golang tutorial - Golang Env Variable | Golang Var | Environment Variables In Golang - golang - go programming language - google go - go language - go program - google language
Environmental Variables In Golang
- Environment variables are a set of dynamic named values that can affect the way running processes will behave on a computer.
- They are part of the environment in which a process runs.
- For example, a running process can query the value of the TEMP environment variable to discover a suitable location to store temporary files, or the HOME or USERPROFILE variable to find the directory structure owned by the user running the process.
- Examples of environment variables include:
- PATH - a list of directory paths.
- HOME (Unix-like) and USERPROFILE (Microsoft Windows) - indicate where a user's home directory is located in the file system.
- HOME/{.AppName} (Unix-like) and APPDATA\{DeveloperName\AppName} (Microsoft Windows) - for storing application settings.
- LOCALAPPDATA or PROGRAMDATA (shared between users) is used.
- TERM (Unix-like) - specifies the type of computer terminal or terminal emulator being used
- PS1 (Unix-like) - specifies how the prompt is displayed in the Bourne shell and variants.
- MAIL (Unix-like) - used to indicate where a user's mail is to be found.
- TEMP - location where processes can store temporary files
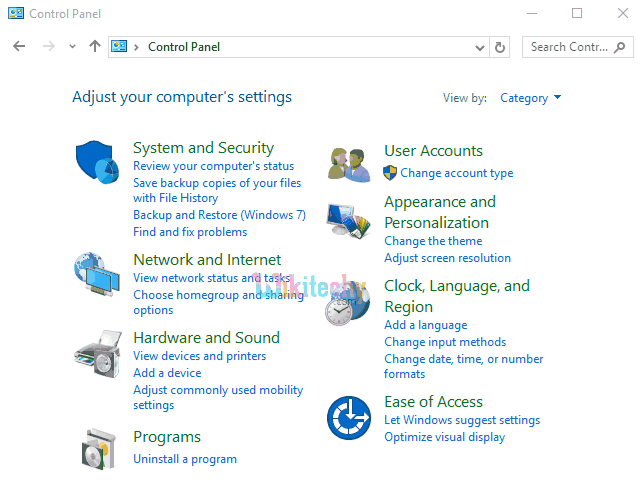
Go language program for environmental variables
package main
import "os"
import "strings"
import "fmt"
func main() {
// To set a key/value pair, use os.Setenv. To get a value for a key, use os.Getenv. This will return an empty string if the key isn’t present in the environment.
os.Setenv("FOO", "1")
fmt.Println("FOO:", os.Getenv("FOO"))
fmt.Println("BAR:", os.Getenv("BAR"))
//Use os.Environ to list all key/value pairs in the environment. This returns a slice of strings in the form KEY=value. You can strings.Split them to get the key and value. Here we print all the keys.
fmt.Println()
for _, e := range os.Environ() {
pair := strings.Split(e, "=")
fmt.Println(pair[0])
}
}
click below button to copy the code. By - golang tutorial - team
golang , gopro , google go , golang tutorial , google language , go language , go programming language
output for the above program
// Running the program shows that we pick up the value for FOO that we set in the program, but that BAR is empty.
$ go run environment-variables.go
FOO: 1
BAR:
// The list of keys in the environment will depend on your particular machine.
TERM_PROGRAM
PATH
SHELL
...
// If we set BAR in the environment first, the running program picks that value up
$ BAR=2 go run environment-variables.go
FOO: 1
BAR: 2
...