react - ReactJS State - react js - reactjs
What is State in Reactjs ?
- State is the place where the data comes from.
- You should try to create your state as simple as possible and minimize number of stateful components.
- For example, ten components that want data from the state, you should create one container component that will keep the state for all of them.
- Every component has a state object and a props object.
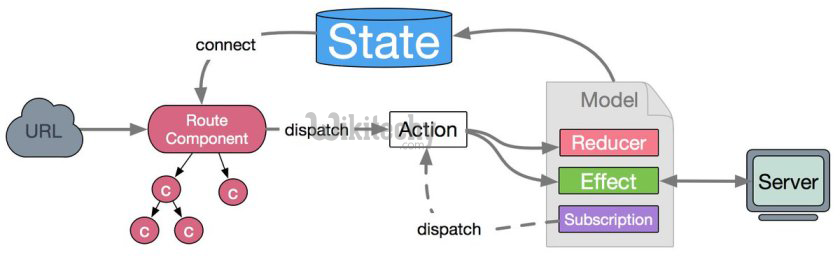
learn reactjs tutorial -
Reactjs State
- reactjs example - react tutorial - reactjs - react
- State is set using the setStatemethod.
- Calling setState triggers UI updates and is the bread and butter of React's interactivity.
- If we want to set an initial state before any interaction occurs we can use the getInitialState method.
reactjs states - States in react js:
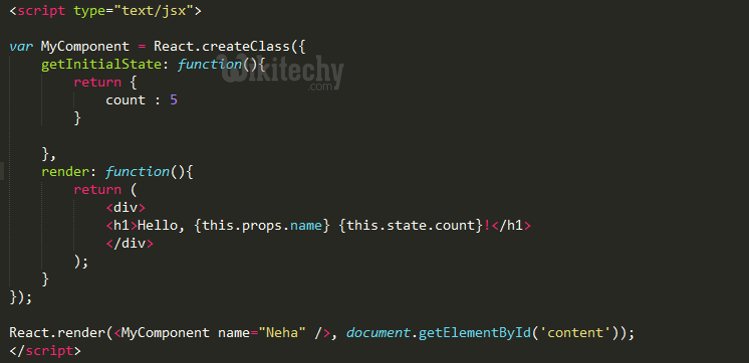
Article tag : react , react native , react js tutorial , create react app , react tutorial , learn react
Example
var MyComponent = React.createClass({
getInitialState: function(){
return {
count: 5
}
},
render: function(){
return (
<h1>{this.state.count}</h1>
)
}
});
click below button to copy the code. By reactjs tutorial team
Using Props
- Below code shows how to create stateful component using EcmaScript2016 syntax.
Article tag : react , react native , react js tutorial , create react app , react tutorial , learn react
App.jsx
import React from 'react';
class App extends React.Component {
constructor(props) {
super(props);
this.state = {
header: "Header from state...",
"content": "Content from state..."
}
}
render() {
return (
<div>
<h1>{this.state.header}</h1>
<h2>{this.state.content}</h2>
</div>
);
}
}
export default App;
click below button to copy the code. By reactjs tutorial team
main.js
import React from 'react';
import ReactDOM from 'react-dom';
import App from './App.jsx';
ReactDOM.render(<App />, document.getElementById('app'));
click below button to copy the code. By reactjs tutorial team
Output
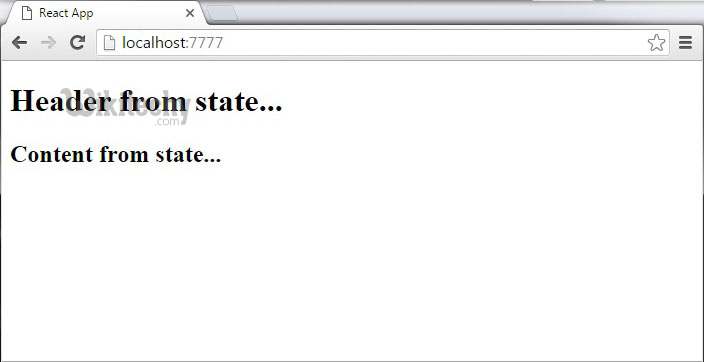