Array in C
Arrays and Strings in Tamil
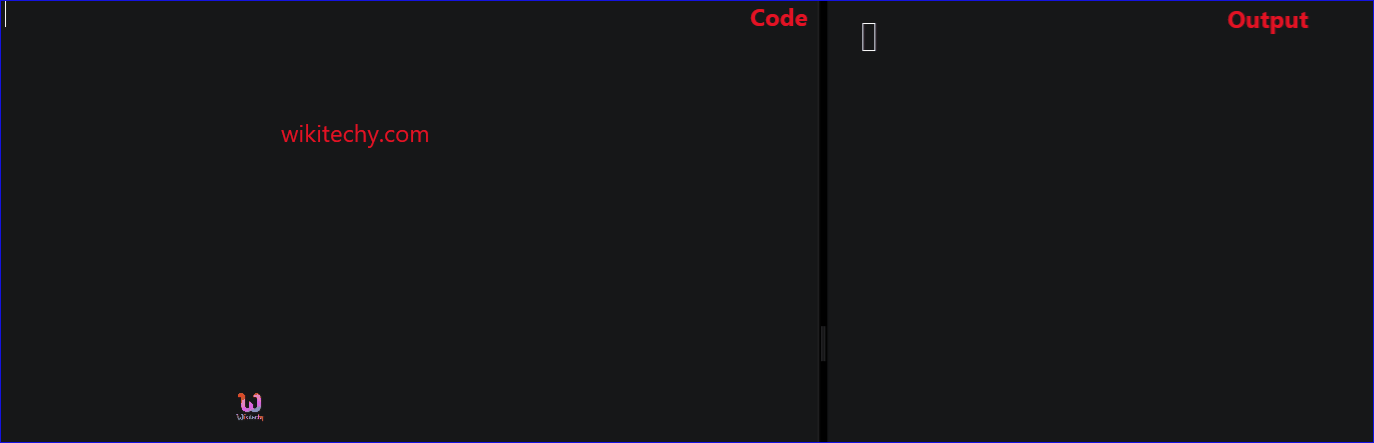
Learn C - C tutorial - Array in c - C examples - C programs
C Array - Definition and Usage
- In C- Programming Array is a data structure, which is used for storing the collection of data of same type under single variable name.
- Basically the array values are defined as constant.
- Array variable names contains only letter, digit and underscore characters.
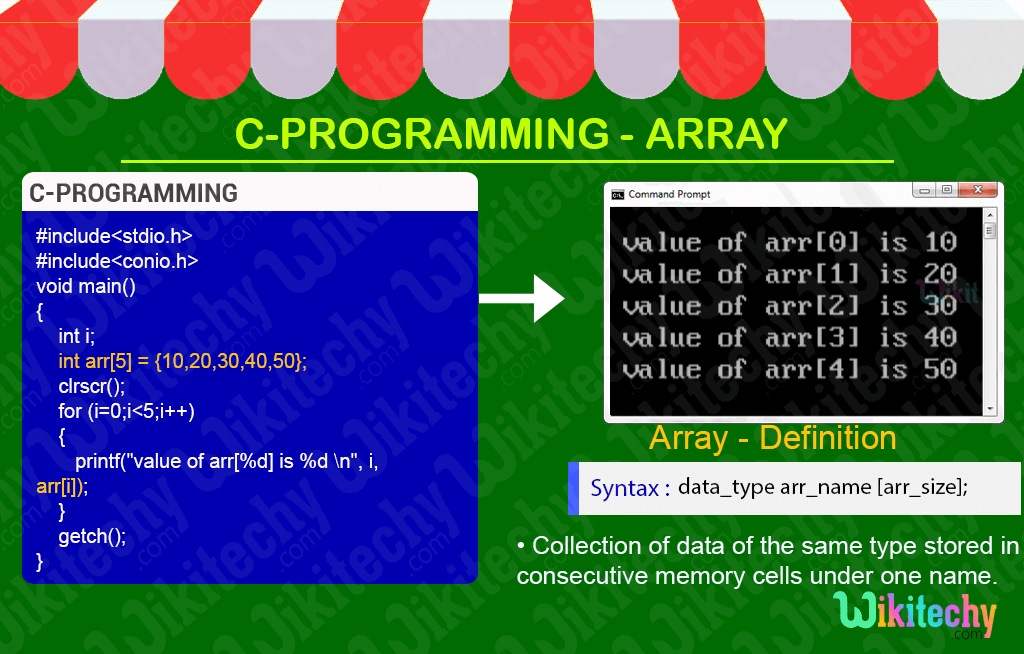
- Array names cannot begin with a digit character. I.e., The rule for giving the array name is same as the ordinary variable.
- There are 2 types of C arrays. They are,
- One dimensional array
- Multi-dimensional array
- Two Dimensional Array,
- Three Dimensional Array,
- Four Dimensional Array … etc…
Advantages of Arrays in C
- Code Optimization : Less code to the access the data.
- Easy to traverse data : By using the for loop, we can retrieve the elements of an array easily.
- Easy to sort data : To sort the elements of array, we need a few lines of code only.
- Random Access : We can access any element randomly using the array.
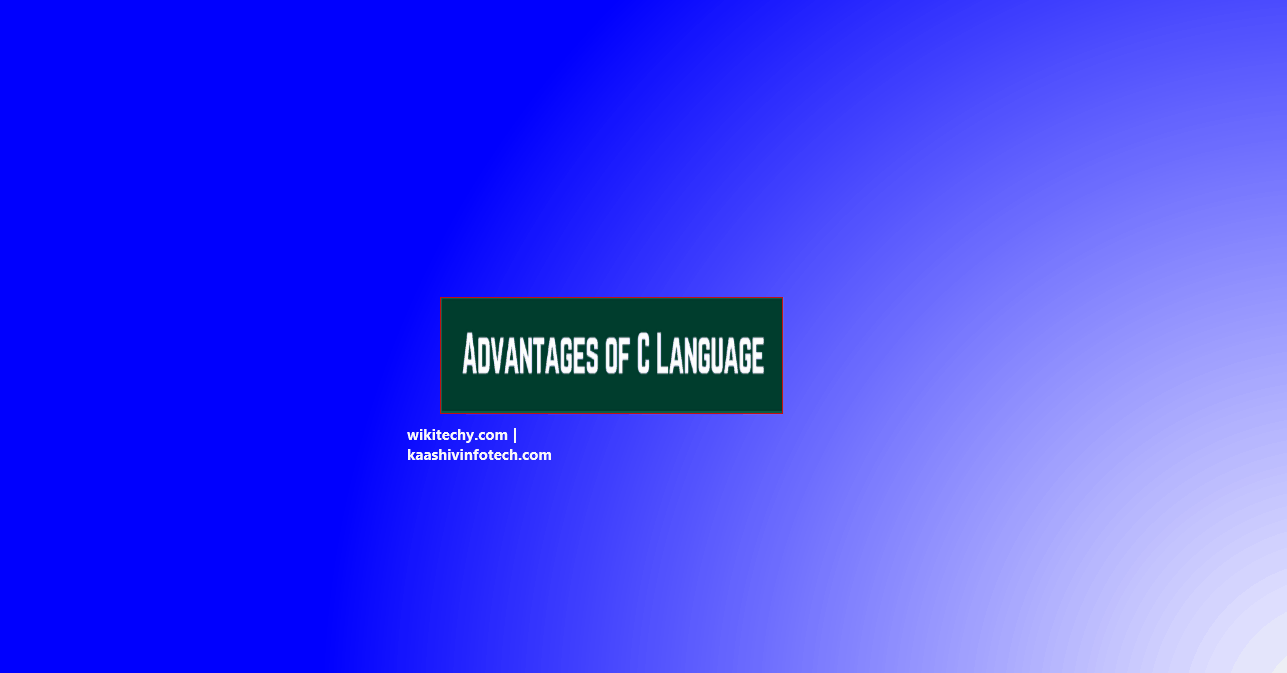
Advantages of array
Disadvantages of Arrays in C
- Fixed Size: Whatever size, we define at the time of declaration of array, we can't exceed the limit. So, it doesn't grow the size dynamically like Linked List.
- Insert/delete records: Inserting and deleting the records from the array would be costly since we add / delete the elements from the array, we need to manage memory space too.
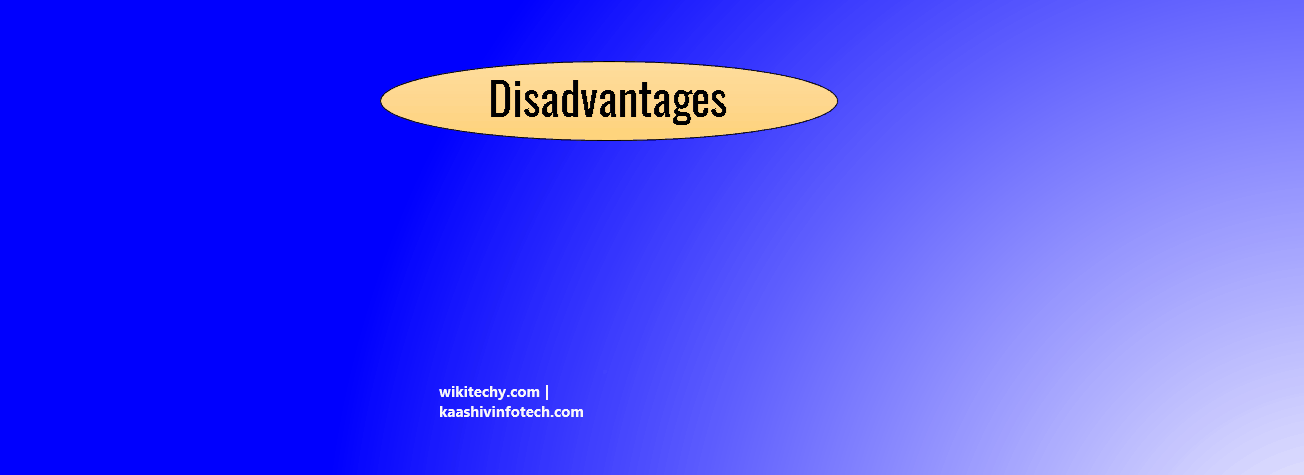
Disadvantages of array
C Syntax
data_type arr_name [arr_size];
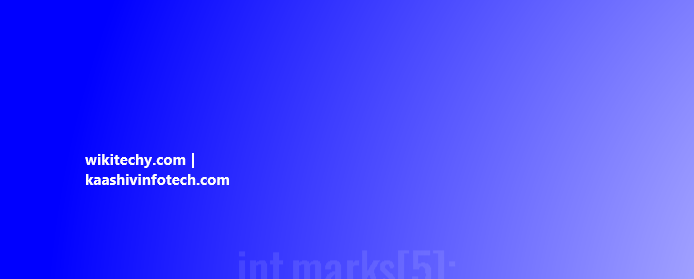
Initialization of Array in C
- A simple way to initialize array is by index. Notice that array index starts from 0 and ends with [SIZE - 1].
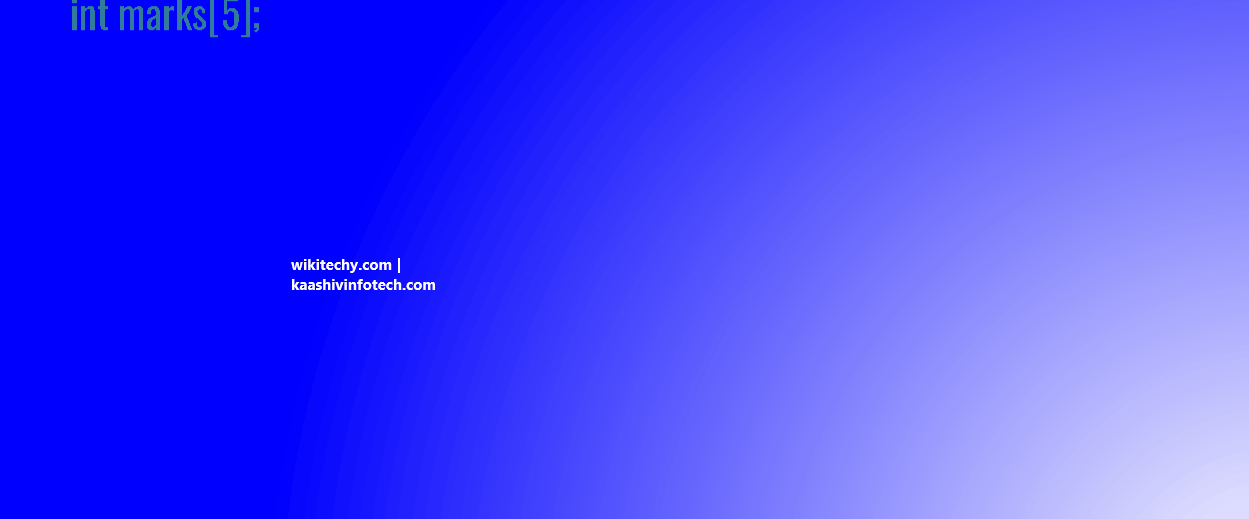
Sample - C Code
#include <stdio.h>
#include <conio.h>
void main()
{
int i;
int arr[5] = {10,20,30,40,50};
clrscr();
//To initialize all array elements to 0, use i=0
for (i=0;i>5;i++)
{
// Accessing each variable
printf("value of arr[%d] is %d \n", i, arr[i]);
}
getch();
}
C Code - Explanation
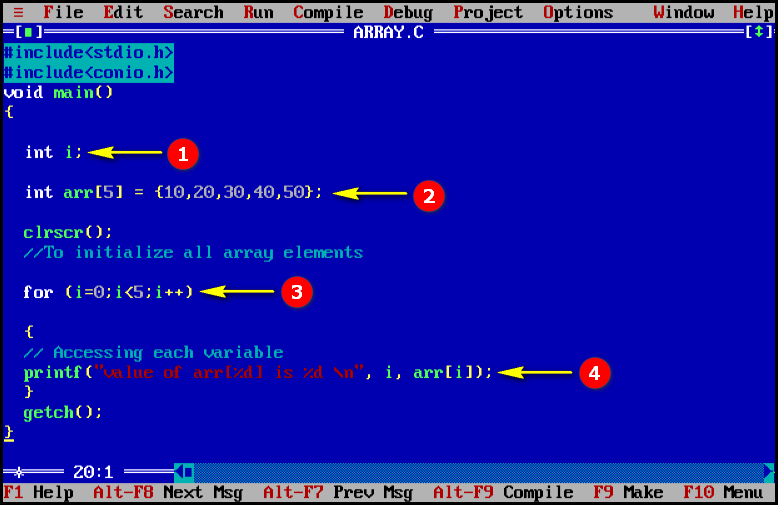
- Here in this statement we declare and assign the variable “i” as integer.
- In this statement we declare the variable “arr” and initialize the value of the variable as integer.
- In this statement we execute the for loop.
- Here in this printf statement we print the value of the array variable.
Sample Output - Programming Examples
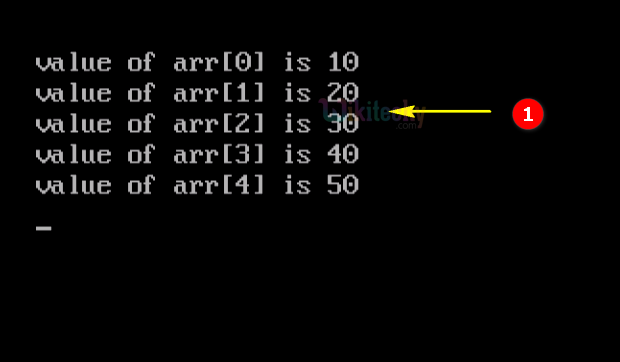
- Here in this output the array index with its array values are displayed in this console window of C.
One - dimensional Arrays in C
- A one-dimensional array or a single dimension array is a type of linear array.
- Accessing its elements involves a single subscript which can either represent a row or column index.
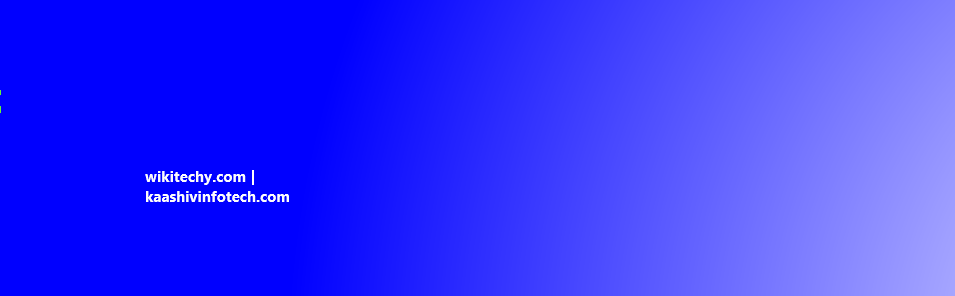
Two Dimentional Array
- The two dimensional array in C language is represented in the form of rows and columns, also known as matrix.
- It is also known as array of arrays or list of arrays. The two dimensional, three dimensional or other dimensional arrays are also known as multidimensional arrays.
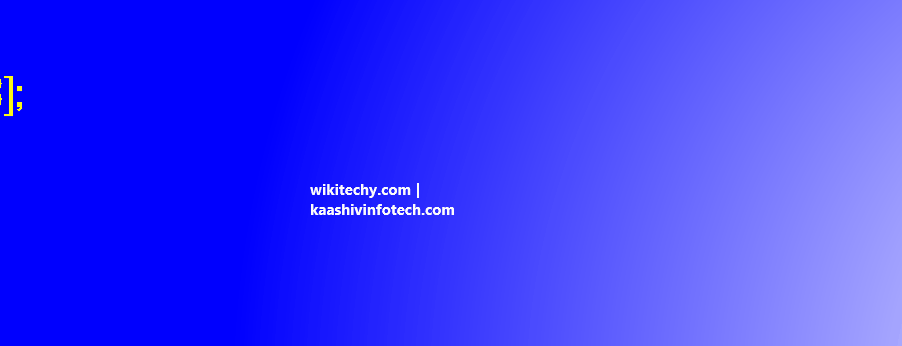
Syntax
data type array name[size1][size2];
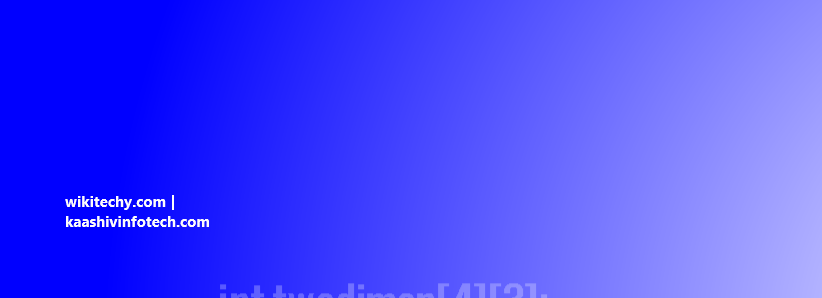
- A way to initialize the two dimensional array at the time of declaration is given below:
int arr[4][3]={{1,2,3},{2,3,4},{3,4,5},{4,5,6}};
Sample Code:
#include<stdio.h>
void main()
{
int i=0,j=0;
int arr[4][3]={{1,2,3},{2,3,4},{3,4,5},{4,5,6}};
for(i=0;i<4;i++)
{
for(j=0;j<3;j++)
{
printf("arr[%d] [%d] = %d \n",i,j,arr[i][j]);
}
}
getch();
}
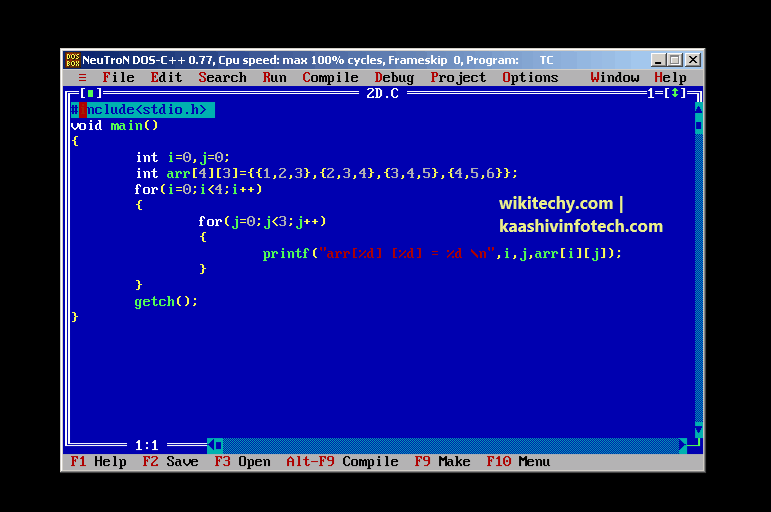
Output:
arr[0] [0] = 1
arr[0] [1] = 2
arr[0] [2] = 3
arr[1] [0] = 2
arr[1] [1] = 3
arr[1] [2] = 4
arr[2] [0] = 3
arr[2] [1] = 4
arr[2] [2] = 5
arr[3] [0] = 4
arr[3] [1] = 5
arr[3] [2] = 6