Class System in Ext JS
Class System in Ext JS
- A Class can be defined as extensible program code-template that helps to create the objects, provides initial value for the member variables, and implement of methods and member functions.
- In Ext JS, Ext is used as a namespace which is used to encapsulate the classes and create an own class system.
- The essential methods are Ext.apply, Ext.create, Ext.define, Ext.getCmp, Ext.override, and Ext.application etc.
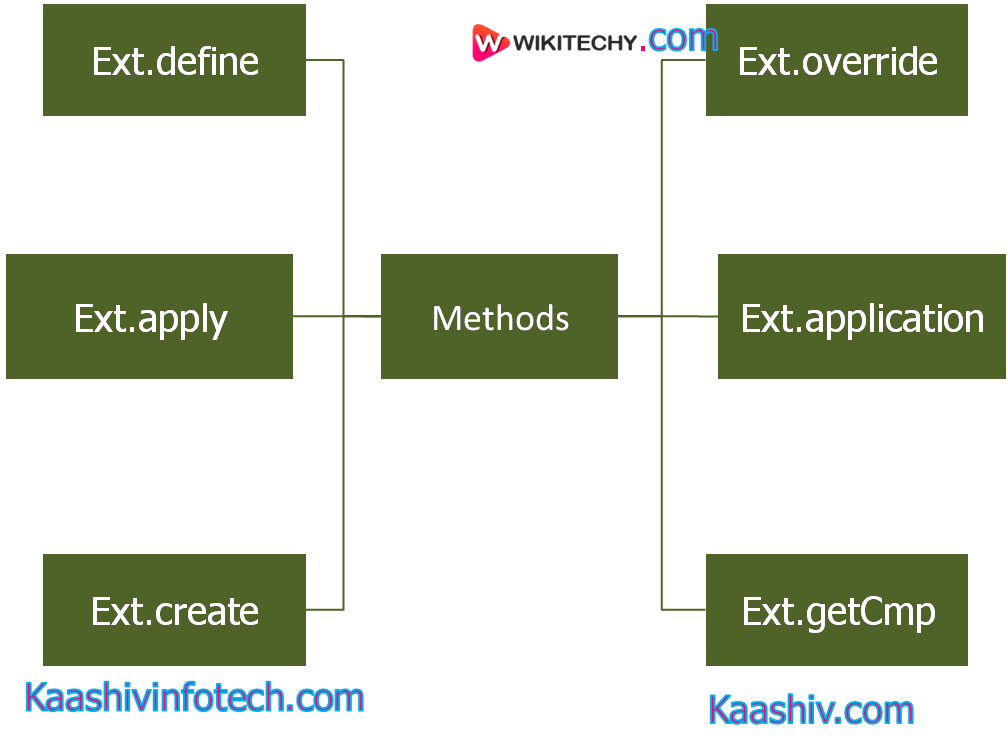
Methods
Defining a Class in Ext JS
To define a class in Ext JS, then you have to use Ext.define ().
Syntax
Ext.define(class name, class member/ properties, callback function);
Here, we have discussed all the values of the syntax.
- Class Name: The class name given by user depending upon the application structure.
- Class Member/Properties: Class member/properties are used to determine the class behaviour.
- Callback Function: A function that is invoked the class is loaded. It is an optional function to use.
Sample Code
Ext.define (employeeApp.view.EmployeeDetailsGrid,
{
extend : 'Ext.grid.GridPanel',
id : 'employeesDetailsGrid',
store : 'EmployeesDetailsGridStore',
renderTo : 'employeesDetailsRenderDiv',
layout : 'fit',
columns : [{
text : 'Employee Name',
dataIndex : 'employee Name'
}, {
text : 'ID',
dataIndex : 'employeeId'
}, {
text : 'Department',
dataIndex: 'department'
}]
});
Creating an object
To create the object in Ext JS like in the other object-oriented programming language (OOPs). Create the object in Ext JS by using two following ways:
Creating an object with the help of Ext.create ()
Ext.create('Ext.Panel', {
renderTo : 'Hello wikitechy’,
height : 50,
width : 50,
title : ‘Kaashiv',
html : 'welcome to wikitechy'
});
Creating object with the help of new keyword
Var employee Object =new employee ();
employeeObject.getEmployeeName ();
Ext JS Inheritance
- In object-oriented programming, the term inheritance can be defined as a technique in a subclass imports the superclass's properties.
Inheritance with the help of Ext.extend method
Ext.define (employeeApp.view.EmployeeDetailsGrid,
// EmployeeDetailsGrid uses the feature of Ext JS Grid Panel
{
Extend: 'Ext.grid.GridPanel',
...
});
Inheritance with the help of Mixins
- The mixins keyword helps us to import the class a properties into class B. Usually, mixins are included in the controller that contains the declaration of various classes such as store, view, etc.
mixins : {
Commons : 'DepartmentApp.utils.DepartmentUtils'
};
Example of Ext JS program
- In the below-written program, code first, we are required to create a simple HTML file named wikitechy.html and also provide the library files in the <head> tag.
Sample Code:
wikitechy.html
<!DOCTYPE html>
<html>
<head>
<link href = "https://cdnjs.cloudflare.com/ajax/libs/extjs/6.0.0/classic/theme-classic/resources/theme-classic-all.css" rel = "stylesheet" />
<script type = "text/javascript" src = "https://cdnjs.cloudflare.com/ajax/libs/extjs/6.0.0/ext-all.js"></script>
<script type = "text/javascript">
Ext.onReady(function() {
Ext.create('Ext.Panel', {
renderTo: 'helloWorldPanel',
height: 500,
width: 500,
title:'Hello Wikitechy',
html: 'Welcome to Wikitechy'
});
});
</script>
</head>
<body>
<div id = "helloWorldPanel" />
</body>
</html>
Read Also
Explanation of program
- Ext.onReady() method: This method is invoked when the Ext Js elements are ready to render.
- Ext.create() method: It is used to build the object. In the above code, we created Ext. Panel object.
- Ext.Panel: It is an inbuilt class used in Ext JS. It also contains the following different properties for different functions.
- Render To: It is responsible for delivering the panel. In the above code, the div id is 'helloWorldPanel'.
- Title: This property provides the title to the panel.
- Height & Weight: These properties are used to give the size of the panel.
- Html: This property helps us to show the Html content in the panel.
Output
- wikitechy.html file in any standard browser and you will get the following output:
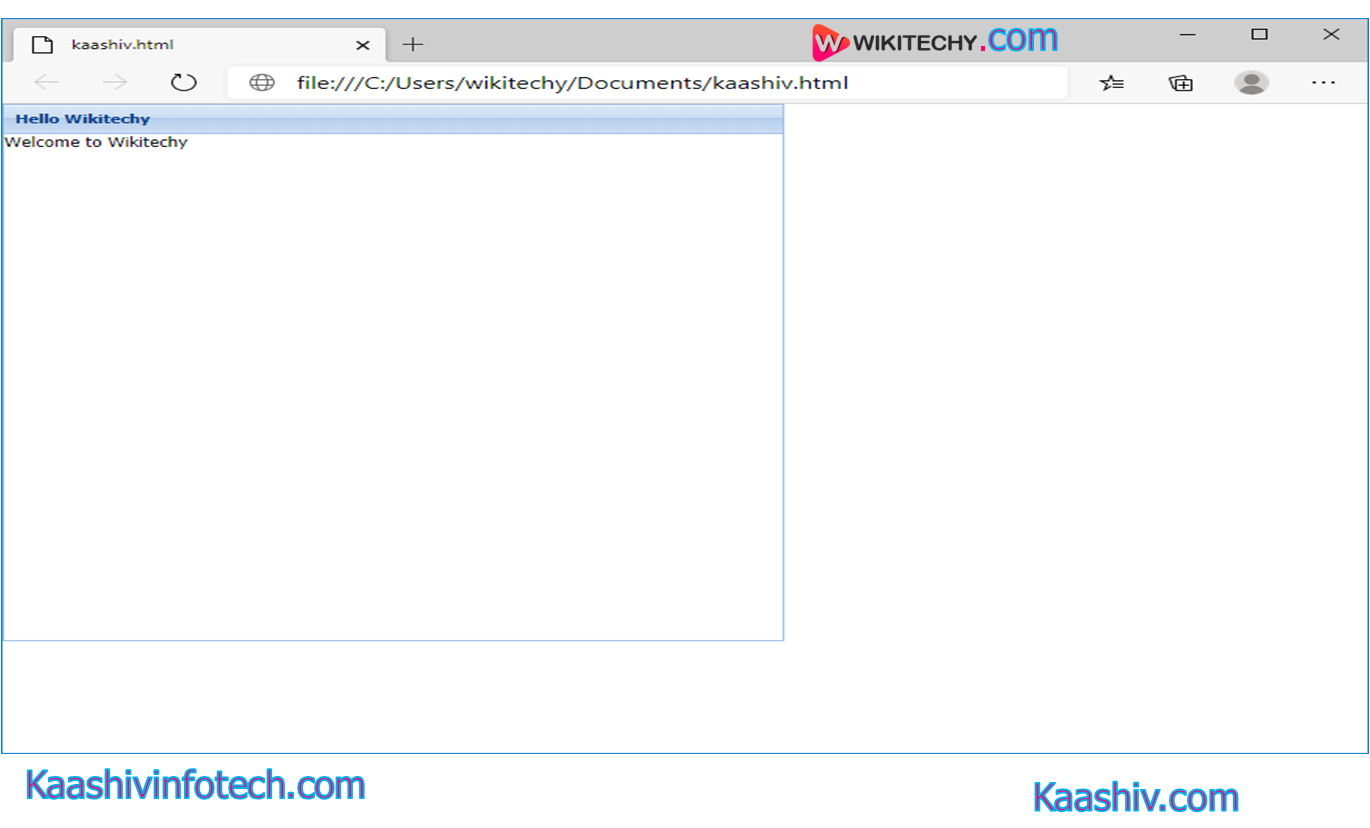
Output
Ext.String Class
Sl. No. | Method | Description |
---|---|---|
1 | Ext.String.htmlEncode | It makes the html value parsable by encoding it. For Example Ext.String.htmlEncode("< p>welcome Ext JS < /p>"); Output - "< p > Hello Ext JS < /p >". /p> |
2 | Ext.String.htmlDecode | It decodes the encoded html value. For Example Ext.String.htmlDecode("< p > welcome Ext JS < /p >"); Output- "< p> welcome Ext JS < /p>" /p> |
3 | Ext.String.trim | This method is used to remove the undesirable space in the string. For Example- Ext.String.trim(' Ext JS '); Output- "Ext JS" |
4 | Ext.String.urlAppend | This method is used to affix the value of the URL string. For Example Ext.String.urlAppend('https://www.google.com' , 'welcome Ext JS'); Output- "https://www.google.com?welcome Ext JS" Ext.String.urlAppend('https://www.google.com?index=1' , 'hello Ext JS'); Output- "https://www.google.com?index=1&welcome Ext JS" |
5 | Ext.String.toggle | This method is used to switch the value between two different values. For Example vartoggleString = 'ASCENDING' toggleString = Ext.String.toggle(p, 'ASCENDING', 'DESCENDING'); Output DESCENDING as toggleString had value ASCENDING. Now again, if you print the same you will get toggleString = "ASCENDING" this time, as it had value 'DESCENDING'. It is same as like ternary operator. toggleString = ((toggleString =='ASCENDING')? 'DESCENDING' : 'ASCENDING' ); |
Ext.is Class
Sl.No. | Method | Description |
---|---|---|
1 | Ext.is.Android | It is used to return true value with the android OS; otherwise it returns false. |
2 | Ext.is.Platforms | This method is used to return the platform used for the current version.
For Example- [Object { property = "platform", regex = RegExp, identity = "Blackberry"}, Object { property = "platform", regex = RegExp, identity = "Mac"}, Object { property = "userAgent", regex = RegExp, identity = "iPad"}, Object { property = "userAgent", regex = RegExp, identity = "iPhone"}, Object { property = "userAgent", regex = RegExp, identity = "Linux"}, Object { property = "platform", regex = RegExp, identity = "iPod"}, Object { property = "platform", regex = RegExp, identity = "Windows"}, Object { property = "platform", regex = RegExp, identity = "Android"} |
3 | Ext.is.Desktop | It returns true if you are using a desktop, otherwise false. |
4 | Ext.is.iPhone | This method returns true if you are using a mobile phone; otherwise, it returns false. |
5 | Ext.is.Phone | This method returns true if you are using an iPhone; otherwise, it returns false. |
6 | Ext.is.iPod | When you use an iPod, then it returns true, otherwise false. |
7 | Ext.is.iPad | If you use an iPad then it returns true, otherwise false. |
8 | Ext.is.Linux | It returns true if you are working with the Linux operating system, otherwise false. |
9 | Ext.is.Mac | It returns true if you are working with the Mac operating system, otherwise false. |
10 | Ext.is.Blackberry | It returns true if you are working with the Blackberry operating system, otherwise false. |
11 | Ext.is.Windows | It returns true if you are working with a Windows operating system, otherwise false. |
Ext.supports Class
Sl.No. | Method | Description |
---|---|---|
1. | Ext.support.Svg | Svg stands for Scalable vector graphics. This method checks whether the device supports the Svg feature or not. It checks for following- doc.createElementNS && !!doc.createElementNS( "http:/" + "/www.w3.org/2000/svg", "svg").createSVGRect. |
2. | Ext.supports.History | This method returns true if the device supports the history of HTML 5; otherwise, it returns false. |
3. | Ext.supports.GeoLocation | This method is used to support the geolocation feature. It also checks for the navigator.geolocation method. |
4. | Ext.supports.Range | Ext.supports. Range method checks the browser, whether it supports the document.createRange method or not. |
5. | Ext.supports.Canvas | This method is used to monitor that the device is suitable for a canvas to draw a method or not. For Example- doc.createElement('canvas').getContext |
Miscellaneous Methods
Sl.No. | Method | Description | ||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
1. | Ext.getVersion() | This method returns the current version of Ext Js version. For Example When you invoke Ext.getVersion(), then it returns an array value such as version, short version, etc. Ext.getVersion().- Returning the current version of Ext JS such as "7.2.0". |
||||||||||||||
2. | Ext.userAgent() | This method provides information about the userAgent browser. The userAgent helps us to describe the Od and browser for server. | ||||||||||||||
3. | Version related function | This method helps us to provide the current browser version. It contains some functions such as Ext.firefoxVersion, Ext.ieVersion, etc. For Example- If you use the Firefox and invoke the method for Internet Explorer, then it returns 0, and when you are using the same browser (IE), then it returns the browser version. |
||||||||||||||
4. | Ext.typeOf() | It is used to display the variable's datatype. For Example- Var p = 10; Var q = 'hello'; Ext.typeOf(p);Output- Number Ext.typeOf(q); Output- String |
||||||||||||||
5. | Browser related functions | These functions are used to return Boolean value according to the current web browser. The methods are Ext.isIE6, Ext.isChrome, Ext.isIE, Ext.isFF06 etc. | ||||||||||||||
6. | Data type related methods | It returns the Boolean value according to the variable. For Example- Var x = ['x', 'yz']; Var y = 'Hello'; Var z = '456'; Var nullvariable; Var deifnedvariable; Function extraFunction() {return true;} |
||||||||||||||
|