while Loop C++ - Learn C++ - C++ Tutorial - C++ programming
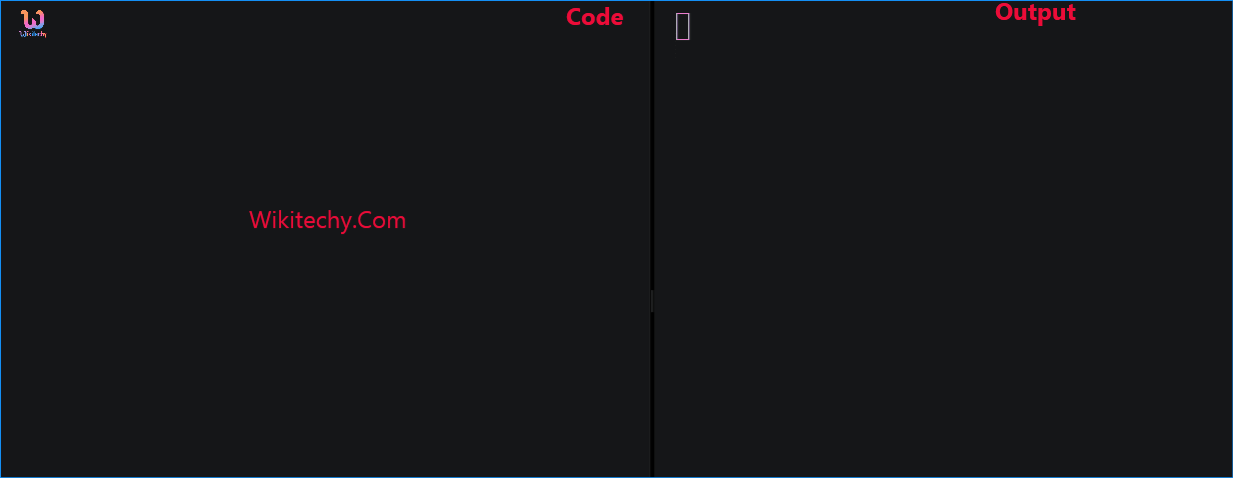
Learn c++ - c++ tutorial - while loop c++ - c++ examples - c++ programs
What is Loop in C++
- Loops are used in programming to repeat a specific block of code. In this article, you will learn to create while and do...while loops in C++ programming.

- A loop statement allows us to execute a statement or group of statements multiple times
- In computer programming, loop repeats a certain block of code until some end condition is met.
- There are 3 type of loops in C++ Programming:
- for Loop
- while Loop
- do...while Loop
- In this we are going to explain while loop and do while loop
C++ while Loop
- A while loop is a control flow statement that allows code to be executed repeatedly based on a given Boolean condition.
- The while loop evaluates the test expression.
- If the test expression is true, codes inside the body of while loop is evaluated.
- Then, the test expression is evaluated again. This process goes on until the test expression is false.
- When the test expression is false, while loop is terminated.
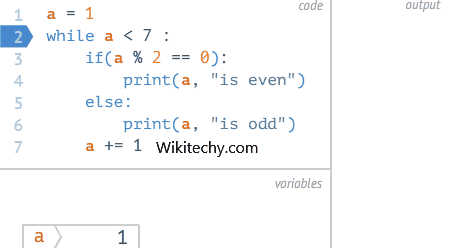
learn c++ tutorials - while loop Example
Syntax of a while loop
while (testExpression)
{
// codes
}
- where, testExpression is checked on each entry of the while loop.
Learn C++ , C++ Tutorial , C++ programming - C++ Language -Cplusplus
Flowchart of while Loop

Example 1: C++ while Loop
// C++ Program to compute factorial of a number
// Factorial of n = 1*2*3...*n
#include <iostream>
using namespace std;
int main()
{
int number, i = 1, factorial = 1;
cout << "Enter a positive integer: ";
cin >> number;
while ( i <= number) {
factorial *= i; //factorial = factorial * i;
++i;
}
cout<<"Factorial of "<< number <<" = "<< factorial;
return 0;
}
Output
Enter a positive integer: 4
Factorial of 4 = 24
- In this program, user is asked to enter a positive integer which is stored in variable number. Let's suppose, user entered 4.
- Then, the while loop starts executing the code. Here's how while loop works:
- Initially, i = 1, test expression i <= number is true and factorial becomes 1.
- Variable i is updated to 2, test expression is true, factorial becomes 2.
- Variable i is updated to 3, test expression is true, factorial becomes 6.
- Variable i is updated to 4, test expression is true, factorial becomes 24.
- Variable i is updated to 5, test expression is false and while loop is terminated.
C++ do...while Loop
- The do...while loop is a variant of the while loop with one important difference.
- The body of do...while loop is executed once before the test expression is checked.
- The codes inside the body of loop is executed at least once. Then, only the test expression is checked.
- If the test expression is true, the body of loop is executed. This process continues until the test expression becomes false.
- When the test expression is false, do...while loop is terminated
Learn C++ , C++ Tutorial , C++ programming - C++ Language -Cplusplus
Syntax of do..while loop
do {
// codes;
}
while (testExpression);
Flowchart of do...while Loop

Example 2: C++ do...while Loop
// C++ program to add numbers until user enters 0
#include <iostream>
using namespace std;
int main()
{
float number, sum = 0.0;
do {
cout<<"Enter a number: ";
cin>>number;
sum += number;
}
while(number != 0.0);
cout<<"Total sum = "<<sum;
return 0;
}
Output
Enter a number: 2
Enter a number: 3
Enter a number: 4
Enter a number: -4
Enter a number: 2
Enter a number: 4.4
Enter a number: 2
Enter a number: 0