What is Maven? - learn maven - In 20 minutes
What is maven?
- Maven, a Yiddish word meaning accumulator of knowledge, was originally started as an attempt to simplify the build processes in the Jakarta Turbine project. There were several projects each with their own Ant build files that were all slightly different and JARs were checked into CVS.
Scenario :
- We will create a new trivial Hibernate Application that reads rows in a database table (shown below) and outputs them to the console.
- First, we will need a class to represent a greeting:
Sample code:
@Entity
public class Greeting {
@Id @GeneratedValue(strategy=GenerationType.IDENTITY)
@Column(name="greeting_id",nullable=false,unique=true)
private Integer id;
@Column(name="greeting_text")
private String text;
@Column(name="version") @Version
private Integer version;
public Integer getId() { return this.id; }
public void setId(Integer id) { this.id = id; }
public String getText() { return this.text; }
public void setText(String text) { this.text = text; }
}
Next, we will need a class with a static main method to actually do stuff...
public class App {
private static SessionFactory sessionFactory;
static {
try {
sessionFactory = new AnnotationConfiguration().configure().buildSessionFactory();
} catch (Throwable t) {
System.out.println("Something terrible has happened.");
t.printStackTrace();
}
}
public static SessionFactory getSessionFactory() { return App.sessionFactory; }
public void run() {
System.out.println("======================================================");
Criteria c = App.getSessionFactory().openSession().createCriteria(Greeting.class);
List<Greeting> greetings = c.list();
for (Greeting greeting : greetings) {
System.out.println(greeting.getId() + ": " + greeting.getText());
}
System.out.println("======================================================");
}
public static void main(String args[]) {
App app = new App().run());
}
}
Third, let's create the directories for our project:
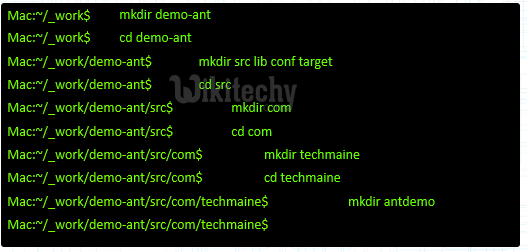
Learn maven Tutorial - maven create directory - maven Example
Here is the project structure for your reference
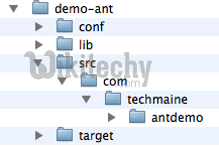
Learn maven Tutorial - maven project structure - maven Example
Visit the Hibernate Website, eventually find their download page…
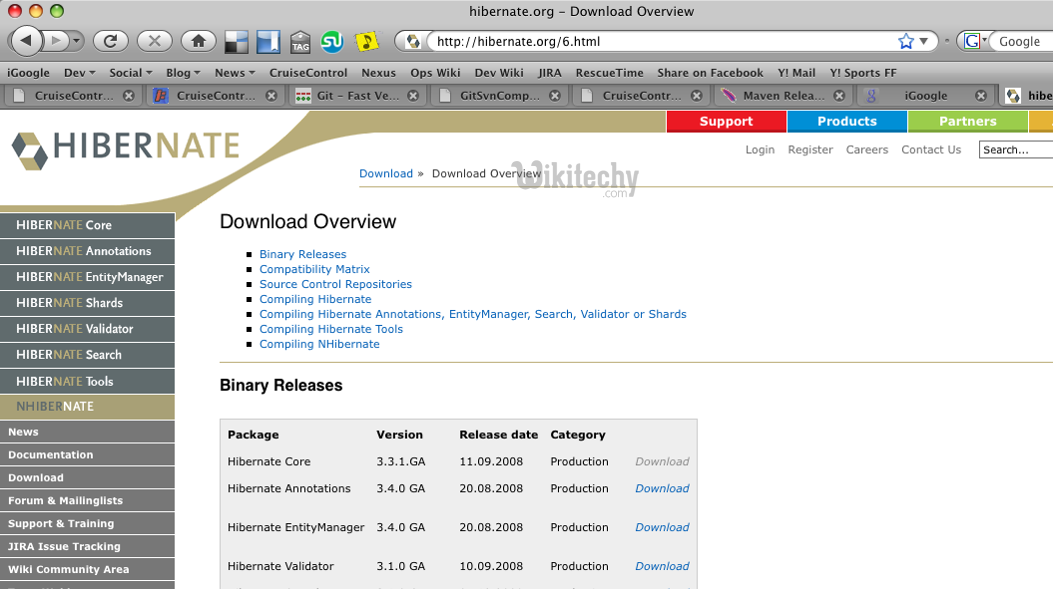
Learn maven Tutorial - maven hybernate website - maven Example
Download gigantic ZIP file
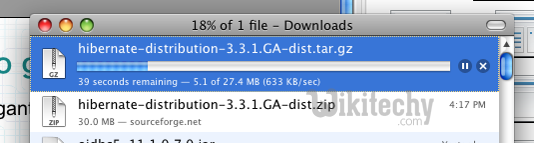
Learn maven Tutorial - maven gignatic wesite - maven Example
Unpack the ZIP file
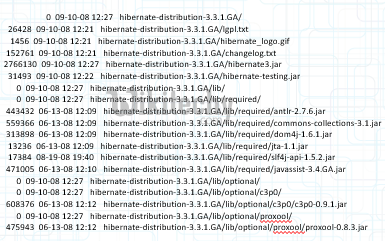
Learn maven Tutorial - maven unpack zipfile - maven Example
- Copy the jar files to your lib directory
- Option 1: Copy Everything (Lazy)
- Option 2: Copy only what you need (Tedious)
- Create your build.xml file
Create the build.xml - Start with an empty file
<?xml version="1.0"?>
<project name="antdemo" default="compile" basedir=".">
</project>
Create some variables
<!-- set global properties for this build -->
<property name="lib" value="lib"/>
<property name="src" value="src"/>
<property name="conf" value="conf"/>
<property name="target" value="target"/>
<property name="classes" value="${target}/classes"/>
Tell ant about all of your libraries
<fileset id="compile.libs" dir="${lib}">
<include name="antlr-2.7.6.jar"/>
<include name="asm.jar"/>
<include name="asm-attrs.jar"/>
<include name="cglib-2.1.3.jar"/>
<include name="commons-collections-3.2.jar"/>
<include name="commons-lang-2.3.jar"/>
<include name="commons-logging-1.0.4.jar"/>
<include name="dom4j-1.6.1.jar"/>
<include name="ejb3-persistence.jar"/>
<include name="javassist.jar"/>
<include name="jboss-archive-browsing.jar"/>
<include name="jdbc2_0-stdext.jar"/>
<include name="jta.jar"/>
<include name="xml-apis.jar"/>
<include name="xercesImpl-2.6.2.jar"/>
<include name="hibernate3.jar"/>
<include name="hibernate-annotations.jar"/>
<include name="hibernate-commons-annotations.jar"/>
<include name="hibernate-entitymanager.jar"/>
</fileset>
Tell ant about compiling
<target name="init">
<tstamp/>
<mkdir dir="${classes}"/>
</target>
<target name="compile" depends="init">
<mkdir dir="${basedir}/${classes}"/>
<javac srcdir="${basedir}/${src}"
destdir="${basedir}/${classes}"
debug="yes"
target="1.5">
<classpath>
<fileset refid="compile.libs"/>
</classpath>
</javac>
<copy file="${basedir}/${conf}/hibernate.cfg.xml"
todir="${basedir}/${classes}"/>
</target>
<target name="clean">
<delete quiet="yes" dir="${basedir}/${target}"/>
<mkdir dir="${basedir}/${target}"/>
</target>
Tell ant how to run the app
<fileset id="runtime.libs" dir="${lib}">
<include name="postgresql-8.2-507.jdbc3.jar"/>
</fileset>
<target name="run" depends="compile">
<java classname="com.techmaine.antdemo.App">
<classpath>
<fileset refid="compile.libs"/>
<fileset refid="runtime.libs"/>
<pathelement location="${basedir}/${classes}"/>
</classpath>
</java>
</target>
Maven Terminology
- With maven, you execute goals in plugins over the different phases of the build lifecycle, to generate artifacts. Examples of artifacts are jars, wars, and ears.
- These artifacts have an artifactId, a groupId, and a version.
- Together, these are called the artifact’s “coordinates.” The artifacts stored in repositories.
- Artifacts are deployed to remote repositories and installed into local repositories. A POM (Project Object Model) describes a project.
Create a Project Directory - Maven has a command for starting a project:
mvn archetype:create \
-DgroupId=com.techmaine \
-DartifactId=demo-mvn \
-DpackageName=com.techmaine.mvndemo \
-Dversion=1.0
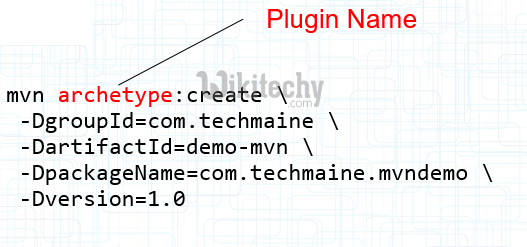
Learn maven Tutorial - maven archetype create - maven Example
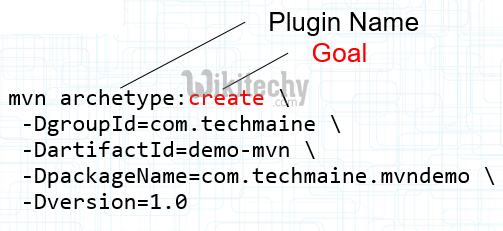
Learn maven Tutorial - maven archetype create goal - maven Example
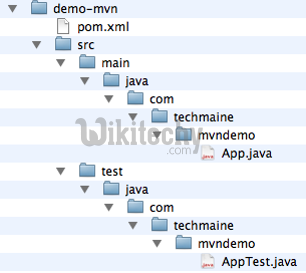
Learn maven Tutorial - maven demo - maven Example
Set up the dependencies - Open pom.xml. We need to tell maven that we have a dependency on Hibernate:
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.techmaine</groupId>
<artifactId>demo-mvn</artifactId>
<packaging>jar</packaging>
<version>1.0</version>
<name>demo-mvn</name>
<url>http://maven.apache.org</url>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>3.8.1</version>
<scope>test</scope>
</dependency>
</dependencies>
</project>
Set up the dependencies
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-annotations</artifactId>
<version>3.3.1.ga</version>
</dependency>
- We don’t need to tell Maven about any of the jars on which Hibernate depends; Maven takes care of all of the transitive dependencies for us!
Set up the compiler
- Maven assumes a default source version of 1.3. We need to tell it if we want 1.5. Here’s a preview of plugin configuration:
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>2.0.2</version>
<configuration>
<source>1.5</source>
<target>1.5</target>
</configuration>
</plugin>
</plugins>
</build>
Set up Hibernate Configuration
- Create a resources directory beneath the main (and, optionally, test) directory, and put the Hibernate configuration file there.
- Files in the resources directory get copied to the root of the classpath when packaging occurs as part of the resource:resource goal (more on that later)
- The resources directories are automatically created for many of the archetypes, but not for the quickstart archetype that we used.
Package
- Next, package everything up before we run it.
- To do this, invoke maven thusly:
- mvn package
- This is an alternate way to invoke maven. Instead of specifying a plugin and goal, you specify a phase (in this case, package is the phase). A phase is a sequenced set of goals. The package phase compiles the java classes and copies the resources
Execute
- Next, use the exec plugin to run our application:
- mvn exec:exec \
- -DmainClass=com.techmaine.mvndemo.App
Configuring Maven
- Settings Files (settings.xml)
- In ~/.m2 (per-user settings) and in Maven’s install directory, under conf (per-system settings)
- Alternate location for repository
- Proxy Configuration
- Per-server authentication settings
- Mirrors
- Download policies, for plugins and repositories; snapshots and releases.
Configuring Maven
- Project Object Model (pom.xml)
- Inherited - individual projects inherit POM attributes from parent projects, and ultimately inherit from the “Super POM”
- The Super POM is in Maven’s installation directory, embedded in the uber jar.
- The Super POM defines, among lots of other things, the default locations for the plugin and jar repositories, which is http://repo1.maven.org/maven2
Repositories
- Local - in ~/.m2/repository
- Remote - e.g., http://repo1.maven.org/maven2 or another internal company repository (any directory reachable by sftp will do).
- Contains dependencies and plugins
- Can be managed by a “Repository Manager” like Nexus
The POM
- Describes the project, declaratively
- General Information - Project Coordinates (groupId, artifactId, Version)
- Build Settings - Configuration of the plugins
- Build Environment - We can configure different profiles that can be activated programatically
- POM Relationships - Dependencies on other projects
Anatomy of a POM File
<project xmlns=<http://maven.apache.org/POM/4.0.0> >
<modelVersion>4.0.0</modelVersion>
<groupId>com.techmaine</groupId>
<artifactId>superduper</artifact>
<packaging>jar</packaging>
<version>1.0.0</version>
<name>Super Duper Amazing Deluxe Project</name>
<modules>
<!-- Sub-modules of this project -->
</modules>
<parent>
<!-- Parent POM stuff if applicable -->
</parent>
<properties>
<!-- Ad-hoc properties used in the build -->
</properties>
<dependencies>
<!-- Dependency Stuff -->
</dependencies>
<build>
<!-- Build Configuration -->
<plugins>
<!-- plugin configuration -->
</plugins>
</build>
<profiles>
<!-- build profiles -->
</profiles>
</project>
User-Defined Properties
<project xmlns=<http://maven.apache.org/POM/4.0.0> >
<modelVersion>4.0.0</modelVersion>
<name>Super Duper Amazing Deluxe Project</name>
<packaging>jar</packaging>
<groupId>com.techmaine</groupId>
<artifactId>superduper</artifact>
<version>1.0.0</version>
<parent>
<!-- Parent POM stuff if applicable -->
</parent>
<modules>
<!-- Sub-modules of this project -->
</modules>
<properties>
<!-- Ad-hoc properties used in the build -->
</properties>
<dependencies>
<!-- Dependency Stuff -->
</dependencies>
<build>
<!-- Build Configuration -->
<plugins>
<!-- plugin configuration -->
</plugins>
</build>
<profiles>
<!-- build profiles -->
</profiles>
</project>