C - File Handling
C File Handling
- In C – Programming the files I/O functions handles data on secondary storage device, such as hard disk.
- In C – Programming the I/O function handles the text files(.txt) into system oriented files.
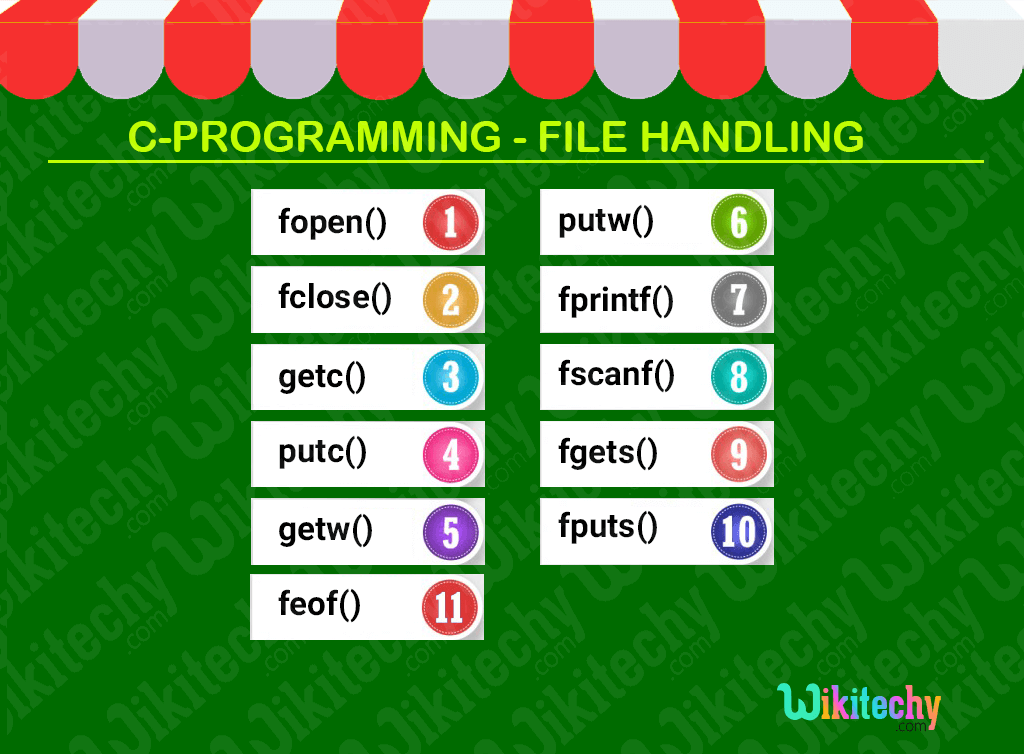
C File Operations :
- There are five major operations that can be performed on a file:
- Creation of a new file.
- Opening an existing file.
- Reading data from a file.
- Writing data in a file.
- Closing a file
Steps for Processing a File :
- Declare a file pointer variable.
- Open a file using fopen() function.
- Process the file using suitable function.
- Close the file using fclose() function.
To handle files in C, file input/output functions available in the stdio library are :
- fopen - Opens a file.
- fclose - Closes a file.
- getc - Reads a character from a file
- putc - Writes a character to a file
- getw - Read integer
- putw - Write integer
- fprintf - Prints formatted output to a file
- fscanf - Reads formatted input from a file
- fgets - Read string of characters from file
- fputs - Write string of characters to file
- feof - Detects end-of-file marker in a file
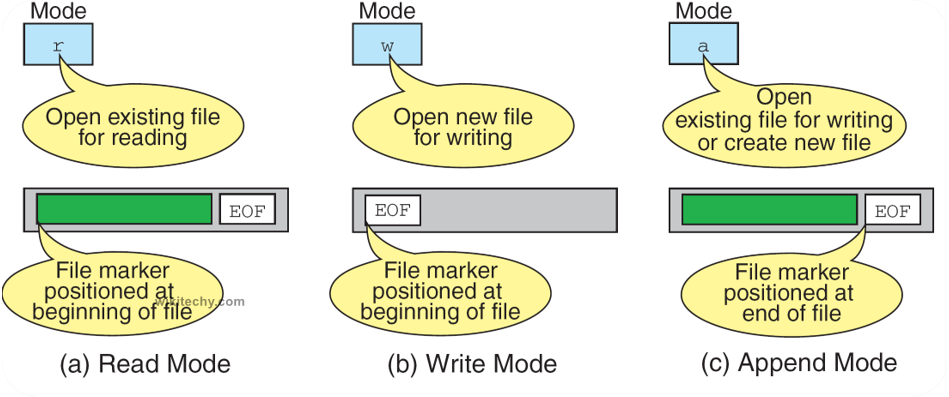
Learn C - C tutorial - File Operations - C examples - C programs
Why File Handling in C
- One more disadvantage is that, it suffers from the performance in writing and reading from the file because data must be converted while writing and reading from the file.
- We can save the memory and improve the performance by directly writing the binary data on to the file. The file with the binary data is called a binary file. One more advantage with binary file is that, can’t be opened with any other applications.