C++ Array - Learn C++ - C++ Tutorial - C++ programming
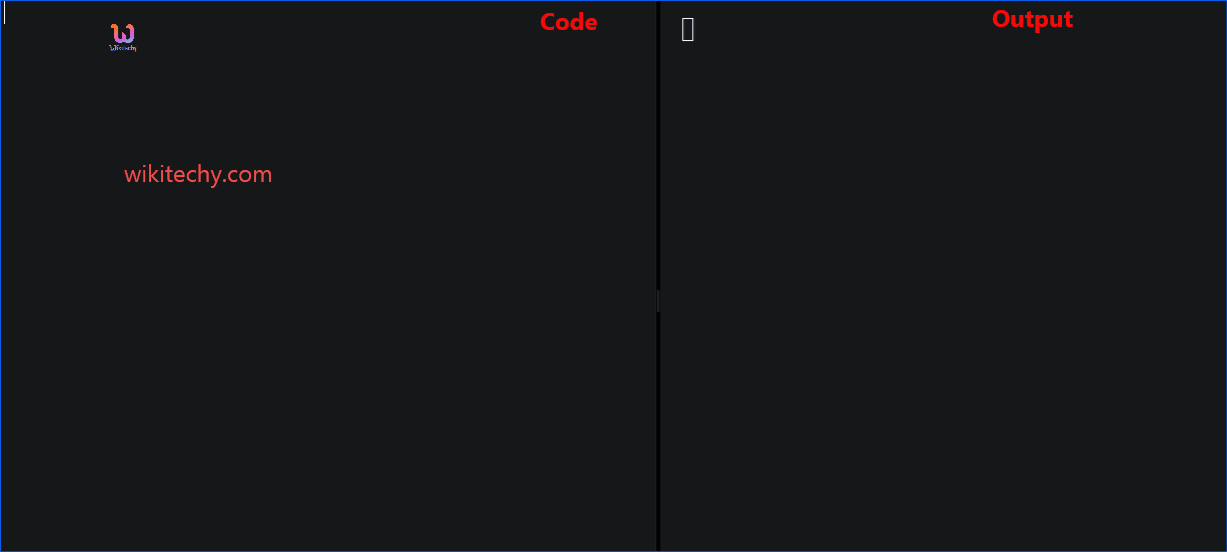
Learn c++ - c++ tutorial - c++ arrays - c++ examples - c++ programs

Learn C++ , C++ Tutorial , C++ programming - C++ Language -Cplusplus
Arrays:
- C++ provides a data structure, the array, which stores a fixed-size sequential collection of elements of the same type.
- An array is used to store a collection of data, but it is often more useful to think of an array as a collection of variables of the same type.
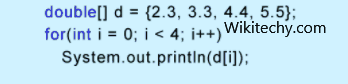
learn c++ tutorials - arrays Example
For example:
int age[100];
- Here, the age array can hold maximum of 100 elements of integer type.
- The size and type of arrays cannot be changed after its declaration.
How to declare an array in C++?
dataType arrayName[arraySize];
For example,
float mark[5];
- Here, we declared an array, mark, of floating-point type and size 5. Meaning, it can hold 5 floating-point values.
Elements of an Array and How to access them?
- You can access elements of an array by using indices.
- Suppose you declared an array mark as above. The first element is mark[0], second element is mark[1] and so on.

Learn C++ , C++ Tutorial , C++ programming - C++ Language -Cplusplus
Few key notes:
- Arrays have 0 as the first index not 1. In this example, mark[0] is the first element.
- If the size of an array is n, to access the last element, (n-1) index is used. In this example, mark[4] is the last element.
- Suppose the starting address of mark[0] is 2120d. Then, the next address, a[1], will be 2124d, address of a[2] will be 2128d and so on. It's because the size of a float is 4 bytes.
How to initialize an array in C++ programming?
- It's possible to initialize an array during declaration. For example,
int mark[5] = {19, 10, 8, 17, 9};
- Another method to initialize array during declaration:
int mark[] = {19, 10, 8, 17, 9};

- Here,
mark[0] is equal to 19
mark[1] is equal to 10
mark[2] is equal to 8
mark[3] is equal to 17
mark[4] is equal to 9
Learn C++ , C++ Tutorial , C++ programming - C++ Language -Cplusplus
How to insert and print array elements?
int mark[5] = {19, 10, 8, 17, 9}
// insert different value to third element
mark[3] = 9;
// take input from the user and insert in third element
cin >> mark[2];
// take input from the user and insert in (i+1)th element
cin >> mark[i];
// print first element of an array
cout << mark[0];
// print ith element of an array
cin >> mark[i-1];
Example: C++ Array
- C++ program to store and calculate the sum of 5 numbers entered by the user using arrays.
#include <iostream>
using namespace std;
int main()
{
int numbers[5], sum = 0;
cout << "Enter 5 numbers: ";
// Storing 5 number entered by user in an array
// Finding the sum of numbers entered
for (int i = 0; i < 5; ++i)
{
cin >> numbers[i];
sum += numbers[i];
}
cout << "Sum = " << sum << endl;
return 0;
}
Output
Enter 5 numbers: 3
4
5
4
2
Sum = 18
Learn C++ , C++ Tutorial , C++ programming - C++ Language -Cplusplus
Multidimensional Arrays in C++:

- In C++, you can create an array of an array known as multi-dimensional array. For example:
int x[3][4];
- Here, x is a two dimensional array. It can hold a maximum of 12 elements.
- You can think this array as table with 3 rows and each row has 4 columns as shown below.

- Three dimensional array also works in a similar way. For example:
float x[2][4][3];
- This array x can hold a maximum of 24 elements. You can think this example as: Each of the 2 elements can hold 4 elements, which makes 8 elements and each of those 8 elements can hold 3 elements. Hence, total number of elements this array can hold is 24.
Multidimensional Array Initialization:
- You can initialize a multidimensional array in more than one way.
Initialisation of two dimensional array
int test[2][3] = {2, 4, -5, 9, 0, 9};
- Better way to initialize this array with same array elements as above.
int test[2][3] = { {2, 4, 5}, {9, 0 0}};
Learn C++ , C++ Tutorial , C++ programming - C++ Language -Cplusplus
Example :Two Dimensional Array
- C++ Program to display all elements of an initialised two dimensional array.
#include <iostream>
using namespace std;
int main()
{
int test[3][2] =
{
{2, -5},
{4, 0},
{9, 1}
};
// Accessing two dimensional array using
// nested for loops
for(int i = 0; i < 3; ++i)
{
for(int j = 0; j < 2; ++j)
{
cou t<< "test[" << i << "][" << j << "] = " << test[i][j] << endl;
}
}
return 0;
}
Output
test[0][0] = 2
test[0][1] = -5
test[1][0] = 4
test[1][1] = 0
test[2][0] = 9
test[2][1] = 1