Typescript Tutorial - Learn Typescript using Step-by-Step Tutorials
What is TypeScript
- TypeScript is a programming language that extends JavaScript and is designed for building large-scale JavaScript applications.
- It contains all elements of the JavaScript.
- One of the key advantages of TypeScript is that it is a superset of JavaScript, meaning that all JavaScript code is also valid TypeScript code.
- To run TypeScript code, it needs to be compiled into plain JavaScript. The TypeScript compiler takes the TypeScript source code (with a .ts extension) and converts it into JavaScript code (with a .js extension) that can be executed by any browser, host, or operating system.
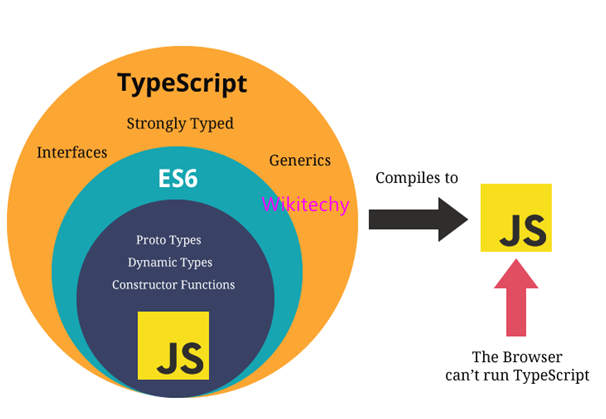
Why use TypeScript ?
- TypeScript supports static typing and strong typing, which helps catch errors and improve code quality.
- It offers object-oriented programming features like classes, interfaces, inheritance, and generics.
- TypeScript is fast, simple, and easy to learn.
- It performs error checking during compilation, highlighting mistakes before running the script.
- TypeScript is compatible with all JavaScript libraries as it is a superset of JavaScript.
- It supports code reusability through inheritance.
- TypeScript enhances app development with tools like autocompletion, type checking, and source documentation.
- It uses .d.ts definition files to provide type information for external JavaScript libraries.
- TypeScript supports the latest JavaScript features, including ECMAScript 2015.
- It offers the benefits of ES6 and improves productivity.
- Developers can save time by using TypeScript.
Features of TypeScript
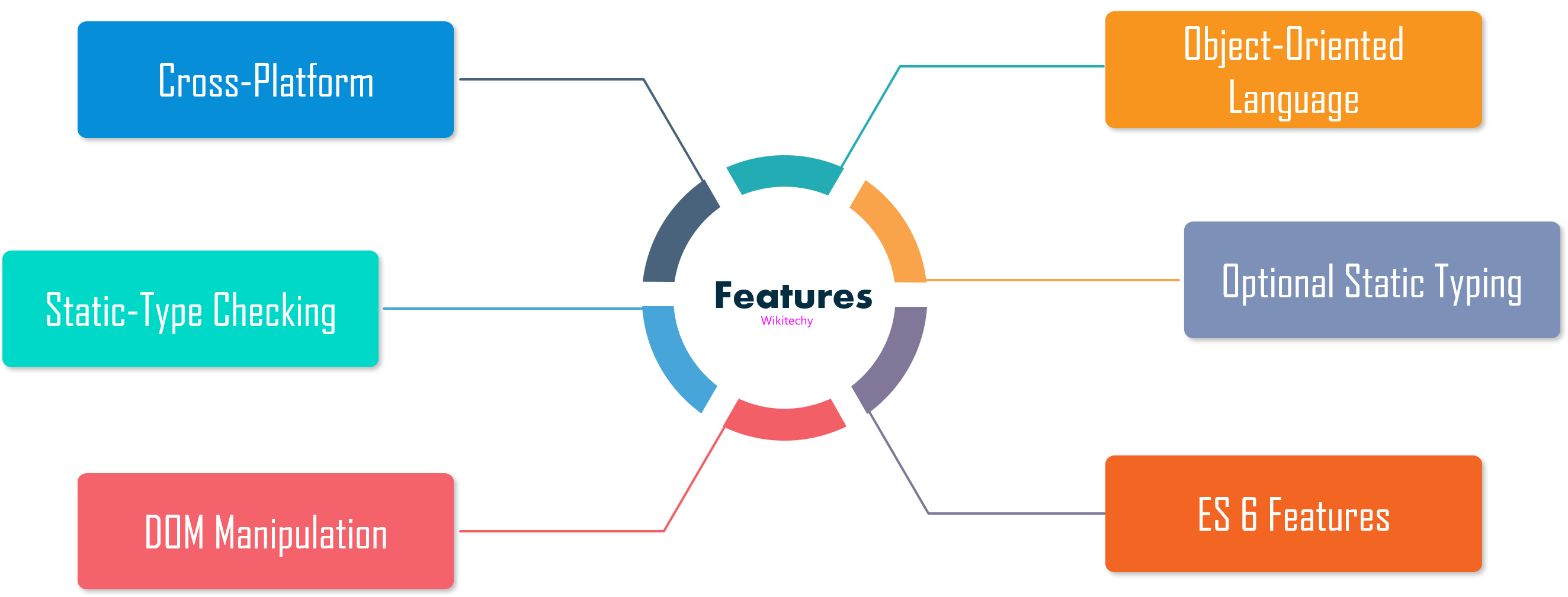
- TypeScript as an Object-Oriented Language:
- TypeScript is a programming language that supports all the features of object-oriented programming.
- It provides features like classes, interfaces, inheritance, and modules, allowing developers to write code for both client-side and server-side development.
- Compatibility with JavaScript Libraries:
- TypeScript is fully compatible with JavaScript libraries.
- Developers can easily use existing JavaScript code within TypeScript projects.
- This means that all JavaScript frameworks, tools, and libraries can be used easily with TypeScript.
- Javascript is Typescript:
- It means the code written in JavaScript with valid .js extension can be converted to TypeScript by changing the extension from .js to .ts and compiled with other TypeScript files.
- Portability:
- TypeScript is portable and can be executed in any environment where JavaScript runs. It is not specific to any particular virtual machine or platform.
- This means that TypeScript code can run on various browsers, devices, operating systems, and server-side environments.
- DOM Manipulation:
- TypeScript can be used to manipulate the Document Object Model (DOM) in a similar way to JavaScript.
- You can add or remove elements, modify attributes, handle events, and perform other DOM operations using TypeScript.
Typescript Vs Javascript
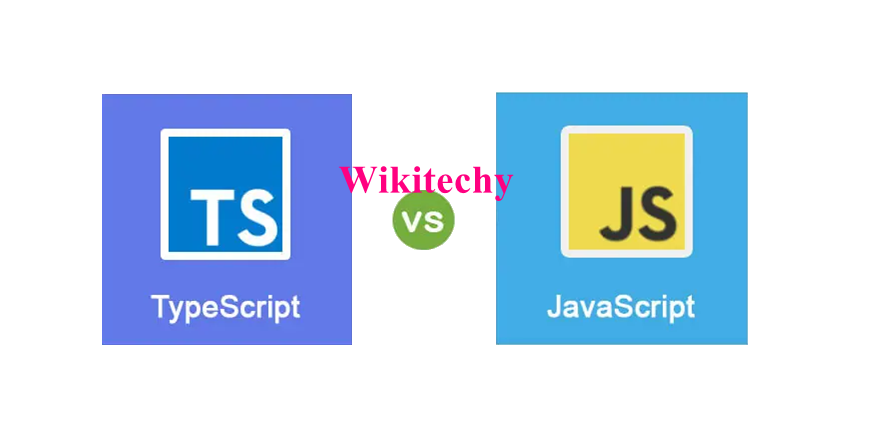
TypeScript Installation
Advantages of TypeScript over JavaScript:
- TypeScript catches errors during compilation, allowing developers to identify and fix them early in the development process. In contrast, JavaScript errors are often only discovered at runtime.
- TypeScript supports strongly typed or static typing, whereas this is not in JavaScript.
- TypeScript runs on any browser or JavaScript engine.
- TypeScript offers excellent tooling support, including IntelliSense. It provides real-time code hints and suggestions, improving productivity and reducing errors during development.
Disadvantages of TypeScript over JavaScript:
- TypeScript takes a long time to compile the code.
- TypeScript does not support abstract classes.
- When running a TypeScript application in the browser, a compilation step is required to convert TypeScript code into JavaScript. This extra step adds complexity compared to directly running JavaScript.
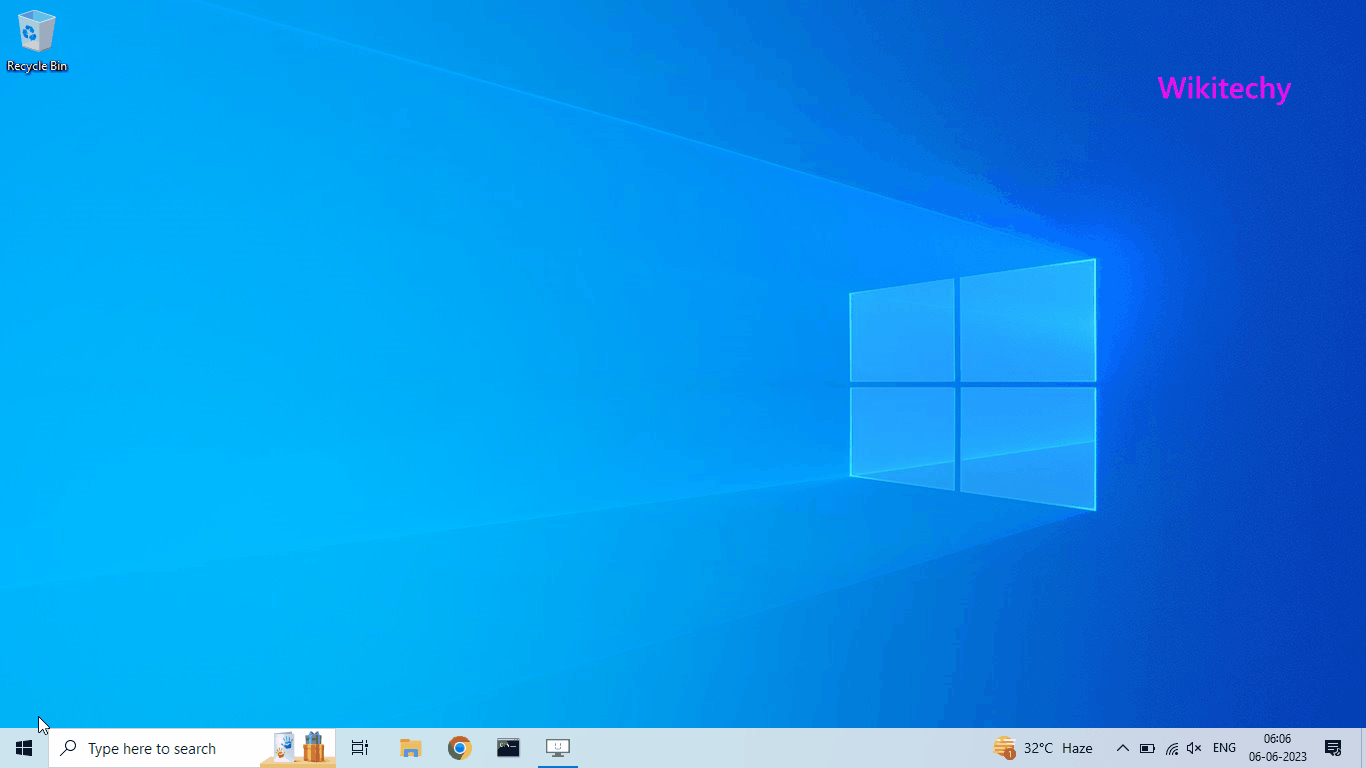
- Open the start menu and click on "Command Prompt."
- To install TypeScript, we need to use NPM (Node Package Manager), which helps us install NodeJS packages on our system.
- In the Command Prompt window, enter the following command to install TypeScript globally:
- This command installs TypeScript on our computer. To check the installation, run the command:
- This command displays the TypeScript version.
- To install a specific version of TypeScript, use the command:
- Replace 'x.x.x' with the desired version number.
- To uninstall TypeScript, use the command:
npm install --global typescript
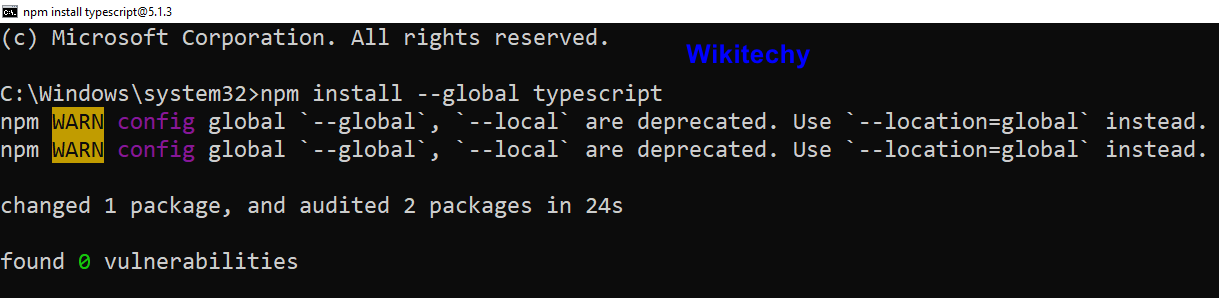
tsc -v
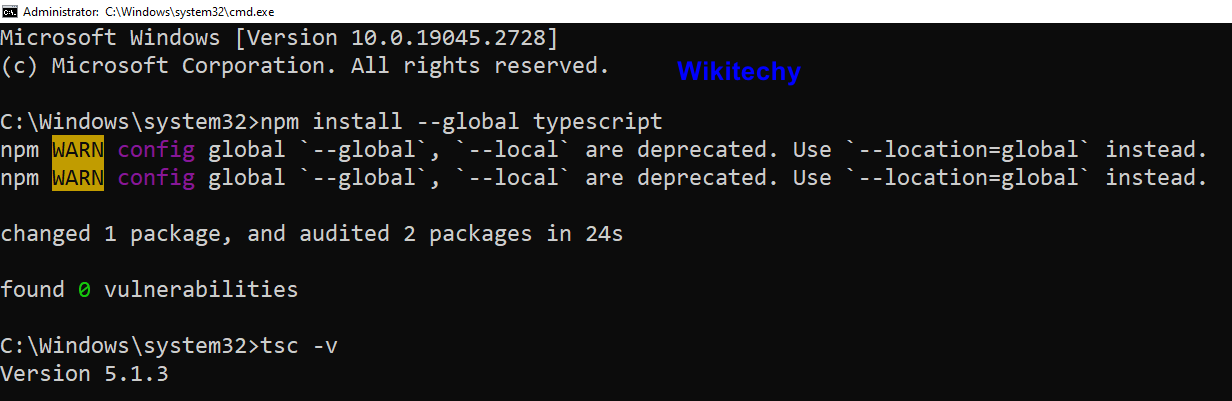
npm install --global typescript@x.x.x
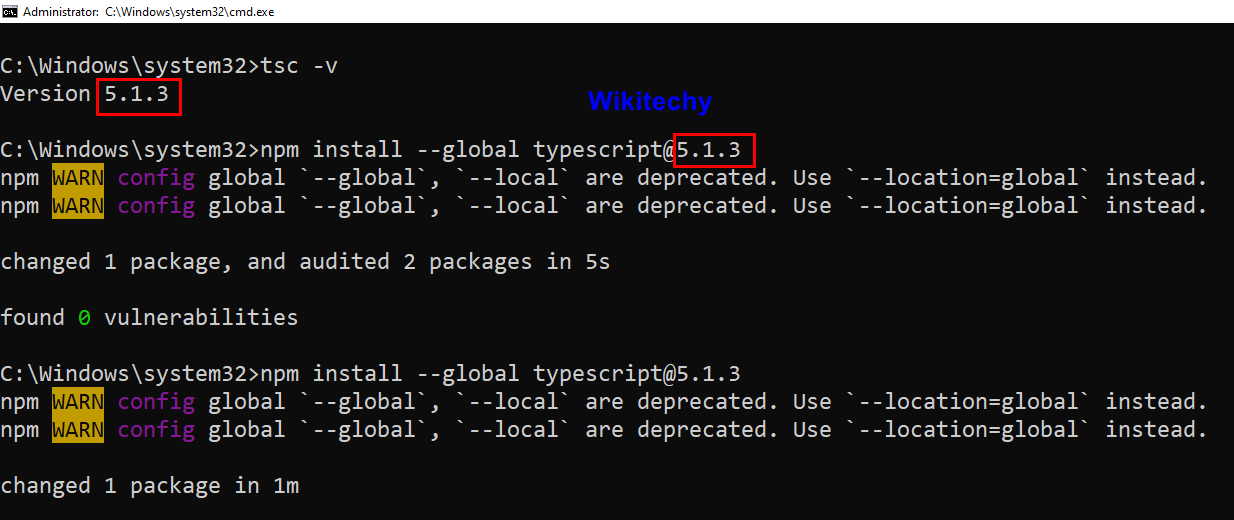
npm uninstall --global typescript
Now, let’s create a simple hello world project through typescript
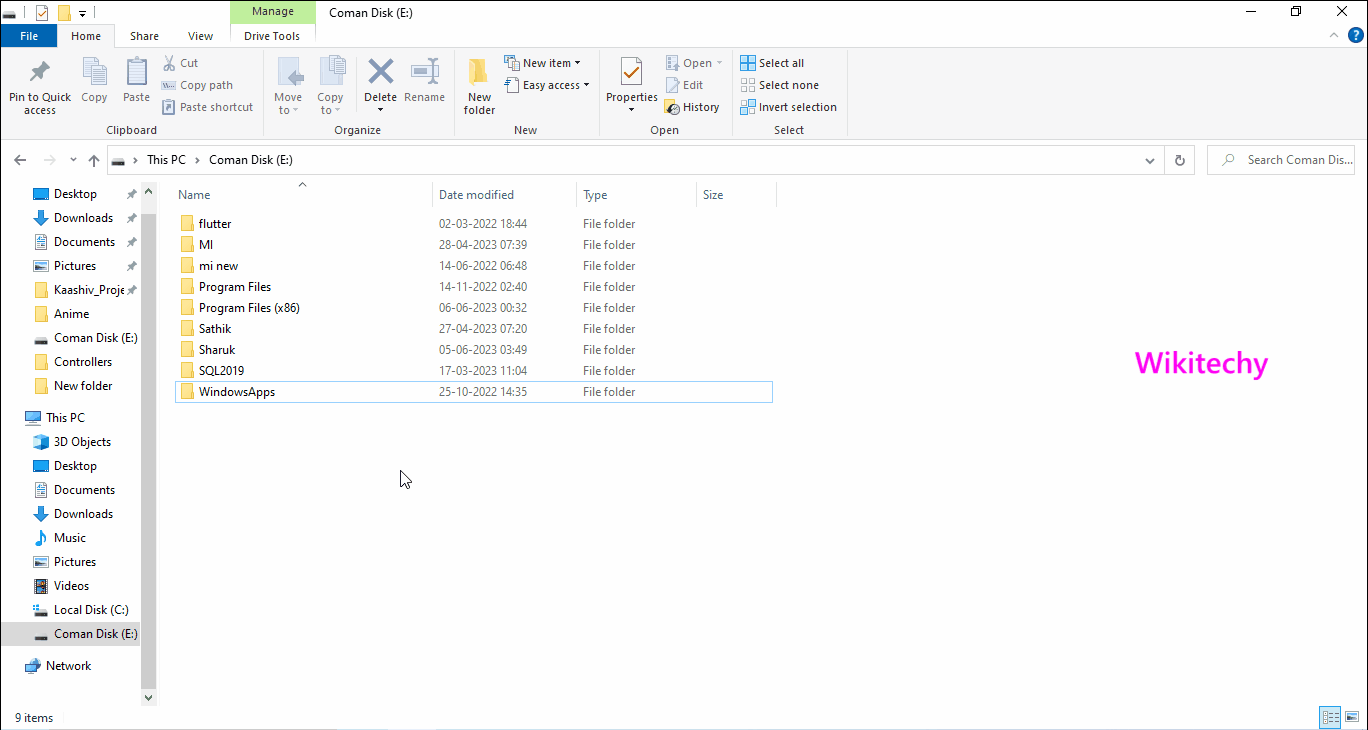
Step 1
- Create a folder on your computer and name it whatever you like. Inside that folder, create a file called "main.ts". This will be our TypeScript file where we'll write our code.
Step 2
- Open the command prompt and navigate to the folder path where you created the folder and the "main.ts" file.
Step 3
- In the command prompt, run the following command to initialize the TypeScript compiler and create a configuration file:
tsc --init
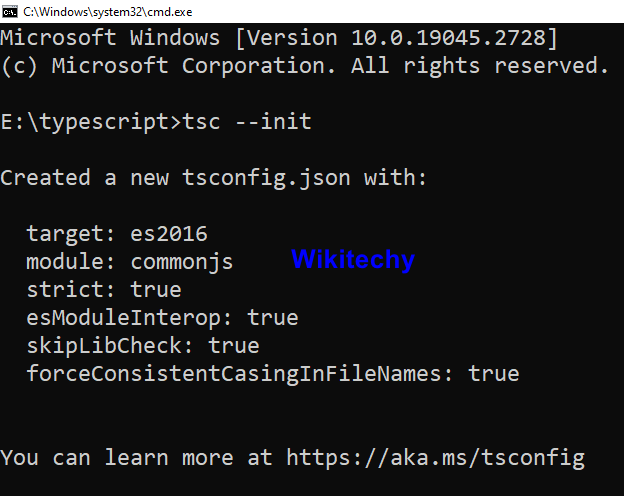
- This command will generate a configuration file called "tsconfig.json" which contains compiler settings and options.
Step 4
- Open the "main.ts" file and add the following code :
main.ts
/** Hello world program using Typescript */
let helloTypescript: string;
helloTypescript = "Typescript is successfully installed"+ " onto this system. Wikitechy";
console.log(helloTypescript);
- Save the changes to the "main.ts" file.
Step 5
- In the command prompt, run the TypeScript compiler using the following command:
tsc main.ts
- This will compile the TypeScript code into JavaScript and generate a "main.js" file.
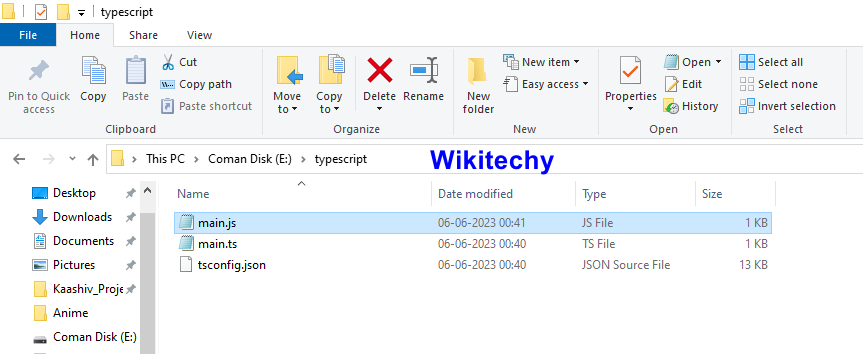
Step 6
- Run the JavaScript file using Node.js by running the following command:
node main.js
Output
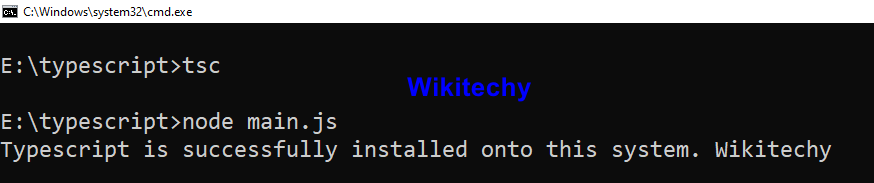
var Keyword
- In TypeScript, the var keyword is used to declare variables with function scope or global scope. However, it's generally recommended to use let and const instead of var in TypeScript, as let and const provide better scoping rules and help catch potential issues at compile-time.
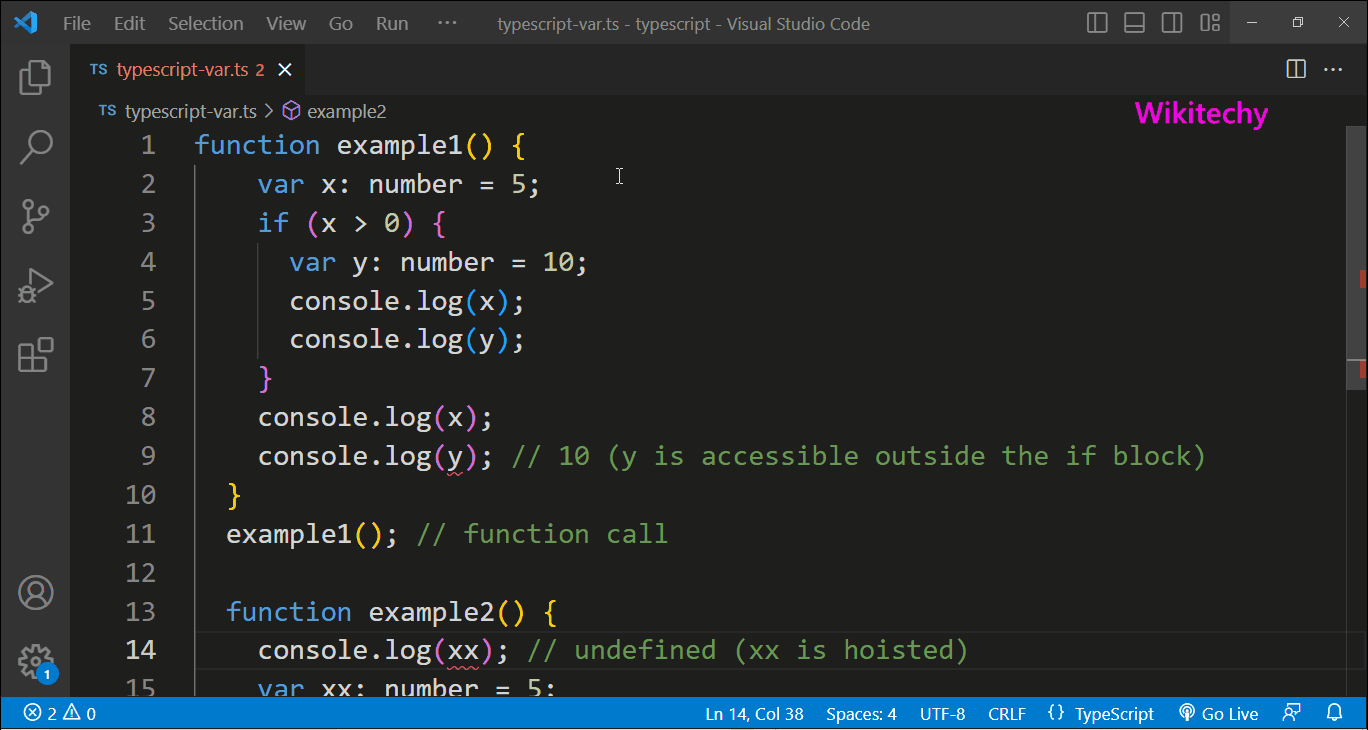
Example 1
function example() {
var x: number = 5;
if (x > 0) {
var y: number = 10;
console.log(x);
console.log(y);
}
console.log(x);
console.log(y); // 10 (y is accessible outside the if block)
}
Example1(); // function call
In the above code, x and y are declared with the var keyword. They are function-scoped variables, meaning they are accessible within the entire function in which they are declared. The value of y defined within the if block is accessible outside the block as well.
However, using var can lead to issues known as "hoisting" and "leaking" due to its less strict scoping rules.
Output
5
10
5
10
Example 2
function example() {
console.log(x); // undefined (x is hoisted)
var x: number = 5;
console.log(x);
}
example2(); // function call
In the above code, x is hoisted, which means that the variable declaration is moved to the top of the function scope. However, the initialization (x = 5) is not hoisted, so accessing x before it is assigned a value results in undefined.
To avoid such issues, it's recommended to use let and const instead of var in TypeScript. The let and const keywords have block scope, which makes the code more predictable and helps catch potential errors at compile-time.
Output
undefined
5
let Keyword
- In TypeScript, the let keyword is used to declare block-scoped variables. It is similar to the let keyword in JavaScript. Variables declared with let can be reassigned within their scope.
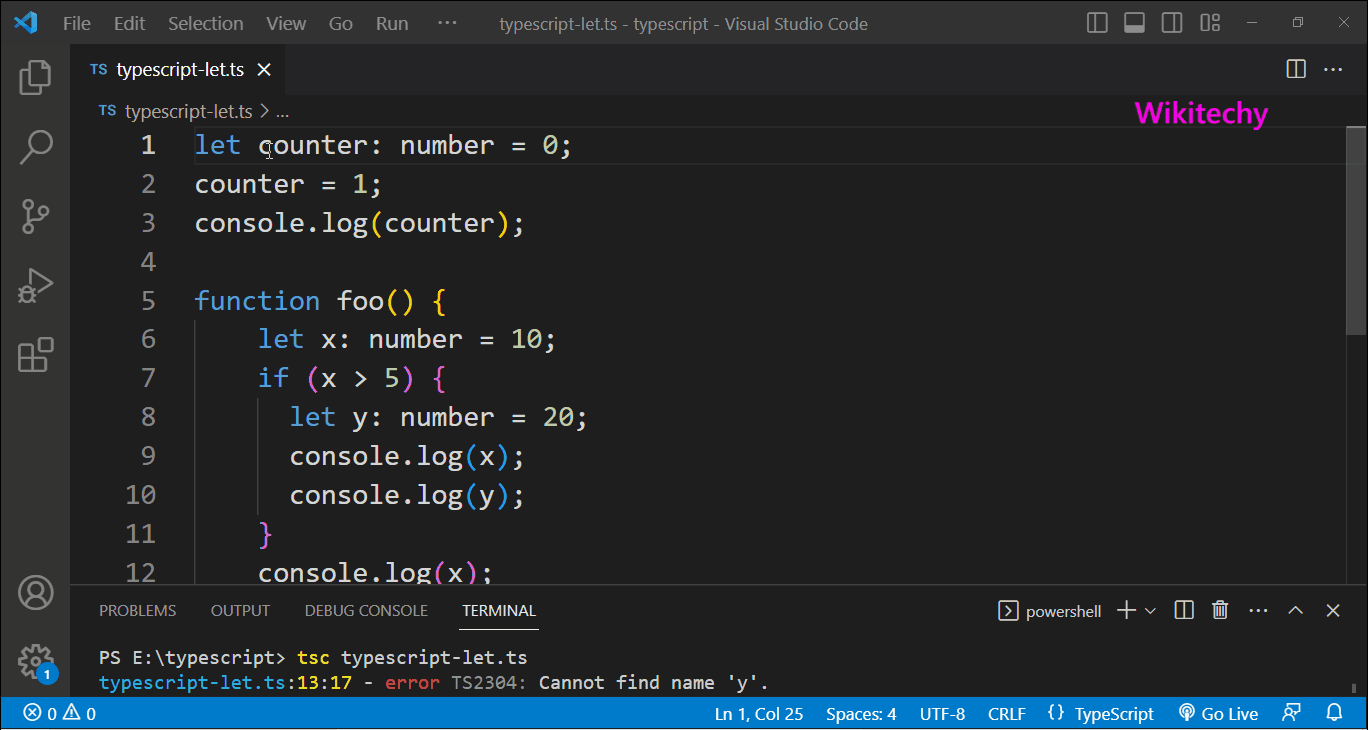
Example 1
let counter: number = 0;
counter = 1;
console.log(counter);
In the above code, counter is declared as a variable of type number using the let keyword. It is initialized with the value 0 and then reassigned to 1. Since let allows variable reassignment, this code is valid.
The scope of a variable declared with let is limited to the block in which it is defined.
Output
1
Example 2
function foo() {
let x: number = 10;
if (x > 5) {
let y: number = 20;
console.log(x);
console.log(y);
}
console.log(x);
console.log(y);
}
foo(); //function call
In the above code, x is defined within the function foo() and is accessible throughout the function. However, y is defined within the if block and is only accessible within that block.
Using let for variable declarations is a good practice because it helps prevent accidental variable hoisting and makes code more predictable. It is recommended to use let when you need to declare variables that can be reassigned within a specific scope.
Output
typescript-let.ts:13:17 - error TS2304: Cannot find name 'y'.
13 console.log(y);
Found 1 error in typescript-let.ts:13
Const Keyword
- In TypeScript, the const keyword is used to declare a constant variable. It is similar to the const keyword in JavaScript and serves the purpose of declaring a variable whose value cannot be reassigned once it has been initialized.
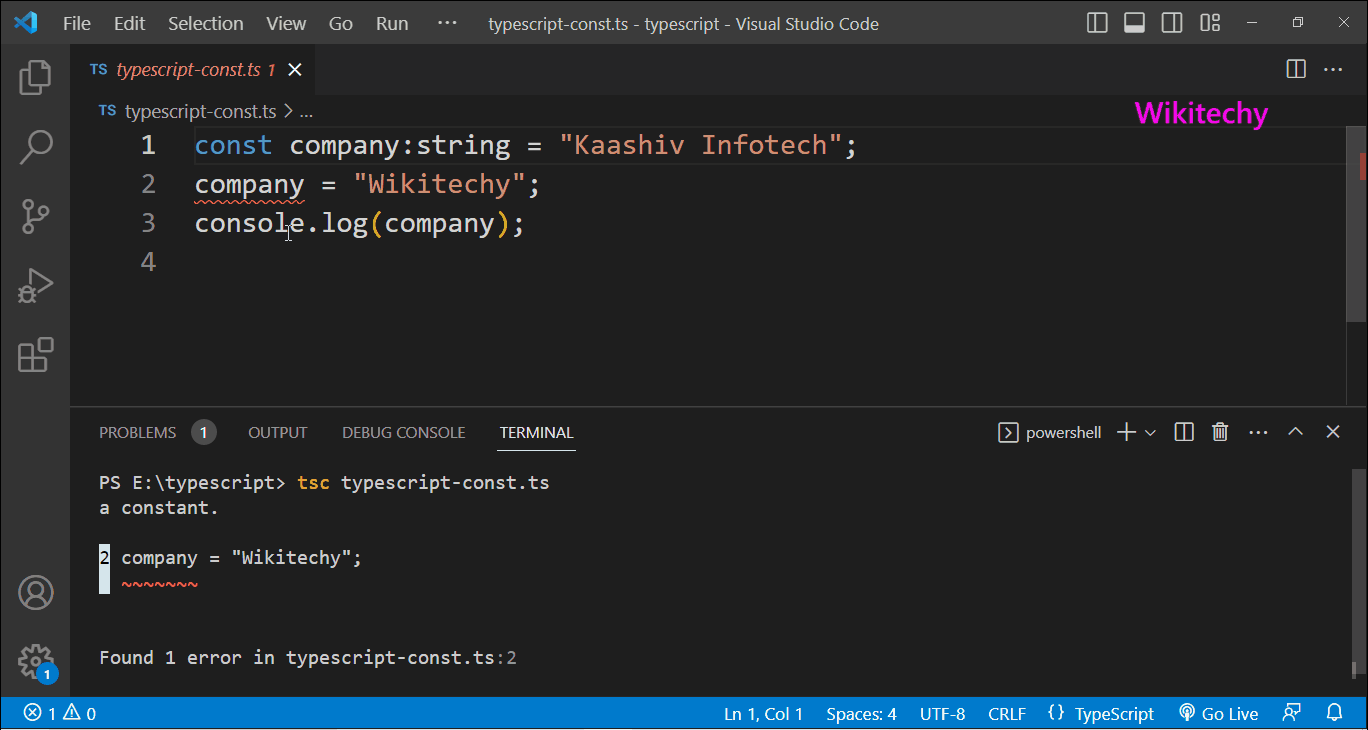
Example 1
const company:string = " Kaashiv Infotech ";
console.log(company);
In the above code, company is declared as a constant variable with the type string, and its initial value is set to Kaashiv Infotech. Since it is declared as a constant, any attempt to reassign a new value to company will result in a compilation error.
Output
Kaashiv Infotech
Example 2
const company:string = "Kaashiv Infotech";
company = "Wikitechy";
console.log(company);
Using const for variables provides benefits such as improved code readability and preventing accidental reassignment, which can help catch potential bugs at compile-time. It is recommended to use const whenever possible for variables that are meant to be constants throughout the program.
Output
typescript-const.ts:2:1 - error TS2588: Cannot assign to 'company' because it is a constant.
2 company = "Wikitechy";
Found 1 error in typescript-const.ts:2
Data Types
Number | number | It is used to represent both Integer as well as Floating-Point numbers |
Boolean | boolean | Represents true and false |
String | string | It is used to represent a sequence of characters |
Void | void | Generally used on function return-types |
Null | null | It is used when an object does not have any value |
Undefined | undefined | Denotes value given to uninitialized variable |
Any | any | If variable is declared with any data-type then any type of value can be assigned to that variable |
Declare Type Script Variables
To declare variables in TypeScript, you can use the following syntax:
let variableName: dataType = initialValue;
Here's an example of declaring variables with different data types:
let a: null = null;
let b: number = 123;
let c: number = 123.456;
let d: string = ‘Wikitechy’;
let e: undefined = undefined;
let f: boolean = true;
let g: number = 0b111001; // Binary
let h: number = 0o436; // Octal
let i: number = 0xadf0d; // Hexa-Decimal
- You can replace the variable names, data types, and initial values as per your requirements.
Declare Type Script any Variable
- In TypeScript, the any type is used to represent a variable that can hold values of any type. Here's an example of declaring variables with the any type:
let a: any = null;
let b: any = 123;
let c: any = 123.456;
let d: any = 'Wikitechy';
let e: any = undefined;
let f: any = true;
Type Script String
- In TypeScript, the string type represents textual data and is used to declare variables or function parameters that store string values. Here are a few examples of working with strings in TypeScript:
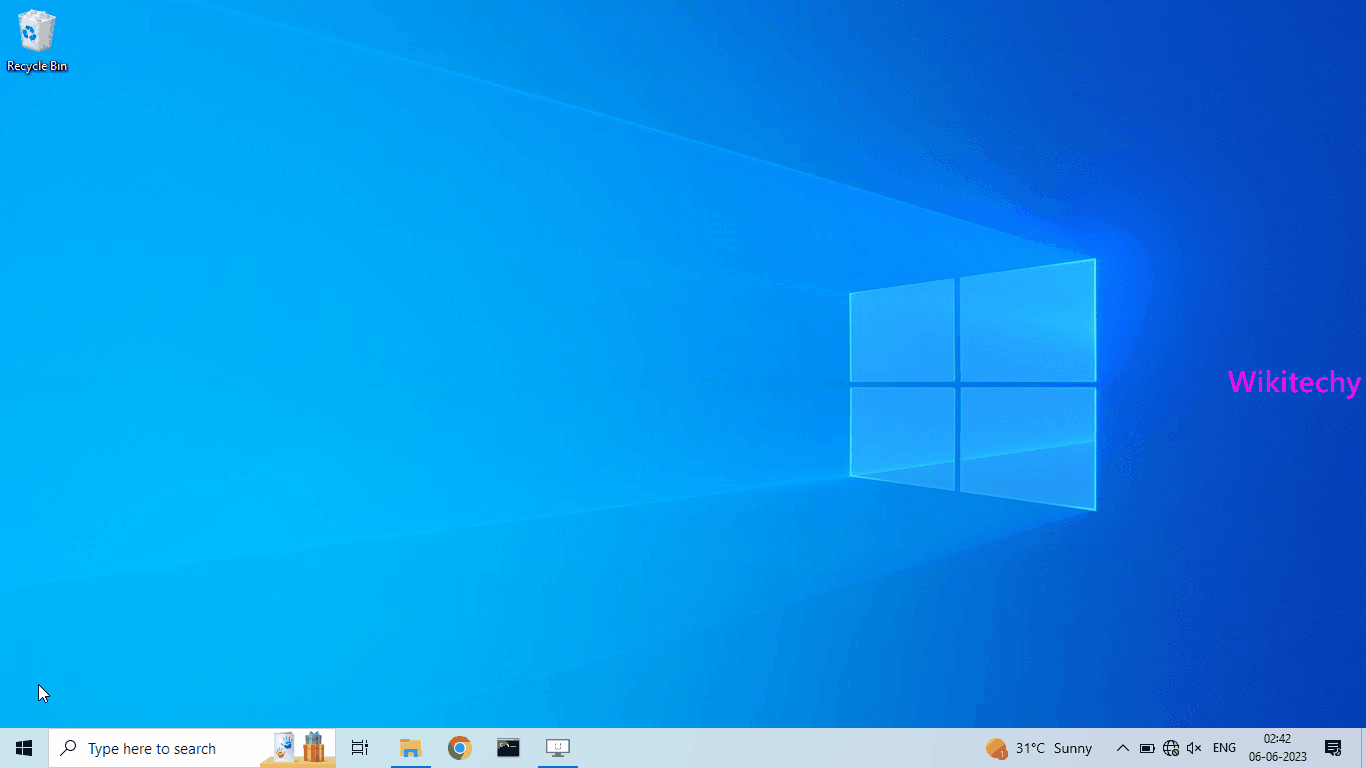
Example 1
var str:string = "wikitechy";
console.log(str.toUpperCase());
Output
WIKITECHY
Example 2
var str1:string = "Kaashiv";
var str2:string = "Infotech";
console.log(str1 + " " + str2);
Output
Kaashiv Infotech
Type Script Numbers
- In TypeScript, the number type is used to represent numeric values. It includes both integer and floating-point numbers. Here are some examples of working with numbers in TypeScript:
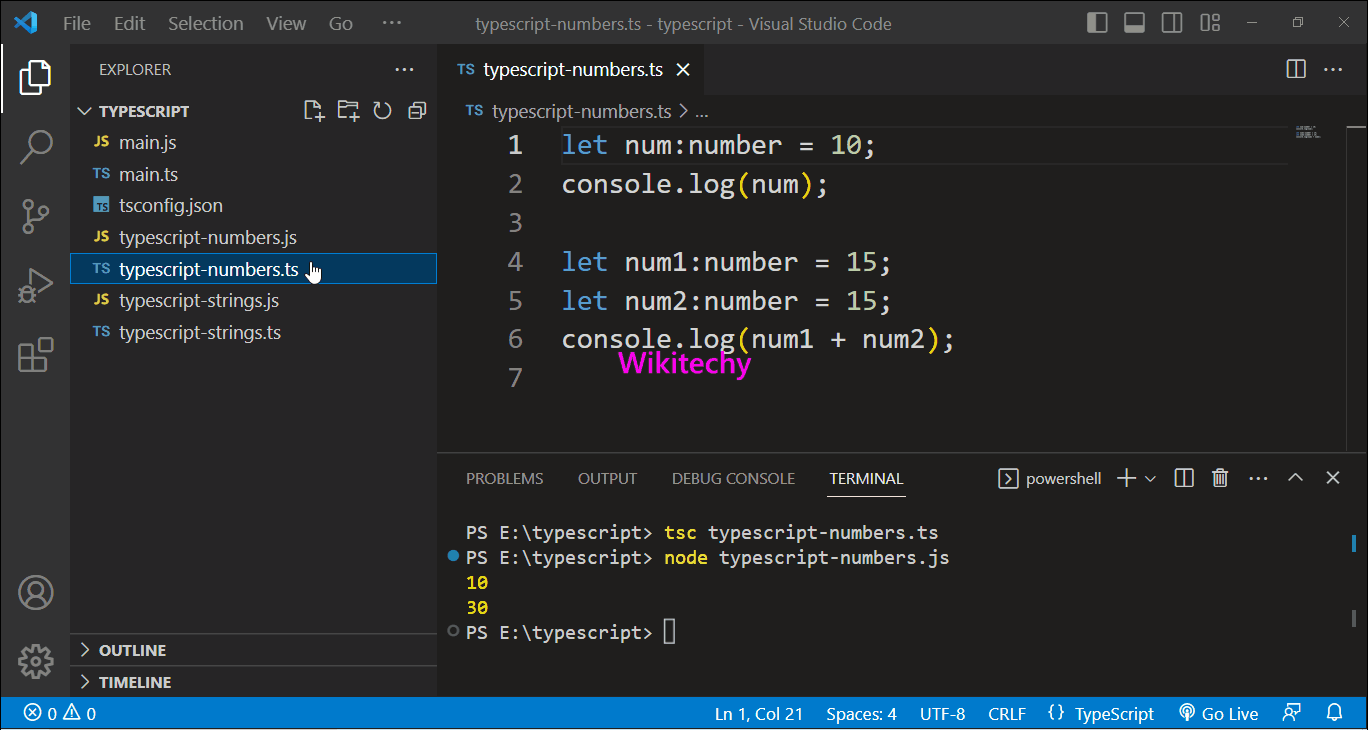
Example 1
let num:number = 10;
console.log(num);
Output
10
Example 2
let num1:number = 15;
let num2:number = 15;
console.log(num1 + num2);
Output
30
Type Script Boolean
- In TypeScript, the boolean type represents logical values that can be either true or false. It is used to declare variables or function parameters that store boolean values.
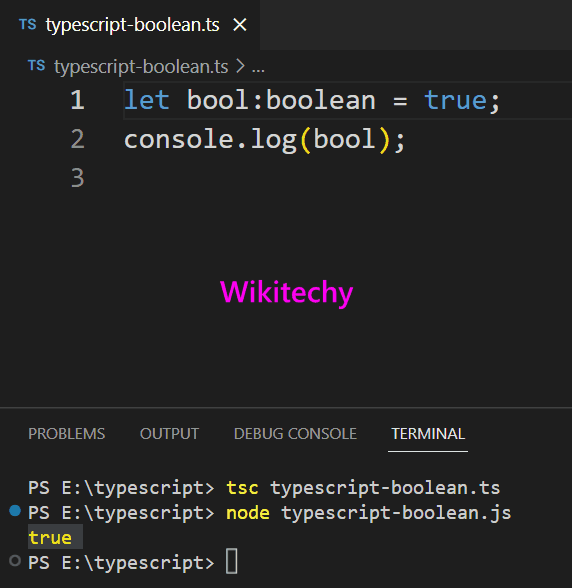
Example 1
let bool:boolean = true;
console.log(bool);
Output
true
Type Script Object
- In TypeScript, the object type is a general type that represents any non-primitive value. It is used to declare variables or function parameters that can hold values of any type.
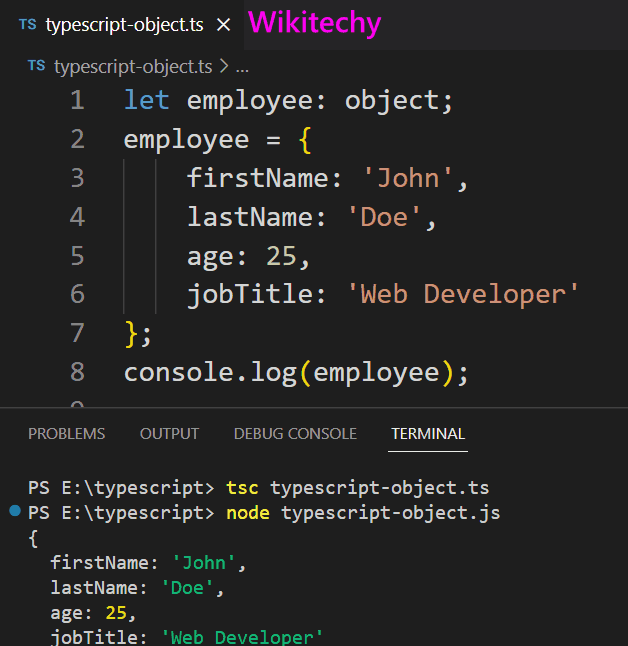
Example 1
let employee: object;
employee = {
firstName: 'John',
lastName: 'Doe',
age: 25,
jobTitle: 'Web Developer'
};
console.log(employee);
Output
{
firstName: 'John',
lastName: 'Doe',
age: 25,
jobTitle: 'Web Developer'
}
Example 2
let obj = {
"name":"obj",
"age":20
}
console.log(obj.name);
console.log(obj.age);
Output
obj
20
Type Script Interface
- In TypeScript, an interface is a way to define a contract or a structure for an object. It specifies the properties and methods that an object must have in order to be considered as implementing that interface.
- Interfaces provide a powerful mechanism for enforcing type checking and enabling code reuse.
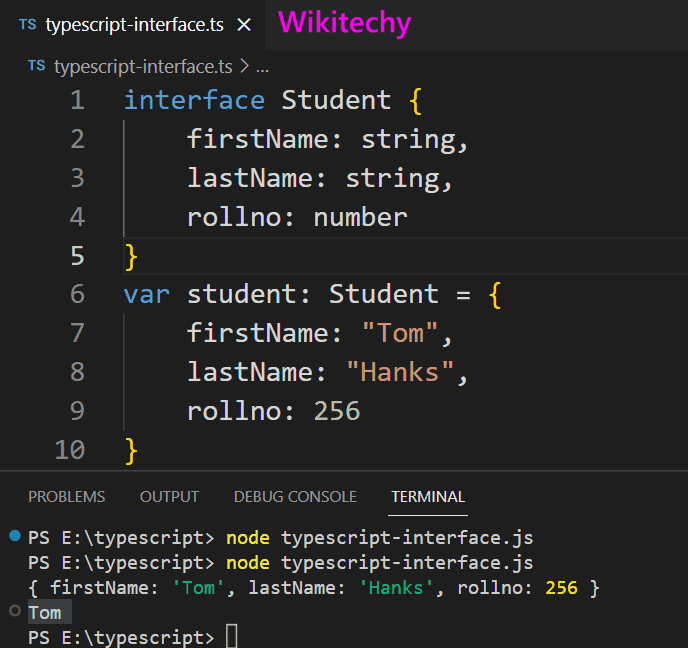
Example 1
interface Student {
firstName: string,
lastName: string,
rollno: number
}
var student: Student = {
firstName: "Tom",
lastName: "Hanks",
rollno: 256
}
console.log(student);
console.log(student.firstName);
Output
{
firstName: "Tom",
lastName: "Hanks",
rollno: 256
}
Tom
Typescript Class
- In TypeScript, a class is a blueprint for creating objects with specific properties and methods. It encapsulates data and behavior into a single unit. Here's how you can define and use a class in TypeScript:
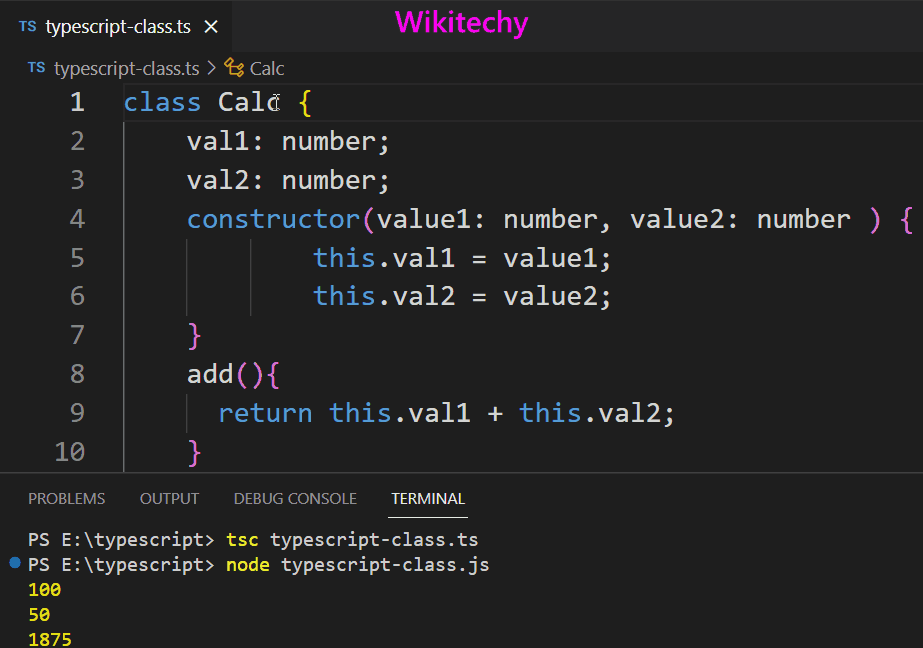
Example 1
class Calc {
val1: number;
val2: number;
constructor(value1: number, value2: number ) {
this.val1 = value1;
this.val2 = value2;
}
add(){
return this.val1 + this.val2;
}
sub(){
return this.val1 - this.val2;
}
mul(){
return this.val1 * this.val2;
}
div(){
return this.val1 / this.val2;
}
}
let calc = new Calc(75,25);
console.log(calc.add());
console.log(calc.sub());
console.log(calc.mul());
console.log(calc.div());
Output
100
50
1875
3
Typescript any
- Any is a data type in TypeScript. Any type is used when we deal with third-party programs and expect any variable but we don’t know the exact type of variable. Any data type is used because it helps in opt-in and opt-out of type checking during compilation.
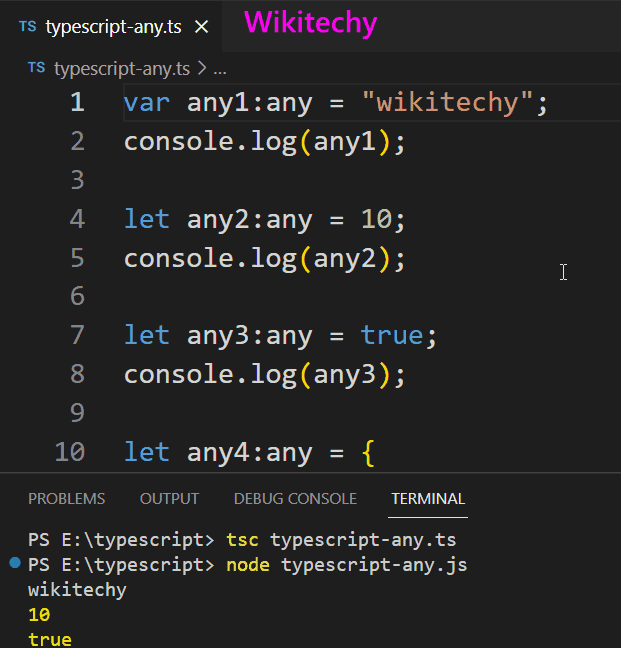
Example 1
var any1:any = "wikitechy";
console.log(any1);
Output
wikitechy
Example 2
let any2:any = 10;
console.log(any2);
Output
10
Example 3
let any3:any = true;
console.log(any3);
Output
true
Example 4
let any4:any = {
"name":"venkat",
"age":20
}
console.log(any4.name);
console.log(any4.age);
Output
venkat
20
Typescript Switch Statement
- The switch statement in TypeScript is used to check the value of an expression against multiple cases. It allows you to specify different blocks of code to be executed based on the matched case.
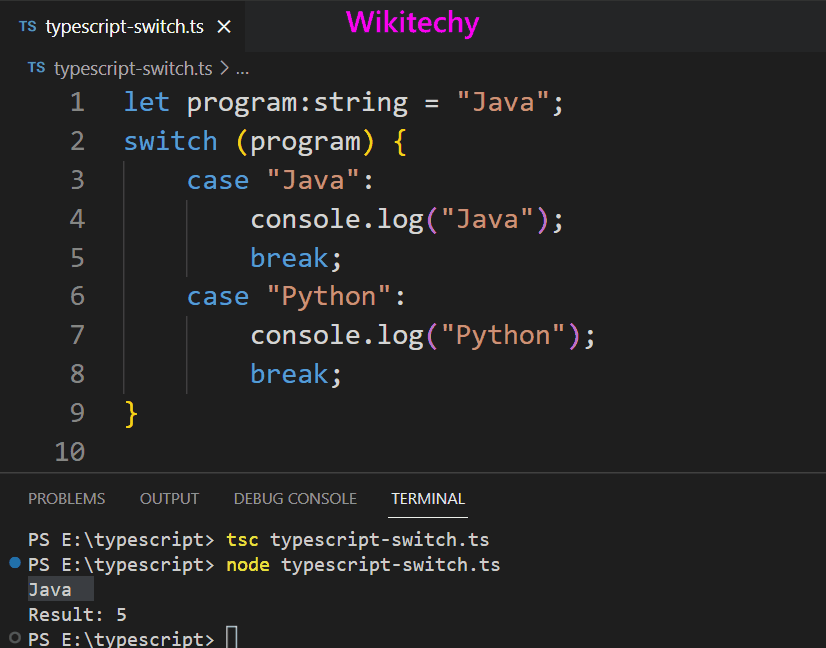
Example 1
let program = "Java";
switch (program) {
case "Java":
console.log("Java");
break;
case "Python":
console.log("Result: 5");
break;
}
Output
Java
Example 2
let numb1 = 10, numb2 = 5;
switch (numb1-numb2) {
case 0:
console.log("Result: 0");
break;
case 5:
console.log("Result: 5");
break;
case 10:
console.log("Result: 10");
break;
}
Output
Result: 5
Typescript if else Statement
- In TypeScript, the "if...else" statement is a control flow statement used to conditionally execute different blocks of code based on a specific condition.
- It evaluates a condition and, if the condition is true, executes the code block associated with the "if" statement. If the condition is false, it executes the code block associated with the "else" statement (if provided).
- This statement helps in controlling the flow of the program based on certain conditions, enabling different actions to be taken accordingly.
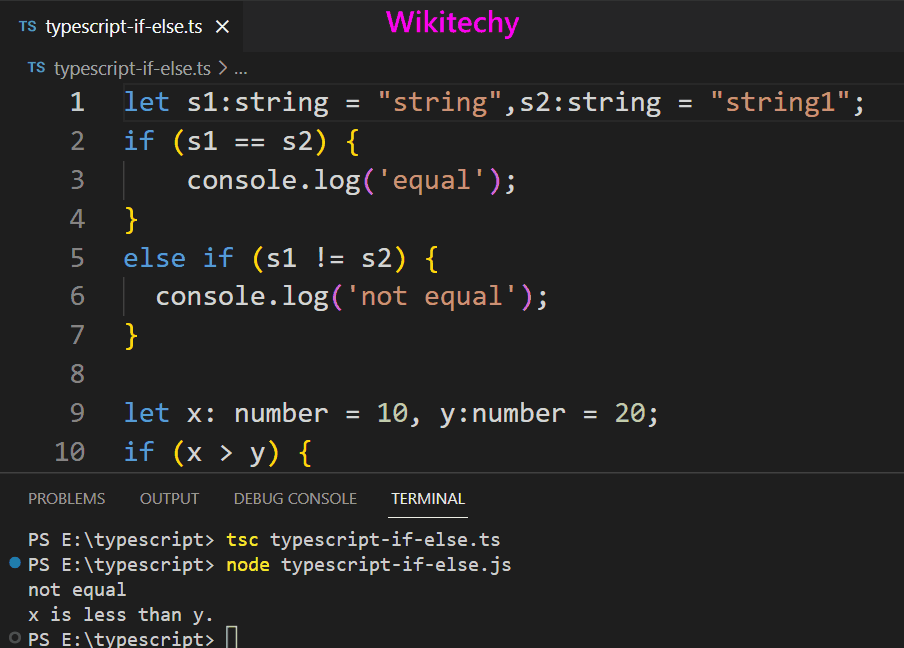
Example 1
let s1:string = "string",s2:string = "string1";
if (s1 == s2)
{
console.log('equal');
}
else if (s1 != s2)
{
console.log('not equal');
}
Output
not equal
Example 2
let x: number = 10, y = 20;
if (x > y) {
console.log('x is greater than y.');
}
else if (x < y){
console.log('x is less than y.');
}
else{
console.log('x is equal to y');
}
Output
x is less than y
Typescript For Loop
- In TypeScript, a "for" loop is a control flow statement used to iterate over a block of code repeatedly for a specified number of times or based on a specific condition.
- It allows you to execute a block of code multiple times, typically with a counter variable that keeps track of the loop iterations.
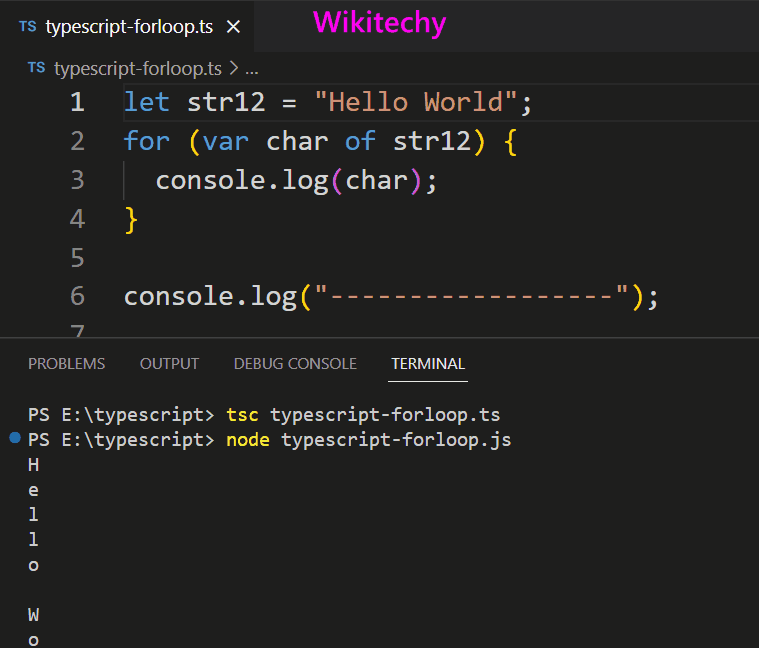
Example 1
let str12 = "Hello World";
for (var char of str12) {
console.log(char);
}
Output
H e l l o W o r l d
Example 2
let arr = [10, 20, 30, 40];
for (var index in arr) {
console.log(index);
console.log(arr[index]);
}
Output
0, 1, 2, 3
10, 20, 30, 40
Example 3
let arr1 = ["a", "b", "c", "d"];
for (var index1 in arr1) {
console.log(index1);
console.log(arr1[index1]);
}
Output
0, 1, 2, 3
a, b, c, d
Typescript While Loop
- A while loop is a control flow statement that allows you to repeatedly execute a block of code as long as a specified condition is true. The loop consists of a condition expression and a code block.
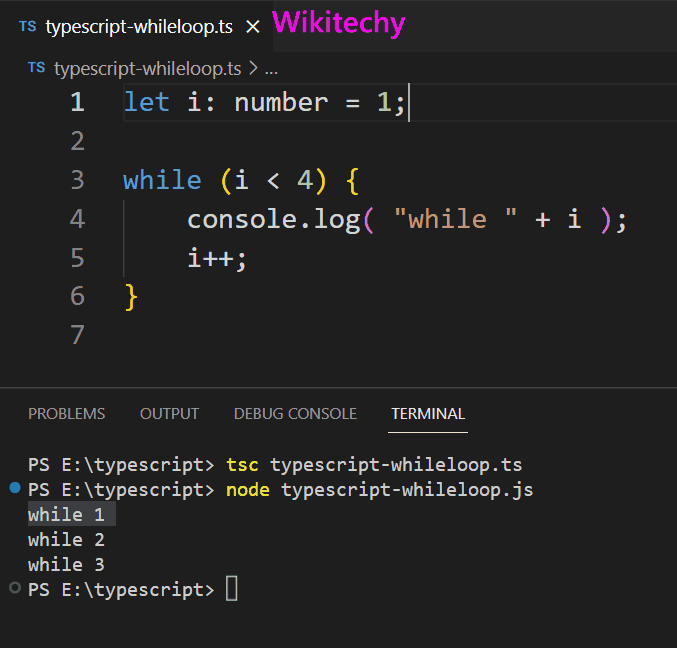
Example 1
let i: number = 1;
while (i < 4) {
console.log( "while " + i );
i++;
}
Output
while 1
while 2
while 3
Typescript dowhile loop
- A do-while loop is a control flow statement that executes a block of code once before checking the loop condition. It continues to execute the code block as long as the specified condition remains true.
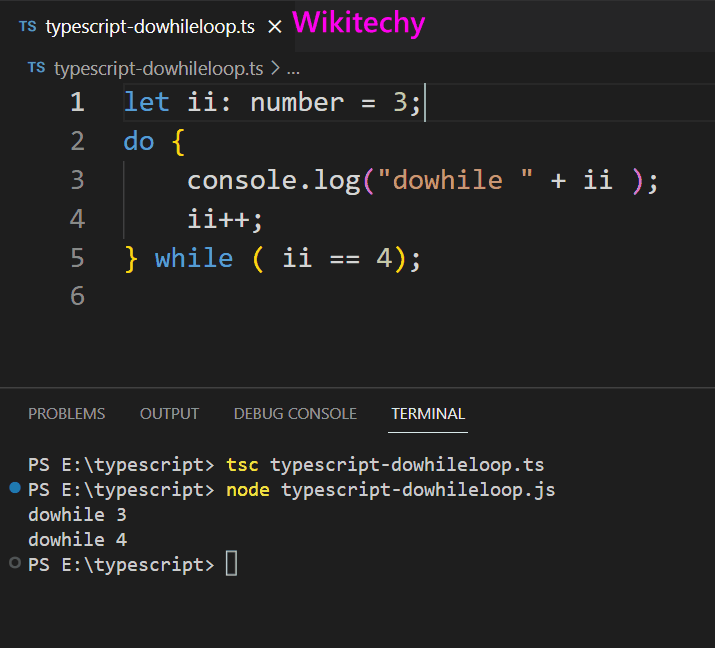
Example 1
let ii: number = 3;
do {
console.log("dowhile " + ii );
ii++;
} while ( ii == 4);
Output
dowhile 3
dowhile 4
Typescript Function
- In TypeScript, a function is a block of code that performs a specific task. It can accept input values (parameters), perform operations, and optionally return a result.
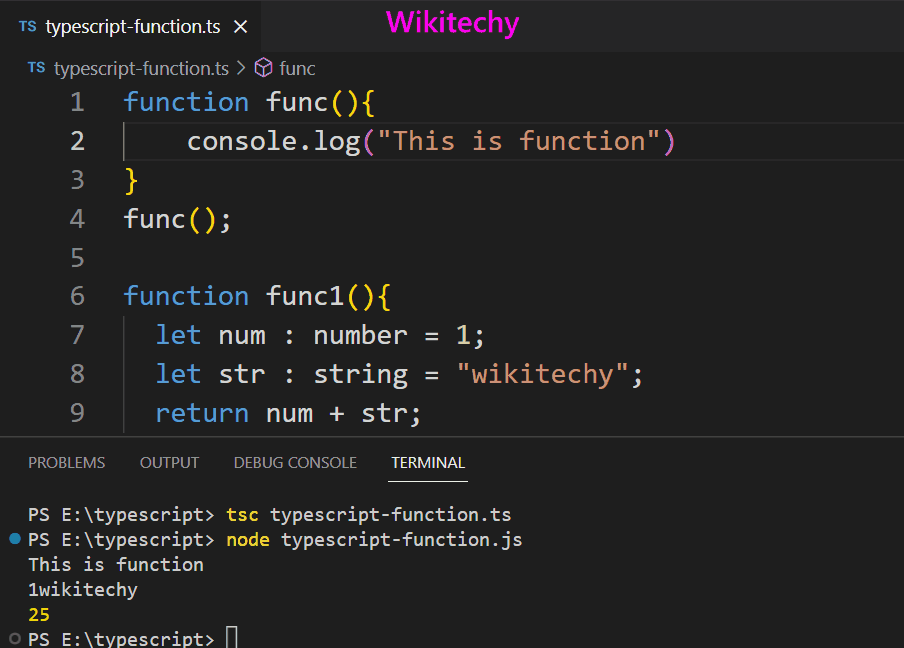
Example 1
function func(){
console.log("This is function")
}
func();
Output
This is function
Example 2
function func1(){
let num : number = 1;
let str : string = "wikitechy";
return num + str;
}
console.log(func1());
Output
1wikitechy
Example 3
function addTwo(a: number, b: number): number{
return a + b;
}
console.log(addTwo(10,15));
Output
25