golang tutorial - Go Lang If | if - if else - switch - go programming - golang - go programming language - google go - go language - go program - google language
Golang If
- Decision making is used to specify the order in which statements are executed. In this tutorial, you will learn to create decisions using different forms of if...else statement.
- Go programming language provides following types of decision making statements.
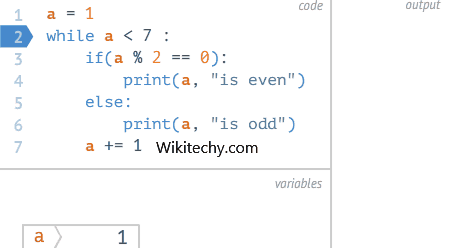
Statement | Description |
---|---|
if statement | The if statement evaluates the test expression inside the parenthesis. If the test expression is evaluated to true (nonzero), statements inside the body of if is executed. If the test expression is evaluated to false (0), statements inside the body of if is skipped from execution |
if...else statement | An if statement can be followed by an optional else statement, which executes when the boolean expression is false. |
nested if statements | You can use one if or else if statement inside another if or else if statement(s). |
switch statement | A switch statement allows a variable to be tested for equality against a list of values. |
select statement | A select statement is similar to switch statement with difference as case statements refers to channel communications. |
golang , gopro , google go , golang tutorial , google language , go language , go programming language
C if statement
if (testExpression)
{
// statements
}
click below button to copy the code. By - golang tutorial - team
- The if statement evaluates the test expression inside the parenthesis.
- If the test expression is evaluated to true (nonzero), statements inside the body of if is executed.
- If the test expression is evaluated to false (0), statements inside the body of if is skipped from execution.
Flowchart of if statement
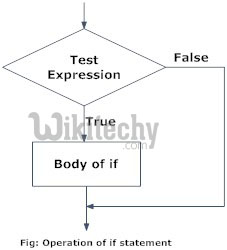
C if...else statement
- The if...else statement executes some code if the test expression is true (nonzero) and some other code if the test expression is false (0).
Syntax of if...else
if (testExpression) {
// codes inside the body of if
}
else {
// codes inside the body of else
}
click below button to copy the code. By - golang tutorial - team
- If test expression is true, codes inside the body of if statement is executed and, codes inside the body of else statement is skipped.
- If test expression is false, codes inside the body of else statement is executed and, codes inside the body of if statement is skipped.
Flowchart of if...else statement
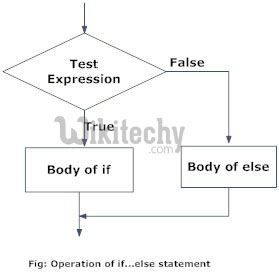
golang , gopro , google go , golang tutorial , google language , go language , go programming language
Nested if...else statement (if...elseif....else Statement)
- The if...else statement executes two different codes depending upon whether the test expression is true or false. Sometimes, a choice has to be made from more than 2 possibilities.
- The nested if...else statement allows you to check for multiple test expressions and execute different codes for more than two conditions.
Syntax of nested if...else statement
if (testExpression1)
{
// statements to be executed if testExpression1 is true
}
else if(testExpression2)
{
// statements to be executed if testExpression1 is false and testExpression2 is true
}
else if (testExpression 3)
{
// statements to be executed if testExpression1 and testExpression2 is false and testExpression3 is true
}
.
.
else
{
// statements to be executed if all test expressions are false
}
click below button to copy the code. By - golang tutorial - team
FlowChart for Nested If Else Statment
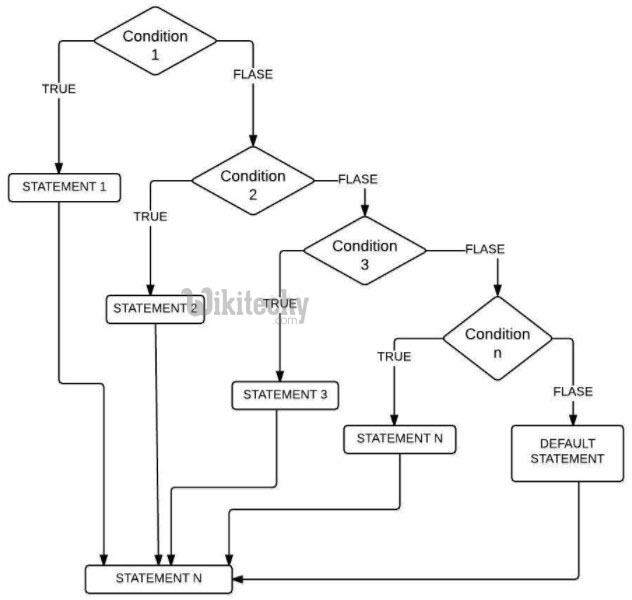
If Loops in Go programming Language
package main
import "fmt"
func main() {
if 7%2 == 0 {
fmt.Println("7 is even")
} else {
fmt.Println("7 is odd")
}
// You can have an if statement without an else.
if 8%4 == 0 {
fmt.Println("8 is divisible by 4")
}
// A statement can precede conditionals; any variables declared in this statement are available in all branches.
if num := 9; num < 0 {
fmt.Println(num, "is negative")
} else if num < 10 {
fmt.Println(num, "has 1 digit")
} else {
fmt.Println(num, "has multiple digits")
}
}
click below button to copy the code. By - golang tutorial - team
golang , gopro , google go , golang tutorial , google language , go language , go programming language
Output of the above go program
$ go run if-else.go
7 is odd
8 is divisible by 4
9 has 1 digit
Go by Example: Switch case in go language programming
- Switch statements express conditionals across many branches.
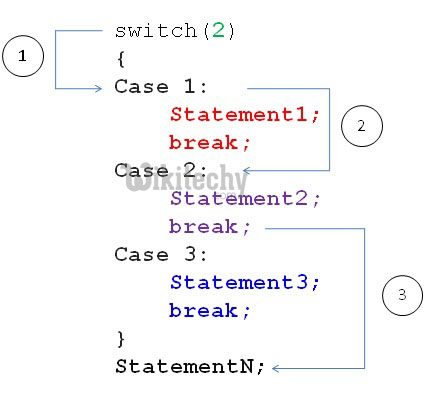
package main
import "fmt"
import "time"
func main() {
// basic switch statement
i := 2
fmt.Print("Write ", i, " as ")
switch i {
case 1:
fmt.Println("one")
case 2:
fmt.Println("two")
case 3:
fmt.Println("three")
}
// You can use commas to separate multiple expressions in the same case statement.
//We use the optional default case in this example as well.
click below button to copy the code. By - golang tutorial - team
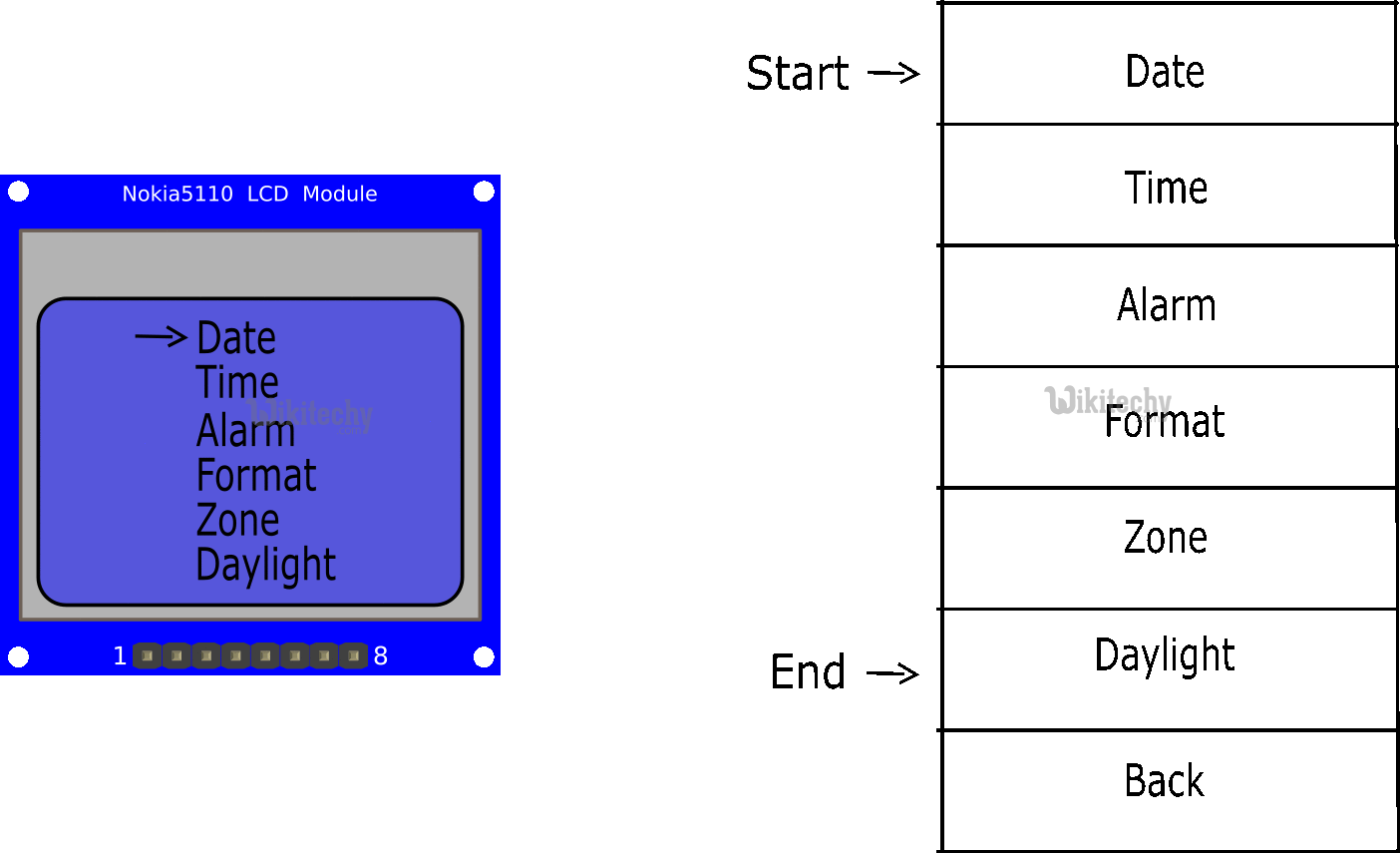
i := 2
fmt.Print("Write ", i, " as ")
switch i {
case 1:
fmt.Println("one")
case 2:
fmt.Println("two")
case 3:
fmt.Println("three")
}
// You can use commas to separate multiple expressions in the same case statement.
//We use the optional default case in this example as well.
switch time.Now().Weekday()
{
case time.Saturday,
time.Sunday:
fmt.Println("It's the weekend")
default:
fmt.Println("It's a weekday")
}
// switch without an expression is an alternate way to express if/else logic.
// Here we also show how the caseexpressions can be non-constants.
t := time.Now()
switch {
case t.Hour() < 12:
fmt.Println("It's before noon")
default:
fmt.Println("It's after noon")
}
// A type switch compares types instead of values.
//You can use this to discover the the type of an interface value. //In this example, the variable t will have the type corresponding to its clause.
whatAmI := func(i interface{})
{
switch t := i.(type)
{
case bool:
fmt.Println("I'm a bool")
case int:
fmt.Println("I'm an int")
default:
fmt.Printf("Don't know type %T\n", t)
}
}
whatAmI(true)
whatAmI(1)
whatAmI("hey")
}
click below button to copy the code. By - golang tutorial - team
Output of the above program
$ go run switch.go
Write 2 as two
It's a weekday
It's after noon
I'm a bool
I'm an int
Don't know type string