TypeScript VS JavaScript - Difference between Typescript and Javascript
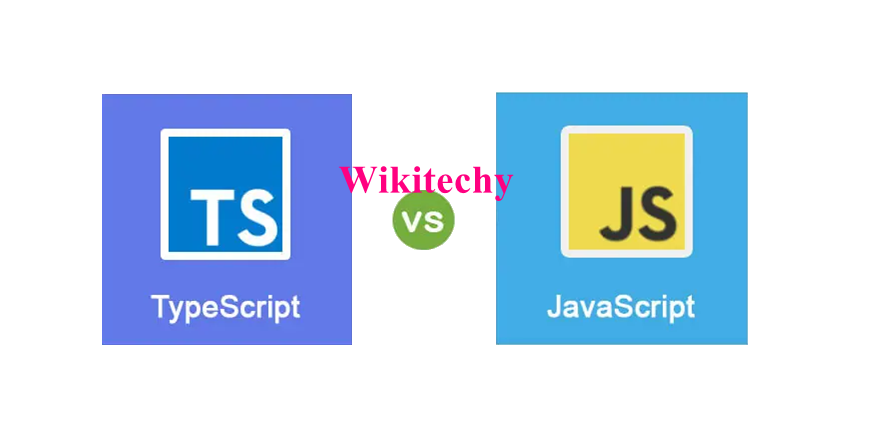
Differences Between TypeScript and JavaScript
- Typescript is an open-source programming language. This means that the Typescript is JavaScript plus other features. We can call it a superset of javascript, which means Typescript is JavaScript plus additional features.
- JavaScript is a lightweight, interpreted programming language. It is a dynamic programming language. JavaScript does not have any multithreading or multiprocessing capabilities. It is used in web development for enhancing HTML pages.
What is Javascript ?
- JavaScript is a versatile and widely-used programming language that plays a fundamental role in web development. It is primarily known for its ability to make web pages interactive and dynamic by allowing developers to create responsive user interfaces.
- Unlike server-side languages, JavaScript runs directly in the user's web browser, enabling client-side scripting. This means that it can manipulate the Document Object Model (DOM) of a webpage, dynamically altering its content and structure in real-time in response to user actions and events.
- JavaScript's versatility extends beyond the web; it is also used in server-side development, mobile app development (using frameworks like React Native), game development (with tools like Phaser), and even for creating desktop applications (using Electron).
- Its asynchronous capabilities, extensive ecosystem of libraries and frameworks, and a vibrant developer community make it a pivotal language in modern software development, powering many of the interactive and feature-rich experiences we encounter on the internet today.
Sample Code
var a = "Wikitechy ";
var b = 1;
console.log(a + b);
Output
Wikitechy 1
What is TypeScript ?
- TypeScript is a statically-typed superset of JavaScript that enhances the capabilities of JavaScript by adding optional static typing and advanced features.
- Developed and maintained by Microsoft, TypeScript helps developers catch errors and improve code quality during development. With TypeScript, you can declare data types for variables, function parameters, and return values, making code more predictable and easier to maintain.
- It also offers features like interfaces, classes, and modules, which promote clean and organized code architecture. TypeScript code is transpiled into plain JavaScript, ensuring compatibility with all modern web browsers and environments.
- Its growing popularity in web and application development stems from its ability to catch errors at compile-time, facilitate code refactoring, and provide better tooling and code navigation support in development environments.
Sample Code
var a:string = "Wikitechy ";
var b:number = 1;
console.log(a + b);
Output
Wikitechy 1
Comparison of TypeScript vs JavaScript (Infographics)
Below are the top 9 comparisons between TypeScript and JavaScript:
1. Type
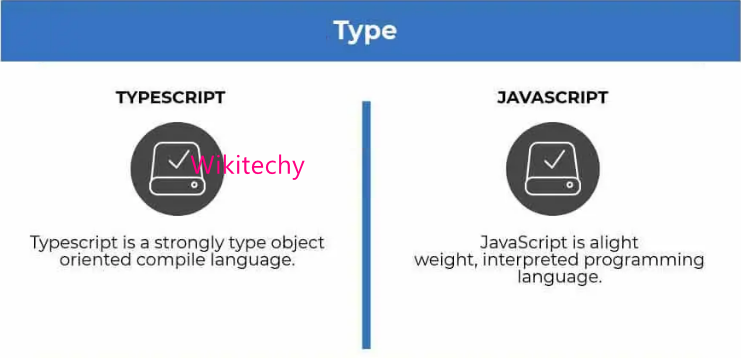
TypeScript is a statically-typed superset of JavaScript. This means that TypeScript is built on top of JavaScript and extends it with features related to static typing. Let's look at the key differences and the concept of types in TypeScript and JavaScript:
JavaScript:
- JavaScript is a dynamically-typed language, meaning you don't need to explicitly specify data types for variables.
- Variables can change their data type during runtime.
- Type-related errors are often discovered at runtime, which can lead to unexpected behavior.
Example in JavaScript:
let x = 10; // x is a number
x = "Hello"; // Now x is a string (no type error at this point)
TypeScript:
- TypeScript introduces static typing, allowing you to specify data types for variables, function parameters, and function return values.
- Type annotations help catch type-related errors at compile-time rather than runtime, which leads to more predictable and robust code.
- TypeScript code is transpiled into plain JavaScript before execution, so it's fully compatible with JavaScript environments.
Example in TypeScript:
let x: number = 10; // x is explicitly defined as a number
x = "Hello"; // Type error: Type '"Hello"' is not assignable to type 'number'
In the TypeScript example, the variable x is explicitly defined as a number, and if you try to assign a string to it, TypeScript will catch the error at compile-time.
2. Design & Developed by
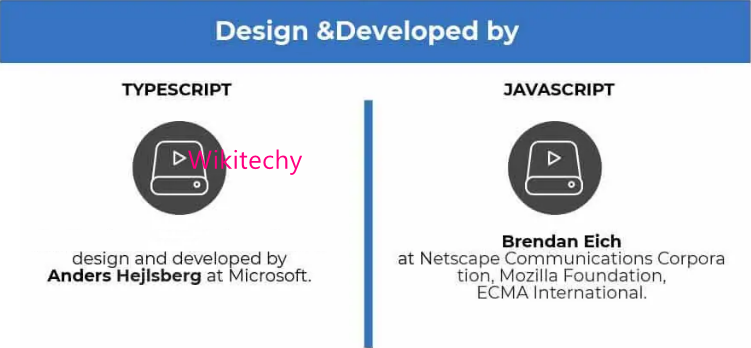
Both TypeScript and JavaScript are open-source programming languages that have been developed and maintained by different entities:
JavaScript:
- JavaScript was created by Brendan Eich while he was working at Netscape Communications Corporation in 1995. It was initially developed for Netscape Navigator web browser to make web pages interactive.
- Over the years, JavaScript has been standardized through the ECMAScript specification, which is managed by the Ecma International organization. Various engines like V8 (used in Google Chrome), SpiderMonkey (used in Firefox), and JavaScriptCore (used in Safari) have implemented the ECMAScript specifications to run JavaScript code efficiently.
TypeScript:
- TypeScript is a superset of JavaScript developed and maintained by Microsoft. It was created by Anders Hejlsberg, the lead architect of C# and Turbo Pascal. TypeScript was first made public in October 2012.
- TypeScript adds static typing and other advanced features to JavaScript, providing developers with a more structured and scalable way to build large applications. TypeScript code is transpiled into JavaScript code before execution.
- TypeScript's development is open-source and is hosted on GitHub. Microsoft, along with contributions from the community, actively maintains and improves the language.
3. Light/Heavy Weight
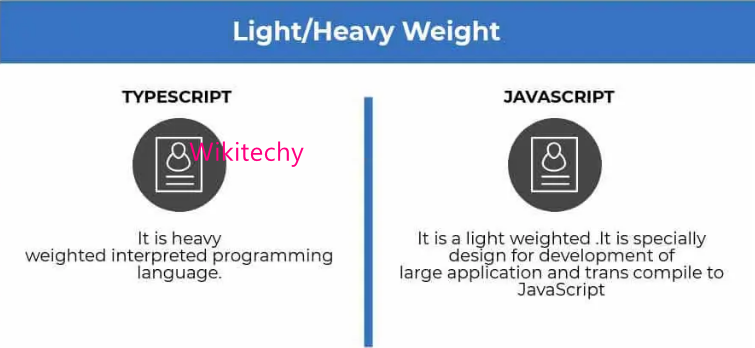
The terms "lightweight" and "heavyweight" are often used to describe the nature of programming languages and technologies. Let's discuss how these terms apply to TypeScript and JavaScript:
JavaScript:
- Lightweight: JavaScript is often considered lightweight due to its simplicity and ease of use. It's a versatile language that can be quickly integrated into web applications for client-side scripting. It has a low barrier to entry, making it accessible for beginners. JavaScript is lightweight in terms of syntax and how quickly developers can start coding with it.
TypeScript:
- Heavyweight: TypeScript can be considered heavyweight in comparison to JavaScript, primarily due to its additional features and complexity. TypeScript adds static typing, interfaces, classes, and other object-oriented programming features on top of JavaScript. These features make TypeScript a more powerful and scalable option for large applications, but they also add complexity to the language. TypeScript's static typing requires developers to define types, making it more robust but potentially more intricate for those new to programming.
4.Client Side/Server Side
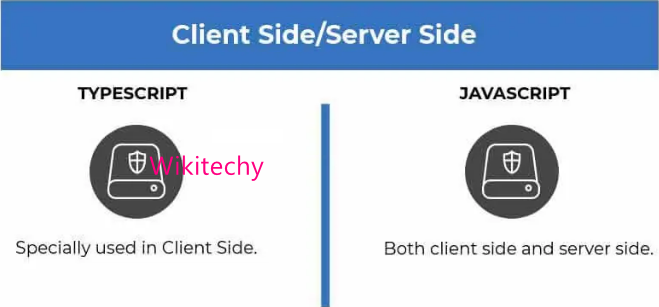
Both TypeScript and JavaScript can be used on both the client-side and the server-side of web development, although they are typically employed in different contexts.
Client-Side:
- JavaScript: JavaScript is primarily used for client-side scripting. It runs in the user's web browser and is responsible for creating interactive and dynamic user interfaces on websites. With JavaScript, developers can manipulate the Document Object Model (DOM) of web pages, handle user events, perform form validations, and make asynchronous requests to servers for data (AJAX). JavaScript is essential for front-end web development.
- TypeScript: TypeScript can also be used for client-side development. TypeScript code is transpiled into JavaScript before execution, making it compatible with web browsers. TypeScript's static typing and advanced features provide developers with a more structured and manageable way to build complex client-side applications, especially in large-scale projects.
Server-Side:
- JavaScript: JavaScript can be used for server-side development through platforms like Node.js. Node.js allows developers to use JavaScript to build scalable and high-performance server-side applications. JavaScript's non-blocking, event-driven I/O model makes it well-suited for building fast and efficient server applications, such as web servers, APIs, and real-time applications.
- TypeScript: TypeScript can also be utilized for server-side development with Node.js. Many developers prefer TypeScript on the server side because of its static typing and improved developer experience. TypeScript allows for better code organization, type safety, and advanced IDE support, making it an attractive choice for building robust server-side applications.
5.File Extension
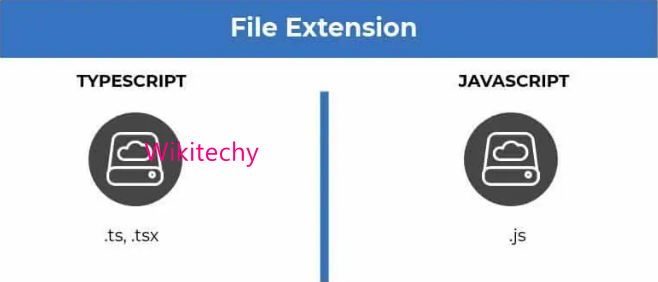
TypeScript and JavaScript have distinct file extensions that are commonly used to identify and differentiate their source code files:
TypeScript:
- The file extension for TypeScript source code files is typically .ts. For example, wikitechy.ts is a TypeScript source code file.
- TypeScript code files use .ts extensions to indicate that they contain TypeScript code, which may include static typing and TypeScript-specific features.
JavaScript:
- The file extension for JavaScript source code files is typically .js. For example, wikitechy.js is a JavaScript source code file.
- JavaScript code files use the .js extension to denote that they contain standard JavaScript code, without the additional features introduced by TypeScript.
6.Syntax
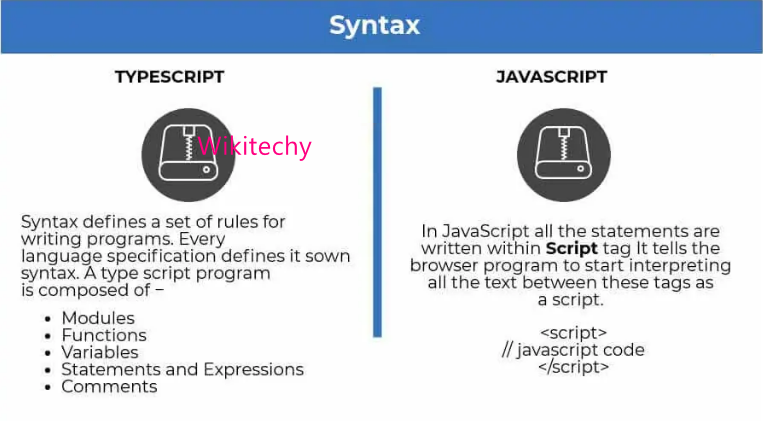
TypeScript and JavaScript share similar syntax since TypeScript is a superset of JavaScript. However, TypeScript introduces additional syntax features related to static typing and modern ECMAScript standards. Here's a brief overview of the syntax for both languages:
JavaScript Syntax: (Variables and Data Types)
- Declare variables using var, let, or const.
- Data types include numbers, strings, booleans, objects, arrays, and functions.
var name = "John";
let age = 30;
const pi = 3.14;
TypeScript Syntax: (Variables and Data Types)
- Declare variable types using : followed by the type.
let name: string = "John";
let age: number = 30;
7. Example
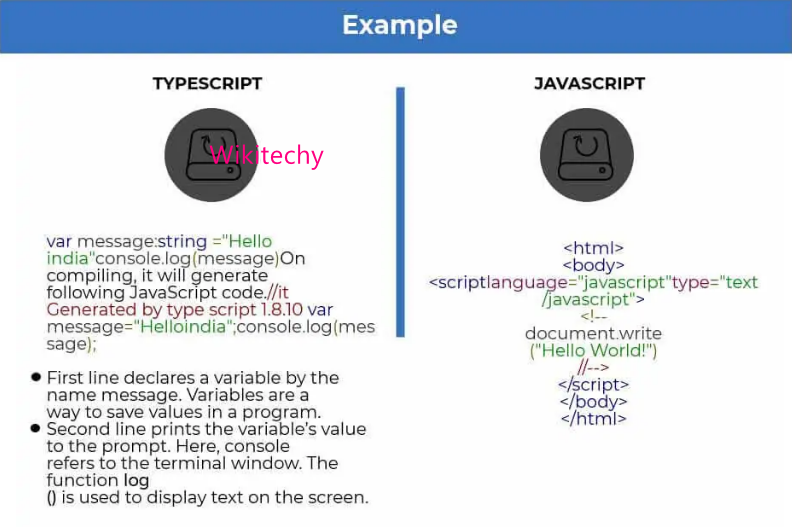
Example: A Simple Function to Calculate the Square of a Number
JavaScript:
function square(number) {
return number * number;
}
const result = square(5);
console.log(result); // Output: 25
In this JavaScript example, we define a function square that calculates the square of a number and then call the function with the argument 5 to get the result.
TypeScript:
function square(number: number): number {
return number * number;
}
const result: number = square(5);
console.log(result); // Output: 25
In this TypeScript example, we define the same square function with type annotations. We explicitly specify that the function takes a parameter of type number and returns a value of type number. The variable result is also explicitly annotated as a number. TypeScript enforces type checking at compile-time.
8. Benefits
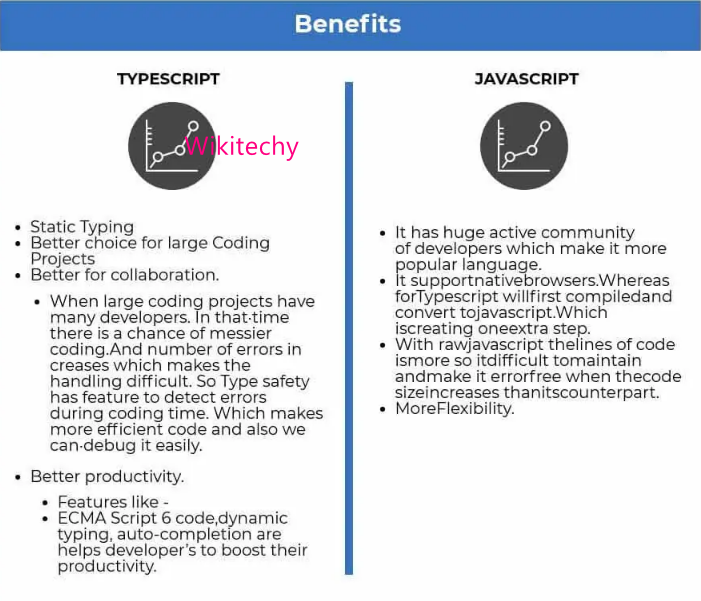
Both TypeScript and JavaScript offer distinct advantages to developers, making them suitable for different scenarios. Let's explore the benefits of both languages:
JavaScript:
1. Ease of Learning and Use:
- JavaScript has a simple and straightforward syntax, making it accessible for beginners. Its flexibility allows developers to experiment and quickly see results.
2. Wide Adoption and Compatibility:
- JavaScript is supported by all major web browsers, making it the de facto language for front-end web development. It also has extensive libraries and frameworks, enhancing its capabilities.
3. Dynamic Typing:
- JavaScript's dynamic typing allows for flexible and agile development. Variables can hold different types of data, which can be an advantage for small projects and rapid prototyping.
4. Asynchronous Programming:
- JavaScript supports asynchronous programming through callbacks, promises, and async/await, enabling non-blocking I/O operations, which is crucial for web applications dealing with concurrent tasks.
TypeScript:
1. Static Typing and Type Safety:
- TypeScript introduces static typing, allowing developers to catch type-related errors during development rather than at runtime. This feature enhances code quality, reduces bugs, and improves maintainability, especially in large-scale projects.
2. Enhanced Tooling and IDE Support:
- TypeScript provides robust tooling and IDE support. Features like autocompletion, type checking, and refactoring tools improve developer productivity and code reliability.
3. Object-Oriented Programming:
- TypeScript supports object-oriented programming principles such as classes, interfaces, and inheritance, making it a preferred choice for developers coming from traditional OOP languages like Java or C#.
4. Modern ECMAScript Features:
- TypeScript supports the latest ECMAScript features even before they are supported in all browsers. This allows developers to write modern, efficient code and transpile it to older JavaScript versions for wider browser compatibility.
5. Code Maintainability:
- TypeScript's static typing and clear interfaces make codebases more maintainable over time. It enhances collaboration among developers and provides better documentation for APIs.
6. Gradual Adoption:
- TypeScript is a superset of JavaScript, meaning existing JavaScript code can be gradually migrated to TypeScript. This allows teams to adopt TypeScript incrementally without rewriting the entire codebase.
9.Preference to chose
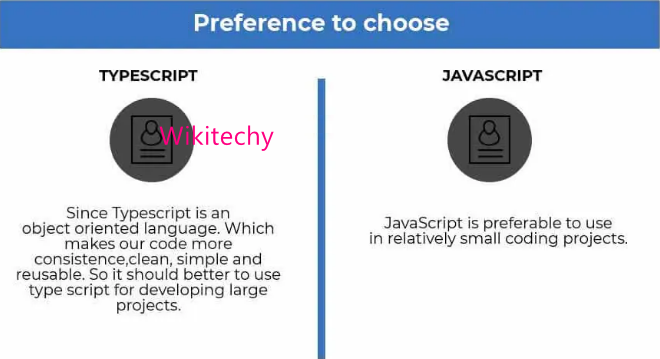
Choosing between TypeScript and JavaScript depends on various factors, including project requirements, team expertise, and specific goals. Here are some scenarios where you might prefer one over the other:
Choose TypeScript If:
1. Large and Complex Projects:
- TypeScript is an excellent choice for large-scale applications where codebase size and complexity are significant concerns. Its static typing helps catch potential issues early, enhancing code quality and maintainability.
2. Team Collaboration:
- If you have a larger team of developers working on the same project, TypeScript's strong typing and clear interfaces can improve collaboration and reduce confusion about data types and function contracts.
3. Codebase Longevity:
- If you are planning for a long-term project and want to ensure the codebase's maintainability and scalability, TypeScript can provide a strong foundation, making it easier to maintain and extend the project over time.
4. Enterprise Applications:
- For enterprise-level applications where robustness, scalability, and collaboration among multiple development teams are crucial, TypeScript's static typing and advanced tooling support are highly beneficial.
5. Transitioning from Other OOP Languages:
- If your team has a background in object-oriented programming languages like Java or C#, TypeScript's class-based object-oriented features can make the transition smoother.
Choose JavaScript If:
1. Quick Prototyping and Small Projects:
- For small-scale projects or when you need to prototype an idea quickly, JavaScript's simplicity and lack of type annotations can allow for rapid development without the overhead of type checking.
2. Front-End Web Development:
- JavaScript is essential for front-end web development. All modern web browsers support JavaScript, making it the default language for building interactive user interfaces and client-side logic.
3. Single-Page Applications (SPAs) and Frameworks:
- JavaScript is often the language of choice for modern front-end frameworks like React, Angular, and Vue.js. If you're building a single-page application, JavaScript is the foundation of these frameworks.
4. Libraries and Ecosystem:
- If your project heavily relies on specific JavaScript libraries or frameworks that are not yet fully compatible with TypeScript, or if you need access to the vast JavaScript ecosystem, sticking with JavaScript might be the practical choice.
5. Learning and Flexibility:
- For beginners or developers who are new to programming, starting with JavaScript provides a gentle introduction to programming concepts due to its forgiving nature. It allows for flexibility and experimentation without strict type constraints.
Ultimately, the decision between TypeScript and JavaScript should be based on a careful evaluation of your project's needs, your team's expertise, and the long-term goals of the application you are developing. Many projects even use both, integrating TypeScript in parts where static typing is crucial while relying on JavaScript for other components.
Comparison Table Between TypeScript vs JavaScript
Following is the comparison table between TypeScript vs JavaScript:
Basis Of Comparison | TypeScript | JavaScript |
---|---|---|
Type | TypeScript compiles code into JavaScript and offers a variety of features such as type annotations, classes, interfaces, and modules that make it easier to create and maintain large-scale applications. |
JavaScript is a lightweight, interpreted programming language. |
Design & Developed by |
Anders Hejlsberg at Microsoft. | Brendan Eich at Netscape Communications Corporation, Mozilla Foundation, ECMA International. |
Light/Heavy Weight | It is heavily weighted. An interpreted programming language. |
It is a light weighted. It is specially designed for the development of large application and trans compile to JavaScript. |
Client Side/ Server Side | Specially used in Client Side. | Both client-side and server-side. |
File Extension | . ts, .tsx | .js |
Syntax | Syntax defines a set of rules for writing programs. Every language specification defines its own syntax. A Typescript program is composed of the following:
|
In JavaScript, all the statements are written within a Script tag. It tells the browser program to start interpreting all the text between these tags as a script<script>// javascript code</script>. |
Preference to Choose |
Since Typescript is an object-oriented language. Which makes our code more consistent, clean, simple, and reusable. So it should be better to use typescript for developing large projects. |
JavaScript is preferable to use in relatively small coding projects. |