golang tutorial - Golang Parser | Parsing Numbers In Golang - golang - go programming language - google go - go language - go program - google language
Golang Parser
- Parsing, syntax analysis or syntactic analysis is the process of analysing a string of symbols, either in natural language or in computer languages, conforming to the rules of a formal grammar.
- The term has slightly different meanings in different branches of linguistics and computer science.
- Traditional sentence parsing is often performed as a method of understanding the exact meaning of a sentence or word, sometimes with the aid of devices such as sentence diagrams.
- It usually emphasizes the importance of grammatical divisions such as subject and predicate.
- Within computational linguistics the term is used to refer to the formal analysis by a computer of a sentence or other string of words into its constituents, resulting in a parse tree showing their syntactic relation to each other, which may also contain semantic and other information.
- Within computer science, the term is used in the analysis of computer languages, referring to the syntactic analysis of the input code into its component parts in order to facilitate the writing of compilers and interpreters. The term may also be used to describe a split or separation.
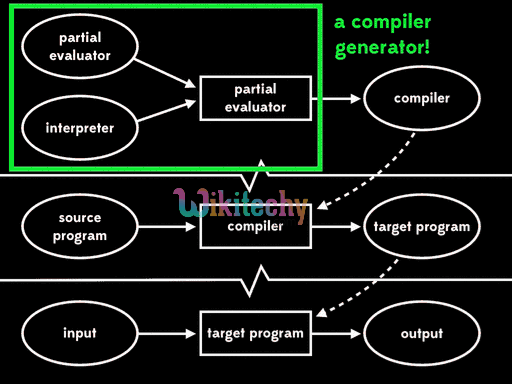
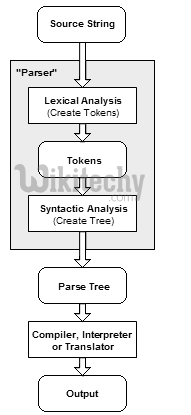
Example : Parsing the Expression 1 + 2 * 3
Set of expression parsing rules (called grammar) is as follows,
Rule1: | E → E + E | Expression is the sum of two expressions. |
Rule2: | E → E * E | Expression is the product of two expressions. |
Rule3: | E → number | Expression is a simple number |
Rule4: | + has less precedence than * |
package main
// The built-in package strconv provides the number parsing.
import "strconv"
import "fmt"
func main() {
// With ParseFloat, this 64 tells how many bits of precision to parse.
f, _ := strconv.ParseFloat("1.234", 64)
fmt.Println(f)
// For ParseInt, the 0 means infer the base from the string. 64 requires that the result fit in 64 bits.
i, _ := strconv.ParseInt("123", 0, 64)
fmt.Println(i)
// ParseInt will recognize hex-formatted numbers.
d, _ := strconv.ParseInt("0x1c8", 0, 64)
fmt.Println(d)
// A ParseUint is also available.
u, _ := strconv.ParseUint("789", 0, 64)
fmt.Println(u)
// Atoi is a convenience function for basic base-10 intparsing.
k, _ := strconv.Atoi("135")
fmt.Println(k)
// Parse functions return an error on bad input.
_, e := strconv.Atoi("wat")
fmt.Println(e)
}
click below button to copy the code. By - golang tutorial - team
golang , gopro , google go , golang tutorial , google language , go language , go programming language
Output for the above go program :
$ go run number-parsing.go
1.234
123
456
789
135
strconv.ParseInt: parsing "wat": invalid syntax