React JSX - React Tutorial for Beginners
What is JSX in ReactJS ?
- JSX denotes for JavaScript XML. It allows us to write HTML in React.
- It makes it easier to write and add HTML in React.
- JSX is a preprocessor step that adds XML syntax to JavaScript. You can definitely use React without JSX but JSX makes React a lot more elegant. Just like XML, JSX tags have a tag name, attributes, and children.
Coding JSX
- It allows us to write HTML elements in JavaScript and place them in the DOM without any appendChild() and/or createElement() methods.
- It converts HTML tags into react elements.
- To use JSX is not compulsory, but JSX makes it easier to write React applications.
- In this example, JSX allows us to write HTML directly within the JavaScript code. JSX is an extension of the JavaScript language and is translated into regular JavaScript at runtime.
Example 1
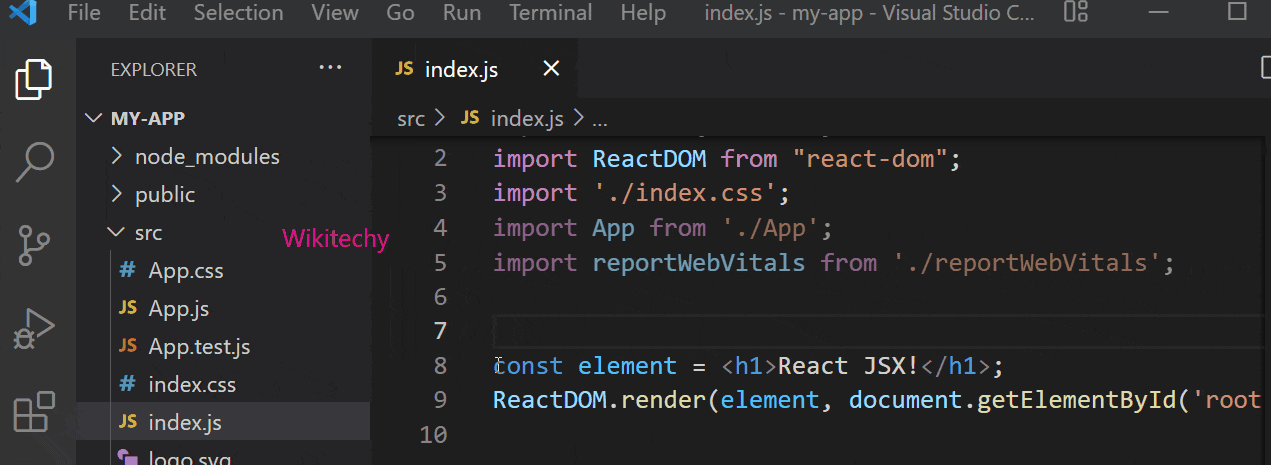
Sample Code - JSX
import React, {useState} from "react";
import ReactDOM from "react-dom";
import './index.css';
const element = <h1> React JSX!</h1>;
ReactDOM.render(element, document.getElementById('root'));
Output
React JSX!
Example 2
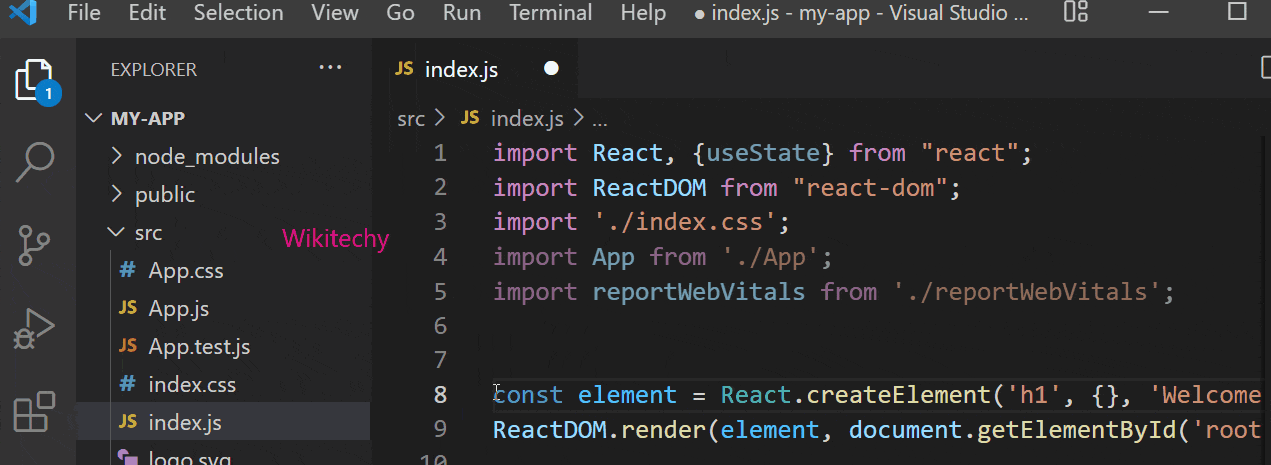
Sample Code - Without JSX
import React, {useState} from "react";
import ReactDOM from "react-dom";
import './index.css';
const element = React.createElement('h1', {}, 'Welcome to React JSX!');
ReactDOM.render(element, document.getElementById('root'));
Output
Welcome to React JSX!
Expressions in JSX
- In JSX you can use expressions inside curly braces { }.
- The expression can be a React property, or variable, or some other valid JavaScript expression. It will execute the expression and return the result.
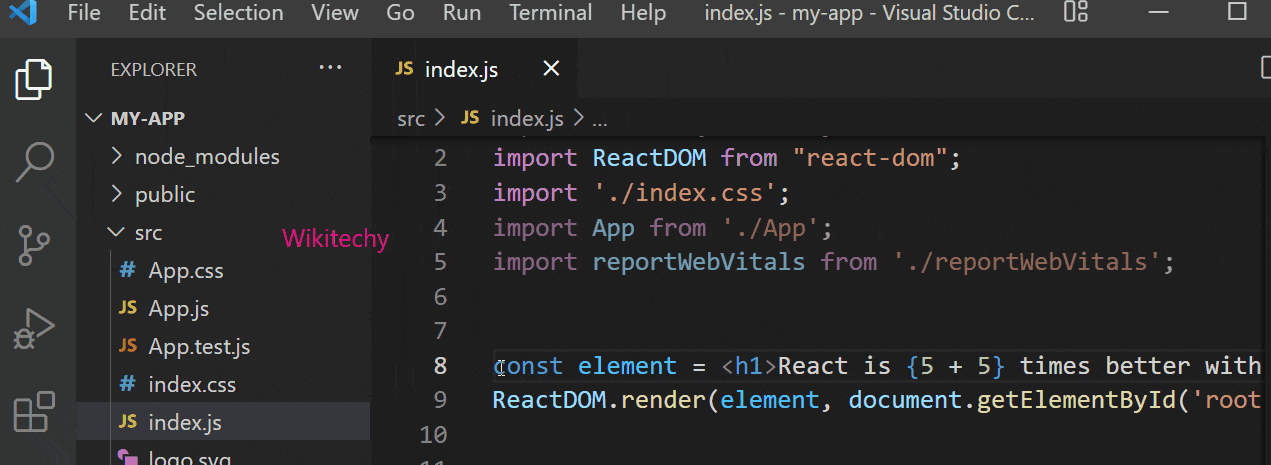
Sample Code
import React, {useState} from "react";
import ReactDOM from "react-dom";
import './index.css';
const element = <h1> React is {5 + 5} times better with React JSX</h1>;
ReactDOM.render(element, document.getElementById('root'));
Output
React is 10 times better with React JSX
Inserting a Large Block of HTML
- To write HTML on several lines, put the HTML inside parentheses. In this example we can create a list with three list items.
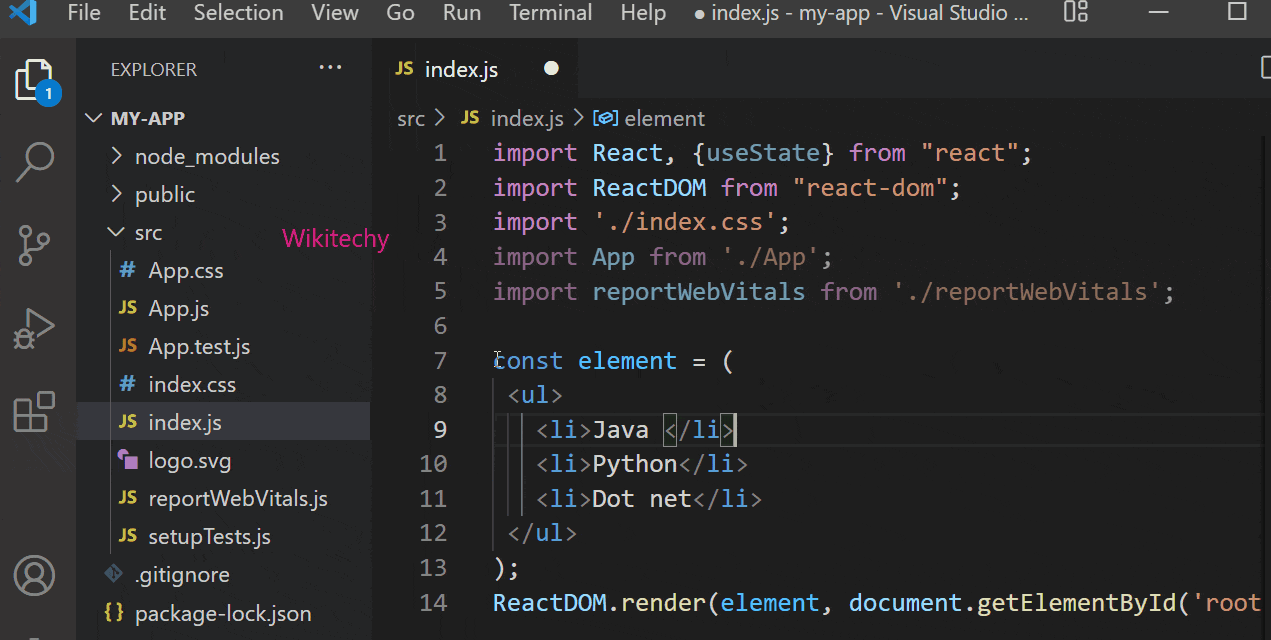
Sample Code
import React, {useState} from "react";
import ReactDOM from "react-dom";
import './index.css';
const element = (
<ul>
<li>Java </li>
<li>Python</li>
<li>Dot net</li>
</ul>
);
ReactDOM.render(element, document.getElementById('root'));
Output
One Top Level Element
- HTML code should be covered in ONE top level element. So if you want to write two paragraphs, you should put them inside a parent element, like a div element.
- In this example , Wrap two paragraphs inside one DIV element. JSX will throw an error if the HTML is not correct, or if the HTML errors a parent element.
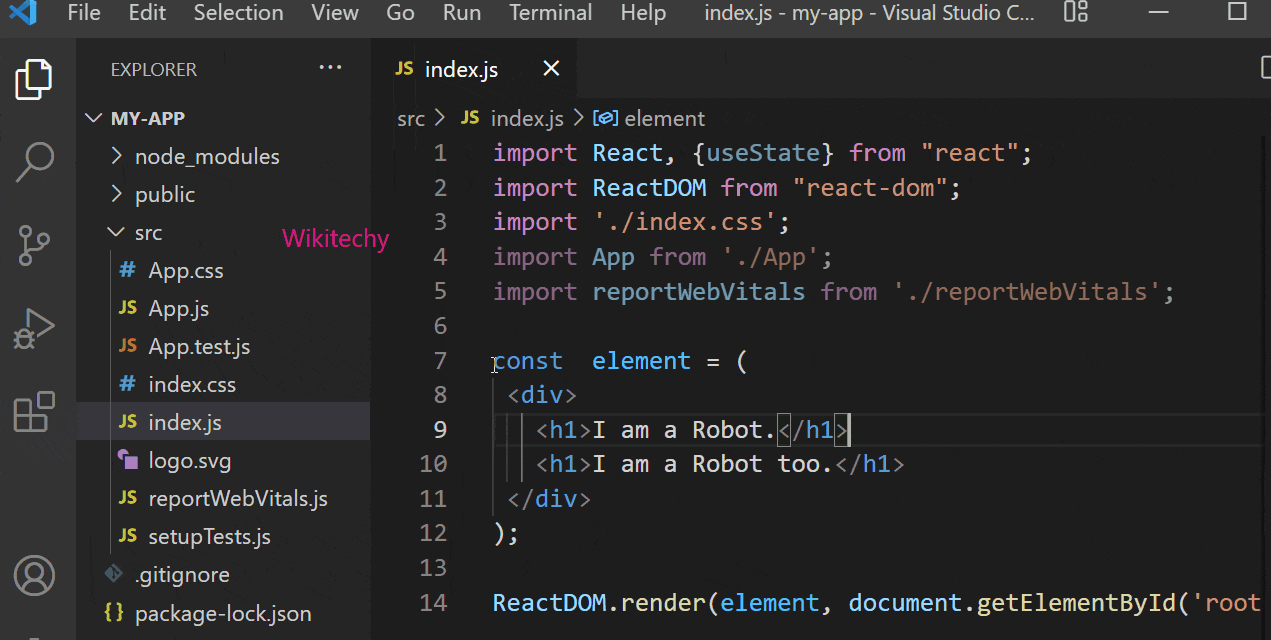
Sample Code
import React, {useState} from "react";
import ReactDOM from "react-dom";
import './index.css';
const element = (
<div>
<h1>I am a Robot.</h1>
<h1>I am a Robot too.</h1>
</div>
);
ReactDOM.render(element, document.getElementById('root'));
Output
- Alternatively, you can use a "fragment" to cover multiple lines. This will stop unreasonably adding extra nodes to the DOM.
- A fragment looks like an empty HTML tag: <></>. In this example, you can wrap two paragraphs inside a fragment.
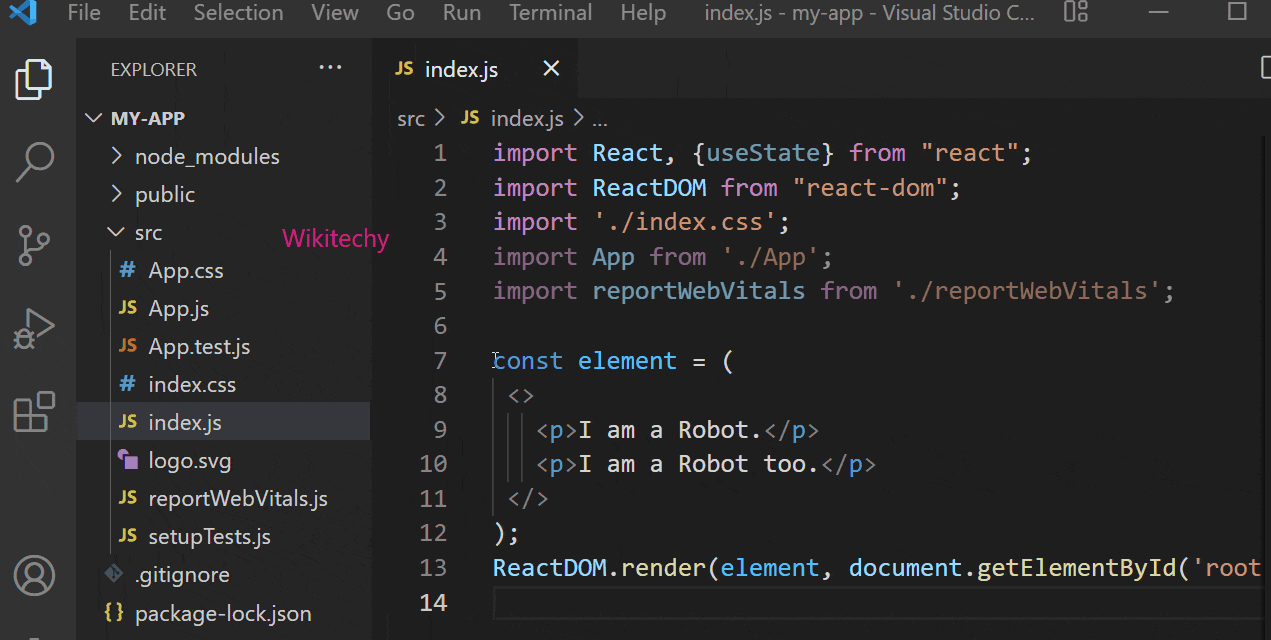
Sample Code
import React, {useState} from "react";
import ReactDOM from "react-dom";
import './index.css';
const element = (
<>
<p>I am a Robot.</p>
<p>I am a Robot too.</p>
</>
);
ReactDOM.render(element, document.getElementById('root'));
Output
Elements Must be Closed
- JSX follows XML rules, and then HTML elements must be correctly closed. In the below example, close empty elements with />. JSX will throw an error if the HTML is not properly closed.
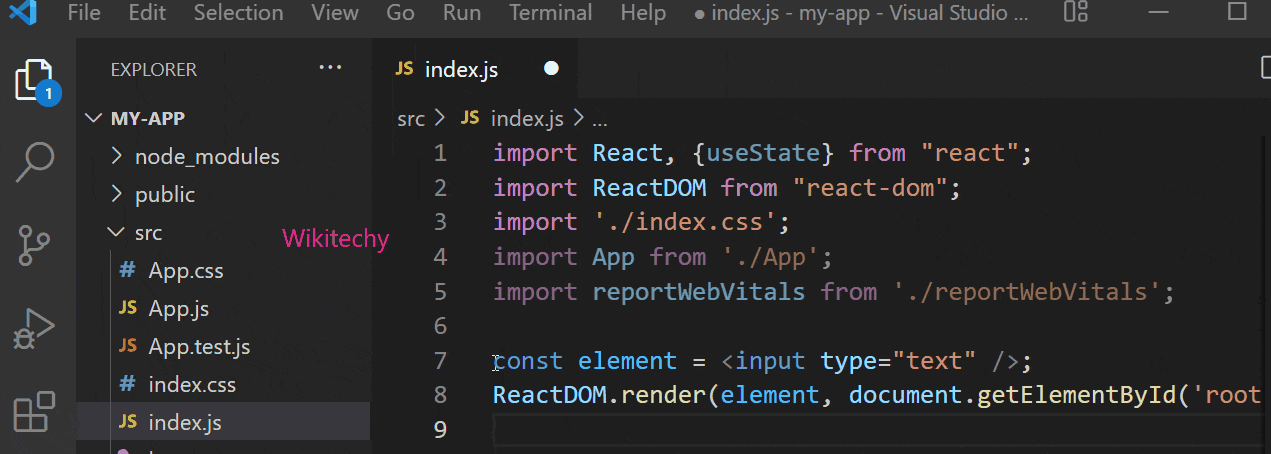
Sample Code
import React, {useState} from "react";
import ReactDOM from "react-dom";
import './index.css';
const element = <input type="text" />;
ReactDOM.render(element, document.getElementById('root'));
Output
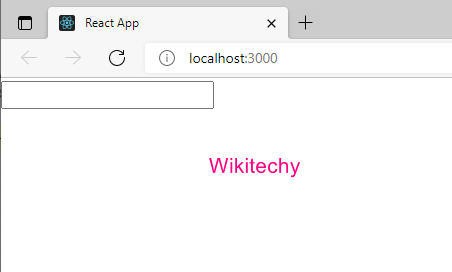
Attribute class = className
- The class attribute is a much-used attribute in HTML, but meanwhile JSX is extracted as JavaScript, and the class keyword is a reserved word in JavaScript, you are not permitted to use it in JSX.
- You can use attribute className instead.
- JSX resolved this by using className in its place. When JSX is rendered, it interprets className attributes into class attributes.
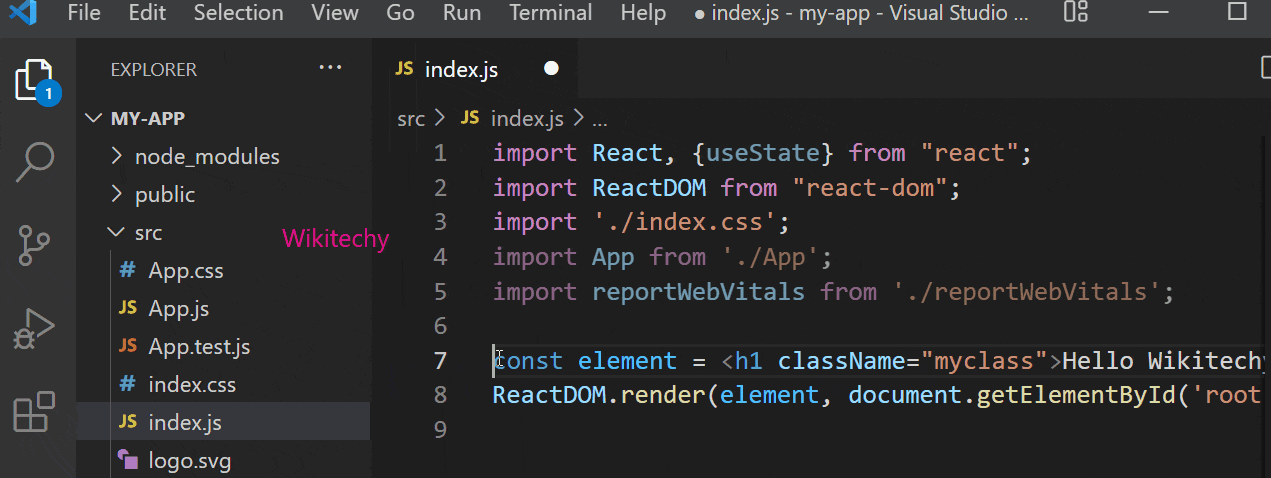
Sample Code
import React, {useState} from "react";
import ReactDOM from "react-dom";
import './index.css';
const element = <h1 className="myclass">Hello Wikitechy</h1>;
ReactDOM.render(element, document.getElementById('root'));
Output
Hello Wikitechy
Conditions - if statements
- React supports if statements, but not inside JSX.
- If you want to use conditional statements in JSX, you must put the if statements outside of the JSX, or you should use a ternary expression instead.
Example 1
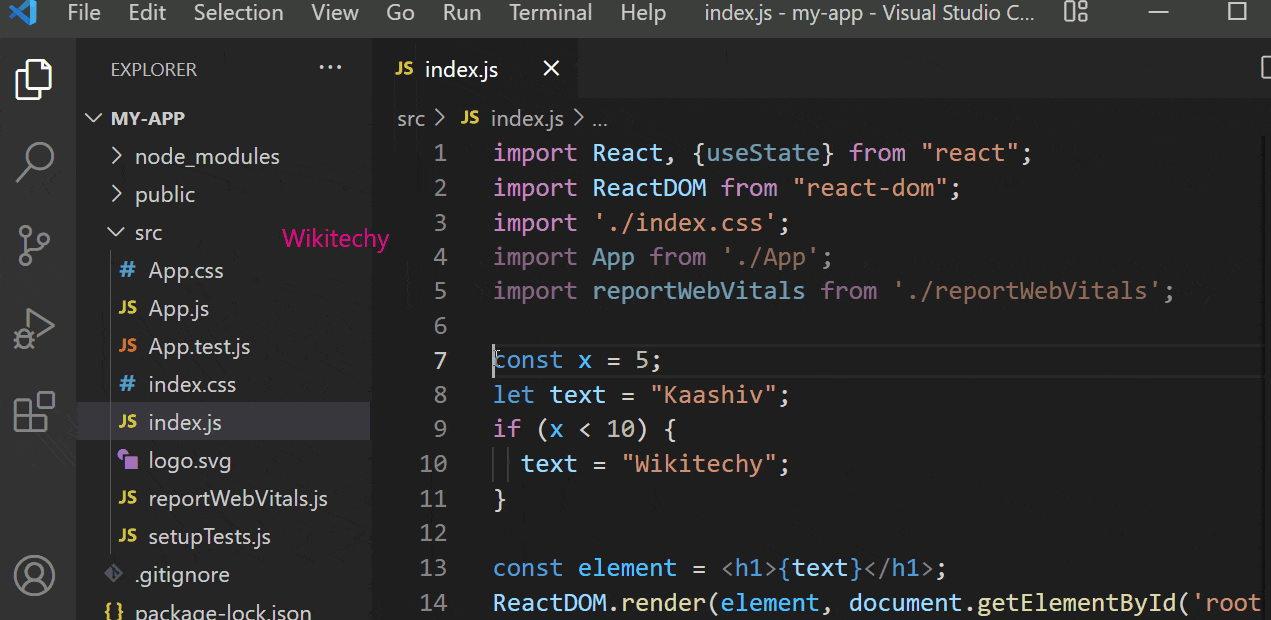
- Write if statements external of the JSX code.
Sample Code
import React, {useState} from "react";
import ReactDOM from "react-dom";
import './index.css';
const x = 5;
let text = "Kaashiv";
if (x < 10) {
text = "Wikitechy";
}
const element = <h1>{text}</h1>;
ReactDOM.render(element, document.getElementById('root'));
Output
Wikitechy
Example 2
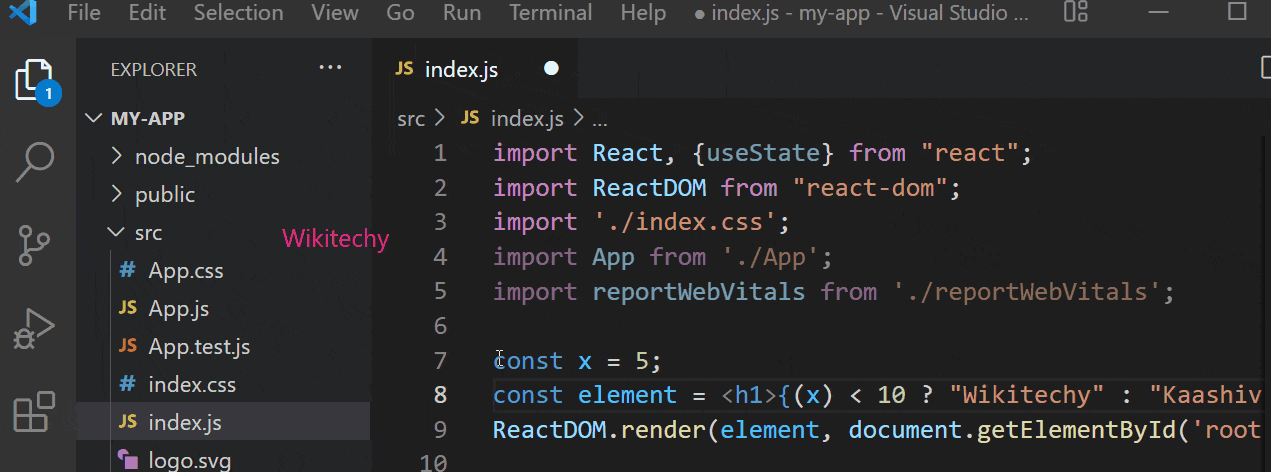
- Here you can use ternary expressions instead.
Sample Code
import React, {useState} from "react";
import ReactDOM from "react-dom";
import './index.css';
const x = 5;
const element = <h1>{(x) < 10 ? "Wikitechy" : "Kaashiv"}</h1>;
ReactDOM.render(element, document.getElementById('root'));
Output
Wikitechy