golang tutorial - Interfaces In Golang | Golang Interfaces - golang - go programming language - google go - go language - go program - google language
Interfaces In Golang
- Go programming provides a very important data type called interfaces which provides a clear representation for a set of method signatures.
- struct data type implements these interfaces to have method definitions for the method signature of the interfaces.
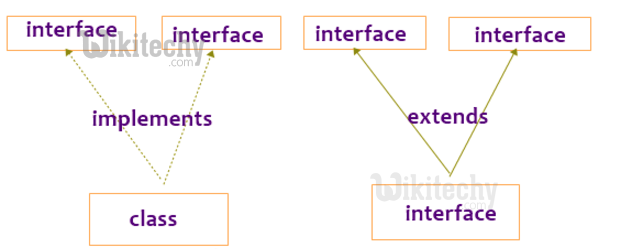
Syntax
/* define an interface */
type interface_name interface {
method_name1 [return_type]
method_name2 [return_type]
method_name3 [return_type]
...
method_namen [return_type]
}
/* define a struct */
type struct_name struct {
/* variables */
}
/* implement interface methods*/
func (struct_name_variable struct_name) method_name1() [return_type] {
/* method implementation */
}
...
func (struct_name_variable struct_name) method_namen() [return_type] {
/* method implementation */
}
click below button to copy the code. By - golang tutorial - team
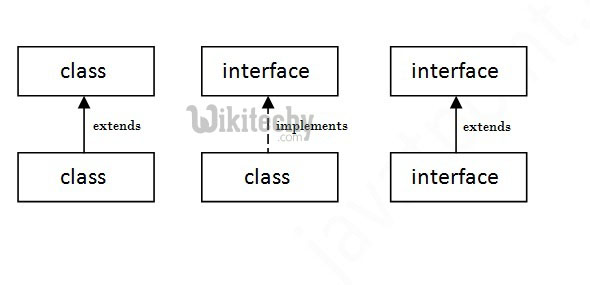
Example
package main
import (
"fmt"
"math"
)
/* define an interface */
type Geometry_Interface interface {
findArea() float64
}
/* define a Circle_Struct1 */
type Circle_Struct1 struct {
x,y,radius float64
}
/* define a Rectangle _Struct2*/
type Rectangle _Struct2 struct {
width, height float64
}
/* define a method for Circle_Struct1 (implementation of Geometry_Interface.findArea())*/
func(Circle_Struct1 Circle_Struct1) findArea() float64 {
return math.Pi * Circle_Struct1.radius * Circle_Struct1.radius
}
/* define a method for Rectangle_Struct2(implementation of Geometry_Interface.findArea())*/
func(rect Rectangle_Struct2) findArea() float64 {
return rect.width * rect.height
}
/* define a method for Geometry_Interface */
func getFindArea(Geometry_Interface Geometry_Interface) float64 {
return Geometry_Interface.findArea()
}
func main() {
Circle_Struct1 := Circle_Struct1{x:0,y:0,radius:5}
Rectangle _Struct2:= Rectangle _Struct2{width:10, height:5}
fmt.Printf("Circle_Struct1 findArea: %f\n",getFindArea(Circle_Struct1))
fmt.Printf("Rectangle _Struct2findArea: %f\n",getFindArea(rectangle))
}
click below button to copy the code. By - golang tutorial - team
When the above code is compiled and executed, it produces the following result:
Circle_Struct1 findArea: 78.539816
Rectangle _Struct2 findArea: 50.000000