golang tutorial - Golang Operators | Operators in go language - golang - go programming language - google go - go language - go program - google language
Golang Operators
- An operator is a symbol that tells the compiler to perform specific mathematical or logical manipulations. Go language is rich in built-in operators and provides the following types of operators
- An operator is a symbol that tells the compiler to perform specific mathematical or logical manipulations. Go language is rich in built-in operators and provides the following types of operators
- Arithmetic Operators
- Relational Operators
- Logical Operators
- Bitwise Operators
- Assignment Operators
- Miscellaneous Operators
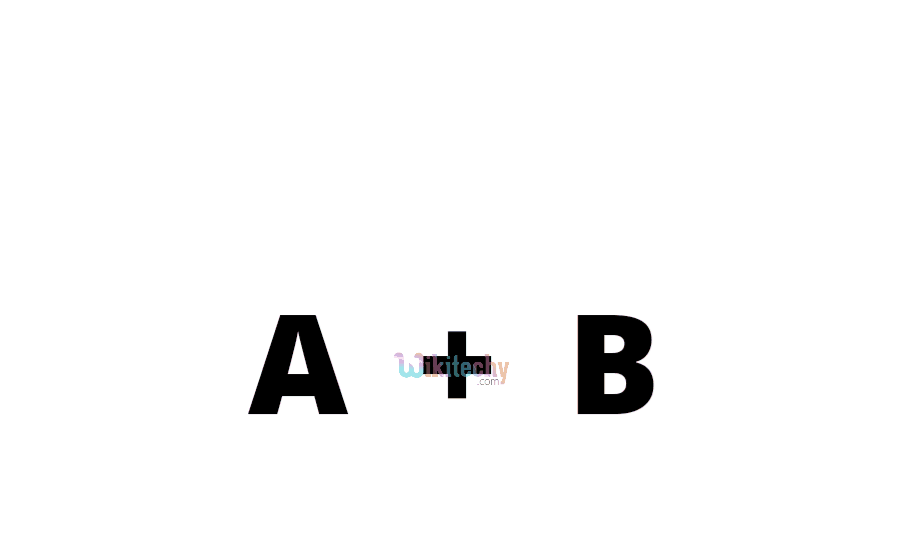
golang , gopro , google go , golang tutorial , google language , go language , go programming language
Arithmetic Operators in go language programming
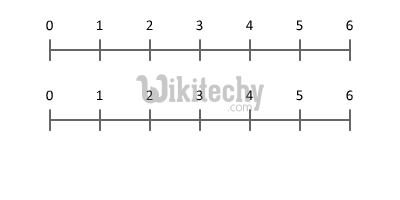
- Considering variable x = 6 and Y = 2. Let’s see how it works,
Operator | Description | Example |
---|---|---|
+ | Adds two operands | X + Y will give 8 |
- | Subtracts second operand from the first | X - Y will give 4 |
* | Multiplies both operands | X * Y will give 12 |
/ | Divides numerator by de-numerator | Y / X will give 3 |
% | Modulus Operator and remainder of after an integer division | Y % X will give 0 |
++ | Increments operator increases integer value by one | X++ will give 7 |
-- | Decrements operator decreases integer value by one | X-- will give 5 |
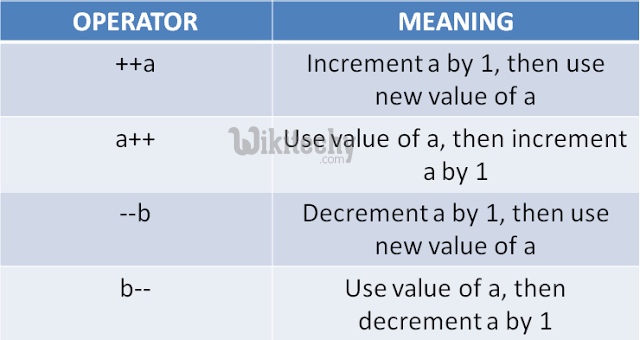
golang , gopro , google go , golang tutorial , google language , go language , go programming language
Relational Operators in go language programming
- Considering I got the variable x = 6 and Y = 2
Show Examples
Operator | Description | Example |
---|---|---|
== | Checks if the values of two operands are equal or not, if yes then condition becomes true. |
(X == Y) is not true. |
!= | Checks if the values of two operands are equal or not, if values are not equal then condition becomes true. |
(X != Y) is true. |
> | Checks if the value of left operand is greater than the value of right operand, if yes then condition becomes true. |
(X > Y) is not true. |
< | Checks if the value of left operand is less than the value of right operand, if yes then condition becomes true. |
(X < Y) is true. |
>= | Checks if the value of left operand is greater than right operand, if yes then condition becomes true. |
(X >= Y) is not true |
<= | Checks if the value of left operand is less than if yes then condition becomes true. |
(X <= Y) is true. |
golang , gopro , google go , golang tutorial , google language , go language , go programming language
Logical Operators in go language programming
- Following table shows all the logical operators supported by Go language. Assume variable X holds 1 and variable Y holds 0, then:
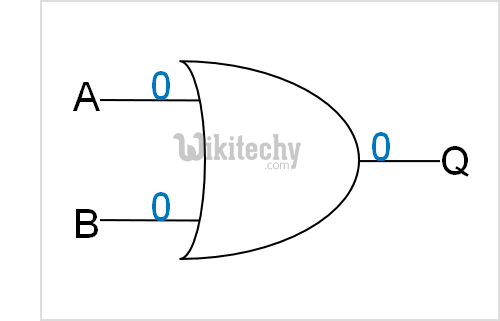
Show Examples
Operator | Description | Example |
---|---|---|
&& | Called Logical AND operator. If both the operands are non-zero, then condition becomes true. |
(X && Y) is false. |
|| | Called Logical OR Operator. If any of the two operands is non-zero, then condition becomes true. |
(X || Y) is true. |
! | Called Logical NOT Operator. Use to reverses the logical state of its operand. If a condition is true then Logical NOT operator will make false. |
!(X && Y) is true. |
golang , gopro , google go , golang tutorial , google language , go language , go programming language
Bitwise Operators in go language programming
- Bitwise operator works on bits and perform bit-by-bit operation.
- The truth tables for AND, OR , NOT is given below,
p | q | p & q | p | q | p ^ q |
---|---|---|---|---|
0 | 0 | 0 | 0 | 0 |
0 | 1 | 0 | 1 | 1 |
1 | 1 | 1 | 1 | 1 |
1 | 0 | 0 | 1 | 1 |
- Assume if X = 60; and Y = 13; now in binary format they will be as follows:
X = 0011 1100
Y = 0000 1101
-----------------
X&Y = 0000 1100
X|Y = 0011 1101
X^Y = 0011 0001
~X = 1100 0011
click below button to copy the code. By - golang tutorial - team
- The Bitwise operators supported by C language are listed in the following table. Assume variable X holds 60 and variable Y holds 13, then:
Operator | Example | |
---|---|---|
& |
|
(X & Y) will give 12, which is 0000 1100 |
| |
|
(X | Y) will give 61, which is 0011 1101 |
<< |
|
X << 2 will give 240 which is 1111 0000 |
^ |
|
(X ^ Y) will give 49, which is 0011 0001 |
>> |
|
X >> 2 will give 15 which is 0000 1111 |
golang , gopro , google go , golang tutorial , google language , go language , go programming language
Assignment Operators in go language programming
- There are following assignment operators supported by Go language
golang , gopro , google go , golang tutorial , google language , go language , go programming language
Show Examples
Operator | Example | |
---|---|---|
= |
|
C = X + Y will assign value of X + Y into C |
+= |
|
C += X is equivalent to C = C + X |
-= |
|
C -= X is equivalent to C = C - X |
*= |
|
= C * X |
/= |
|
C /= X is equivalent to C = C / X |
%= |
|
C %= X is equivalent to C = C % X |
<<= | 39GwAQ7PeH7fJTFa4DXguurfn7GULq2pTs | C <<= 2 is same as C = C << 2 |
>>= | Right shift AND assignment operator | C >>= 2 is same as C = C >> 2 |
&= | Bitwise AND assignment operator | C &= 2 is same as C = C & 2 |
^= | bitwise exclusive OR and assignment operator | C ^= 2 is same as C = C ^ 2 |
|= | bitwise inclusive OR and assignment operator | C |= 2 is same as C = C | 2 |
Misc Operators in go language programming
Operator | Example | |
---|---|---|
& | Returns the address of an variable | &a; will give actual address of the variable. |
* | Pointer to a variable. | *a; will pointer to a variable. |
Operators Precedence in go language programming
- Operator precedence determines the grouping of terms in an expression. This affects how an expression is evaluated. Certain operators have higher precedence than others.
- For example, the multiplication operator has higher precedence than the addition operator.
- For example,
x = 6 + 3 * 2;
click below button to copy the code. By - golang tutorial - team
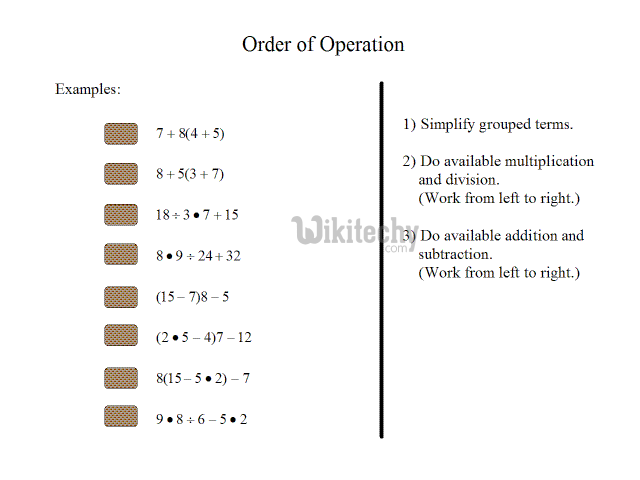
- Following table lists all operators from highest precedence to lowest.
method | Description | |
---|---|---|
Yes | * / % >> << & | Multiply, divide, modulo, Right and left bitwise shift, Bitwise 'AND' |
Yes | + - | ^ | Addition and subtraction, regular `OR', Bitwise exclusive `OR' |
Yes | <= < > >= == != | Comparison operators, Equality and pattern match operators (!= may not be defined as methods) |
&& | Logical 'AND' | |
|| | Logical 'OR' |