TypeDef in C
TypeDef in C
- The C programming language provides a keyword called typedef, by using this keyword you can create a user defined name for existing data type.
- Generally typedef are use to create an alias name (nickname).
Declaration of typedef
typedef datatype alias_name;
Example:
typedef int intdata;
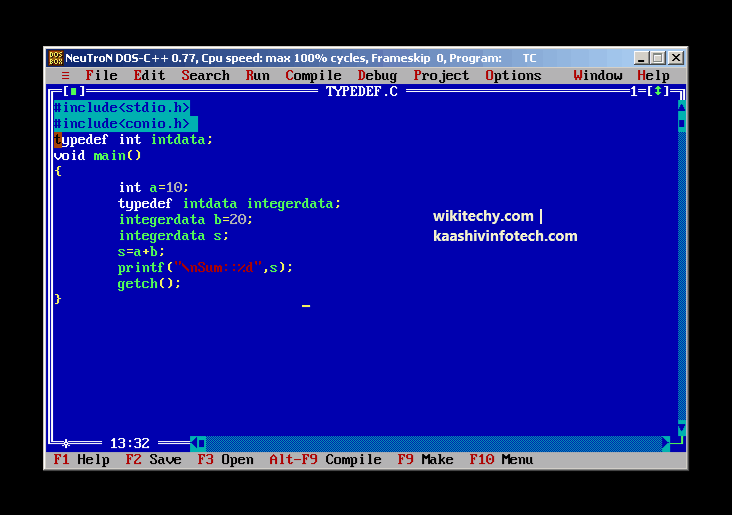
Sample Code
#include<stdio.h>
#include<conio.h>
typedef int intdata;
void main()
{
int a=10;
typedef intdata integerdata;
integerdata b=20;
integerdata s;
s=a+b;
printf("\nSum::%d",s);
getch();
}
Read Also
TypeDef in linuxCode Explanation
- In above program Intdata is an user defined name or alias name for an integer datatype.
- All properties of the integer will be applied on Intdata also.
- Integerdata is an alias name to existing user defined name called Intdata.
Output
Sum::30
Advantages of typedef:
- It makes the program more portable.
- typedef make complex declaration easier to understand.
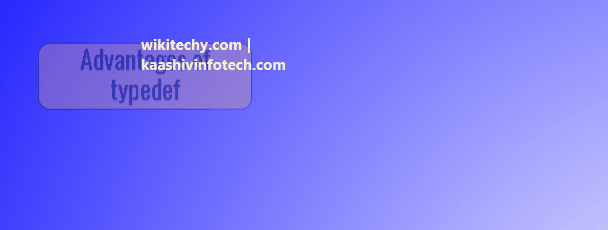
TypeDef With Struct
- We have to include keyword struct every time you declare a new variable, but if we use typedef then the declaration will as easy as below.
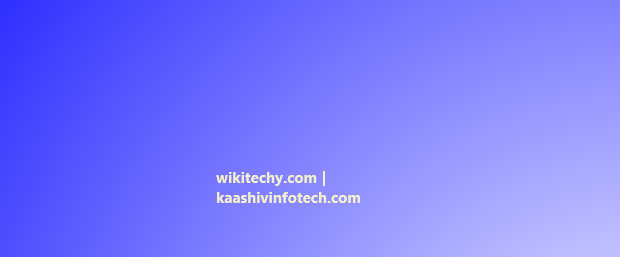
Syntax
typedef struct{
int id;
char *name;
float percentage;
}student;
student a,b;