Maven - Building Java OSGi Project with Maven and Tycho - maven tutorial
1- Introduction
- This document is based on:
- Eclipse 3.4 (LUNA)
- Tycho 0.20.0
- We must first ensure that you have installed Tycho on Eclipse. If not installed you can see the instructions here:
maven tutorial tags : apache maven , maven repository , maven central
2- Quick Create OSGi Project (SimpleOSGi)
- First we create an OSGi project quickly accordance usual way.
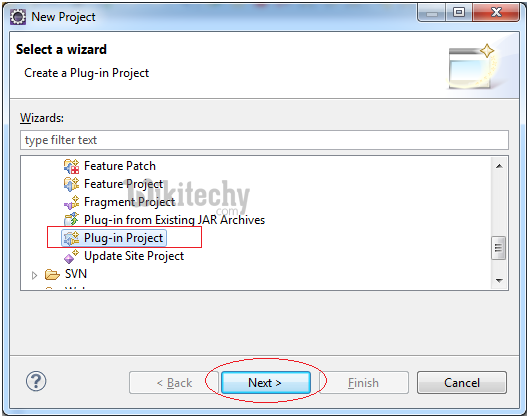
learn maven tutorial - quick create maven project step1 - maven example
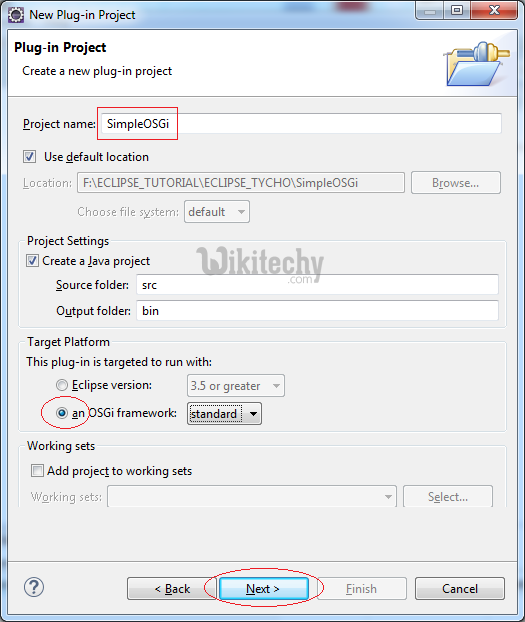
learn maven tutorial - quick create maven project step2 - maven example
- Enter:
- Version: 2.0.0.qualifier
- Activator: wikitechy.simpleosgi.Activator
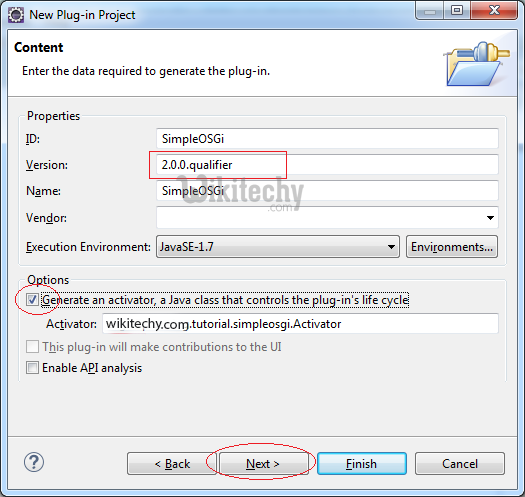
learn maven tutorial - quick create maven project step3 - maven example
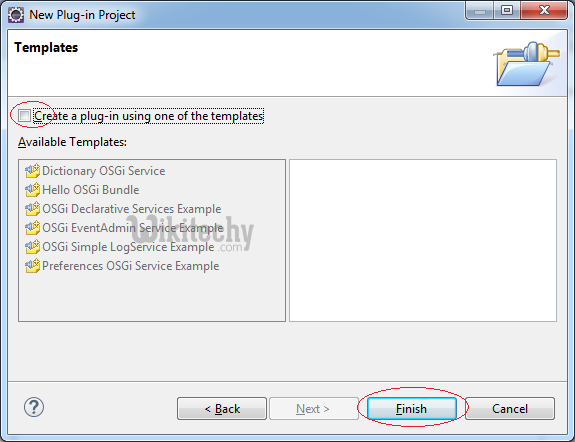
learn maven tutorial - quick create maven project step4 - maven example
- Add:
- org.eclipse.osgi
- org.eclipse.equinox.console
- org.apache.felix.gogo.command
- org.apache.felix.gogo.runtime
- org.apache.felix.gogo.shell
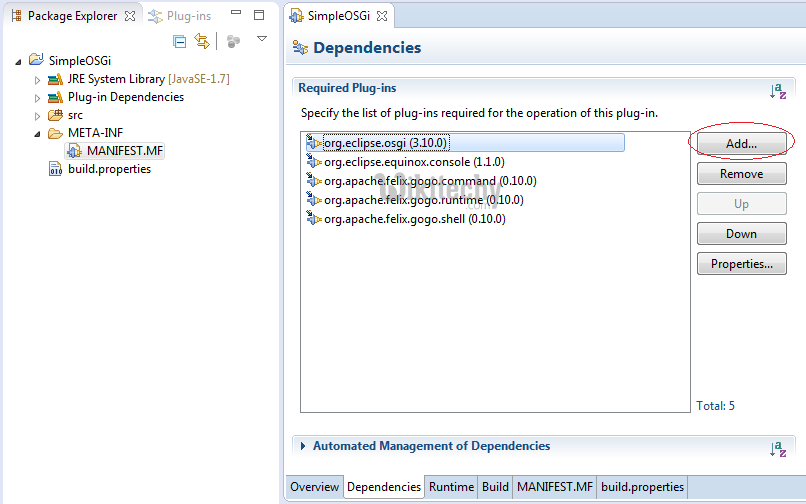
learn maven tutorial - quick create maven project step5 - maven example
Activator.java
maven tutorial tags : apache maven , maven repository , maven central
sample code
package wikitechy.tutorial.simpleosgi;
import org.osgi.framework.BundleActivator;
import org.osgi.framework.BundleContext;
public class Activator implements BundleActivator {
private static BundleContext context;
static BundleContext getContext() {
return context;
}
public void start(BundleContext bundleContext) throws Exception {
Activator.context = bundleContext;
System.out.println("SimpleOSGi Started");
}
public void stop(BundleContext bundleContext) throws Exception {
Activator.context = null;
System.out.println("SimpleOSGi Stopped");
}
}
2.1- Run OSGi (SimpleOSGi)
- We'll quickly configure and run this OSGi, to see that the status of the OSGi just created is good.
- Right-click on the Project SimpleOSGi, select "Run As / Run Configuration .."
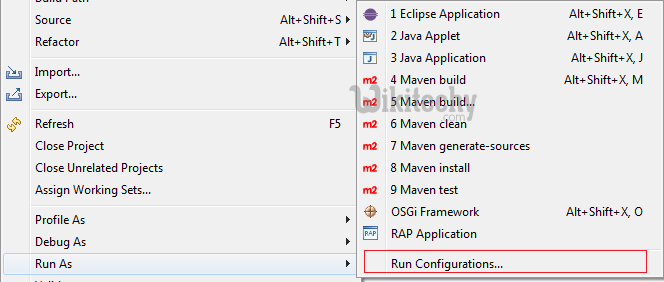
learn maven tutorial - run osgi step1 - maven example
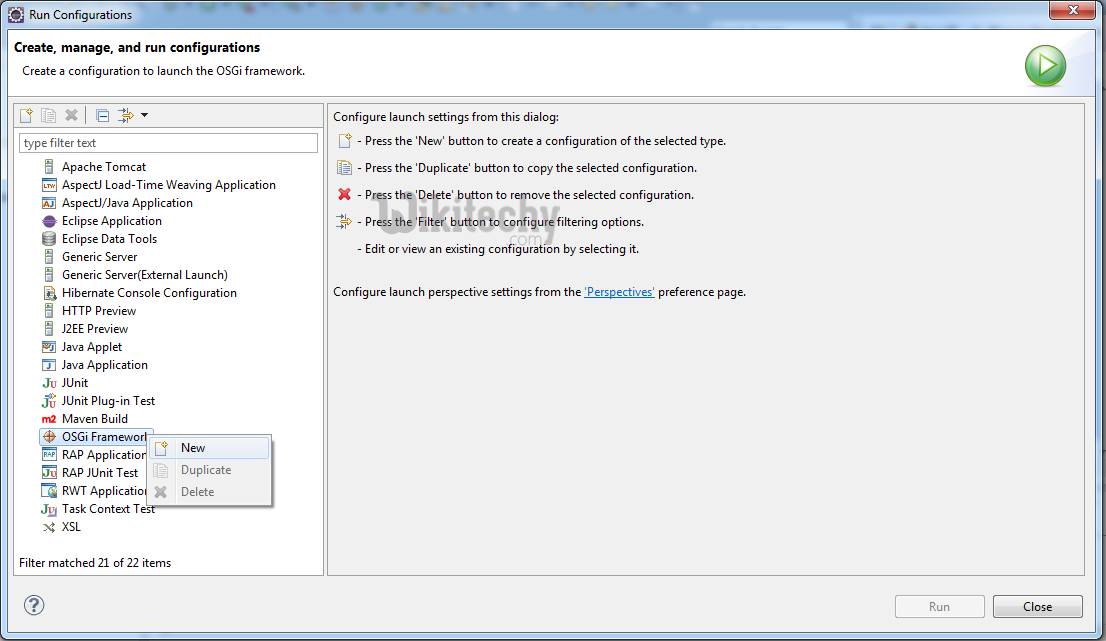
learn maven tutorial - run osgi step2 - maven example
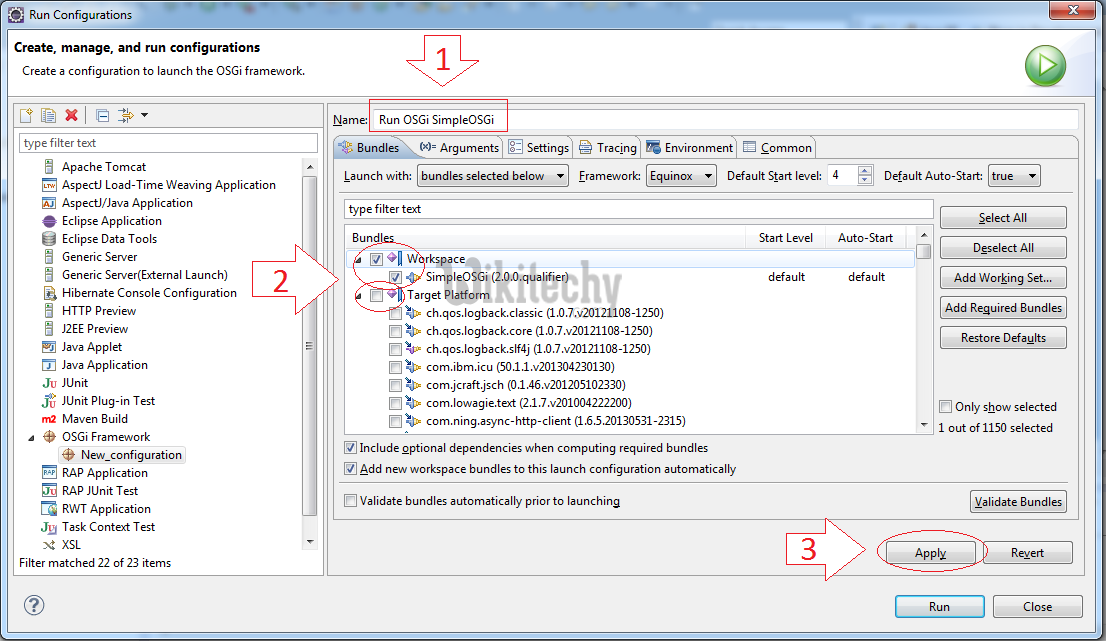
learn maven tutorial - run osgi step3 - maven example
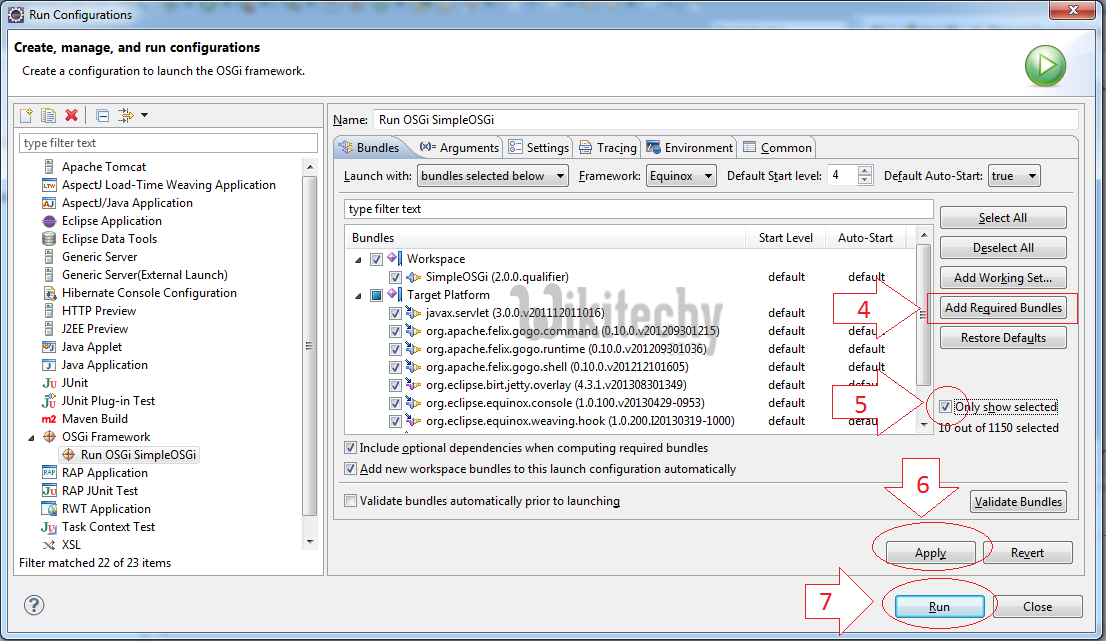
learn maven tutorial - run osgi step4 - maven example
- Running OSGi, and get successful results.
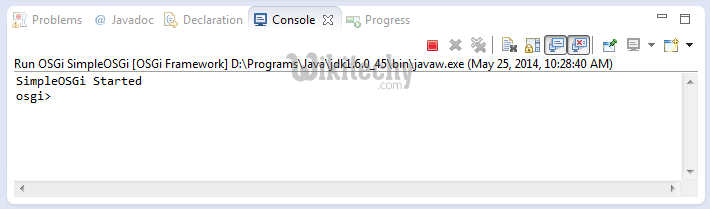
learn maven tutorial - run osgi step5 - maven example
3- Quick Create Maven Project (SimpleMaven)
- Next we will quick create Maven Project. Goals for the future, we will build 2 project (SimpleOSGi & SimpleMaven) with Maven & Tycho. And see that both project to be treated equally.
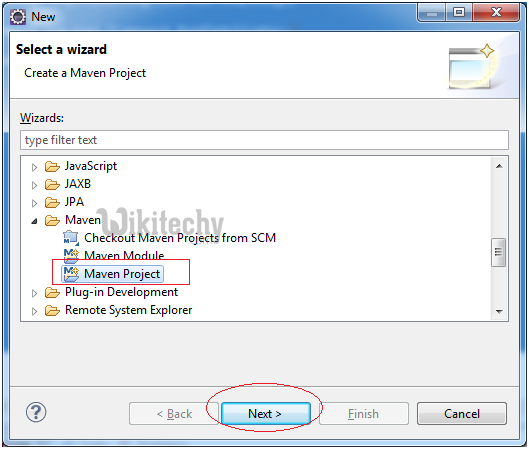
learn maven tutorial - quick create maven project step1- maven example
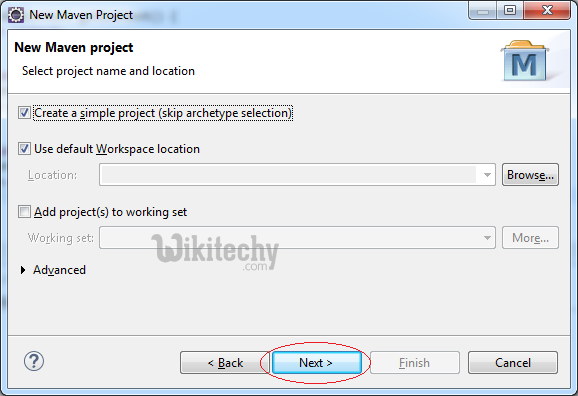
learn maven tutorial - quick create maven project step2 - maven example
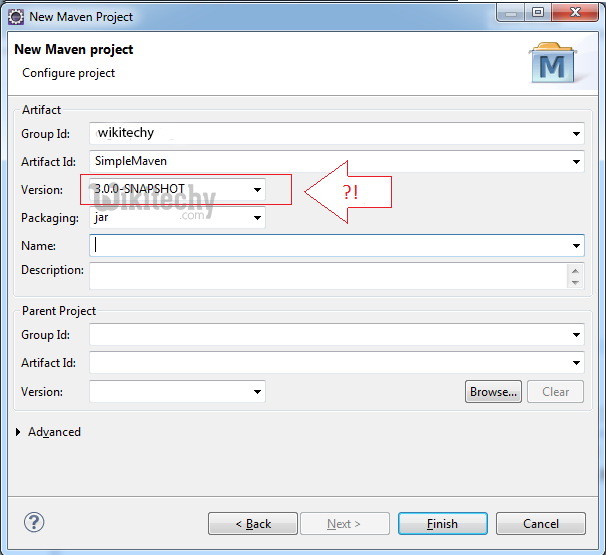
learn maven tutorial - quick create maven project step3 - maven example
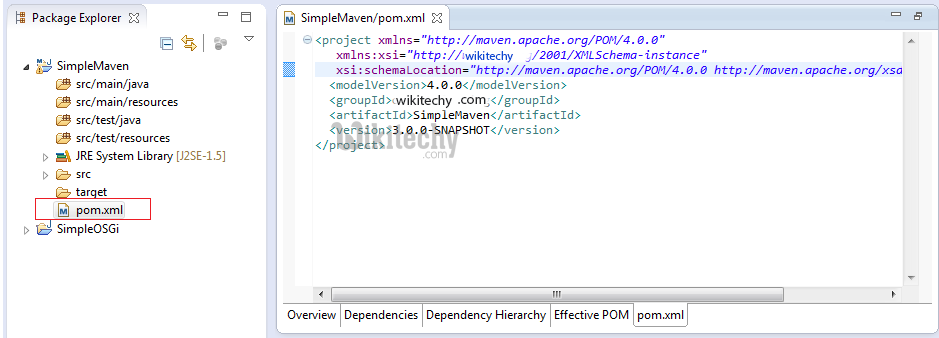
learn maven tutorial - quick create maven project step4 - maven example
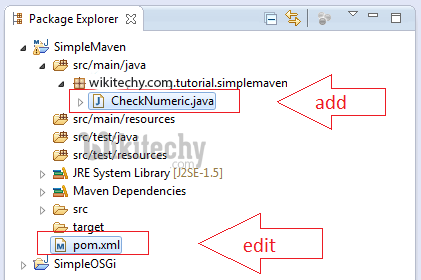
learn maven tutorial - quick create maven project step5 - maven example
maven tutorial tags : apache maven , maven repository , maven central
CheckNumeric.java
sample code
package wikitechy.tutorial.simplemaven;
import org.apache.commons.lang3.StringUtils;
public class CheckNumeric {
public static void main(String[] args) {
String text1 = "0123a4";
String text2 = "01234";
boolean result1 = StringUtils.isNumeric(text1);
boolean result2 = StringUtils.isNumeric(text2);
System.out.println(text1 + " is a numeric? " + result1);
System.out.println(text1 + " is a numeric? " + result2);
}
}
pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.o7planning</groupId>
<artifactId>SimpleMaven</artifactId>
<version>3.0.0-SNAPSHOT</version>
<dependencies>
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.3.2</version>
</dependency>
</dependencies>
</project>
4- Create MavenParent Project
- The purpose of this project to configure Maven & Tycho to build two projects ( SimpleOSGi & SimpleMaven).
- Create a common Java Project
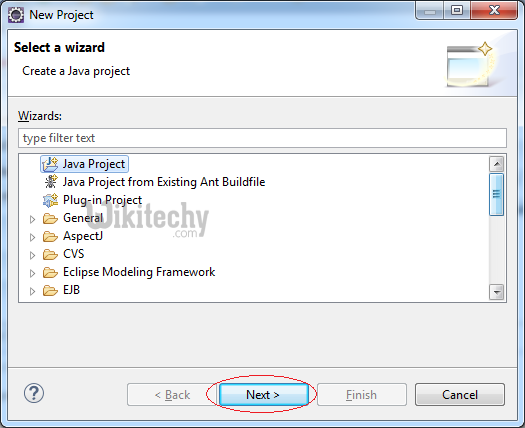
learn maven tutorial - create mavenparent project step1 - maven example
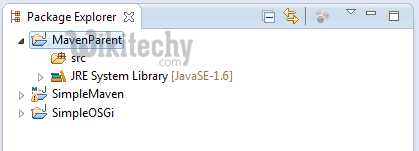
learn maven tutorial - create mavenparent project step2 - maven example
5- Configure Maven & Tycho
5.1- Configuring the OSGi project (SimpleOSGi)
- First we convert SimpleOSGi into Maven Project. SimpleOSGi will be a Maven plugin and OSGi Project
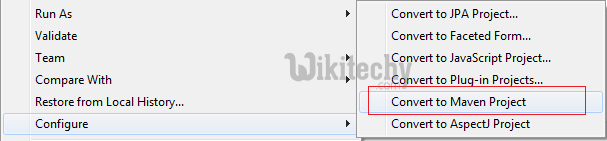
learn maven tutorial - configure maven tycho step1- maven example
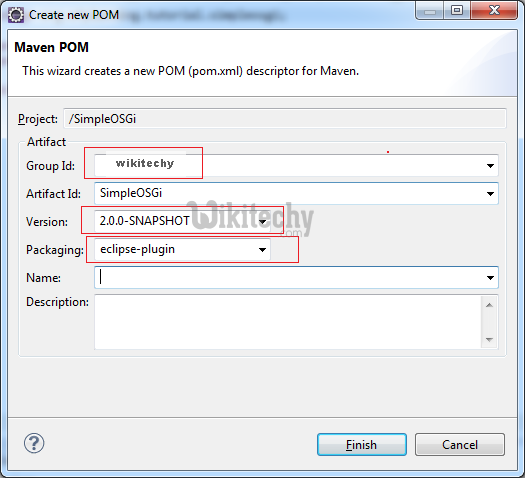
learn maven tutorial - configure maven tycho step2 - maven example
- Project has been converted into a Maven project, and have error message, you do not want to worry about that.
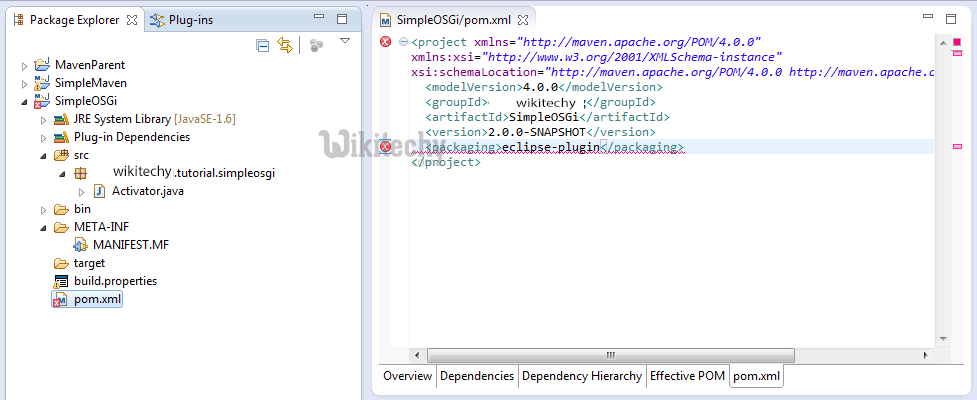
learn maven tutorial - configure maven tycho step3 - maven example
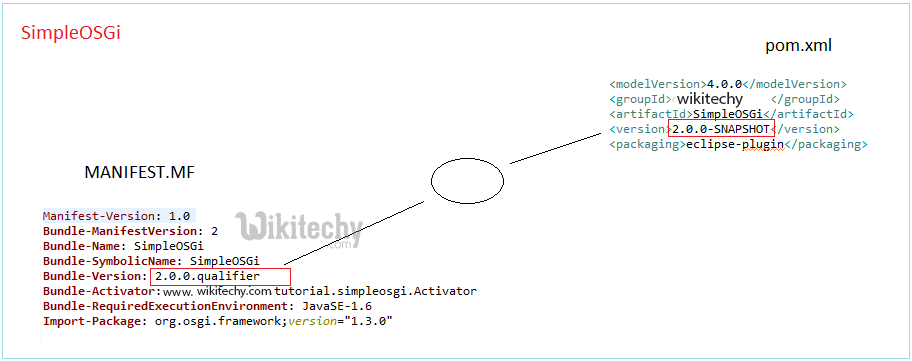
learn maven tutorial - configure maven tycho step4 - maven example
- Open the pom.xml file and reconfigure as follows:
maven tutorial tags : apache maven , maven repository , maven central
SimpleOSGi/pom.xml
sample code
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.wikitechy.com/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>wikitechy</groupId>
<artifactId>SimpleOSGi</artifactId>
<version>2.0.0-SNAPSHOT</version>
<packaging>eclipse-plugin</packaging>
<parent>
<groupId>org.o7planning</groupId>
<artifactId>MavenParent</artifactId>
<version>1.0.0</version>
<relativePath>../MavenParent/pom.xml</relativePath>
</parent>
</project>
5.2- Configuring Maven Project (SimpleMaven)
- Open pom.xml file on Project SimpleMaven and add the following code:
<parent>
<groupId>wikitechy</groupId>
<artifactId>MavenParent</artifactId>
<version>1.0.0</version>
<relativePath>../MavenParent/pom.xml</relativePath>
</parent>
SimpleMaven/pom.xml
maven tutorial tags : apache maven , maven repository , maven central
sample code
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.wikitechy.com/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>wikitechy</groupId>
<artifactId>SimpleMaven</artifactId>
<version>3.0.0-SNAPSHOT</version>
<dependencies>
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.3.2</version>
</dependency>
</dependencies>
<parent>
<groupId>wikitechy</groupId>
<artifactId>MavenParent</artifactId>
<version>1.0.0</version>
<relativePath>../MavenParent/pom.xml</relativePath>
</parent>
</project>
5.3- Configuring Maven Parent
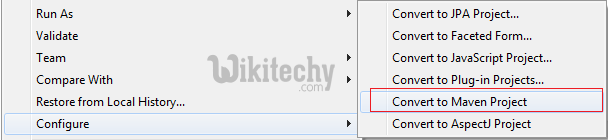
learn maven tutorial - configure maven parent step1 - maven example
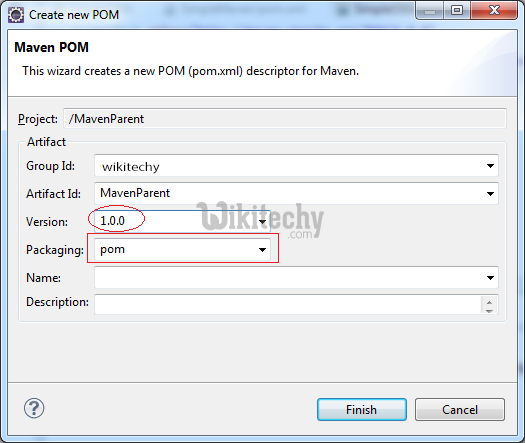
learn maven tutorial - configure maven parent step2 - maven example
MavenParent/pom.xml
maven tutorial tags : apache maven , maven repository , maven central
sample code
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.wikitechy.com/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>wikitechy</groupId>
<artifactId>MavenParent</artifactId>
<version>1.0.0</version>
<packaging>pom</packaging>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<!-- 0.22.0 : Target location type: Directory is not supported -->
<tycho-version>0.22.0</tycho-version>
</properties>
<repositories>
<!-- Select the P2 repository to be used when resolving dependencies -->
<repository>
<id>eclipse-luna</id>
<layout>p2</layout>
<url>http://download.eclipse.org/releases/luna</url>
</repository>
</repositories>
<pluginRepositories>
<pluginRepository>
<id>tycho-snapshots</id>
<url>https://oss.sonatype.org/content/groups/public/</url>
</pluginRepository>
</pluginRepositories>
<build>
<plugins>
<plugin>
<!-- enable tycho build extension -->
<groupId>org.eclipse.tycho</groupId>
<artifactId>tycho-maven-plugin</artifactId>
<version>${tycho-version}</version>
<extensions>true</extensions>
<configuration>
<dependency-resolution>
<optionalDependencies>ignore</optionalDependencies>
</dependency-resolution>
</configuration>
</plugin>
<plugin>
<groupId>org.eclipse.tycho</groupId>
<artifactId>tycho-versions-plugin</artifactId>
<version>${tycho-version}</version>
<configuration>
<dependency-resolution>
<optionalDependencies>ignore</optionalDependencies>
</dependency-resolution>
</configuration>
</plugin>
<!--
<plugin>
<groupId>org.eclipse.tycho</groupId>
<artifactId>target-platform-configuration</artifactId>
<version>${tycho-version}</version>
<configuration>
<target>
<artifact>
<groupId>org.o7planning</groupId>
<artifactId>TargetPlatform</artifactId>
<version>1.0.0</version>
</artifact>
</target>
</configuration>
</plugin>
-->
</plugins>
</build>
<modules>
<module>../SimpleMaven</module>
<module>../SimpleOSGi</module>
</modules>
</project>
5.4- Building
- Right-click MavenParent select Run As / Maven Install.
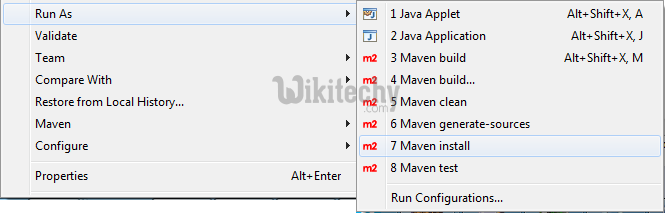
learn maven tutorial - maven building step1- maven example
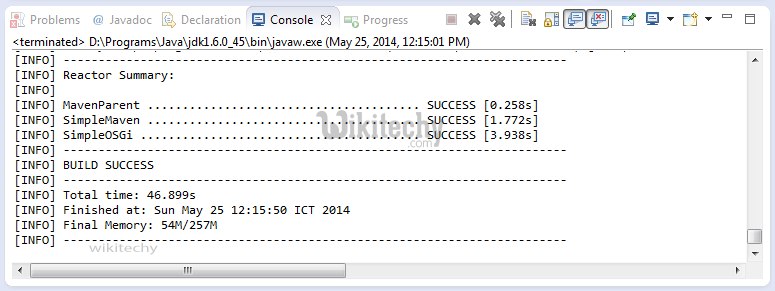
learn maven tutorial - maven building step2 - maven example