Components in ReactJS - ReactJS Components - React Tutorial for Beginners
Components in ReactJS
- Components are like functions that return HTML elements.
- Components are independent and reusable bits of code. They help the same purpose as JS functions, but work in isolation and return HTML.
- Components are two types:
- Class components
- Function components
Create Your First Component
- When creating a React component, the component's name should start with an upper case letter.
Class Component
- A class component must contain the extends React.Component statement.
- This statement creates an inheritance to React.Component, and gives your component access to React.Component's functions.
- The component also needs a render() method, this method returns HTML.
Example
class Props extends React.Component { render() { return <h2>Welcome to Wikitechy</h2>; } }
Function Component
- Here is the same example, but created using a Function component instead.
- A Function component also returns HTML, and acts as a Class component, but Function components can be written using less code, are easier to un-derstand.
Example
function React() { return <h2>Welcome to Wikitechy!</h2>>; }
Rendering a Component
- Now your React application has a component called React, which returns an <h2> element.
- To use this component in your application, use similar syntax as normal HTML: <Car />
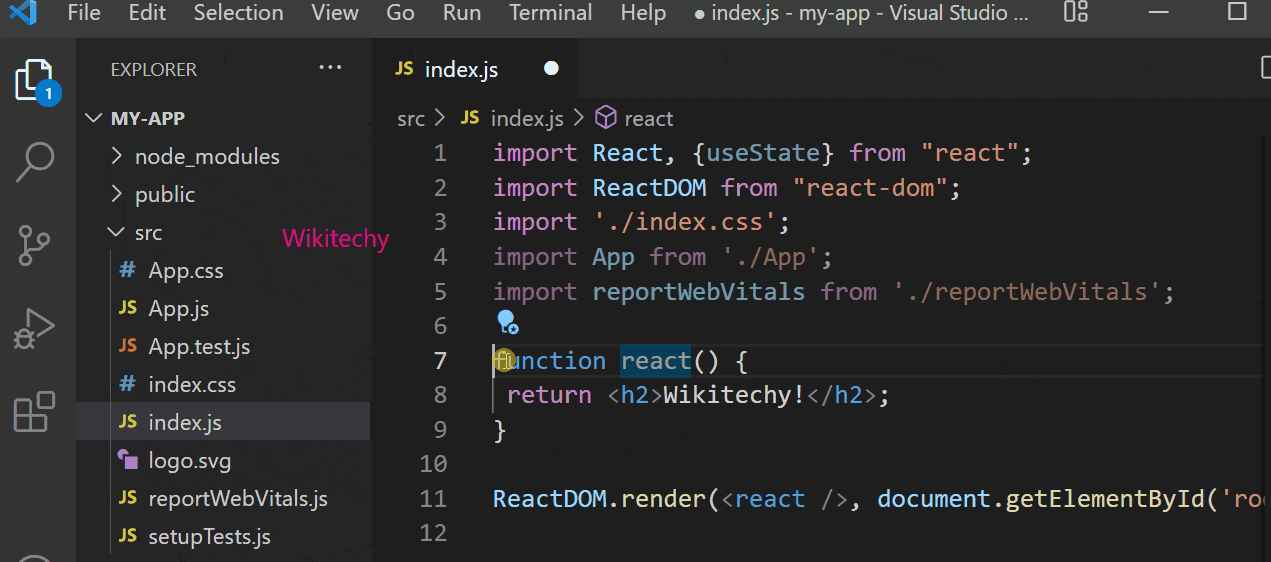
Sample code
import React, {useState} from "react";
import ReactDOM from "react-dom";
import './index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
function react() {
return <h2>Wikitechy!</h2>;
}
ReactDOM.render(<react />, document.getElementById('root'));
Output
Wikitechy!
Props
- Components can be passed as props, which stands for properties.
- Props are like function arguments, and you send them into the component as attributes.
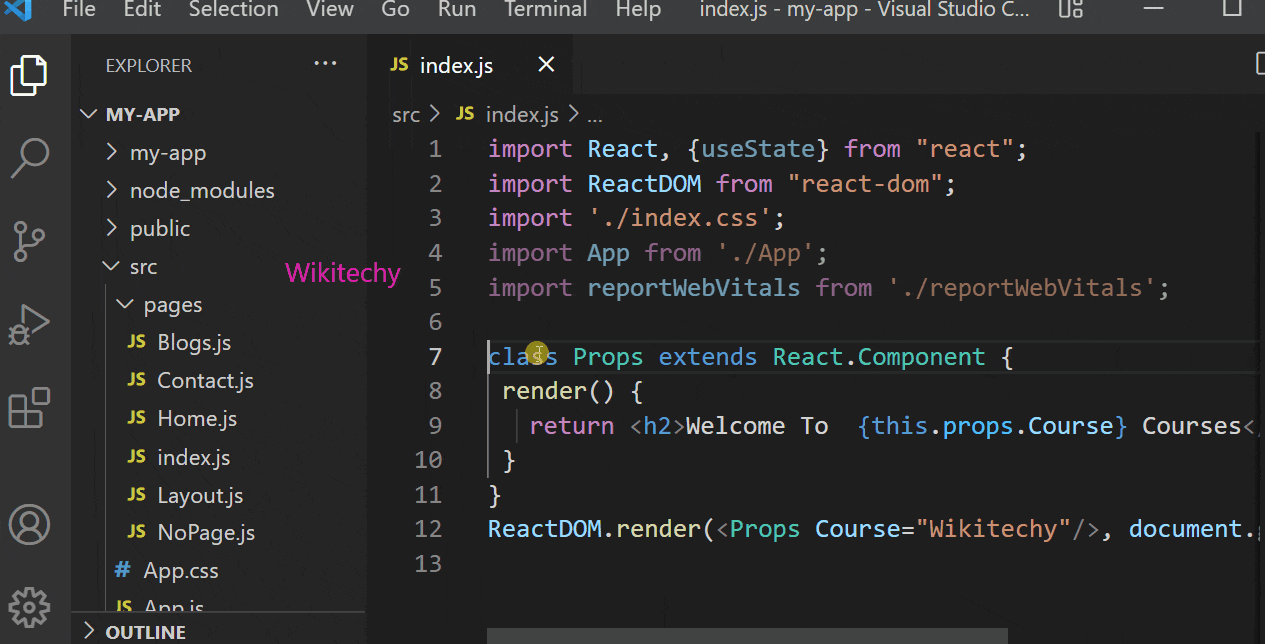
Sample code
import React, {useState} from "react";
import ReactDOM from "react-dom";
import './index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
class Props extends React.Component {
render() {
return <h2>Welcome To {this.props.Course} Courses</h2>;
}
}
ReactDOM.render(<Props Course="Wikitechy"/>, document.getElementById('root'));
Output
Welcome To Wikitechy Courses
Components in Components
- We can refer to components inside other components.
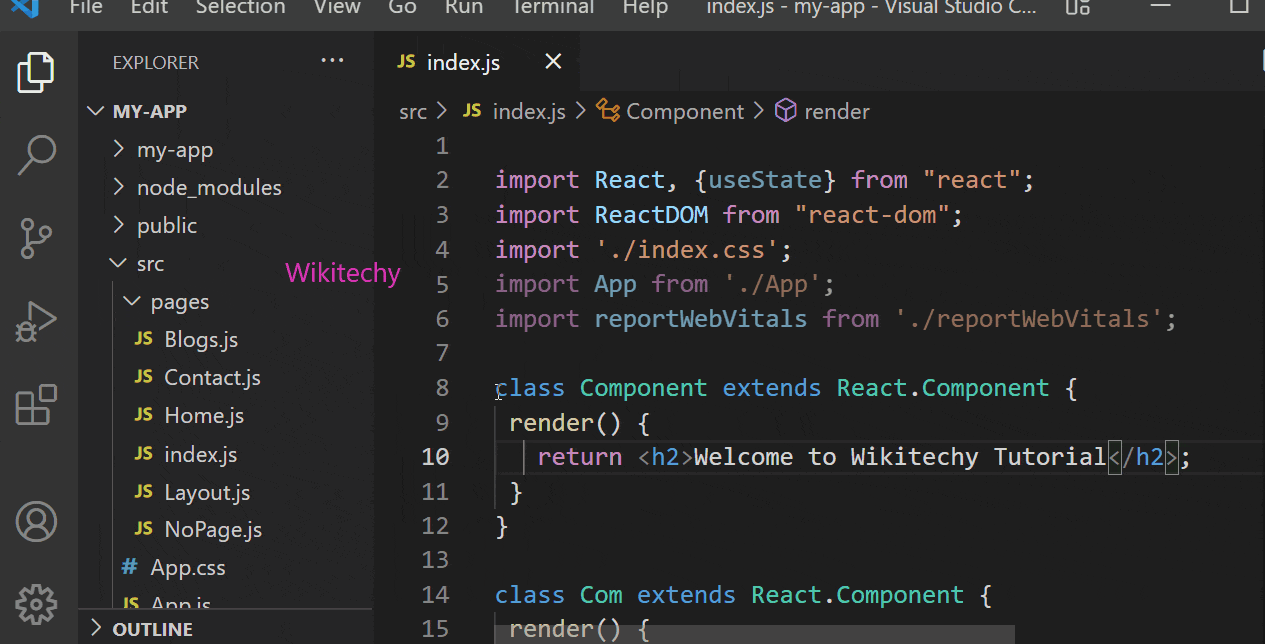
Sample code
import React, {useState} from "react";
import ReactDOM from "react-dom";
import './index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
class Component extends React.Component {
render() {
return <h2>Welcome to Wikitechy Tutorial!</h2>;
}
}
class Com extends React.Component {
render() {
return (
<div>
<h1>Welcome to Wikitechy</h1>
<Component/>
</div>
);
}
}
ReactDOM.render(<Com />, document.getElementById('root'));
Output
Welcome to Wikitechy Welcome to Wikitechy Tutorial
Components in Files
- React is all about re-using code, and it is suggested to split your compo-nents into separate files.
- Here create a new file with a .js file extension and put the code inside it.
- Note that the filename must start with an uppercase character.
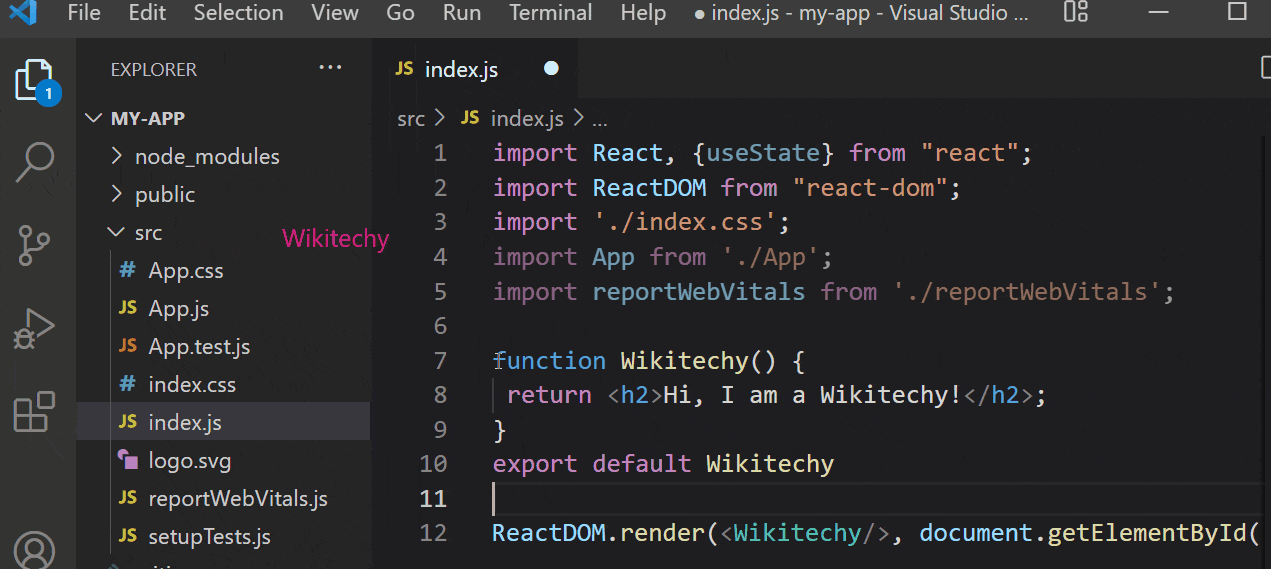
Sample code
import React, {useState} from "react";
import ReactDOM from "react-dom";
import './index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
function Wikitechy() {
return <h2>Hi, I am a Wikitechy!</h2>;
}
export default Wikitechy
ReactDOM.render(<Wikitechy/>, document.getElementById('root'));
Output
Hi, I am a Wikitechy!