Default Arguments in C++ - Learn C++ , C++ Tutorial , C++ programming
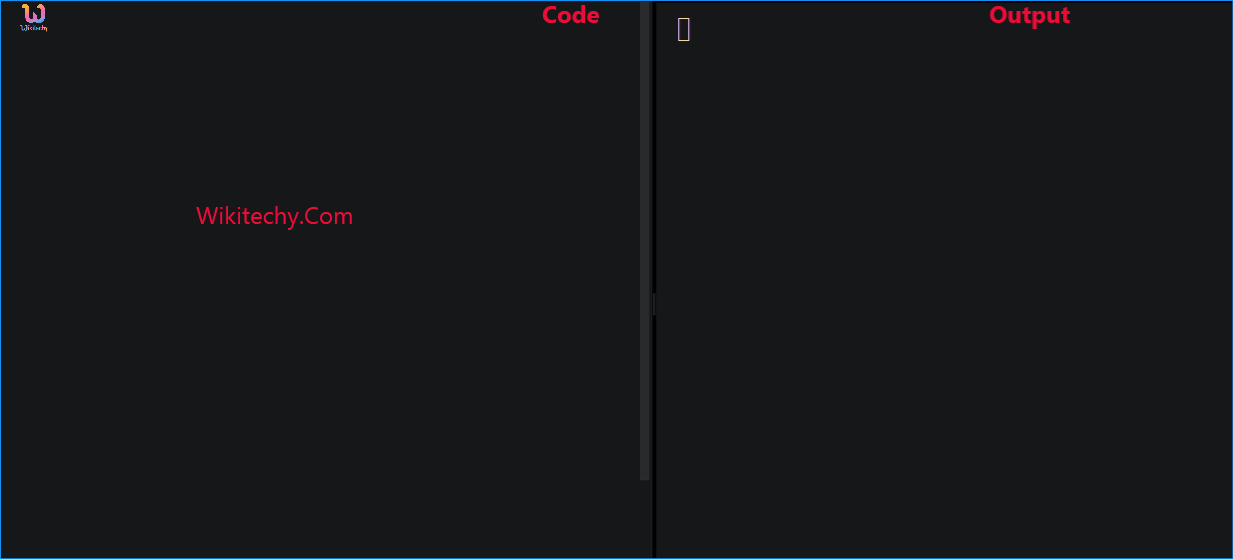
Learn c++ - c++ tutorial - default arguments in c++ - c++ examples - c++ programs
Default Arguments in C++:
- A default argument is a value provided in function declaration that is automatically assigned by the compiler if caller of the function doesn’t provide a value for the argument with default value.
- The idea behind default argument is simple. If a function is called by passing argument/s, those arguments are used by the function.
- But if the arguments are not passed while invoking a function then, the default values are used.
- Default value/s are passed to argument/s in the function prototype
Working of default arguments:

Example: Default Argument:
// C++ Program to demonstrate working of default argument
#include <iostream>
using namespace std;
void display(char = '*', int = 1);
int main()
{
cout << "No argument passed:\n";
display();
cout << "\nFirst argument passed:\n";
display('#');
cout << "\nBoth argument passed:\n";
display('$', 5);
return 0;
}
void display(char c, int n)
{
for(int i = 1; i <= n; ++i)
{
cout << c;
}
cout << endl;
}
Output:
No argument passed:
*
First argument passed:
#
Both argument passed:
$$$$$
- In the above program, you can see the default value assigned to the arguments void display(char = '*', int = 1);.
- At first, display() function is called without passing any arguments. In this case, display()function used both default arguments c = * and n = 1.
- Then, only the first argument is passed using the function second time. In this case, function does not use first default value passed. It uses the actual parameter passed as the first argument c = # and takes default value n = 1 as its second argument.
- When display() is invoked for the third time passing both arguments, default arguments are not used. So, the value of c = $ and n = 5.