What is ExpressJS - ExpressJS Tutorial
What is ExpressJS
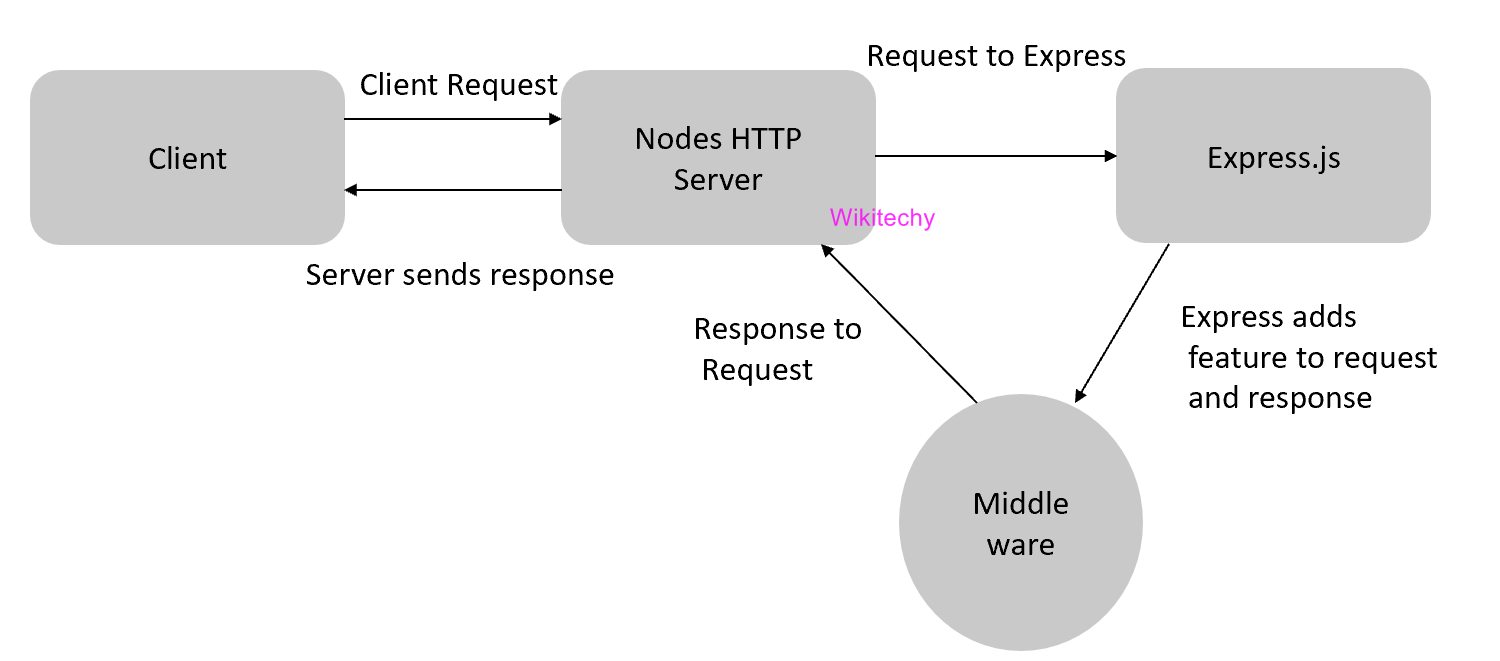
ExpressJs
- It is a web framework for Node.js which is fast, robust and asynchronous in nature.
- We can assume express as a layer built on the top of the Node.js that helps manage a server and routes.
- It provides a robust set of features to develop mobile and web applications.
Features of ExpressJS
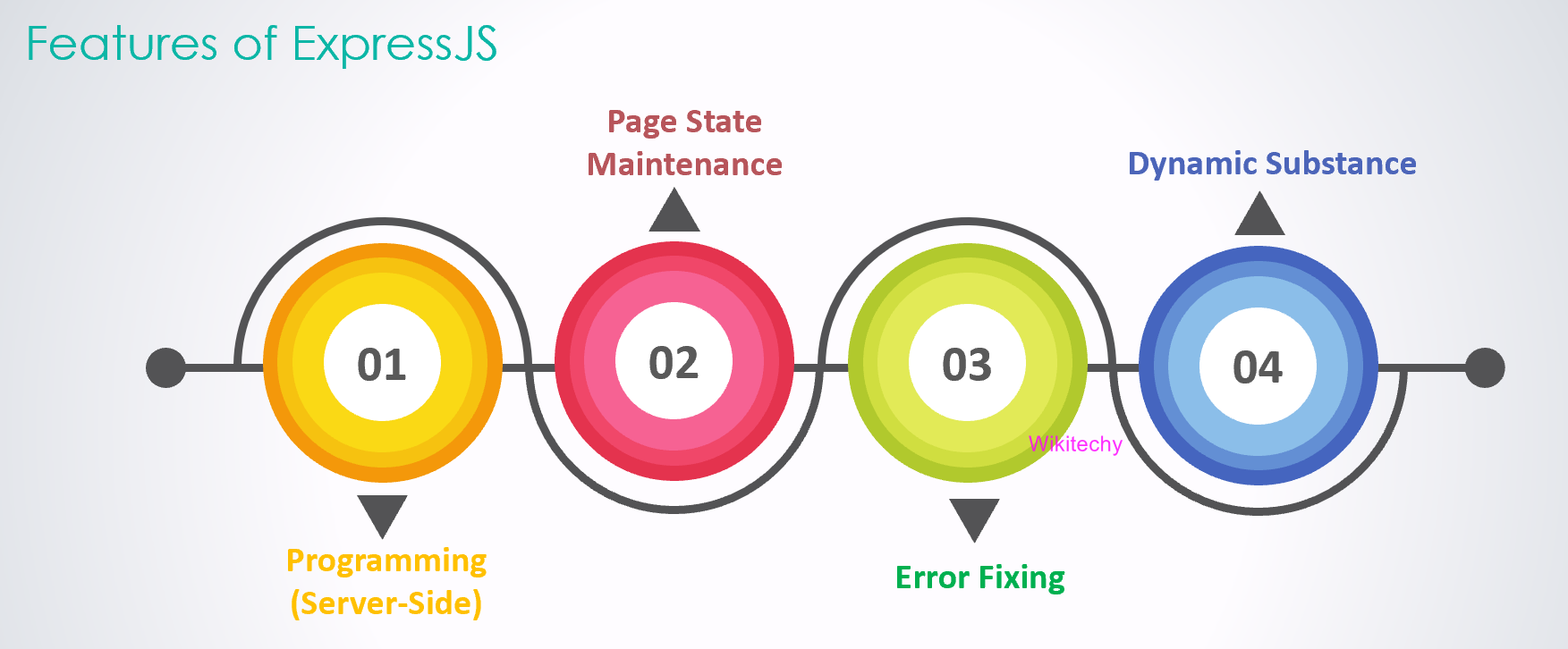
Features of ExpressJS
Programming (Server-side)
- In this the key element of Express.js is that it utilizes numerous Node.js features to call functions anywhere.
- In NodeJS numerous tasks that take various lines of code and long periods of programming can be written in a smaller number of lines and in almost no time.
Page State Maintenance
- It permits protecting page state utilizing routing through their URLs.
- We can impart this URL to various clients, regardless of whether we make changes, these URLs will take the client to a similar page state it had when you shared it.
Error Fixing
- At any application bugs and blunders can make the entire application to collapse.
Dynamic Substance
- Templating engine of Express.js offers the arrangement of having a dynamic substance on the website page utilizing HTML layouts.
- It removes a huge amount of weight from the customer side that manages hardware specifications as well.
Basic Example for ExpressJS
var express = require('express'); var app = express(); app.get('/', function (req, res) { res.send('Welcome to Wikitechy!'); }); var server = app.listen(8000, function () { var host = server.address().address; var port = server.address().port; console.log('Example app listening at http://%s:%s', host, port); });
Output
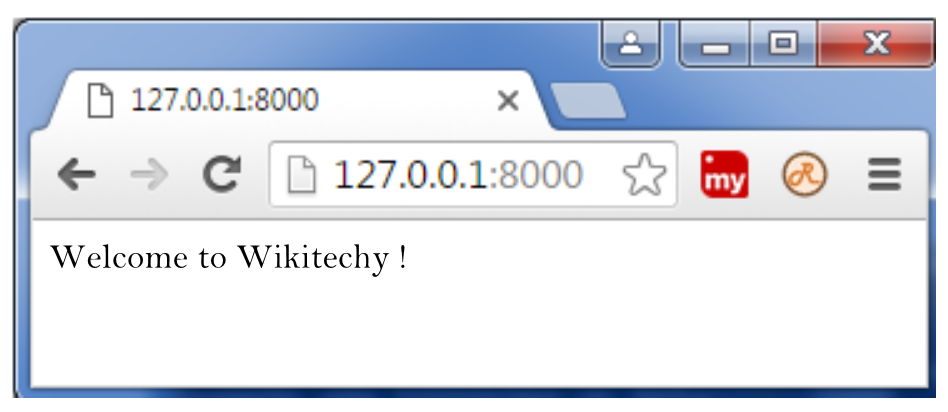
Express.js Request Object
- Response objects and Express.js Request are the parameters of the callback function which is used in Express applications.
- The express.js request object represents the HTTP request and has properties for the request query string, body, parameters, HTTP headers and so on.
Syntax
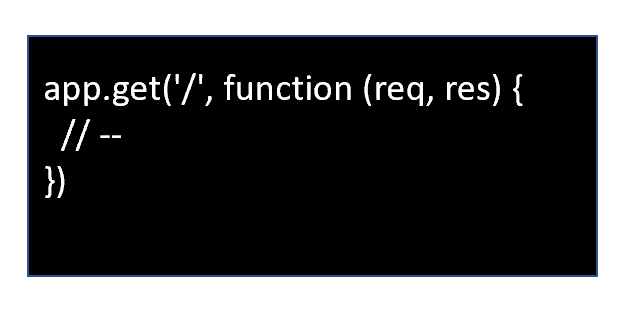
Request Object Methods
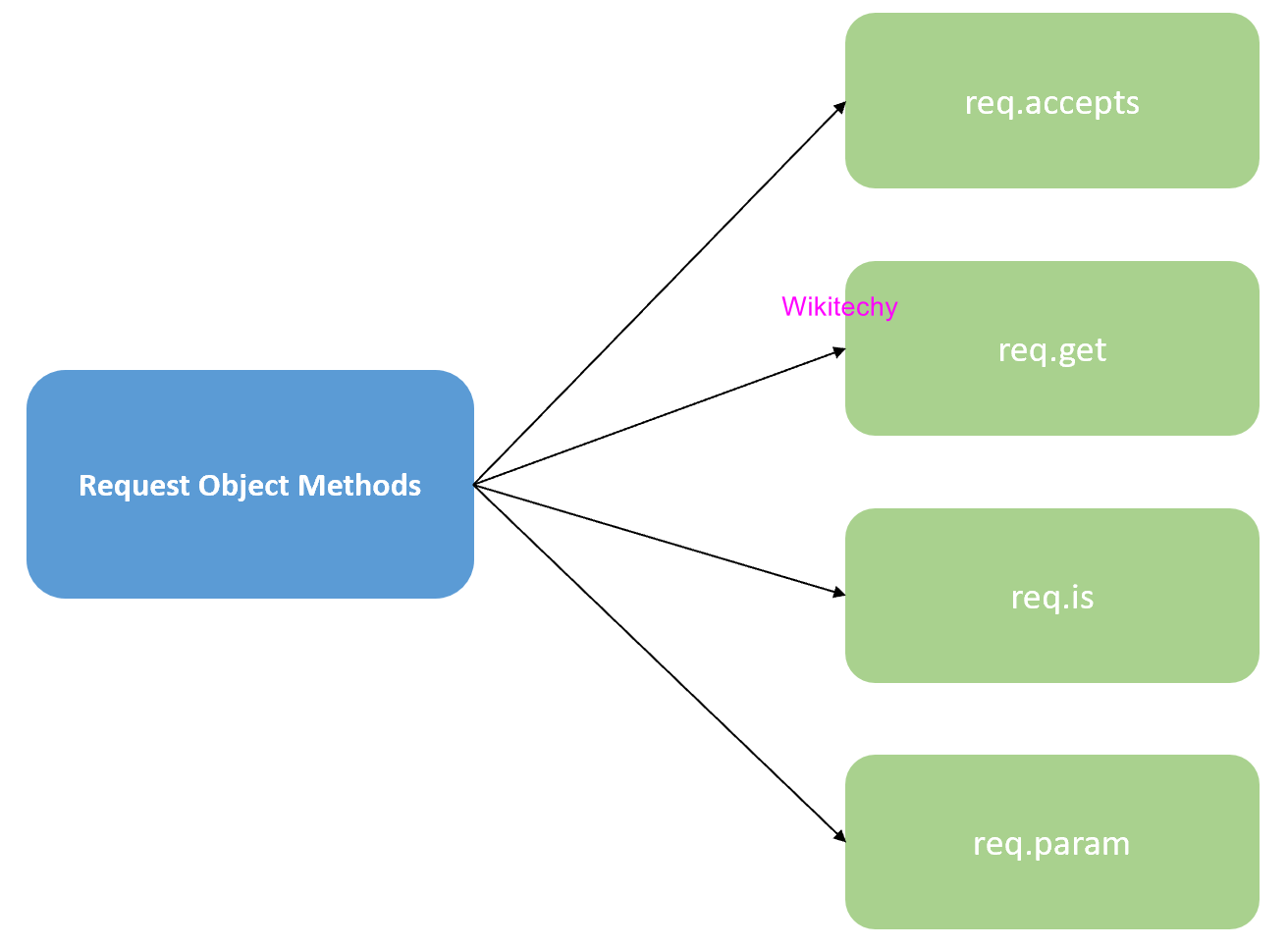
req.accepts (types)
- It is used to check whether the specified content types are acceptable, based on the request's Accept HTTP header field.
Example
req.accepts('html'); //=>?html? req.accepts('text/html'); // => ?text/html?
req.get(field)
- It returns the specified HTTP request header field.
Example
req.get('Content-Type'); // => "text/plain" req.get('content-type'); // => "text/plain" req.get('Something'); // => undefined
req.is(type)
- It returns true if the incoming request's "Content-Type" HTTP header field matches the MIME type specified by the type parameter.
Example
// With Content-Type: text/html; charset=utf-8 req.is('html'); req.is('text/html'); req.is('text/*'); // => true
req.param(name [, defaultValue])
- It is used to fetch the value of param name when present.
Example
// ?name=sasha req.param('name') // => "sasha" // POST name=sasha req.param('name') // => "sasha" // /user/sasha for /user/:name req.param('name') // => "sasha"
Express.js Response Object
- It specifies HTTP response which is sent by an Express app when it gets an HTTP request.
- It facilitates you to put new cookies value and that will write to the client browser under cross domain rule and under cross domain rule.
- Once we res.send() or res.redirect() or res.render(), we cannot do it again, otherwise, there will be uncaught error.
Response Object Properties
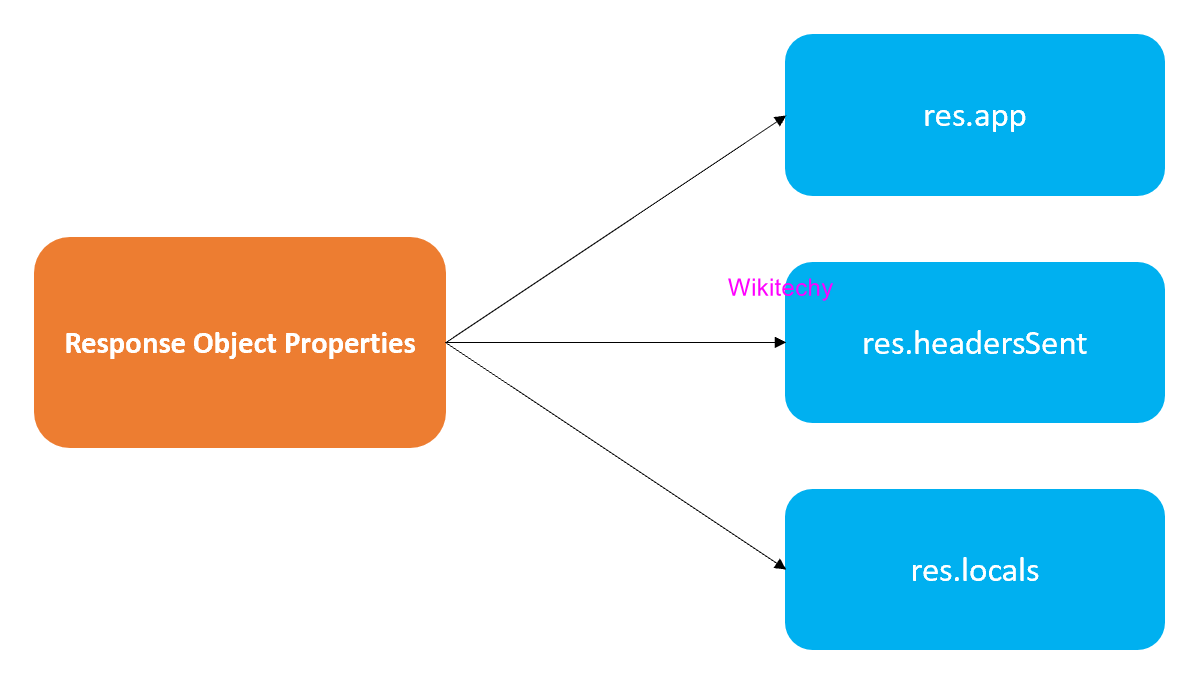
res.app
- It holds a reference to the instance of the express application that is using the middleware.
res. headersSent
- It is a Boolean property that indicates if the app sent HTTP headers for the response.
res. locals
- It specifies an object that contains response local variables scoped to the request.
Express.js GET Request
- POST and GET both are two common HTTP requests used for building REST API's.
- POST requests are used to send large amount of data because data is sent in the body while GET requests are used to send only limited amount of data because data is sent into header.
- Express.js facilitates us to handle GET and POST requests using the instance of express.
For Example, index.html
<html>
<body>
<form action="http://127.0.0.1:8081/process_get" method="GET">
First Name: <input type="text" name="first_name"> <br>
Last Name: <input type="text" name="last_name">
<input type="submit" value="Submit">
</form>
</body>
</html>
get_example1.js
var express = require('express');
var app = express();
app.use(express.static('public'));
app.get('/index.html', function (req, res) {
res.sendFile( __dirname + "/" + "index.html" );
})
app.get('/process_get', function (req, res) {
response = {
first_name:req.query.first_name,
last_name:req.query.last_name
};
console.log(response);
res.end(JSON.stringify(response));
})
var server = app.listen(8000, function () {
var host = server.address().address
var port = server.address().port
console.log("Example app listening at http://%s:%s", host, port)
})
Output
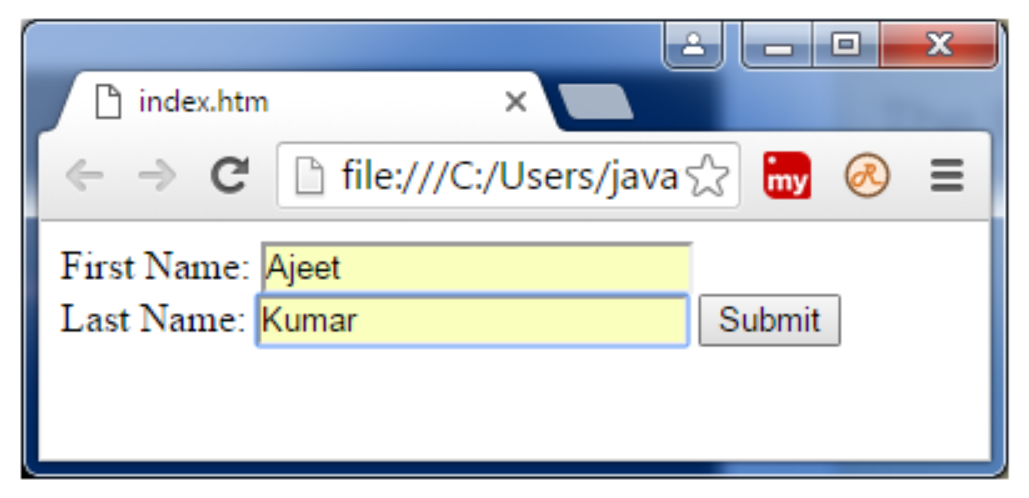
Express.js Cookies
- Cookies are small piece of information were sent from a website and stored in user's web browser when user browses that website.
- Always the user loads that website back, the browser sends that stored data back to website or server, to recognize user.
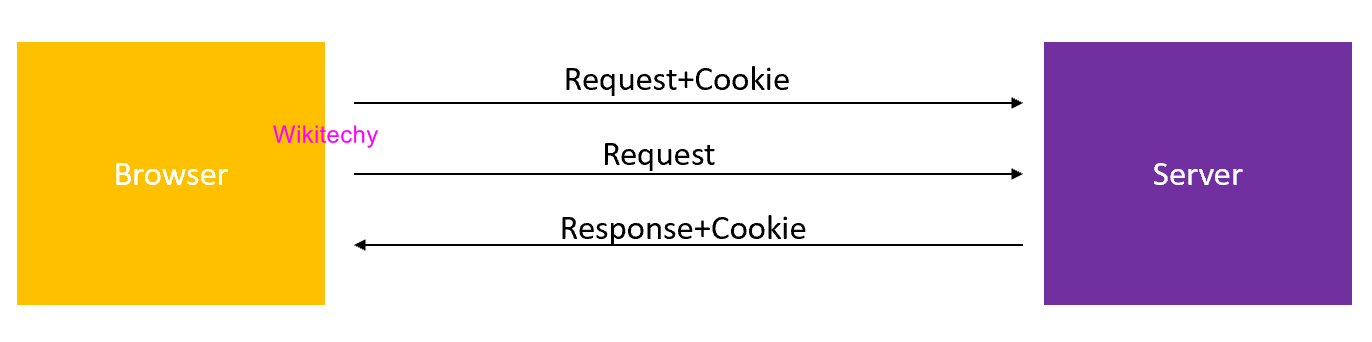
Define a route
- Cookie-parser parses Cookie header and populate req.cookies with an object keyed by the cookie names.
- Every time when it requests that website, browser sends back that cookie to the server.
For Example, Express.js Cookies
var express = require('express'); var cookieParser = require('cookie-parser'); var app = express(); app.use(cookieParser()); app.get('/cookieset',function(req, res){ res.cookie('cookie_name', 'cookie_value'); res.cookie('company', 'javatpoint'); res.cookie('name', 'sonoo'); res.status(200).send('Cookie is set'); }); app.get('/cookieget', function(req, res) { res.status(200).send(req.cookies); }); app.get('/', function (req, res) { res.status(200).send('Welcome to Wikitechy!'); }); var server = app.listen(8000, function () { var host = server.address().address; var port = server.address().port; console.log('Example app listening at http://%s:%s', host, port); });
Output
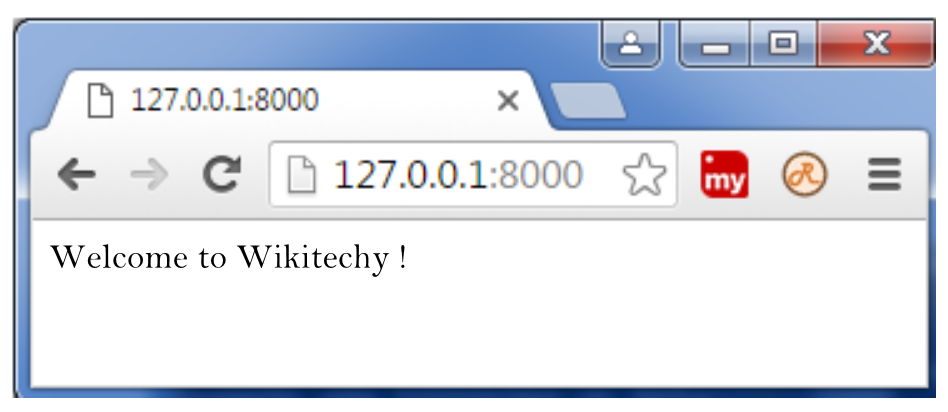
Express.js Middleware
- It is a different type of functions that are invoked by the Express.js routing layer before the final request handler.
- As the name specified, Middleware appears in the middle between an initial request and final intended route.
- At stack, middleware functions are always invoked in the order in which they are added.
- It is commonly used to perform tasks like body parsing for URL-encoded or JSON requests, cookie parsing for basic cookie handling, or even building JavaScript modules on the fly.
- They are the functions that access to the request and response object (req, res) in request-response cycle.
- It can execute any code and make changes to the request and the response objects.
- It can call the next middleware function in the stack and end the request-response cycle.
Following is a list of possibly used middleware in Express.js app
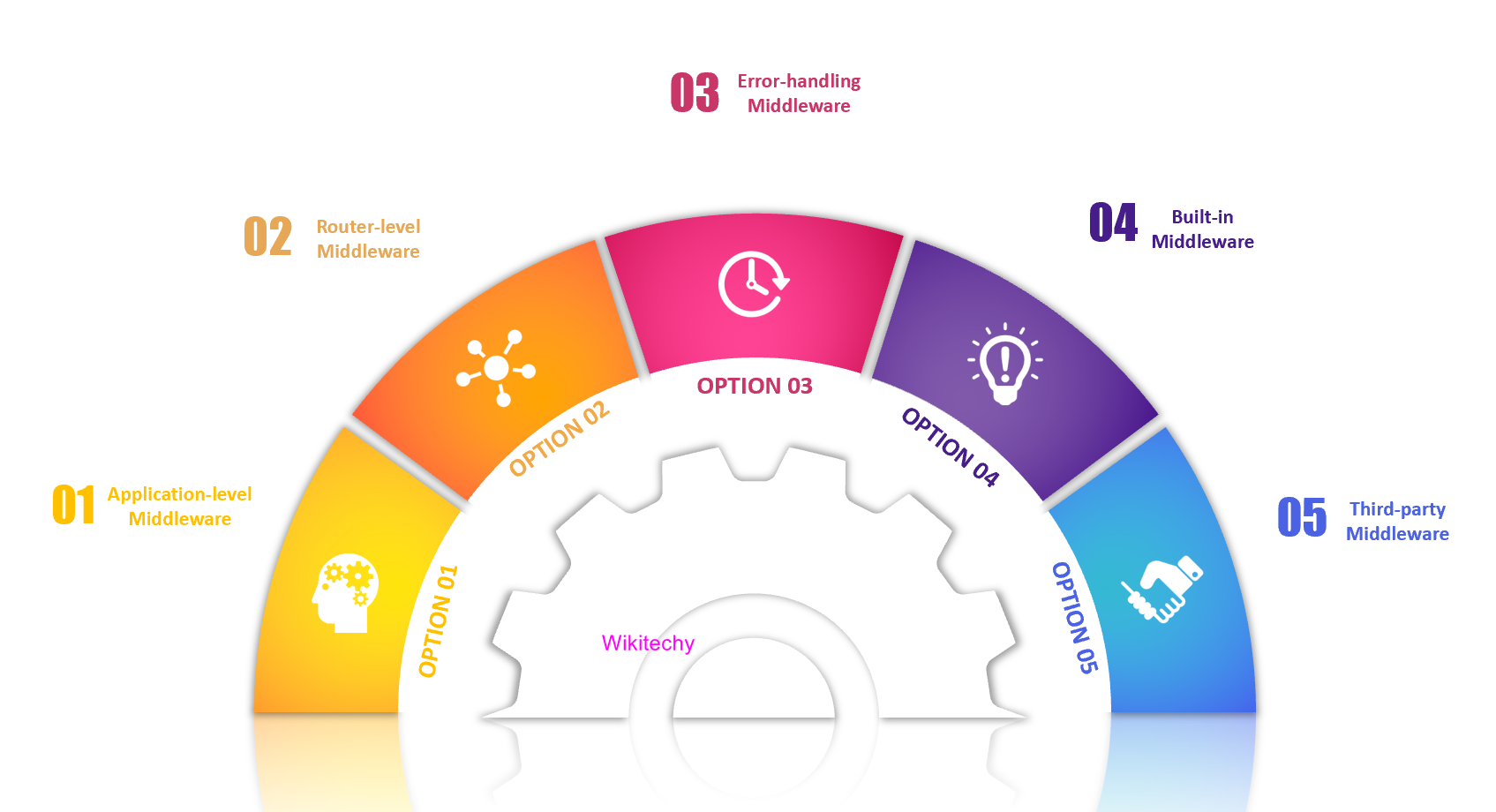
For Example,
var express = require('express'); var app = express(); app.get('/', function(req, res) { res.send('Welcome to Wikitechy!'); }); app.get('/help', function(req, res) { res.send('How can I help You?'); }); var server = app.listen(8000, function () { var host = server.address().address var port = server.address().port console.log("Example app listening at http://%s:%s", host, port) })
Output-1
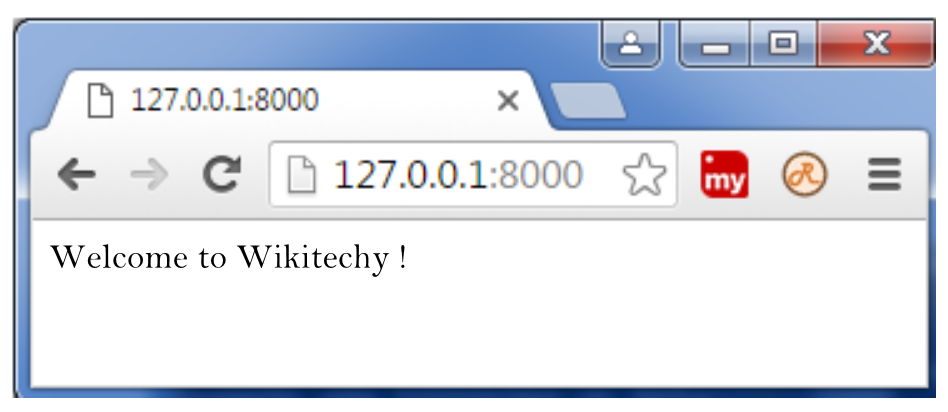
Output-2
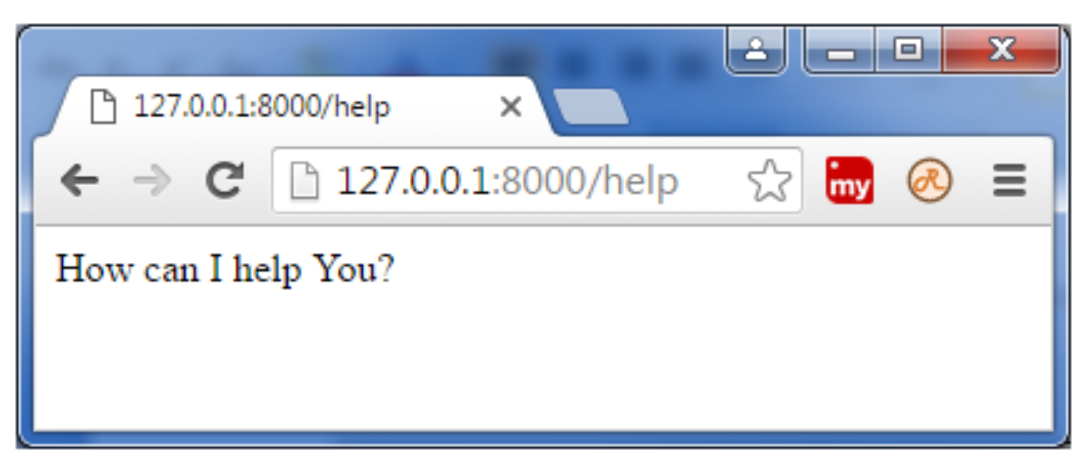
Express.js Scaffolding
- Scaffolding is a technique that is supported by some MVC frameworks.
- It is mainly supported by the following frameworks Ruby on Rails, OutSystems Platform, Express Framework, Play framework, Django, Monorail, Brail, Symfony, Laravel, CodeIgniter, Yii, CakePHP, Phalcon PHP, Model-Glue, PRADO, Grails, Catalyst, Seam Framework, Spring Roo, ASP.NET etc.
- It facilitates the programmers to specify how the application data may be used.
- This specification is used by the frameworks with predefined code templates, to generate the final code that the application can use for CRUD (Create, Read, Update, Delete) operations.
Scaffolding Installation
npm install express-scaffold
- Execute the following command to install express generator, after previous steps :
npm install -g express-generator