C program remove spaces or blanks from a string, For example consider the string
"c programming"
There are two spaces in this string, so our program will print a string
“c programming”. It will remove spaces when they occur more than one time consecutively in string anywhere.
[ad type=”banner”]
C programming code
#include <stdio.h>
int main()
{
char text[1000], blank[1000];
int c = 0, d = 0;
printf("Enter some text\n");
gets(text);
while (text[c] != '\0') {
if (text[c] == ' ') {
int temp = c + 1;
if (text[temp] != '\0') {
while (text[temp] == ' ' && text[temp] != '\0') {
if (text[temp] == ' ') {
c++;
}
temp++;
}
}
}
blank[d] = text[c];
c++;
d++;
}
blank[d] = '\0';
printf("Text after removing blanks\n%s\n", blank);
return 0;
}
If you want you can copy blank into text string so that original string is modified.
Output of program:
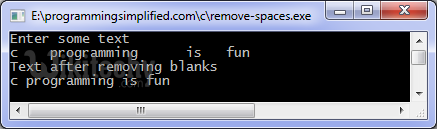
C programming code using pointers
[ad type=”banner”]
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#define SPACE ' '
char *process(char*);
int main()
{
char text[1000], *r;
printf("Enter a string\n");
gets(text);
r = process(text);
printf("\"%s\"\n", r);
free(r);
return 0;
}
char *process(char *text) {
int length, c, d;
char *start;
c = d = 0;
length = strlen(text);
start = (char*)malloc(length+1);
if (start == NULL)
exit(EXIT_FAILURE);
while (*(text+c) != '\0') {
if (*(text+c) == ' ') {
int temp = c + 1;
if (*(text+temp) != '\0') {
while (*(text+temp) == ' ' && *(text+temp) != '\0') {
if (*(text+temp) == ' ') {
c++;
}
temp++;
}
}
}
*(start+d) = *(text+c);
c++;
d++;
}
*(start+d)= '\0';
return start;
}
Super concept
it is really amazing!