Namespace in C++ - Learn C++ - C++ Tutorial - C++ programming
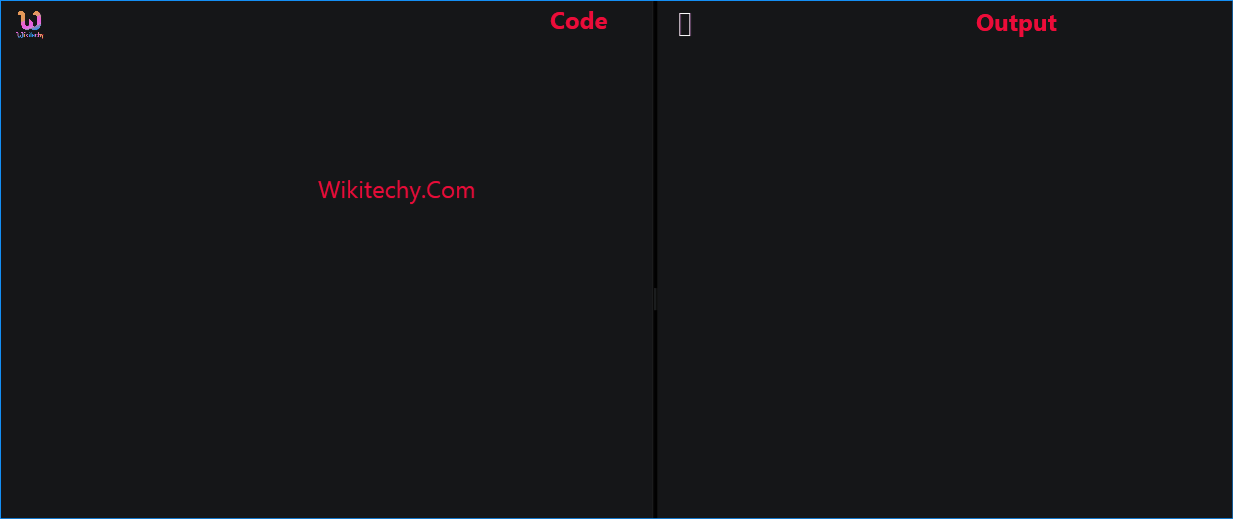
Learn c++ - c++ tutorial - namespace in c++ - c++ examples - c++ programs
Namespace:
- Namespace is a container for identifiers. It puts the names of its members in a distinct space so that they don't conflict with the names in other namespaces or global namespace.
- Namespaces in C++ are used to organize too many classes so that it can be easy to handle the application.
- For accessing the class of a namespace, we need to use namespacename::classname. We can use using keyword so that we don't have to use complete name all the time.
- In C++, global namespace is the root namespace. The global::std will always refer to the namespace "std" of C++ Framework.
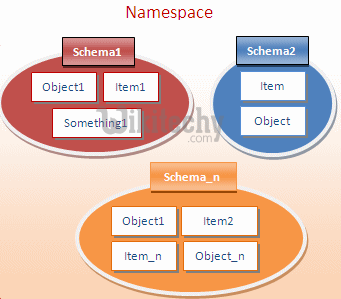
learn c++ tutorials - namespace Example
Learn C++ , C++ Tutorial , C++ programming - C++ Language -Cplusplus
Creating a Namespace:
- Creating a namespace is similar to creation of a class.
namespace MySpace
{
// Declarations
}
int main() {}
- This will create a namespace called MySpace, inside which we can put our member declarations.
Rules to create Namespace:
- The namespace definition must be done at global scope, or nested inside another namespace.
- Namespace definition doesn't terminate with a semicolon like in class definition.
- You can use an alias name for your namespace name, for ease of use. Example for Alias :
namespace wikitechyDotCom
{
void study();
class Learn { };
}
namespace St = wikitechyDotCom; // St is now alias for wikitechyDotCom
- You cannot create instance of namespace.
- There can be unnamed namespaces too. Unnamed namespace is unique for each translation unit. They act exactly like named namespaces. Example for Unnamed namespace :
namespace
{
class Head { };
class Tail { };
int i,j,k;
}
int main() { }
- A namespace definition can be continued and extended over multiple files, they are not redefined or overriden.
Example :
Header1.h
namespace MySpace
{
int x;
void f();
}
Header2.h
#include "Header1.h";
namespace MySpace
{
int y;
void g();
}
C++ namespace Example:
- Let's see the simple example of namespace which include variable and functions.
#include <iostream>
using namespace std;
namespace First {
void sayHello() {
cout<<"Hello First Namespace"<<endl;
}
}
namespace Second {
void sayHello() {
cout<<"Hello Second Namespace"<<endl;
}
}
int main()
{
First::sayHello();
Second::sayHello();
return 0;
}
Output:
Hello First Namespace
Hello Second Namespace
Accessing a namespace with the scope resolution operator (::)
- The first way to tell the compiler to look in a particular namespace for an identifier is to use the scope resolution operator (::).
- This operator allows you to prefix an identifier name with the namespace you wish to use.
- Here is an example of using the scope resolution operator to tell the compiler that we explicitly want to use the version of doSomething that lives in the Foo namespace:
int main(void)
{
std::cout << Foo::doSomething(4, 3);
return 0;
}
Output:
7
- If we wanted to use the version of doSomething() that lives in Goo instead:
int main(void)
{
std::cout << Goo::doSomething(4, 3);
return 0;
}
Learn C++ , C++ Tutorial , C++ programming - C++ Language -Cplusplus
Output:
1
- The scope resolution operator is very nice because it allows us to specifically pick which namespace we want to look in. It even allows us to do the following:
int main(void)
{
std::cout << Foo::doSomething(4, 3) << '\n';
std::cout << Goo::doSomething(4, 3) << '\n';
return 0;
}
Output:
7
1
- It is also possible to use the scope resolution operator without any namespace (eg. ::doSomething).
- In that case, it refers to the global namespace.
Nested namespaces and namespace aliases
- Namespaces can be nested inside other namespaces. For example:
#include <iostream>
namespace Foo
{
namespace Goo
{
const int g_x = 5;
}
}
int main()
{
std::cout << Foo::Goo::g_x;
return 0;
}
- Note that because namespace Goo is inside of namespace Foo, we access g_x as Foo::Goo::g_x.
- Because typing the fully qualified name of a variable or function inside a nested namespace can be painful, C++ allows you to create namespace aliases.
namespace Foo
{
namespace Goo
{
const int g_x = 5;
}
}
namespace Boo = Foo::Goo; // Boo now refers to Foo::Goo
int main()
{
std::cout << Boo::g_x; // This is really Foo::Goo::g_x
return 0;
}