C++ Basics | Structure of a program - Learn C++ , C++ Tutorial , C++ programming
Basics Of C++
- A computer program is a sequence of instructions that tell the computer what to do.
- The most common type of instruction in a program is the statement.
- A statement in C++ is the smallest independent unit in the language.
- In human language, it is analogous to a sentence. We write sentences in order to convey an idea.
- In C++, we write statements in order to convey to the compiler that we want to perform a task. Statements in C++ are often terminated by a semicolon.
- Before starting the abcd of C++ language, you need to learn how to write, compile and run the first C++ program.
- To write the first C++ program, open the C++ console and write the following code:
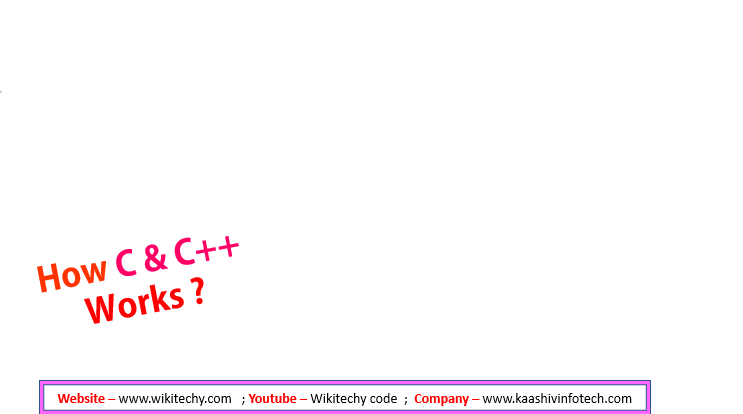
#include <iostream.h>
#include<conio.h>
void main()
{
clrscr();
cout << "Welcome to C++ Programming.";
getch();
}
- #include<iostream.h> includes the standard input output library functions. It provides cin and cout methods for reading from input and writing to output respectively.
- #include includes the console input output library functions. The getch() function is defined in conio.h file.
- Those are special type of statement called a preprocessor directive. Preprocessor directives tell the compiler to perform a special task.
- In this case, we are telling the compiler that we would like to add the contents of the iostream header to our program.
- The iostream header allows us to access functionality from the iostream library, which will allow us to write text to the screen.
- The 3rd line is blank, and is ignored by the compiler.
- void main() The main() function is the entry point of every program in C++ language.
- The void keyword specifies that it returns no value. declares the main() function, which as you learned above, is mandatory. Every program must have a main() function.
- The 5th and 9th line of the code explains tell the compiler which lines are part of the main function.
- Everything between the opening curly brace on line 4 and the closing curly brace on line 7 is considered part of the main() function.
- cout << "Welcome to C++ Programming." is used to print the data "Welcome to C++ Programming." on the console.
- getch() The getch() function asks for a single character. Until you press any key, it blocks the screen.
