golang tutorial - Writing Files In Golang | Writing Files In Go - golang - go programming language - google go - go language - go program - google language
Writing Files In Golang
- Writing files in Go follows similar patterns to the ones we saw reading files.
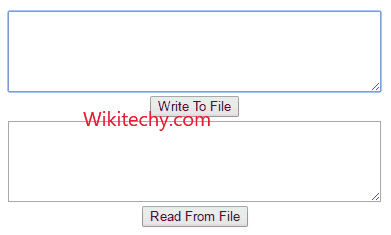
package main
import (
"bufio"
"fmt"
"io/ioutil"
"os"
)
func check(e error) {
if e != nil {
panic(e)
}
}
func main() {
// To start, here’s how to dump a string (or just bytes) into a file.
d1 := []byte("hello\ngo\n")
err := ioutil.WriteFile("/tmp/dat1", d1, 0644)
check(err)
// For more granular writes, open a file for writing.
f, err := os.Create("/tmp/dat2")
check(err)
// It’s idiomatic to defer a Close immediately after opening a file.
defer f.Close()
// You can Write byte slices as you’d expect.
d2 := []byte{115, 111, 109, 101, 10}
n2, err := f.Write(d2)
check(err)
fmt.Printf("wrote %d bytes\n", n2)
//A WriteString is also available.
n3, err := f.WriteString("writes\n")
fmt.Printf("wrote %d bytes\n", n3)
// Issue a Sync to flush writes to stable storage.
f.Sync()
// bufio provides buffered writers in addition to the buffered readers we saw earlier.
w := bufio.NewWriter(f)
n4, err := w.WriteString("buffered\n")
fmt.Printf("wrote %d bytes\n", n4)
// Use Flush to ensure all buffered operations have been applied to the underlying writer.
w.Flush()
}
// Try running the file-writing code.
$ go run writing-files.go
wrote 5 bytes
wrote 7 bytes
wrote 9 bytes
// Then check the contents of the written files.
$ cat /tmp/dat1
click below button to copy the code. By - golang tutorial - team
golang , gopro , google go , golang tutorial , google language , go language , go programming language
Output of the above go program
hello
go
$ cat /tmp/dat2
some
writes
buffered