golang tutorial - Golang Function | Go - Functions - golang - go programming language - google go - go language - go program - google language
Golang Function
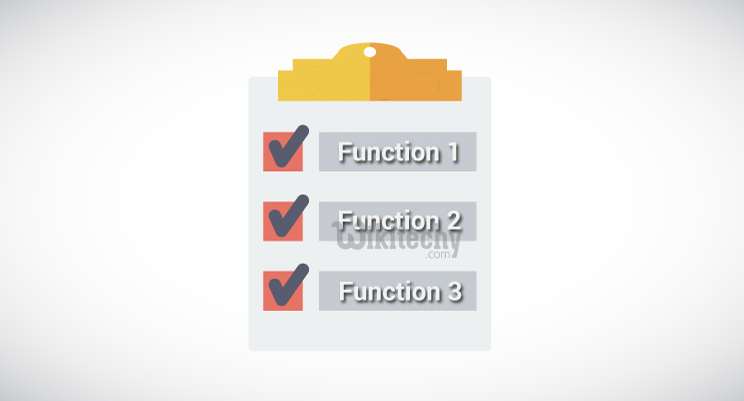
- A function is a block of code that performs a specific task.
- Suppose, a program related to graphics needs to create a circle and color it depending upon the radius and color from the user. You can create two functions to solve this problem:
- create a circle function
- color function
- Dividing complex problem into small components makes program easy to understand and use.
Types of functions
- Depending on whether a function is defined by the user or already included in go compilers, there are two types of functions in go programming
- There are two types of functions in go programming:
- Standard library functions
- User defined functions
golang , gopro , google go , golang tutorial , google language , go language , go programming language
Standard library functions
- The standard library functions are built-in functions in go programming to handle tasks such as
- The standard library functions are built-in functions in go programming to handle tasks such as
- mathematical computations,
- I/O processing,
- string handling etc.
- These functions are defined in the header file. When you include the header file, these functions are available for use. For example
The fmt.Println
is a standard library function to send formatted output to the screen (display output on the screen). This function is defined in import "fmt" header file.
click below button to copy the code. By - golang tutorial - team
User-defined functions
- As mentioned earlier, go allow programmers to define functions. Such functions created by the user are called user-defined functions.
- Depending upon the complexity and requirement of the program, you can create as many user-defined functions as you want.
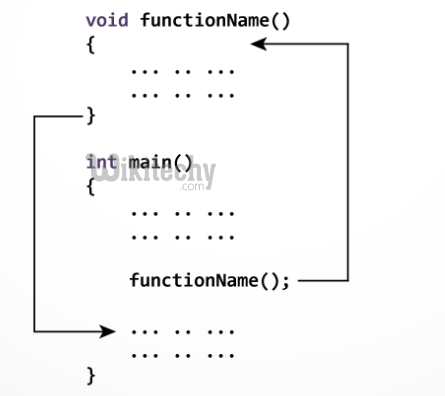
golang , gopro , google go , golang tutorial , google language , go language , go programming language
How user-defined function works?
package main
import "fmt"
func function_name(arguments list if any) return_type
()
{
... .. ...
... .. ...
}
click below button to copy the code. By - golang tutorial - team
func main()
{
... .. ...
... .. ...
functionName(arguments if any);
... .. ...
... .. ...
}
click below button to copy the code. By - golang tutorial - team
- Step 1: The execution of a C program begins from the main() function.
- Step 2: When the compiler encounters functionName(); inside the main function, control of the program jumps to
func function_name(arguments list if any) return_type
click below button to copy the code. By - golang tutorial - team
- Step 3: the compiler starts executing the codes inside the user-defined function.
- Step 4: The control of the program jumps to statement next to functionName(); once all the codes inside the function definition are executed.
Sample program for go functions
package main
import "fmt"
// Here’s a function that takes two ints and returns their sum as an int.
func plus(a int, b int) int {
return a + b
}
// Go requires explicit returns, i.e. it won’t automatically return the value of the last expression.
func plusPlus(a, b, c int) int {
return a + b + c
}
// When you have multiple consecutive parameters of the same type, you may omit the type name for the like-typed parameters up to the final parameter that declares the type.
func main() {
//Call a function just as you’d expect, with name(args).
res := plus(1, 2)
fmt.Println("1+2 =", res)
res = plusPlus(1, 2, 3)
fmt.Println("1+2+3 =", res)
}
click below button to copy the code. By - golang tutorial - team
golang , gopro , google go , golang tutorial , google language , go language , go programming language
Output of the above go language program
$ go run functions.go
1+2 = 3
1+2+3 = 6
Advantages of user-defined function
- The program will be easier to understand, maintain and debug.
- Reusable codes that can be used in other programs
- A large program can be divided into smaller modules. Hence, a large project can be divided among many programmers.