C - Structure - Struct
Structure and Linked List with C Programming in Tamil
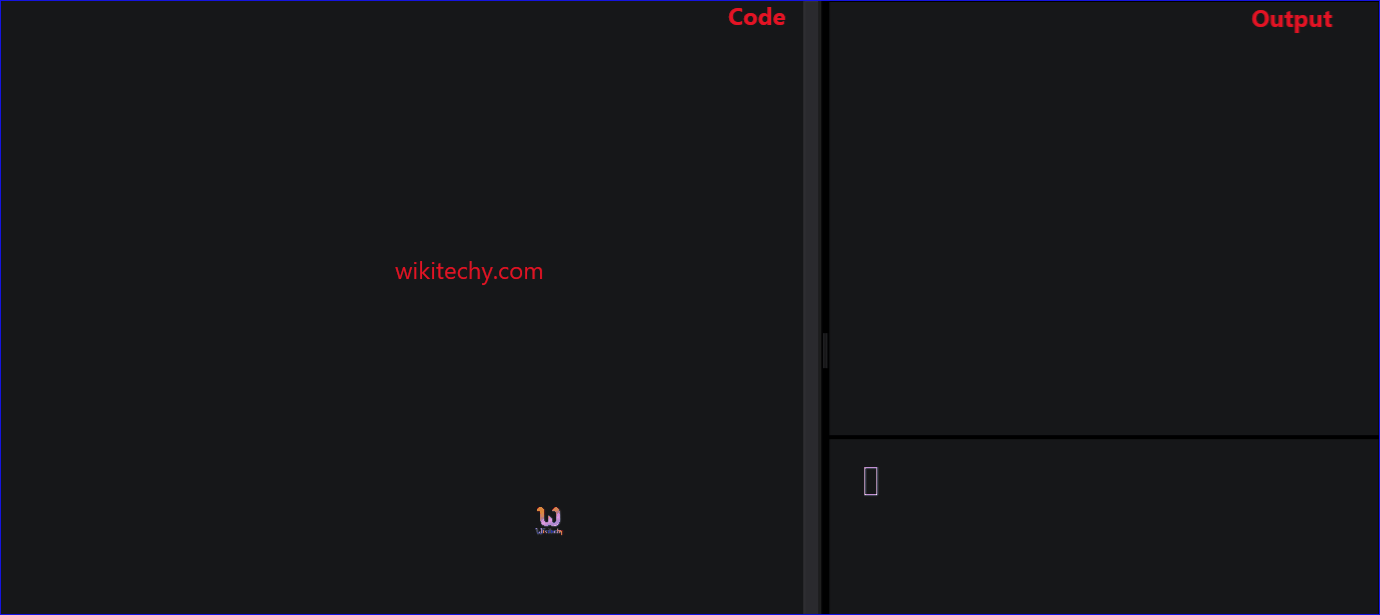
Learn C - C tutorial - struct - C examples - C programs
C Structure - struct - Definition and Usage
- In C- Programming the Structure is defined as a collection of different data types which are grouped together and each element in a C structure is called member.
- In C-Programming Structure is similar to array but we can declare different data type in a single structure
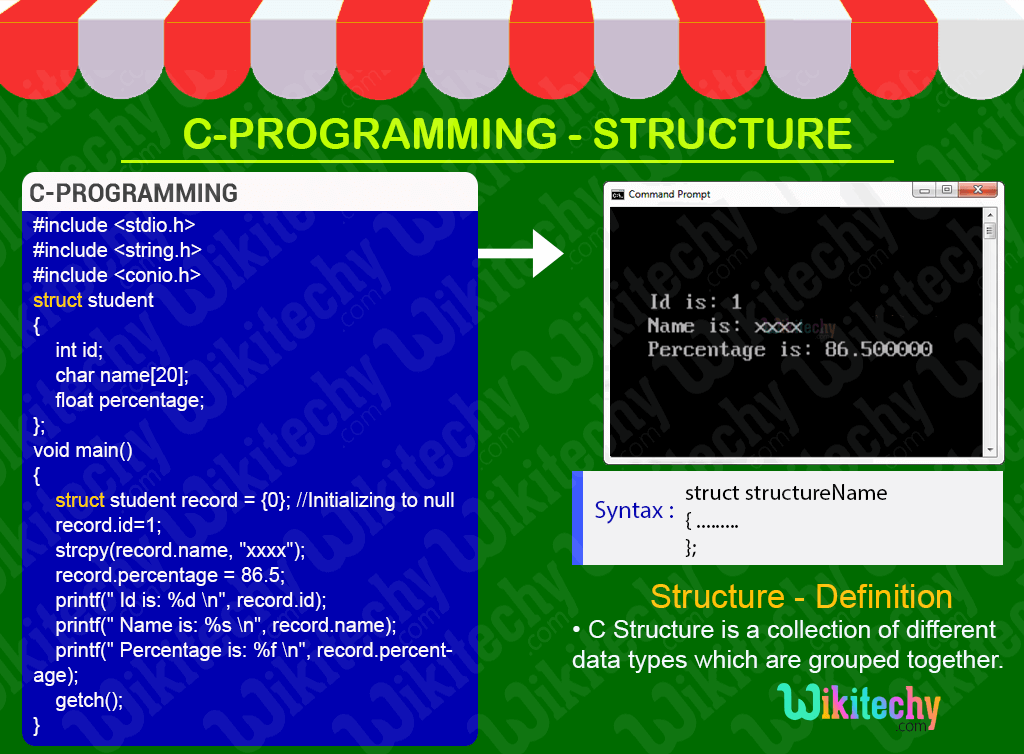
C Syntax
struct structureName
{
//member definitions
};
Example
Let's see the example to define structure for employee in c.
struct employee
{
int id;
char name[50];
float salary;
};
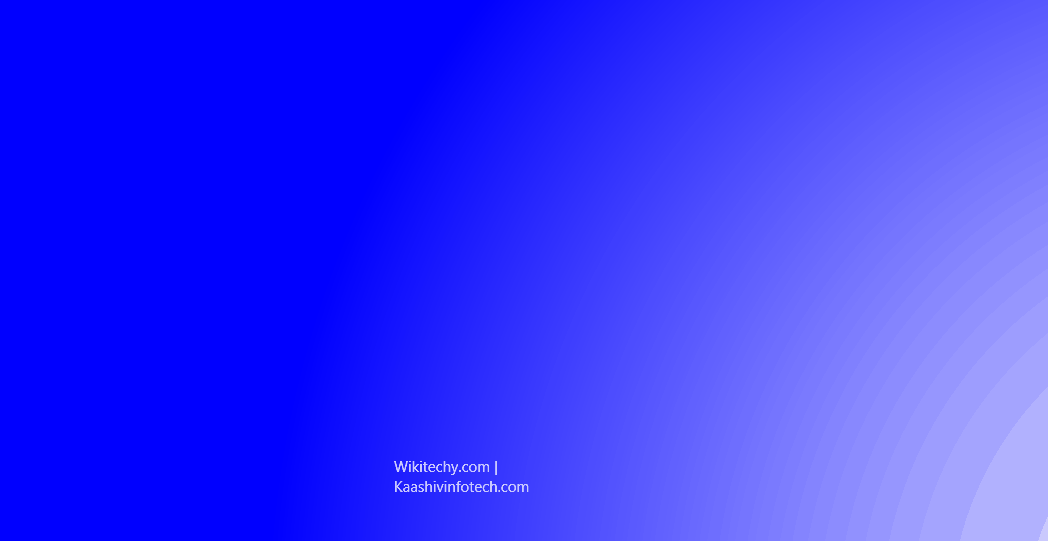
- Here,
- struct is the keyword,
- employee is the tag name of structure,
- id, name and salary are the members or fields of the structure.
Declaring Structure Variable
- We can declare variable for the structure, so that we can access the member of structure easily.
- There are two ways to declare structure variable:
- By struct keyword within main() function
- By declaring variable at the time of defining structure.
Accessing Members of a Structure
- There are two ways to access structure members:
- By . (member or dot operator)
- By -> (structure pointer operator)
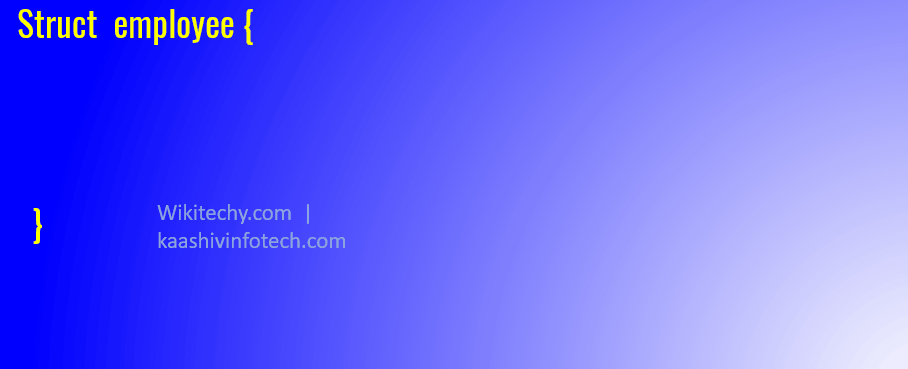
Example 1:
Sample - C Code
#include <stdio.h>
#include <string.h>
#include <conio.h>
void main()
struct student
{
int id;
char name[20];
float percentage;
};
void main()
{
struct student record = {0}; //Initializing to null
record.id=1;
strcpy(record.name, "xxxx");
record.percentage = 86.5;
printf(" Id is: %d \n", record.id);
printf(" Name is: %s \n", record.name);
printf(" Percentage is: %f \n", record.percentage);
getch();
}
C Code - Explanation
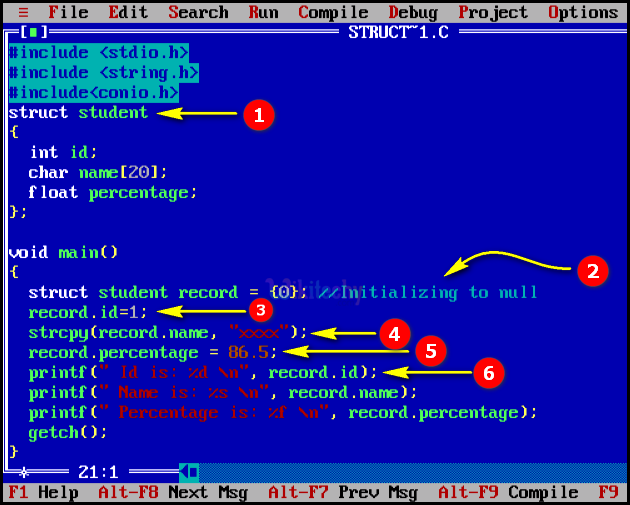
- In this statement we create the structure with the structure name as “student” .
- Here in this statement we initialize the structure with its element value as zero.
- In this statement we assign the id value as “id=1” .
- In this statement we assign the name value as “xxxx” .
- In this statement we assign the percentage value as “86.5” .
- Here in this statement we print the value of the record id using structure name with its elements. In this program we define three printf statement which will print the record id, name & percentage value.
Sample Output - Programming Examples
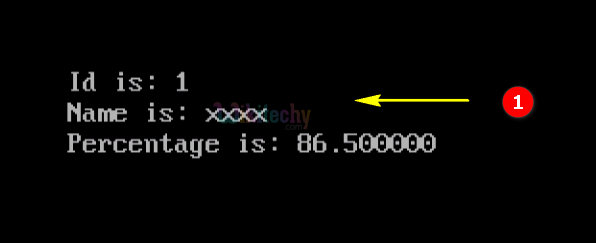
- Here in this output the structure variable id, name and percentage values (1, XXXX and 86.500000) are printed using the printf statement as shown here in the console window.
Example 2:
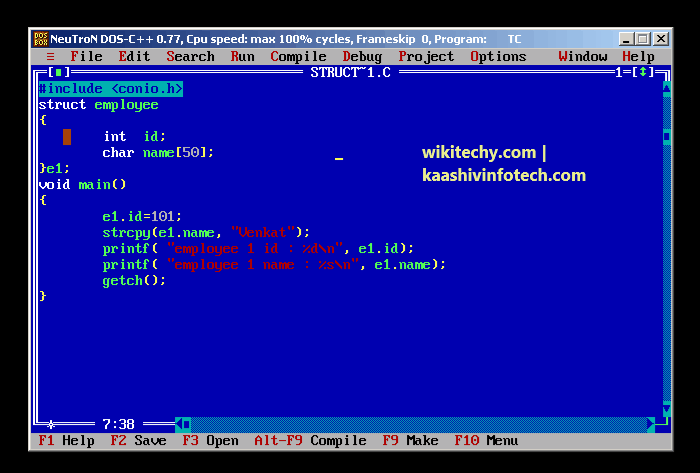
Sample Code
#include<stdio.h>
#include<string.h>
#include<conio.h>
struct employee
{
int id;
char name[50];
} e1;
void main()
{
e1.id=101;
strcpy(e1.name, "Venkat");
printf( "employee 1 id : %d\n", e1.id);
printf( "employee 1 name : %s\n", e1.name);
getch();
}
Output:
employee 1 id : 101
employee 1 name : Venkat
-
Nested structure
- A structure can be nested inside another structure. In other words, the members of a structure can be of any other type including structure.
- There are two ways to define nested structure in c language:
- By separate structure
- By Embedded structure
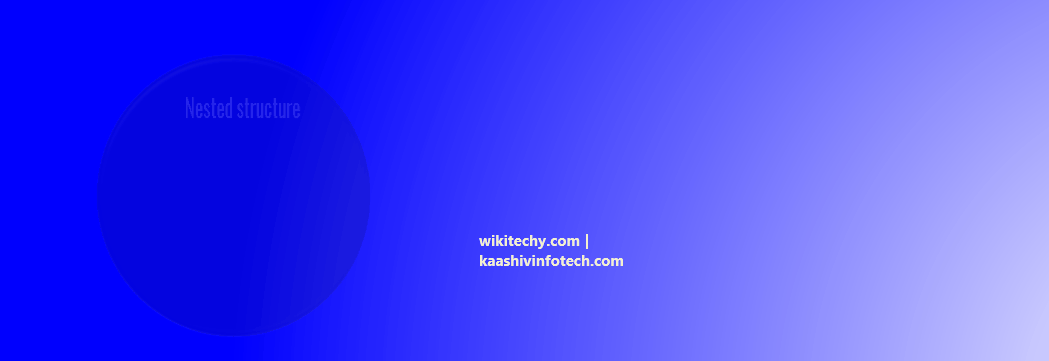
Separate Structures
- We can create 2 structures, but dependent structure should be used inside the main structure as a member.
Example
struct Date
{
int dd; int mm; int yyyy;
};
struct Employee
{
int id; char name[20]; struct Date doj;
}emp1;
Embedded Structures
- We can define structure within the structure also. It requires less code than previous way. But it can't be used in many structures.
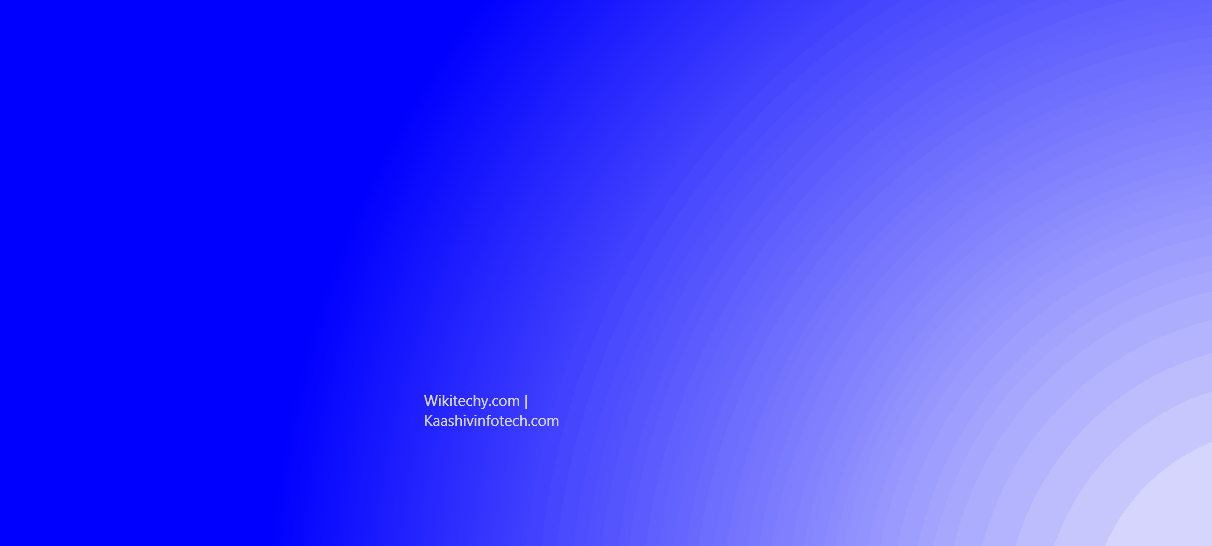
struct Employee
{
int id;
char name[20];
struct Date
{
int dd;
int mm;
int yyyy;
} doj;
} emp1;
- We can access the member of nested structure by
Outer_Structure.Nested_Structure.member
: - e1.doj.dd
- e1.doj.mm
- e1.doj.yyyy
Example
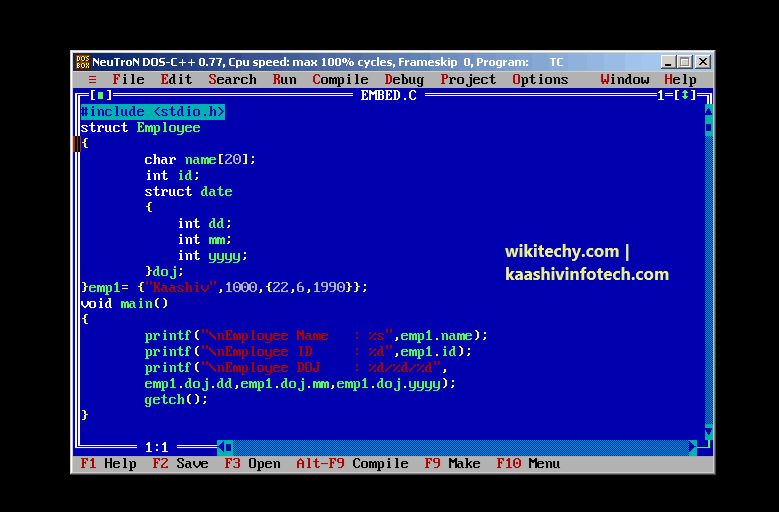
Sample Code
#include <stdio.h>
struct Employee
{
char name[20]; int id;
struct date
{
int dd;
int mm;
int yyyy;
} doj;
} emp1= {“Kaashiv",1000,{22,6,1990}};
void main()
{
printf("\nEmployee Name : %s",emp1.name);
printf("\nEmployee ID : %d",emp1.id);
printf("\nEmployee DOJ : %d/%d/%d",emp1.doj.dd,emp1.doj.mm,emp1.doj.yyyy);
getch();
}
Output
Employee Name : Kaashiv
Employee ID : 1000
Employee DOB : 22/6/1990
Array of Structures
- There can be array of structures in C programming to store many information of different data types.
- The array of structures is also known as collection of structures.
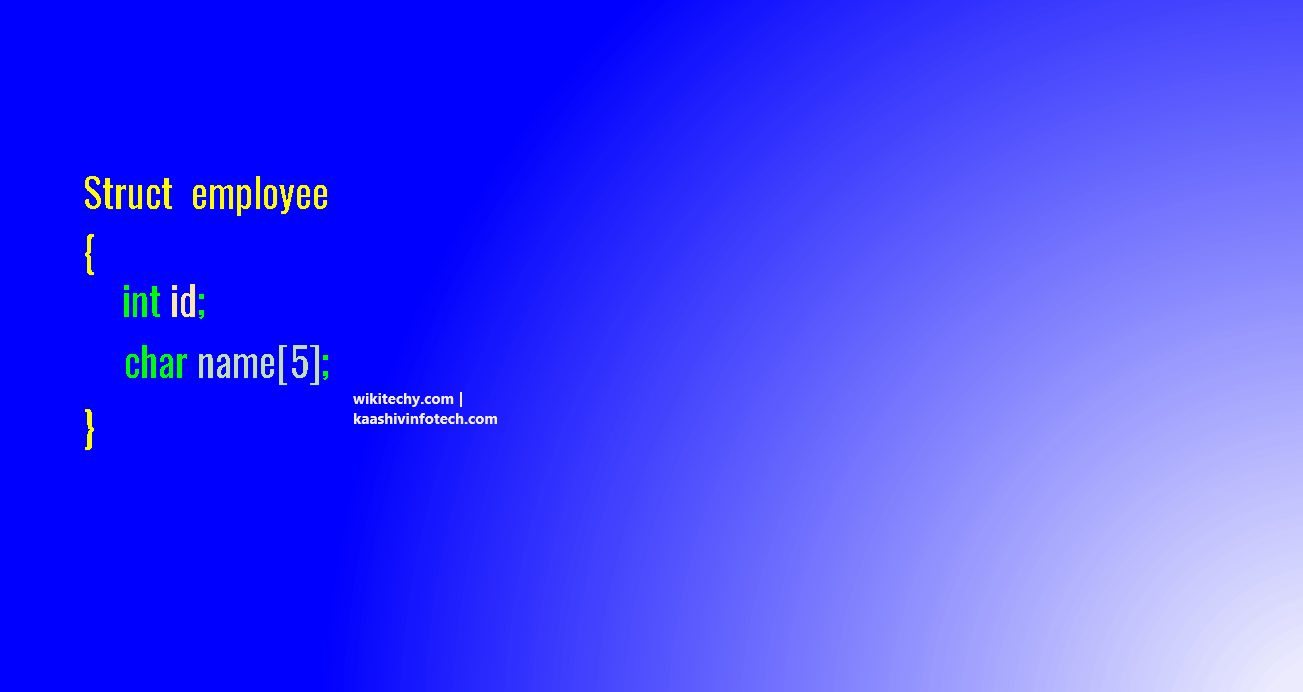
Sample Code
#include<stdio.h>
#include <string.h>
struct student
{
int rollno;
char name[10];
};
void main()
{
int i;
struct student st[3];
printf("Enter Records of 3 students");
for(i=0;i<3;i++)
{
printf("\nEnterRollno:");
scanf("%d",&st[i].rollno);
printf("\nEnter Name:");
scanf("%s",&st[i].name);
}
printf("\nStudent Information List:");
for(i=0;i<3;i++)
{
printf("\nRollno:%d,Name:%s",st[i].rollno,st[i].name);
}
getch();
}
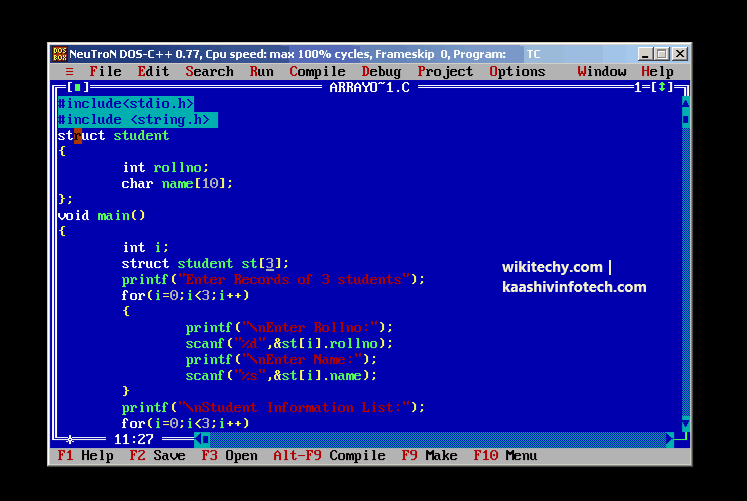
Output
Enter Records of 3 students
Enter Rollno:1
Enter Name:venkat
Enter Rollno:2
Entern Name:arun
Enter Rollno:3
Enter Name:ranjith
Student Information List:
Rollno:1, Name:venkat
Rollno:2, Name:arun
Rollno:3, Name:ranjith