Ruby on Rails - Ruby Rails Session - ruby on rails tutorial - rails guides - rails tutorial - ruby rails
What is rails Session in Ruby?
- To save data across multiple requests, you can use either the session or the flash hashes.
- A flash stores a value (normally text) until the next request, while a session stores data during the complete session.
- A session usually consists of a hash of values and a session ID, usually a 32-character string, to identify the hash.
- Every cookie sent to the client's browser includes the session ID. And the other way round: the browser will send it to the server on every request from the client.
- In Rails, you can save and retrieve values using the session method.
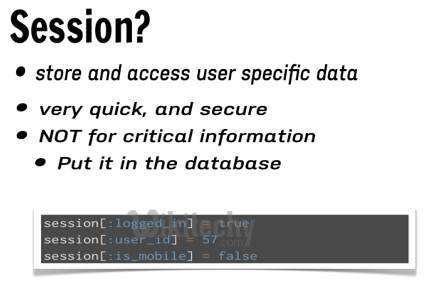
session[:user] = @user
flash[:message] = "Data was saved successfully"
<%= link_to "login", :action => 'login' unless session[:user] %>
<% if flash[:message] %>
<div><%= h flash[:message] %></div>
<% end %>
Clicking "Copy Code" button will copy the code into the clipboard - memory. Please paste(Ctrl+V) it in your destination. The code will get pasted. Happy coding from Wikitechy - ruby on rails tutorial - rails guides - ruby rails - rubyonrails - learn ruby on rails - team
It's possible to turn off session management
session :off # turn session management off
session :off, :only => :action # only for this :action
session :off, :except => :action # except for this action
session :only => :foo, # only for :foo when doing HTTPS
:session_secure => true
session :off, :only=>:foo, # off for foo,if uses as Web Service
:if => Proc.new { |req| req.parameters[:ws] }
Clicking "Copy Code" button will copy the code into the clipboard - memory. Please paste(Ctrl+V) it in your destination. The code will get pasted. Happy coding from Wikitechy - ruby on rails tutorial - rails guides - ruby rails - rubyonrails - learn ruby on rails - team
Rails session:
- Rails session is only available in controller or view and can use different storage mechanisms.
- It is a place to store data from first request that can be read from later requests.
Following are some storage mechanism for sessions in Rails:
- ActionDispatch::Session::CookieStore - Stores everything on the client.
- ActionDispatch::Session::CacheStore - Stores data in the Rails cache.
- ActionDispatch::Session::ActiveRecordStore - Stores data in the database using Active Record.
- ActionDispatch::Session::MemCacheStore - Stores data in a memcached cluster
- All the storage mechanisms use cookie to store a unique ID for each session.
- Complex objects should not be stored in the session, as server may not reassemble them between requests which will ultimately results in error.
Accessing the Session
- The session can be accessed through the session instance method.
- If sessions will not be accessed in action's code, they will not be loaded.
- Session values are stored using key/value pair like a hash.
- They are usually 32 bit character long string.
In Rails, data can be save and retrieve using session method:
session[:user_id] = @current_user.id User.find(session[:user_id])
Clicking "Copy Code" button will copy the code into the clipboard - memory. Please paste(Ctrl+V) it in your destination. The code will get pasted. Happy coding from Wikitechy - ruby on rails tutorial - rails guides - ruby rails - rubyonrails - learn ruby on rails - team
To store data in the session, assign it to the key:
class LoginsController < ApplicationController
# "Create" a login, aka "log the user in"
def create
if user = User.authenticate(params[:username], params[:password])
# Save the user ID in the session so it can be used in
# subsequent requests
session[:current_user_id] = user.id
redirect_to root_url
end
end
end
To remove data from the session, assign that key to be nil.
class LoginsController < ApplicationController
# "Delete" a login, aka "log the user out"
def destroy
# Remove the user id from the session
@_current_user = session[:current_user_id] = nil
redirect_to root_url
end
end
Clicking "Copy Code" button will copy the code into the clipboard - memory. Please paste(Ctrl+V) it in your destination. The code will get pasted. Happy coding from Wikitechy - ruby on rails tutorial - rails guides - ruby rails - rubyonrails - learn ruby on rails - team
To reset the entire session, use reset_session.
ruby on rails tutorial tags - ruby , rail , ruby on rails , rail forum , ruby on rails tutorial , ruby tutorial , rails guides , rails tutorial , learn ruby
Session Example:
- We will create a simple log in form using session.
- Once a user is signed in, his credentials will be saved.
- Only signed in users will be able to log in. You can also view all the sign in users.
Step 1 Create an application log
rails new log
Clicking "Copy Code" button will copy the code into the clipboard - memory. Please paste(Ctrl+V) it in your destination. The code will get pasted. Happy coding from Wikitechy - ruby on rails tutorial - rails guides - ruby rails - rubyonrails - learn ruby on rails - team
Step 2 Change your directory to log
cd log
Clicking "Copy Code" button will copy the code into the clipboard - memory. Please paste(Ctrl+V) it in your destination. The code will get pasted. Happy coding from Wikitechy - ruby on rails tutorial - rails guides - ruby rails - rubyonrails - learn ruby on rails - team
Step 3
Go to the Gemfile of your application. Activate the line gem 'bcrypt' and deactivate the line gem 'jbuilder' in the file.Step 4 Now run the bundle
bundle
Clicking "Copy Code" button will copy the code into the clipboard - memory. Please paste(Ctrl+V) it in your destination. The code will get pasted. Happy coding from Wikitechy - ruby on rails tutorial - rails guides - ruby rails - rubyonrails - learn ruby on rails - team
Step 5 Create a controller.
rails g controller Page index
Clicking "Copy Code" button will copy the code into the clipboard - memory. Please paste(Ctrl+V) it in your destination. The code will get pasted. Happy coding from Wikitechy - ruby on rails tutorial - rails guides - ruby rails - rubyonrails - learn ruby on rails - team
Step 6 Change the config/routes.rb file.
Rails.application.routes.draw do
get 'page/index'
root 'page#index'
end
Clicking "Copy Code" button will copy the code into the clipboard - memory. Please paste(Ctrl+V) it in your destination. The code will get pasted. Happy coding from Wikitechy - ruby on rails tutorial - rails guides - ruby rails - rubyonrails - learn ruby on rails - team
Step 7 Generate a user scaffold.
rails g scaffold User first_name last_name email password:digest
Clicking "Copy Code" button will copy the code into the clipboard - memory. Please paste(Ctrl+V) it in your destination. The code will get pasted. Happy coding from Wikitechy - ruby on rails tutorial - rails guides - ruby rails - rubyonrails - learn ruby on rails - team
Step 8 Migrate your database.
rake db:migrate
Clicking "Copy Code" button will copy the code into the clipboard - memory. Please paste(Ctrl+V) it in your destination. The code will get pasted. Happy coding from Wikitechy - ruby on rails tutorial - rails guides - ruby rails - rubyonrails - learn ruby on rails - team
Step 9 Go to app/models/user.rb file and write following code.
class User < ActiveRecord::Base
has_secure_password
validates :first_name,
presence: true
validates :last_name,
presence: true
validates :email,
presence: true,
uniqueness: true,
format: {
with: /\A([^@\s]+)@((?:[-a-z0-9]+\.)+[a-z]{2,})\z/i
}
def to_s
"#{first_name} #{last_name}"
end
end
Clicking "Copy Code" button will copy the code into the clipboard - memory. Please paste(Ctrl+V) it in your destination. The code will get pasted. Happy coding from Wikitechy - ruby on rails tutorial - rails guides - ruby rails - rubyonrails - learn ruby on rails - team
Step 10 We need a session mechanism to create a login and logout procedure.
rails g controller sessions new create destroy
Clicking "Copy Code" button will copy the code into the clipboard - memory. Please paste(Ctrl+V) it in your destination. The code will get pasted. Happy coding from Wikitechy - ruby on rails tutorial - rails guides - ruby rails - rubyonrails - learn ruby on rails - team
Step 11 Go to config/routes.rb file and change the following data.
Rails.application.routes.draw do
resources :sessions, only: [:new, :create, :destroy]
get 'signup', to: 'users#new', as: 'signup'
get 'login', to: 'sessions#new', as: 'login'
get 'logout', to: 'sessions#destroy', as: 'logout'
resources :users
get 'page/index'
root 'page#index'
end
Clicking "Copy Code" button will copy the code into the clipboard - memory. Please paste(Ctrl+V) it in your destination. The code will get pasted. Happy coding from Wikitechy - ruby on rails tutorial - rails guides - ruby rails - rubyonrails - learn ruby on rails - team
Step 12 Create a login form in app/views/sessions/new.html.erb file.
<h1>Log In</h1>
<%= form_tag sessions_path do %>
<div>
<%= label_tag :email %><br>
<%= text_field_tag :email %>
</div>
<div>
<%= label_tag :password %><br>
<%= password_field_tag :password %>
</div>
<div>
<%= submit_tag "Log In" %>
</div>
<% end %>
Clicking "Copy Code" button will copy the code into the clipboard - memory. Please paste(Ctrl+V) it in your destination. The code will get pasted. Happy coding from Wikitechy - ruby on rails tutorial - rails guides - ruby rails - rubyonrails - learn ruby on rails - team
Step 13 Go to app/controllers/sessions_controller.rb file and write the following code.
class SessionsController < ApplicationController
def new
end
def create
user = User.find_by_email(params[:email])
if user && user.authenticate(params[:password])
session[:user_id] = user.id
redirect_to root_url, notice: 'Logged in!'
else
render :new
end
end
def destroy
session[:user_id] = nil
redirect_to root_url, notice: 'Logged out!'
end
end
Clicking "Copy Code" button will copy the code into the clipboard - memory. Please paste(Ctrl+V) it in your destination. The code will get pasted. Happy coding from Wikitechy - ruby on rails tutorial - rails guides - ruby rails - rubyonrails - learn ruby on rails - team
Step 14 We need to create a current_user method to access current user in the application. Go to the app/controllers/application_controller.rb file and write the following code.
class ApplicationController < ActionController::Base
# Prevent CSRF attacks by raising an exception.
# For APIs, you may want to use :null_session instead.
protect_from_forgery with: :exception
private
def current_user
User.where(id: session[:user_id]).first
end
helper_method :current_user
end
Clicking "Copy Code" button will copy the code into the clipboard - memory. Please paste(Ctrl+V) it in your destination. The code will get pasted. Happy coding from Wikitechy - ruby on rails tutorial - rails guides - ruby rails - rubyonrails - learn ruby on rails - team
Step 15 Go to the app/views/layouts/application.html.erb file and write the following code in the body.
<div id="user_header">
<% if current_user %>
Logged in as <%= current_user.email %>.
<%= link_to "Log Out", logout_path %>
<% else %>
<%= link_to "Sign Up", signup_path %> or
<%= link_to "Log In", login_path %>
<% end %>
</div>
<% flash.each do |key, value| %>
<%= content_tag(:div, class: "alert alert-#{key}") do %>
<p><%= value %></p>
<% end %>
<% end %>
Clicking "Copy Code" button will copy the code into the clipboard - memory. Please paste(Ctrl+V) it in your destination. The code will get pasted. Happy coding from Wikitechy - ruby on rails tutorial - rails guides - ruby rails - rubyonrails - learn ruby on rails - team
Step 16 Delete following line from app/views/users/show.html.erb and app/views/layouts/index.html.erb files.
<p id="notice"><%= notice %></p>
Clicking "Copy Code" button will copy the code into the clipboard - memory. Please paste(Ctrl+V) it in your destination. The code will get pasted. Happy coding from Wikitechy - ruby on rails tutorial - rails guides - ruby rails - rubyonrails - learn ruby on rails - team
Step 17 When a new user sign up, he/she will be auto login. For this, we need to set session in the app/controllers/users_controller.rb file.
def create
@user = User.new(user_params)
if @user.save
session[:user_id] = @user.id
redirect_to root_url, notice: 'User successfully created.'
else
render :new
end
end
Clicking "Copy Code" button will copy the code into the clipboard - memory. Please paste(Ctrl+V) it in your destination. The code will get pasted. Happy coding from Wikitechy - ruby on rails tutorial - rails guides - ruby rails - rubyonrails - learn ruby on rails - team
Step 18 Start your Rails server in the console.
rails s
Clicking "Copy Code" button will copy the code into the clipboard - memory. Please paste(Ctrl+V) it in your destination. The code will get pasted. Happy coding from Wikitechy - ruby on rails tutorial - rails guides - ruby rails - rubyonrails - learn ruby on rails - team
Step 19 Go to the browser.
Localhost:3000
Clicking "Copy Code" button will copy the code into the clipboard - memory. Please paste(Ctrl+V) it in your destination. The code will get pasted. Happy coding from Wikitechy - ruby on rails tutorial - rails guides - ruby rails - rubyonrails - learn ruby on rails - team
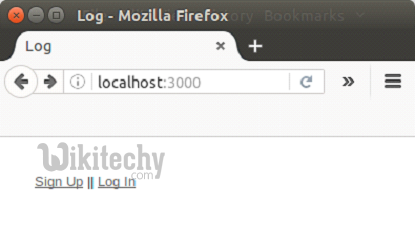
Learn ruby - ruby tutorial - signup account in ruby rails session - ruby examples - ruby programs
Let us Sign Up for a user Venkat Wikitechy.
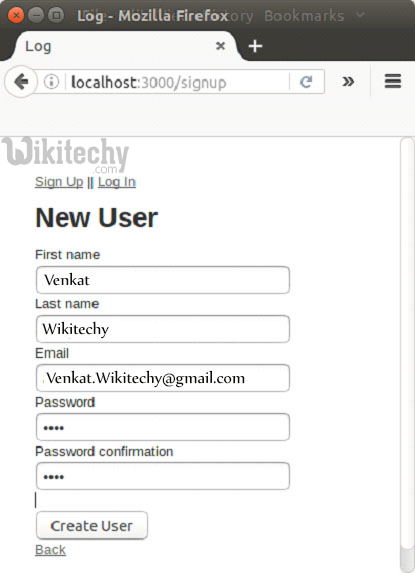
Learn ruby - ruby tutorial - create account in ruby rails session - ruby examples - ruby programs
On clicking Back button, you can see all signed in users.
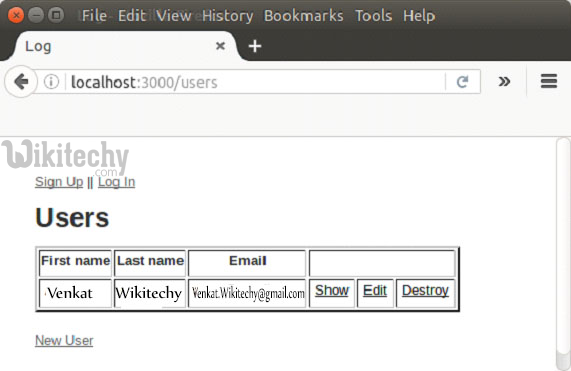
Learn ruby - ruby tutorial - signed users list in ruby rails session - ruby examples - ruby programs
Now let us log in from the user VenkatWikitechy.
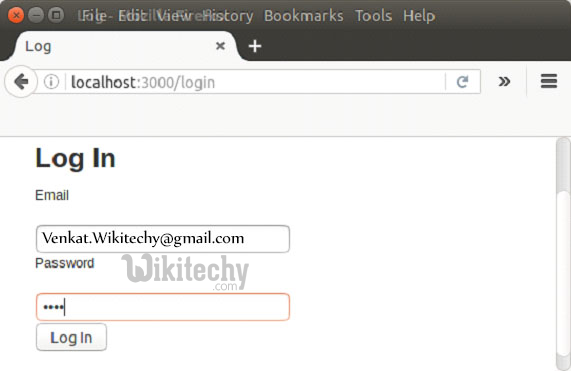
Learn ruby - ruby tutorial - login process in ruby rails session - ruby examples - ruby programs
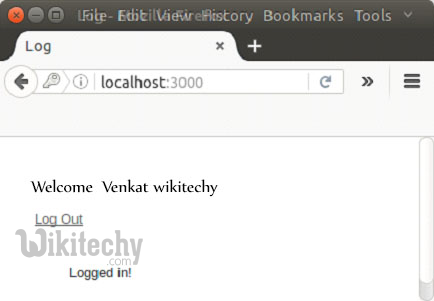
Learn ruby - ruby tutorial - loggedin in ruby rails session - ruby examples - ruby programs
ruby on rails tutorial tags - ruby , rail , ruby on rails , rail forum , ruby on rails tutorial , ruby tutorial , rails guides , rails tutorial , learn ruby
Ruby on Rails - Cookies :
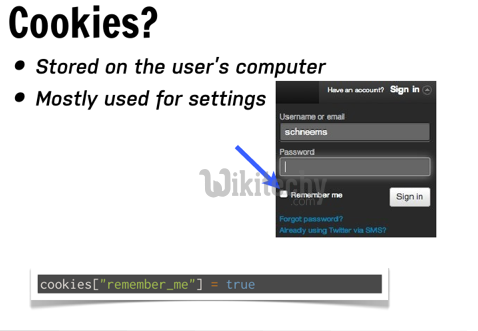
ruby on rails tutorial tags - ruby , rail , ruby on rails , rail forum , ruby on rails tutorial , ruby tutorial , rails guides , rails tutorial , learn ruby
Ruby on Rails - Integrating Flash :
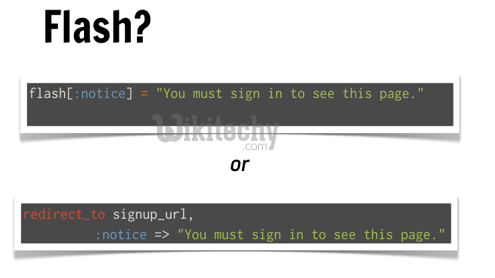