data has been defined for our AngularJS Application.
value = 2312.9117;
Logic:
- Controller logic for the AngularJS Application.
app.controller('numberCtrl', function($scope)
{
$scope. value = 2312.9117;
});
HTML:
- Viewable HTML contents in AngularJS Application.
<div ng-app="myApp" ng-controller="numberCtrl">
<h1>Wikitechy number filter in AngularJS</h1>
<h3>The original number : {{value}}</h3>
<h3>Default formatting: {{ value | number}}</h3>
<h3>No fractions : {{ value | number: 0 }}</h3>
<h3>Negative number: {{ -value | number: 4 }} </h3>
</div>
Code Explanation for number filter in AngularJS:
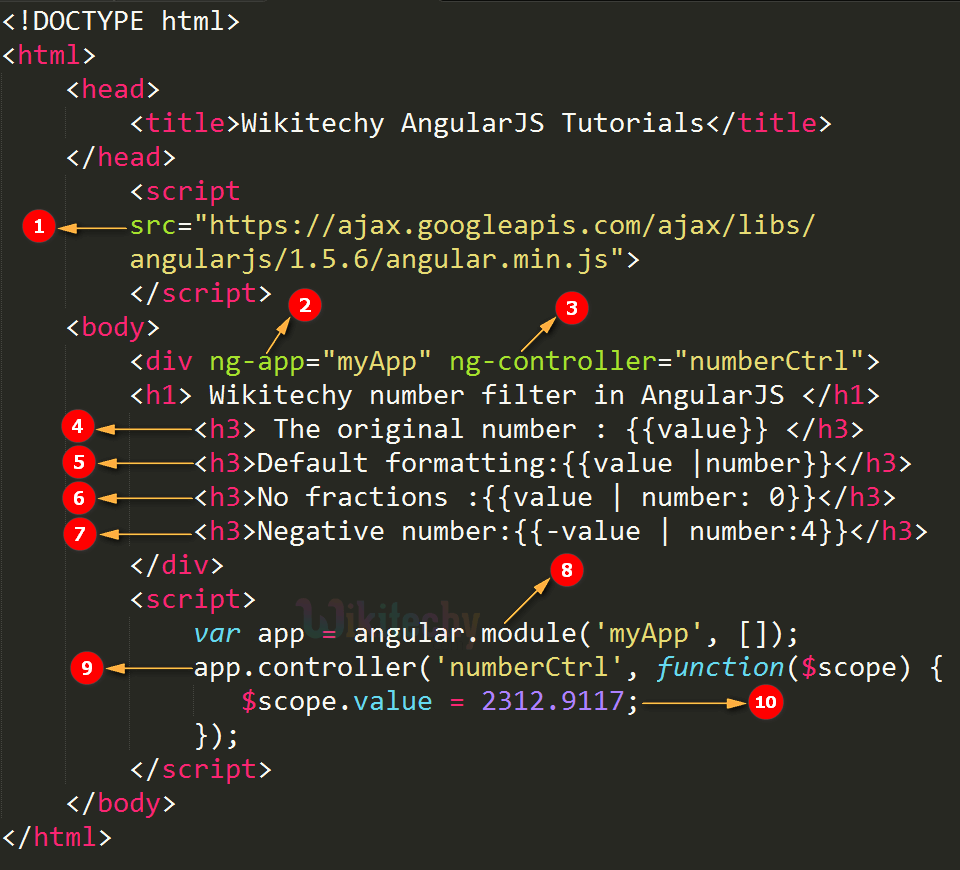
- AngularJS is distributed as a JavaScript file, and can be added to a HTML page with a <script> tag.
- The AngularJS application is defined by ng-app="myApp". The application runs inside the <div> tag. It’s also used to define a <div> tag as a root element.
- The ng-controller=”numberCtrl” is an AngularJS directive. It is used to define a controller name as “numberCtrl”.
- {{value}} is used to bind a input value.
- The {{value | number}} is used to bind a default number format. Here the original input value is displayed with the comma and three decimal point, for example (2,312.912)
- The {{value | number: 0}} is used to bind a no fraction number format. Here the input value displayed without decimal, for example (2,313).
- The {{-value | number: 4}} is used to bind a negative number format. Here the input number is displayed with negative symbol and four decimal point, for example (-2,312.9117)
- The angular.module function is used to create a Module. We have passed an empty array to it.
- Here we have declared a controller numberCtrl module using apps.controller() function. The value of the controller modules is stored in scope object. In AngularJS, $scope is passed as first argument to apps.controller during its constructor definition.
- Here we have set the value of $scope.value=2,312.9117; which are to be used to display the number format values in the HTML <div> element.
Sample Output for number filter in AngularJS:
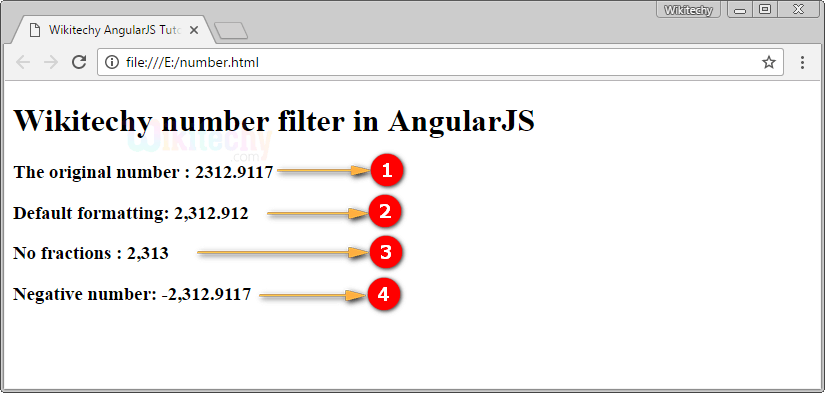
- The output displays an original input value by using {{value}}.
- The output displays a default number formatting by using a number filter.
- The output displays a no fraction number format by using a number filter.
- The output displays a negative number format by using a number filter.