Write a C function to reverse a given Doubly Linked List
See below diagrams for example.
(a) Original Doubly Linked List
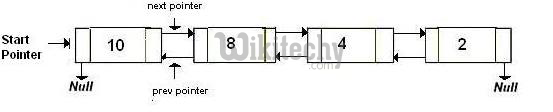
(b) Reversed Doubly Linked List
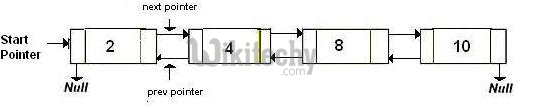
Here is a simple method for reversing a Doubly Linked List. All we need to do is swap prev and next pointers for all nodes, change prev of the head (or start) and change the head pointer in the end.\
[ad type=”banner”]
Java programming:
class LinkedList {
static Node head;
static class Node {
int data;
Node next, prev;
Node(int d) {
data = d;
next = prev = null;
}
}
void reverse() {
Node temp = null;
Node current = head;
while (current != null) {
temp = current.prev;
current.prev = current.next;
current.next = temp;
current = current.prev;
}
if (temp != null) {
head = temp.prev;
}
}
void push(int new_data) {
Node new_node = new Node(new_data);
new_node.prev = null;
new_node.next = head;
if (head != null) {
head.prev = new_node;
}
head = new_node;
}
void printList(Node node) {
while (node != null) {
System.out.print(node.data + " ");
node = node.next;
}
}
public static void main(String[] args) {
LinkedList list = new LinkedList();
list.push(2);
list.push(4);
list.push(8);
list.push(10);
System.out.println("Original linked list ");
list.printList(head);
list.reverse();
System.out.println("");
System.out.println("The reversed Linked List is ");
list.printList(head);
}
}
Time Complexity: O(n)
We can also swap data instead of pointers to reverse the Doubly Linked List. Method used for reversing array can be used to swap data. Swapping data can be costly compared to pointers if size of data item(s) is more.
[ad type=”banner”]